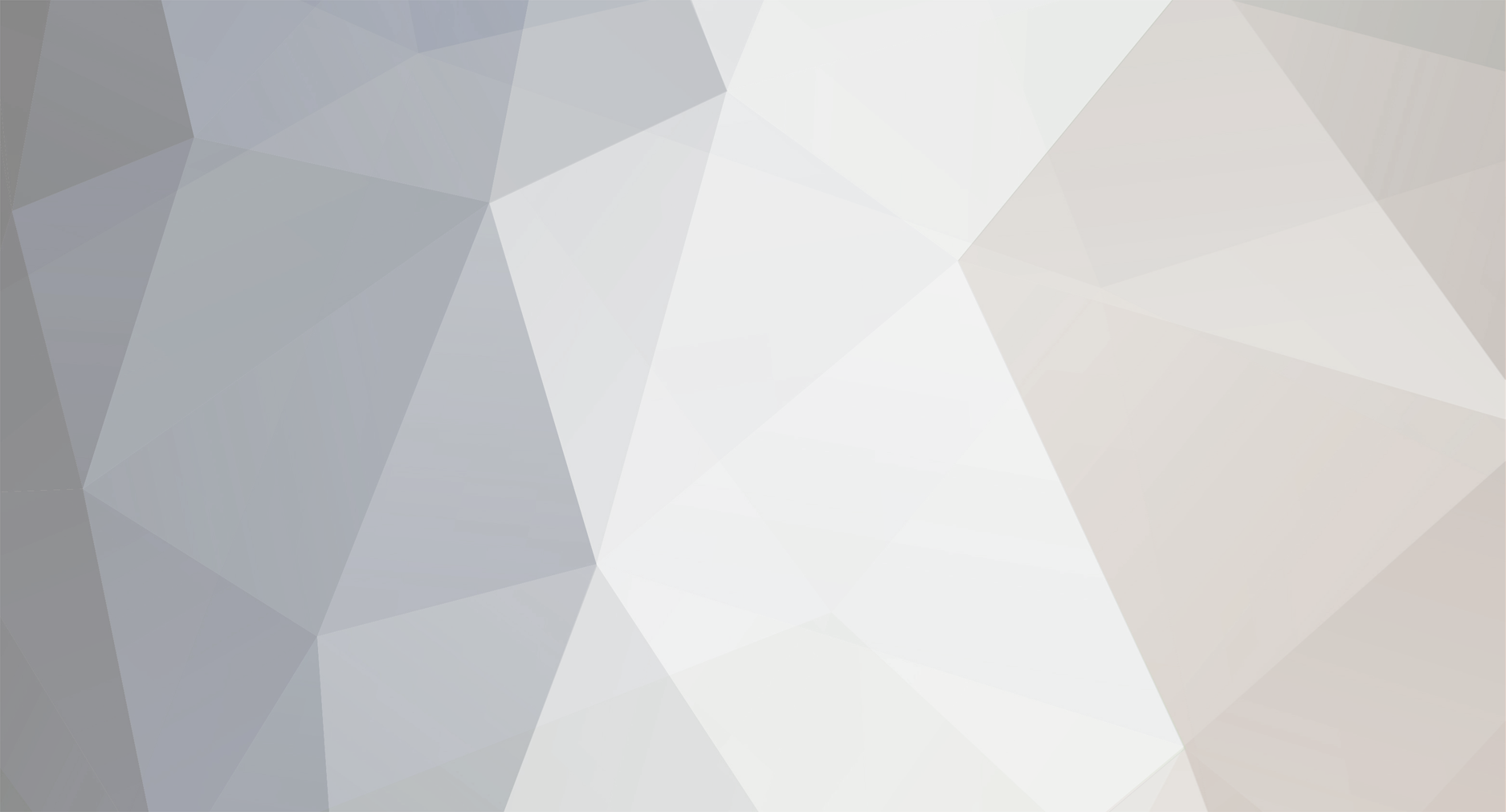
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
You could do it with PHP and javascript using AJAX techniques. However, it wont be much good if your expecting heavy usage.
-
For starters, add error checking on the query: <?php $sql = "SELECT * FROM uploads where id = $id"; $result = mysql_query() or die(mysql_error().'<br />On the query:'.$sql); if(mysql_num_rows($result) == 1){ $row = mysql_fetch_assoc($result); $filename = $row['name']; $imgdir = "imageuploads/"; unlink($imgdir . $filename); }else{ echo 'ID does not exist!'; } ?>
-
[SOLVED] problem with apostrophe in name field...
GingerRobot replied to rsammy's topic in PHP Coding Help
Sorry yes, i made a mistake it my testing of it. Wasn't sure if the alert would show the character code or the quote - turns out its the character code. So yes, addslashes() is the way to go. -
Eh? Whats the problem exactly?
-
[SOLVED] problem with apostrophe in name field...
GingerRobot replied to rsammy's topic in PHP Coding Help
Use the htmlentities() funtion on all of your variables. The problem occurs because the single quotes confuse javascript - they close the string being used for the confirm box. The function will convert the quotes to their HTML character codes. -
Indeed - do some error checking! <?php $query = "SELECT training.filenumber, training.date, training.title, trainingupload.name, trainingupload.size ". "FROM training t LEFT JOIN trainingupload ta ON t.filenumber = ta.filenumber"; $result = mysql_query($query) or die(mysql_error()); if(mysql_num_rows($result) > 0){ $row = mysql_fetch_array($result); }else{ echo 'No results!'; } ?>
-
Excuse me? Where did you hear that rubbish? jorgep: You might want to check your session.cookie_lifetime. It's possible the sessions are timing out.
-
Help with submitting records into a database.
GingerRobot replied to shuffweb's topic in PHP Coding Help
1.) I suggest you add a key to your form's array containing the student ID - you need to be able to identify which student the attendance mark refers to: <input type="checkbox" name="attendance['.$row['student_id'].'" value="1" > 2.) You need to loop through the students. How you do this depends on the result you want. If you would like to only insert those students who were present, you can loop through the $_POST['attendance'] array, using the key for the student's ID. However, if you want to insert ALL students who should have been there with marks for who was present and who was absent, you'll need to return to your code to select the students who were on the register. You'll then have to cycle though this list, and compare it to the $_POST['attendance'] array. If the student ID is in the array, insert a record showing them present. If its not, insert a record showing them absent. 3.) As for the sessions - where are they set? You'll need to trace the error back to that. -
You need to close your table outside of the while loop: <?php $WalkNo = $_GET['WalkNo']; $WalkNo = mysql_real_escape_string($WalkNo); $Venue = mysql_real_escape_string($Venue); $WalkDate = mysql_real_escape_string($WalkDate); $Leader = mysql_real_escape_string($Leader); $account = mysql_fetch_array(mysql_query("SELECT * FROM walks WHERE WalkNo='$WalkNo'")); ?> </table> <table class="join" cellspacing="0" width="861"> <tr> <td class="Header">WalkNo</td> <td class="Body"><?php echo $account["WalkNo"]; ?></td> </tr> <tr> <td class="Header">Venue</td> <td class="Body"><?php echo $account["Venue"]; ?></td> </tr> <tr> <td class="Header">WalkDate</td> <td class="Body"><?php echo $account["WalkDate"]; ?></td> </tr> <tr> <td class="Header">Leader</td> <td class="Body"><?php echo $account["Leader"]; ?></td> </tr> </table> <p> <hr class="hr_blue"/></p> <font face="Arial" size="3"> Walk Participants</font> <p></p> <?php $WalkNo = $_GET['WalkNo']; $query = "SELECT w.WalkNo, m.MemberRef, m.Forename, m.Surname, w.DateJoined FROM members m, walker w WHERE w.MemberRef=m.MemberRef AND w.WalkNo='$WalkNo'"; $result = mysql_query($query) or die('Query error!<br />Error:' . mysql_error() . 'Query: <pre>' . $query . '</pre>'); if(mysql_num_rows($result) == 0) { print "No Participants exist"; } else{ ?> <table class="join" cellspacing="0" width="861"> <tr> <td class="Header">WalkNo</td> <td class="Header">Member Ref</td> <td class="Header">Forename</td> <td class="Header">Surname</td> <td class="Header">DateJoined</td> </tr> <?php while ($account = mysql_fetch_assoc($result)) { ?> <tr> <td class="Body"><?php echo $account["WalkNo"]; ?></td> <td class="Body"><?php echo $account["MemberRef"]; ?></td> <td class="Body"><?php echo $account["Forename"]; ?></td> <td class="Body"><?php echo $account["Surname"]; ?></td> <td class="Body"><?php echo $account["DateJoined"]; ?></td> </tr> <?php } ?> </table> <?php if (isset($_POST['submit'])) { $error_stat = 0; $MemberRef_message = ''; $Password_message = ''; $Password2_message = ''; $User_message = ''; $Walk_message = ''; $MemberRef = mysql_real_escape_string(stripslashes($_POST['MemberRef'])); $Password = mysql_real_escape_string(stripslashes($_POST['Password'])); //Error checking // MemberRef Check) if (!$MemberRef) { //Set the error_stat to 1, which means that an error has occurred $error_stat = 1; //Set the message to tell the user to enter a username $MemberRef_message = '*Please enter MemberRef*'; } else if (!ctype_digit($MemberRef)) { $error_stat = 1; $MemberRef_message .= '*MemberRef must be a number*'; } if (!$Password) { //Set the error_stat to 1, which means that an error has occurred $error_stat = 1; //Set the message to tell the user to enter a password $Password_message = '*Please enter a Password*'; } // MemberRef and Password Check if (isset($_POST['submit']) && $error_stat == 0) { $account = mysql_query("SELECT * FROM members WHERE MemberRef='$MemberRef' AND Password='$Password'"); $numrows = mysql_num_rows($account); //get rows returned if($numrows == 0) { $error_stat = 1; $User_message = '*Username and/or Password are invalid*'; } // Walker Check if (isset($_POST['submit']) && $error_stat == 0) { $account = mysql_query("SELECT * FROM walker WHERE MemberRef='$MemberRef' AND WalkNo='$WalkNo'"); $numrows = mysql_num_rows($account); //get rows returned if($numrows == 1) { $error_stat = 1; $Walk_message = '*User is already on walk*'; } } } } ?> <hr class="hr_blue"/></p><font face="Arial" size="3">Join Walk </font><form method="post" class="addwalkerform" action=""> </font> <fieldset> <label for="MemberRef">MemberRef:</label> <input name="MemberRef" type="text" id="MemberRef" value="<?php echo $_POST['MemberRef']; ?>"/> <span class="redboldtxt"><?php echo "$MemberRef_message";?></fieldset></span> </fieldset> <fieldset> <label for="Password">Password:</label> <input name="Password" type="text" id="Password" value="<?php echo $_POST['Password']; ?>"/> <span class="redboldtxt"><?php echo "$Password_message";?></fieldset></span> <span class="redboldtxt"><?php echo "$Password2_message";?></fieldset></span> <fieldset> <p class="submit"><input type="submit" name="submit" value="Join Walk" /> <span class="redboldtxt"><?php echo "$User_message";?></fieldset></span> <p></p> <span class="redboldtxt"><?php echo "$Walk_message";?></fieldset></span> </fieldset> </fieldset> </form> <?php } ?>
-
<textarea class="input" id="about_me" name="about_me" rows="4" cols="40" disabled="disabled"><?php echo $message ?></textarea> If this form is then being submitted you'll still need to check the value of it, however.
-
making a switch + requesting subforum
GingerRobot replied to unsider's topic in PHPFreaks.com Website Feedback
I completely agree. When you look at forums that cover many languages, i think the quality of help available tends to drop off severely. -
[SOLVED] Putting a variable in URL of $upload_path
GingerRobot replied to alconebay's topic in PHP Coding Help
You need to enclose thumbnail in quotes, and add a semi-colon: $myvariable = 'thumbnail'; $upload_path = "../pics/$myvariable-image/"; -
It is possible to achieve something similar by parsing the $_SERVER['QUERY_STRING'] variable. However, there are limitations and mod rewriting is much more powerful.
-
A quick search on the forums for the class produced this:
-
You may have better luck contacting the author of the class - unless anyone here is using the same class it's unlikely we'll be able to help you. Given the error message, that method obviously doesn't exist.
-
<?php if(!empty($_POST['field']){ //show the field and its value } Is that what you were after? Your question was a little vague.
-
<?php if(!empty($_POST['field']){ //show the field and its value } Is that what you were after? Your question was a little vague.
-
That's up to the game developers. All other types of software developers should support Linux systems as well. Software incompatibility is what's holding me back from using Linux full-time. It's like the chicken and the egg though isn't it. Game developpers wont bother to produce games for linux 'till there's a solid userbase.
-
Indeed. You need to be passing something that will identify the row of the database uniquely in the URL. Hopefully this will be in the form of an ID number. You'd then do something like: $result = mysql_query("SELECT col_4 FROM {$table}"); if (!$result) { die("Query to show fields from table failed:".mysql_error()); } while($row = mysql_fetch_array($result)) { echo '<a href="Display.php?id='.$row['id'].'">'.$row['col_4'].'</a>'; echo "<br />"; } mysql_free_result($result); ?> You'd then have Display.php set up something like: <?php $id = (int) $_GET['id']; $sql = "SELECT * FROM tbl WHERE id=$id"; $result = mysql_query($sql) or die(mysql_error()); if(mysql_num_rows($result) == 1){ $row = mysql_fetch_assoc($sql); //print out information }else{ echo 'That record ID does not exist!'; } ?>
-
Well it was news to me
-
[SOLVED] Syntax error, then moving to sessions..
GingerRobot replied to GameYin's topic in PHP Coding Help
Most of your code is still inside this if statement: if(isset($_POST['login'])) { Positioning of braces isn't something you can just hope for the best with - change the position of one and it changes the logic of your code completely. -
This works nicely: getID3() Cheers. Shame most of my library is in WMA which appears unsupported. Edit: Actually, it can read WMA. Excellent - this could come in handy.
-
[SOLVED] Syntax error, then moving to sessions..
GingerRobot replied to GameYin's topic in PHP Coding Help
Your closing braces are in the wrong place. ALL of your code (well, bar the first 5 lines) is inside the first if statement. This is why it was not just a simple matter of adding in the closing brackets. You need to go through your code very carefully, and make sense of what it is supposed to be doing where. -
Out of interest, how are you grabbing the ID3 tags?
-
You need to be using a double == sign. The single equals sign is the asignment operator, which will evaluate to true. You want the the double equals sign to compare two things.