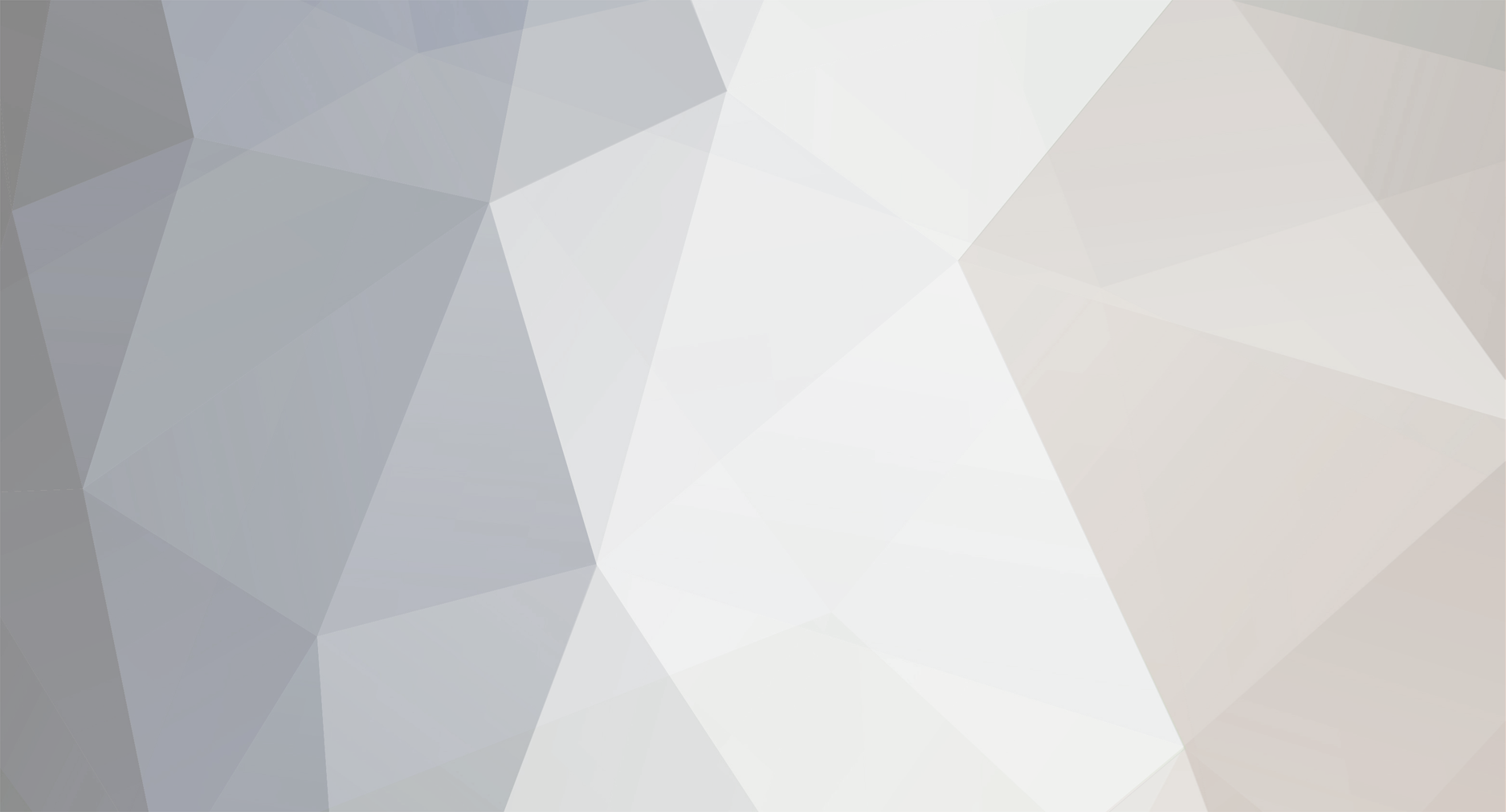
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
Was bored: <?php $eqn = '-2x^2+32-2x=3x+2x-32-x^2'; function rearrange($eqn){ $sides = explode('=',$eqn); $coefficients = array(); foreach($sides as $k => $v){ preg_match_all('%(\-|\+)?[0-9x^]+%s',$v,$terms); foreach($terms[0] as $v2){ //work out which term is being used if(strpos($v2, 'x^2') !== false){ $key = 0; }elseif(strpos($v2, 'x') !== false){ $key = 1; }else{ $key = 2; } //work out the coefficient preg_match('%^(\-|\+)?[0-9]+%s',$v2,$matches); if(empty($matches)){ if($v2[0] == '-'){ $num = -1; }else{ $num = 1; } }else{ $num = $matches[0]; } @$coefficients[$k][$key] += $num;//+= because terms may not be collected } } for($x=0;$x<=2;$x++){ //collect terms. Surpress error since coeffiecent may not be set @$rearranged[$x] = $coefficients[0][$x] - $coefficients[1][$x]; } return $rearranged; } function solve($coefficients){ list($a,$b,$c) = $coefficients; $discriminant = pow($b,2) - 4*$a*$c; if($discriminant > 0){ $roots[0] = (-$b + sqrt($discriminant))/(2*$a); $roots[1] = (-$b - sqrt($discriminant))/(2*$a); }elseif($discriminant == 0){ $roots[0] = -$b/(2*$a); }else{ $real = -$b/(2*$a); $imaginary = sqrt(-$discriminant)/(2*$a); $roots[0] = $real.' + '.$imaginary.'i'; $roots[1] = $real.' - '.$imaginary.'i'; } return $roots; } print_r(solve(rearrange($eqn))); ?> Will work as long as there's nothing unexpected in the input.
-
It wouldn't be that difficult to have it rearranged and solved.
-
Yeah, very easy - just use the quadratic formula. Check the discriminant to work out if you're going to get any real answers, then plug the values of a,b and c into the formula. You could set it up to give you non-real answers too.
-
Storing the salt in your database?! The whole point of salting is that people can't brute force the password with the encyrpted password - if someone's able to get the encrypted password from your database, they're more than likely able to grab the salt too!
-
Indeed. The main security issue with sessions occurs when you allow the session ID to be passed around in the URL. What happens is that people may give someone else a link, not realising their session ID is in that link. The person that recieves the link then becomes logged in. Hence why this option is generally disabled.
-
Yes. It's best you make the salt of a reasonable length and of mixed characters.
-
Sessions: stored on the server Cookies: stored on the client's machine. If you would like people to remain logged in whever they come to your website, use cookies. Otherwise use sessions. Sessions usually set a cookie however, to track the session ID. This can be parsed around in the URL though. As was mentioned, it's probably best you do some reading.
-
The point of using the md5() hash twice is the same as salting - if someone has the hash, then brute force is harder and rainbow tables are useless. As I mentioned earlier however, there appears to be some debate as to wether it's a good idea. I'd recommend adding a salt anyway.
-
Pretty much - you're selecting the number of rows that match, and your giving that the name 'counter' in your record set. Personally i'd just use the one query: <?php $query = "SELECT * FROM user WHERE id not in (" . $seenIds . ") ORDER BY RAND() LIMIT 1"; $result = mysql_query($query); $num = mysql_num_rows($result); if($num > 0) { $row = mysql_fetch_assoc($result); echo $row['name']; echo $row['age']; } ?>
-
[SOLVED] I need some guidance on comparing dates
GingerRobot replied to jim.davidson's topic in PHP Coding Help
When comparing dates, convert them to unix timestamps(the number of seconds since 1st Jan 1970) first: <?php $date_written = $row_GetArticle['date_written']; $timestamp = strtotime($date_written); $now = time(); if($now-$timestamp > 60*60*24*365){ echo 'Article was written more than a year ago'; }else{ echo 'Article is less than a year old'; } ?> -
How about posting the code you've tried and what happened when you did.
-
1.) The file system functions such as fwrite and fread will be needed: http://uk2.php.net/manual/en/ref.filesystem.php 2.) These tutorials may be of interest to you: http://www.phpfreaks.com/forums/index.php/topic,186690.0.html http://www.phpfreaks.com/forums/index.php/topic,186697.0.html http://www.phpfreaks.com/forums/index.php/topic,186705.0.html
-
How's about: <?php $fraction = '5/16'; list($numerator,$denominator) = explode('/',$fraction); $decimal = $numerator/$denominator; echo $decimal; ?> Or we can juse use the eval() function: <?php $fraction = '5/16'; eval("\$decimal=$fraction;"); echo $decimal; ?>
-
[SOLVED] Multiple Drop Down, convert from php 4 to 5?
GingerRobot replied to aeafisme23's topic in PHP Coding Help
The reason why it now works is that you're no longer trying to make use of a variable that hasn't been previously defined. The reason why it worked with php4 was that it was setup to ignore notices. That is, the error was still there, but PHP can deal with it. It tends to lend to messy code and can make typos much harder to find though, so it's generally better to work with notices on. I guessed that it was not needed since the code previously did what was expected, whilst dealing with the error itself. -
Meh, i wish they'd just drop the short tags. Would save a hell of a lot of confusion. Not only that, but it's a pain when people use them on the forum in tags, since we don't get syntax highlighting!
-
Yes, of course -any salting does not prevent brute force attacks. However, if someone is going to try and brute force though your system that adds the salt, then its going to take a hell of a long time. Sure, writing a program to brute force may not - but adding in the requests to an external server will. As for the idea of using the MD5 algorithm twice - i occassionaly see it mentioned that this is infact less secure than a single use of the MD5. I think this tends to be talked about with reference to hash collisions. That said, i've never seen anything which proves this or otherwise - and it certainly seems counter intuitive to me. Anyone have any thoughts on that?
-
[SOLVED] Multiple Drop Down, convert from php 4 to 5?
GingerRobot replied to aeafisme23's topic in PHP Coding Help
I think you just need to remove all reference to $sel in makeSelect3. Did you try the version of the function that i posted? -
That's because the decimal separator that you need to use is a point and not a comma. PHP doesn't recognise the elements of your array as numbers, so tries to convert them to a number. What it ends up doing is taking everything up to the first non-numeric character as being the number.
-
[SOLVED] Multiple Drop Down, convert from php 4 to 5?
GingerRobot replied to aeafisme23's topic in PHP Coding Help
Im not sure i entirely follow your code, but the problem is that $sel is never defined in your makeSelect3 function. If this was working correctly prior to the upgrade, i suggest you remove the reference to it: <?php function makeSelect3($opts, $opt1, $opt2) { echo "<SELECT name='option3' onchange='this.form.submit()'>"; echo "<option value=''>- select 3rd choice -</option>"; if ($opt1 && $opt2) { unset($opts[$opt1]); //remove 1st choice unset($opts[$opt2]); //remove 2nd choice foreach ($opts as $v => $t) { echo "<option value='$v'>$t</option>"; } } echo '</SELECT>'; } ?> -
Sorry, there was a stray dollar sign in there. Try: <?php //Extra componenten declareren function extraComponenten(){ $ECaantal = $_POST["aantalextra"]; for($i = 0;$i <= $ECaantal;$i++){ $extracomponent = $_POST["extracomponent".$i]; $ECtype[$i] = substr($extracomponent, 0, 6); $deel = explode(" - ", $extracomponent); $ECprijs[$i] = $deel[1]; return $ECprijs; } } //Prijs uit string halen function prijs(){ $product[0] = $_POST["processor"]; $product[1] = $_POST["moederbord"]; $product[2] = $_POST["hardeschijf1"]; $product[3] = $_POST["hardeschijf2"]; $product[4] = $_POST["geheugen"]; $product[5] = $_POST["cddvdstation1"]; $product[6] = "AMD456 - 0,00"; //$product[6] = $_POST["cddvdstation2"]; $product[7] = $_POST["grafischekaart"]; $product[8] = $_POST["voeding"]; $product[9] = $_POST["tvkaart"]; $product[10] = $_POST["geluidskaart"]; $product[11] = $_POST["netwerkkaart"]; $product[12] = $_POST["diskettestation"]; $product[13] = $_POST["kaartlezer"]; $product[14] = $_POST["monitor"]; for($i = 0;$i < 15;$i++){ $deel = explode(" - ", $product[$i]); $prijs[$i] = $deel[1]; } $geheugenaantal = $_POST["geheugenaantal"]; $prijs[4] = $prijs[4] * $geheugenaantal; echo $prijs[4]; return $prijs; } //Totaal berekenen function totaalBerekenen(){ $ECprijs = extraComponenten(); $prijs = prijs(); $totaal = array_sum($ECprijs) + array_sum($prijs); echo $totaal; } if(isset($_POST["totaal"])){ totaalBerekenen(); } ?>
-
That's not the kind of thing you should be looking to store - just something to calculate when you should need it: SELECT COUNT(*) FROM tbl WHERE cat=2
-
Or, if you know you want the last field: <?php print "<table width=\"800\">"; print "<tr>"; $num_fields = mysql_num_fields($data); for($i = 0;$i < $num_fields;$i++) { print "<th>" . mysql_field_name($data, $i) . "</th>"; } print "</tr>"; for ($i = 0; $i < mysql_num_rows($data); $i++) { print "<tr>"; $row = mysql_fetch_row($data); for($j = 0;$j < $num_fields;$j++) { if($j = $num_fields){//last column if($row[$j] >= 2.9999) $class = 'red'; elseif($row[$j] >= 1.5) $class = 'orange'; else $class = 'green'; }else{ $class = ''; } echo("<td class=\"$class\">" . $row[$j] . "</td>"); } print "</tr>"; } print "</table>"; ?> <?php print "<table width=\"800\">"; print "<tr>"; for($i = 0;$i < mysql_num_fields($data);$i++) { print "<th>" . mysql_field_name($data, $i) . "</th>"; } print "</tr>"; for ($i = 0; $i < mysql_num_rows($data); $i++) { print "<tr>"; $row = mysql_fetch_row($data); for($j = 0;$j < mysql_num_fields($data);$j++) { if($row[$j] >= 2.9999) { echo("<td class=\"red\">" . $row[$j] . "</td>"); } else { echo("<td>" . $row[$j] . "</td>"); } } print "</tr>"; } print "</table>"; ?>
-
Have your first two functions return their respective arrays. Otherwise the variables are undefined: <?php //Extra componenten declareren function extraComponenten(){ $ECaantal = $_POST["aantalextra"]; for($i = 0;$i <= $ECaantal;$i++){ $extracomponent = $_POST["extracomponent".$i]; $ECtype[$i] = substr($extracomponent, 0, 6); $deel = explode(" - ", $extracomponent); $ECprijs[$i] = $deel[1]; return $ECprijs; } } //Prijs uit string halen function prijs(){ $product[0] = $_POST["processor"]; $product[1] = $_POST["moederbord"]; $product[2] = $_POST["hardeschijf1"]; $product[3] = $_POST["hardeschijf2"]; $product[4] = $_POST["geheugen"]; $product[5] = $_POST["cddvdstation1"]; $product[6] = "AMD456 - 0,00"; //$product[6] = $_POST["cddvdstation2"]; $product[7] = $_POST["grafischekaart"]; $product[8] = $_POST["voeding"]; $product[9] = $_POST["tvkaart"]; $product[10] = $_POST["geluidskaart"]; $product[11] = $_POST["netwerkkaart"]; $product[12] = $_POST["diskettestation"]; $product[13] = $_POST["kaartlezer"]; $product[14] = $_POST["monitor"]; for($i = 0;$i < 15;$i++){ $deel = explode(" - ", $product[$i]); $prijs[$i] = $deel[1]; } $geheugenaantal = $_POST["geheugenaantal"]; $prijs[4] = $prijs[4] * $geheugenaantal; echo $prijs[4]; return $prijs } //Totaal berekenen function totaalBerekenen(){ $ECprijs = extraComponenten(); $$prijs = prijs(); $totaal = array_sum($ECprijs) + array_sum($prijs); echo $totaal; } if(isset($_POST["totaal"])){ totaalBerekenen(); } ?>
-
Yes that's true - but you are still making a request to the server. In my view, the including of files is therefore a server side operation.
-
You can add in the line: curl_setopt($datax_ch, CURLOPT_SSL_VERIFYPEER, FALS; Which should fix the issue. Of course, this does give some potential security issues given that you're no longer interested in the SSL certificate. But if you're not sending any sensitive information, I dont suppose it will be an issue. Otherwise, you may have luck manipulating some of the SSL related options listed here: http://uk.php.net/curl_setopt