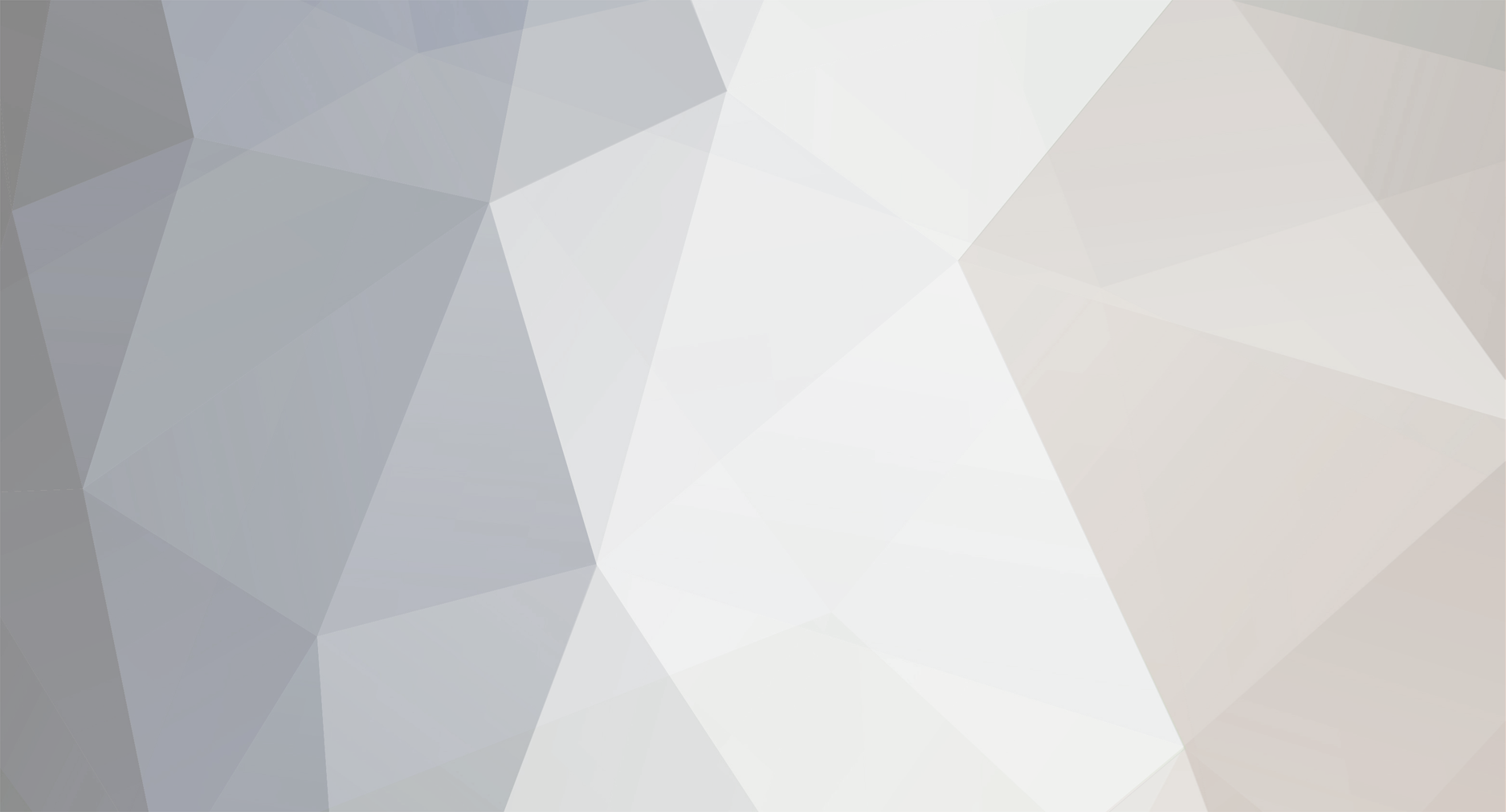
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
[SOLVED] Display of results of a db search
GingerRobot replied to denewey's topic in PHP Coding Help
You want option one and a technique called pagination. See this tutorial: http://www.phpfreaks.com/tutorial/basic-pagination -
How are you actually storing the tags? You first said you have something like "tag1,tag2,tag3,tag4", but you then have a field called "tags" in you query. Am i right in thinking that the tags field is a comma delimited string of the various tags a post has? If so, a quick and dirty 'solution' to your problem is to use the LIKE keyword: SELECT * FROM `posts` WHERE `tags` LIKE '%$tagname%'" This will return all rows where the tagname appears anywhere in the string of tags. However, a much better approach would be to use a separate table for your tags. You might like to read the following tutorial which would serve as an introduction to using multiple tables: http://www.phpfreaks.com/tutorial/data-joins-unions
-
[SOLVED] Extract all image references and place in an array
GingerRobot replied to Jalz's topic in PHP Coding Help
If you take another look at the manual page and look at the 'flags' parameter and I tell you that the default value of that parameter is PREG_PATTERN_ORDER, then hopefully you might see what's going on. The function returns an array of arrays. By default index 0 is the full match, index 1 is, as the manual says, the first parenthesized expression. In your case, there are no parenthesized expressions, so you just have index 0. This is shown in the output from print_r. When you use the foreach loop on $imgsrc, the only time it runs is for that index 0. (I.e. $key is 0 and $img_ref is set as the array. You want to loop through $imgsrc[0] and not $imgsrc: foreach( $imgsrc[0] as $key => $img_ref){ echo $img_ref; } -
-
[SOLVED] CSS dropdown menu with select statment
GingerRobot replied to iceman023's topic in PHP Coding Help
I should probably explain that the above code will show the links for city only if there is at least one photo for it. If not, it won't be displayed. Perhaps this is the behavior you're after, but I don't know. -
The use of the braces is simply to 'help' the PHP engine. In this case, they're not required as it can work out that you want to happen. But if you had this, for example: <?php class foo{ private $var = 'some value'; function __construct(){ echo "html {$this->getVar()}" . "\n"; } function getVar(){ return $this->var; } } $foo = new foo(); Then it won't work without the braces -- you'll get "html ()" as the output.
-
[SOLVED] CSS dropdown menu with select statment
GingerRobot replied to iceman023's topic in PHP Coding Help
If all the cities are required and all the cities have some associated photos, you could go with this: <?php $sql = "SELECT COUNT(*) as cnt, City FROM Photos GROUP BY City ORDER BY City ASC"; $result = mysql_query($sql) or trigger_error(mysql_error(),E_USER_ERROR); if($result){ while($row = mysql_fetch_assoc($result)){ echo '<li><a href="Cities.php?City=' . $row['City'] . '" title="' . $row['City'] . '" target="_self" >' . $row['City'] . '(' . $row['cnt'] . ')</a></li>' . "\n"; } } ?> If not, then do you have another table containing the cities? -
[SOLVED] Extract all image references and place in an array
GingerRobot replied to Jalz's topic in PHP Coding Help
You'll need to use preg_match_all if you want to find more than one occurrence of the pattern. -
Strings should be quoted, otherwise PHP treats them as constants. Depending on your PHP setup, you might find that things still 'work' however as PHP can 'guess' that an undefined constant is supposed to be a string. Relying on that is bad practice because of the aforementioned dependency on the setup. Also, as AlexWD's solution shows, you cannot simply list the possible values a variable could be. If you have the following expression: if(somecondition == somevalue || someothervalue) Then the evaluation of that will first test if somecondition == somevalue is true. If it is, the the whole logical expression is true and hence someothervalue is not tested for truth owing to short-cut evaluation. If, however, somecondition == somevalue is false we then test if someothervalue is 'true' -- i.e. it could hold the boolean value TRUE, or any non-zero integer value. If that's still not clear, the above expression is logically equivalent to: if(someothervalue || somecondition == somevalue) There is, however, probably a problem with AlexWD's solution. Logical AND takes precedence over logical OR. Hence, the if statement evaluates to true if either $logged['level'] has the value 'admin' or 'owner', regardless of the value of $logged['username']. Of course, if that's what you meant that that's fine. Otherwise, you need some parenthesis: if ($logged['username'] && ($logged['level'] == 'mod' || $logged['level'] == 'admin' || $logged['level'] == 'owner'))
-
[SOLVED] Extract all image references and place in an array
GingerRobot replied to Jalz's topic in PHP Coding Help
Question 1.) You'll be wanting regular expressions. Have you used them at all in the past? Question 2.) Indeed, that's pretty inefficient. If you're just using the img tag's attributes to set the size of the image, then the full image is still being loaded. That's bad for you and the visitor to your website, because of the increased bandwidth and hence download time. If you have the GD library installed, you can resize images using the imagecopyresampled() function. As for the best way to do it...well that depends on your requirements and current set up. Obviously you have existing images on the server and you'll have to process them initially. You could write a script to open each of the images and create a thumbnail. I'd probably recommend keeping the larger images saved though, perhaps you'll want them in the future and, let's face it, storage space is pretty cheap. If you have lots of images, you'll probably be better off doing this in batches of the images. If you're also uploading images, then you'll probably also want to add a resize feature on the upload too. -
One would of thought that maybe, just maybe, you might have deduced from the previously responses that posting the actual error message might be useful. Or am i being optimistic?
-
MySQL? Use the DATE_FORMAT() function.
-
[Java] Displaying an integer in place of a char/string
GingerRobot replied to 9three's topic in Other Programming Languages
Perhaps i also misunderstood, but aren't you essentially looking to convert an integer to a string? http://java.sun.com/j2se/1.4.2/docs/api/java/lang/Integer.html#toString() -
[SOLVED] jQuery: Adding event to dymanically added element
GingerRobot replied to GingerRobot's topic in Javascript Help
Looks perfect, i'll try that when I get back home. Thanks for the help. -
Ok, so i'm very new with jQuery and my javascript's a bit rusty. In an attempt to learn how to use jQuery a bit, i thought i'd try and create a form where new text fields are added once the others have already been used. Here's the HTML: <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Strict//EN" "http://www.w3.org/TR/html4/strict.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> <script type="text/javascript" src="jquery.js"></script> <script type="text/javascript" src="jquery.listen.js"></script> <script type="text/javascript" src="form.js"></script> </head> <body> <form id="theform"> <input type="button" value="Add" name="add" id="add" /> <br /> <input type="password" name="password" id="password" /> <br /> <div id ="container[]">Input 1: <input type="text" name="input[]"/></div> <div id ="container[]">Input 2: <input type="text" name="input[]"/></div> <div id ="container[]">Input 3: <input type="text" name="input[]"/></div> </form> </body> </html> (The button and password field were just there to practice with selectors) And my first attempt at the jQuery/Javascript: jQuery(document).ready(function(){ $("#theform input[type=text]").keypress(function(){ var n = $("#theform input[type=text]").size(); n++; var m = $("#theform input[type=text][value='']").size(); if(m==0){ $("#theform").append("<div id =\"container[]\">Input "+ n +": <input type=\"text\" name=\"input[]\" /></div>"); } }); }); Now, the problem is that the keypress event isn't added for the dynamically added text fields. That is, once the first 3 text fields have something in them, a 4th is added. When you type something into the 4th, nothing happens -- until you type something in one of the first 3 fields, at which point a 5th text field is added. After a bit of Googling, a suggestion was the listen/intercept plugins. So i gave this a go: jQuery(document).ready(function(){ $("#theform input[type=text]").listen('keypress','', function(){ var n = $("#theform input[type=text]").size() + 1; var m = $("#theform input[type=text][value='']").size(); if(m==0){ $("#theform").append("<div id =\"container[]\">Input "+ n +": <input type=\"text\" name=\"input[]\" /></div>"); } }); }); But the result is the same. Any help would be much appreciated.
-
Well i suppose you could write your own function and pass the argument by reference: <?php function mystrtoupper(&$string){ $string = strtoupper($string); } $string = "mystring"; mystrtoupper($string); echo $string . "\n"; ?> But i don't see much point.
-
You need to check if $_SESSION['user'] has been set -- use isset
-
No problem. Don't forget to mark your topic solved if you're done with it. You can find the option at the bottom left of the page -- just above the quick reply. I've done this one for you.
-
Your issue is with these lines: $NameCnt = "Name".$cnt; $NRICcnt = "NRICno".$cnt; $AgeCnt = "Age".$cnt;/td> There's no POST field with the name "Namexx", "NRICnoxx" or "Agexx" (where xx is some number). You should have 'student_name'.$cnt. Like you do on these lines: <tr> <td><input type = 'text' name = 'student_name<?php echo $cnt ?>' value='<?php echo $_POST[$NameCnt] ?>'></td> <td><input type = 'text' name = 'nric<?php echo $cnt ?>' value='<?php echo $_POST[$NRICcnt] ?>'></td> <td><input type = 'text' name = 'age<?php echo $cnt ?>' value='<?php echo $_POST[$AgeCnt] ?>'></td> </tr> Though it would be muuuch neater to use arrays.
-
Cant figure why this mysql giving me a errorr?
GingerRobot replied to Monkuar's topic in PHP Coding Help
Learn something new every day. I didn't know isset() could take a variable number of arguments. -
Well if you're going to need to search the same string multiple times, i'd build an array: <?php $id=339; $str="338:1, 339:3 ,340:15"; $pieces = explode(",",$str); $array = array(); foreach($pieces as $piece){ $piece = trim($piece); list($key,$value) = explode(":",$piece); $array[$key] = $value; } //can then access based on id if(isset($array[$id]){ echo $array[$id]; }else{ echo "That id doesn't exist!"; } ?> If it's just the once, a regex would be neater. Edit: e.g: <?php $id=359; $str="338:1, 339:3 ,340:15"; preg_match("|$id:([0-9]+)|",$str,$matches); if(count($matches) > 0){ echo $matches[1]; }else{ echo "That ID doesn't exist!"; } ?>
-
Ok, so first off I know this isn't a computer help forum, but i figure i'll ask you guys first anyway. Basically, i spilled some water on my laptop a week ago. I dried it out and left it to dry for a couple days, but no dice. Or so i thought. At the moment, one of a few things happen when i press the power button: 1.) It starts up fine (not happening particularly often though) 2.) The bios screen loads, before telling me it cannot find an internal hard drive. When this happens, i am able to boot using an Ubuntu live CD. Plus, if i retry then somtimes 1.) happens. 3.) Other than the powerlight turning on, nothing happens. Also, when things are working normally, the hotkeys at the top of the keyboard light up in sequence after pressing the power button. Sometimes there's a delay -- from 5ish seconds to 30 -- before this happens. Laptop then proceeds to one of the steps above. In some ways, this sounds like a bad connection somewhere -- when something works sometimes but not others, that usually a safe bet. But, if that were the case, i'd expect to run into problems once the laptop's turned on. But, touch wood, i've not been. Once i'm booted up, things seem to go ok. Anyone have any thoughts?
-
[SOLVED] multi image upload and two thumbnails.....HOW?
GingerRobot replied to StefanRSA's topic in PHP Coding Help
Ensure the GD library is installed on your server and use imagecopyresampled -
Is arial.ttf in the folder that this file is?
-
Halo 3 Saved Film HD Recording Service - Testers Needed
GingerRobot replied to HaLo2FrEeEk's topic in Beta Test Your Stuff!
Though, SQL injection isn't limited to INSERT statements.