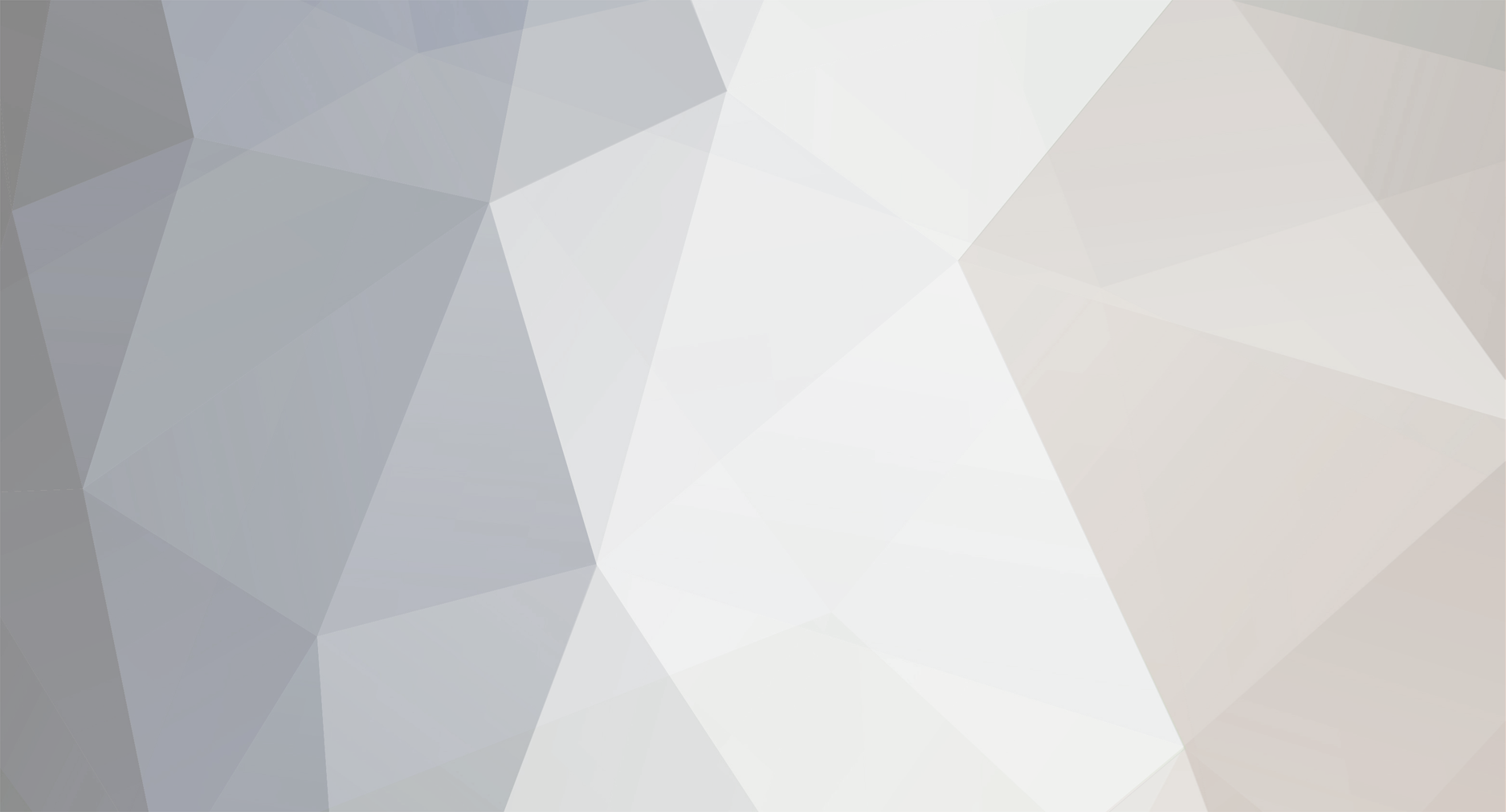
webdeveloper123
-
Posts
437 -
Joined
-
Last visited
-
Days Won
1
Posts posted by webdeveloper123
-
-
you mean do something like this?
$customers['first_name'] = $firstName = $_POST['fname']; <input type="text" id="fname" name="fname" value="<?= htmlspecialchars($firstName) ?>"><br>
-
But will that make the error show after I have hit submit and the page/form reloads?
-
I found a few ways such as:
echo "<meta http-equiv='refresh' content='0'>";
or
header('Location: insert.php');
but none of them show me the error messages related to my validation
-
Hi Guys,
I have a simple insert sql form in PDO which inserts the data just fine. Thing is I am now validating the data that goes into the database & this is the first proper time i've done it. At the moment I have only validated First Name field to be between 3-18 characters and that the user must agree to the T's & C's before that form data can be inserted into the database. What happens at the moment If I have less than 3 characters for the first name, when I press submit it goes to a new page, without the form there, but with the error message at the top of the page saying "First name has to be between 3-18 characters. Same kind of thing with T&C, it will take me to a new page without the form there and display error "You have to accept terms" etc
What I want to do is if there are errors on the form, when I press submit, I want the form to re-appear and then be able to put the error messages next to the html element it came from
I know this code isn't great but it's my first proper crack at PDO and validation.
<?php declare(strict_types = 1); ?> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title> Document </title> </head> <body> <?php include 'includes/db.php'; //include 'includes/functions.php'; $terms = ''; $message = ''; $firstName = ''; $messageName = ''; function is_text($text, int $min = 0, int $max = 1000): bool { $length = mb_strlen($text); return ($length >= $min and $length <= $max); } if ($_SERVER['REQUEST_METHOD'] == 'POST') { $sql = "INSERT INTO customer_details (first_name, last_name, address, town, county, post_code, fav_food, birthdate, email, terms) VALUES (:first_name, :last_name, :address, :town, :county, :post_code, :fav_food, :birthdate, :email, :terms);"; $customers['first_name'] = $firstName = $_POST['fname']; $customers['last_name'] = $lastName = $_POST['lname']; $customers['address'] = $address = $_POST['address']; $customers['town'] = $town = $_POST['town']; $customers['county'] = $county = $_POST['county']; $customers['post_code'] = $postCode = $_POST['postcode']; $customers['fav_food'] = $favouriteFood = $_POST['fav_food']; $customers['birthdate'] = $birthdate = $_POST['birthday']; $customers['email'] = $email = $_POST['email']; $customers['terms'] = $terms = (isset($_POST['terms']) and $_POST['terms'] == true) ? true : false; $message = $terms ? 'Thank you' : 'You must agree to the terms and conditions'; if ($terms == false){ echo $message; exit; } $valid = is_text($firstName, 3, 18); if ($valid) { $messageName = ''; } else { $messageName = 'First Name must be 3-18 characters'; } if ($valid == false){ echo $messageName; exit; } $statement = $pdo->prepare($sql); $statement->execute($customers); } ?> <?= $message ?> <?= $messageName ?> <form action="insert.php" method="post"> <label for="fname">First name:</label><br> <input type="text" id="fname" name="fname" value="<?= htmlspecialchars($firstName) ?>"><br> <label for="lname">Last name:</label><br> <input type="text" id="lname" name="lname"><br> <label for="address">Address</label><br> <input type="text" id="address" name="address"><br> <label for="town">Town</label><br> <input type="text" id="town" name="town"><br> <label for="county">County</label><br> <input type="text" id="county" name="county"><br> <label for="postcode">Post Code</label><br> <input type="text" id="postcode" name="postcode"><br><br> <label for="food">What is your favourite food?</label><br> <input type="radio" id="burger" name="fav_food" value="Burgers"> <label for="burger">Burgers</label><br> <input type="radio" id="pizza" name="fav_food" value="Pizza"> <label for="pizza">Pizza</label><br> <input type="radio" id="kebab" name="fav_food" value="Kebabs"> <label for="kebab">Kebabs</label><br><br> <label for="birthday">Birthday:</label> <input type="date" id="birthday" name="birthday"><br><br> <label for="email">Email</label><br> <input type="text" id="email" name="email"><br><br> <input type="checkbox" id="terms" name="terms" value="true" <?= $terms ? 'checked' : '' ?>> <label for="terms">I agree to the terms.</label><br><br> <input type="submit" value="Submit"> </form> </body> </html>
Thanks
-
Hey thanks guys, I did it!
-
1
-
-
ok the consensus seems to be go down the WHERE route, If ID exits take them to the edit form, if not display error or error page
thank you
-
btw this custom error page won't load unless I include the functions.php file
-
@requinix nailed it. What he said is what i am trying to acheive
Use Get - Store the customer Id into a variable from the query string
Sql select to pull all customer id from the customer table
execute in pdo
save resultset into members array
check to see if the customer ID I got in get exists in the database (e.g compare it against the resultset in members array
if its not valid, take me to custom error page
otherwise take me to the correct next page (which in this case is a form to edit the record in the database)
thats it
Maybe there is something in the functions file stopping it?
-
@benanamen - but that's not what I am trying to achieve. this is very strange
-
lol why would I lie and make this up? I could be getting on with validating the rest of my code!
-
And it's always the last id, not the first or 2nd or 3rd, always the last one
-
2 minutes ago, ginerjm said:
but your code does exactly what you have said you wanted it to do.
Then why does the last record ALWAYS take me to an error page, even though the ID & the record exists in the database
-
5 minutes ago, requinix said:
You have an ID in $_GET. To see if that's a valid ID, you grab every single ID from the database and then check to see if the one you want is in there?
yes, correct!
6 minutes ago, benanamen said:A high level overview of what you are doing would be helpful to give you the best and more specific advice.
https://example.com/customer/edit.php?user_id=7 - So basically I am validating the user id here, in this case example 7 (which exists and takes me to the correct next page) I can see your point it would have to be manually done to get to the error page but I thought it was a good place to start. So if i only have users 1 - 100 in there and someone types in 101 (which doesn't exist) they should be shown to an error page
5 minutes ago, ginerjm said:BTW what is your default fetch mode?
I'm using fetchAll for this one. Where is the default fetch mode saved?
-
11 minutes ago, ginerjm said:
You're seem to be talking about a loop process, but not showing us this loop.
There is no loop, that's the whole code.
@benanamen - Im trying to validate the query string here. So If I have members 1 to 100 it will take me to the correct next page, but if 101 is entered it takes me to an error page
-
Hi,
I've got some code here that works for re-directing the user to an custom error page if there ID in the query string is not in the database. Problem is that although it works, the last record will always re-direct to the error page even though that ID exists in the database. So I realised this at Id 17, redirected me to an error page, than I added a new record (18) and now 17 shows me to the page its supposed to take me to, but now 18 is re directing to the error page. Doesn't make any sense.
Here is my code:
include 'includes/db.php'; include 'includes/functions.php'; $keys = "SELECT customer_id FROM customer_details;"; $statement = $pdo->query($keys); $members = $statement->fetchAll(); //var_dump($members); $id = $_GET['user_id'] ?? ''; $valid = array_key_exists($id, $members); if (!$valid) { http_response_code(404); header('Location: page-not-found.php'); exit; }
Shall I paste my functions.php include file? it's pretty long.
Thanks
-
ok thanks guys
-
10 minutes ago, mac_gyver said:
any value you output on the web page needs to have htmlentities applied to it to help prevent cross site scripting.
Yes I used htmlspecialchars on my "display" page. Basically a page which shows all records from the table. This is what I meant for sanitizing.
Thank you that is a long list of things to think about.
Relating to my post before what should I be using instead of:
$customers['first_name'] = $firstName = $_POST['fname'];
$statement = $pdo->prepare($sql); $statement->execute($customers);
Like what benanamen said, "just use the post array"
-
I'm doing the validation today & I have already started to sanitize (on a diff page)
16 hours ago, benanamen said:Your double variable assignment is pointless and just litters the codebase.
You mean most of this is not necessary?
$customers['first_name'] = $firstName = $_POST['fname'];
16 hours ago, benanamen said:You already have the POST array, just use it.
Could you give me an example please?
-
haha rookie error! Thanks kicken!
-
Hi Guys,
I've decided to try my hand at PDO moving on from mysqli as it's considered much better and more secure. I have the script below and If I don't comment out the include functions.php line I get a friendly error message. But when I do comment it out I get this:
Fatal error: Uncaught PDOException: SQLSTATE[HY093]: Invalid parameter number: parameter was not defined in /var/www/vhosts/xxx.xxx/xxx.xxx.xx/customer/insert.php:36 Stack trace: #0 /var/www/vhosts/xxx.xx/xx.xx.xxx/customer/insert.php(36): PDOStatement->execute(Array) #1 {main} thrown in /var/www/vhosts/xxx.xx/xxxx.xx.xx/customer/insert.php on line 36
Here is the code:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title> Document </title> </head> <body> <?php include 'includes/db.php'; //include 'includes/functions.php'; if ($_SERVER['REQUEST_METHOD'] == 'POST') { $sql = "INSERT INTO customer_details (first_name, last_name, address, town, county, post_code, fav_food, birthdate, email, terms) VALUES (:first_name, :last_name, :address, :town, :county, :post_code, :fav_food, :birthdate, :email, :terms);"; $customers['fname'] = $firstName = $_POST['fname']; $customers['lname'] = $lastName = $_POST['lname']; $customers['address'] = $address = $_POST['address']; $customers['town'] = $town = $_POST['town']; $customers['county'] = $county = $_POST['county']; $customers['postcode'] = $postCode = $_POST['postcode']; $customers['fav_food'] = $favouriteFood = $_POST['fav_food']; $customers['birthday'] = $birthdate = $_POST['birthday']; $customers['email'] = $email = $_POST['email']; $customers['terms'] = $terms = (isset($_POST['terms']) and $_POST['terms'] == true) ? true : false; $statement = $pdo->prepare($sql); $statement->execute($customers); } ?> <form action="insert.php" method="post"> <label for="fname">First name:</label><br> <input type="text" id="fname" name="fname"><br> <label for="lname">Last name:</label><br> <input type="text" id="lname" name="lname"><br> <label for="address">Address</label><br> <input type="text" id="address" name="address"><br> <label for="town">Town</label><br> <input type="text" id="town" name="town"><br> <label for="county">County</label><br> <input type="text" id="county" name="county"><br> <label for="postcode">Post Code</label><br> <input type="text" id="postcode" name="postcode"><br><br> <label for="food">What is your favourite food?</label><br> <input type="radio" id="burger" name="fav_food" value="Burgers"> <label for="burger">Burgers</label><br> <input type="radio" id="pizza" name="fav_food" value="Pizza"> <label for="pizza">Pizza</label><br> <input type="radio" id="kebab" name="fav_food" value="Kebabs"> <label for="kebab">Kebabs</label><br><br> <label for="birthday">Birthday:</label> <input type="date" id="birthday" name="birthday"><br><br> <label for="email">Email</label><br> <input type="text" id="email" name="email"><br><br> <input type="checkbox" id="terms" name="terms" value="true"> <label for="terms">I agree to the terms.</label><br><br> <input type="submit" value="Submit"> </form> </body> </html>
I'm pretty sure it's to do with the $customers array becuase most of the code is from the book
Many thanks
-
After all, that is what you are paying them for
-
Oh come on if she is a beginner how it she supposed to re install word press and re upload the site? I suggest you get in touch with your hosting service and take there advice/let them do it for you.
-
Hey I have used Cpanel before, there is something along the lines of File Manager which in the top right ( i think) there is a search function. type cron.php in there and see what comes up.
-
do what @dodgeitorelse3 suggested and click on the wp-includes folder and look in there. At the moment you have screen shotted the wrong folder. You have screen shotted the public_html folder, the folder your looking for is within public_html where the screen shot with the red arrow is at. go into that folder and screen shot it
posting a form that has errors then re-displaying that form with errors on page
in PHP Coding Help
Posted
hey i think i figured it out
It was the exit command stopping the form/error messages loading