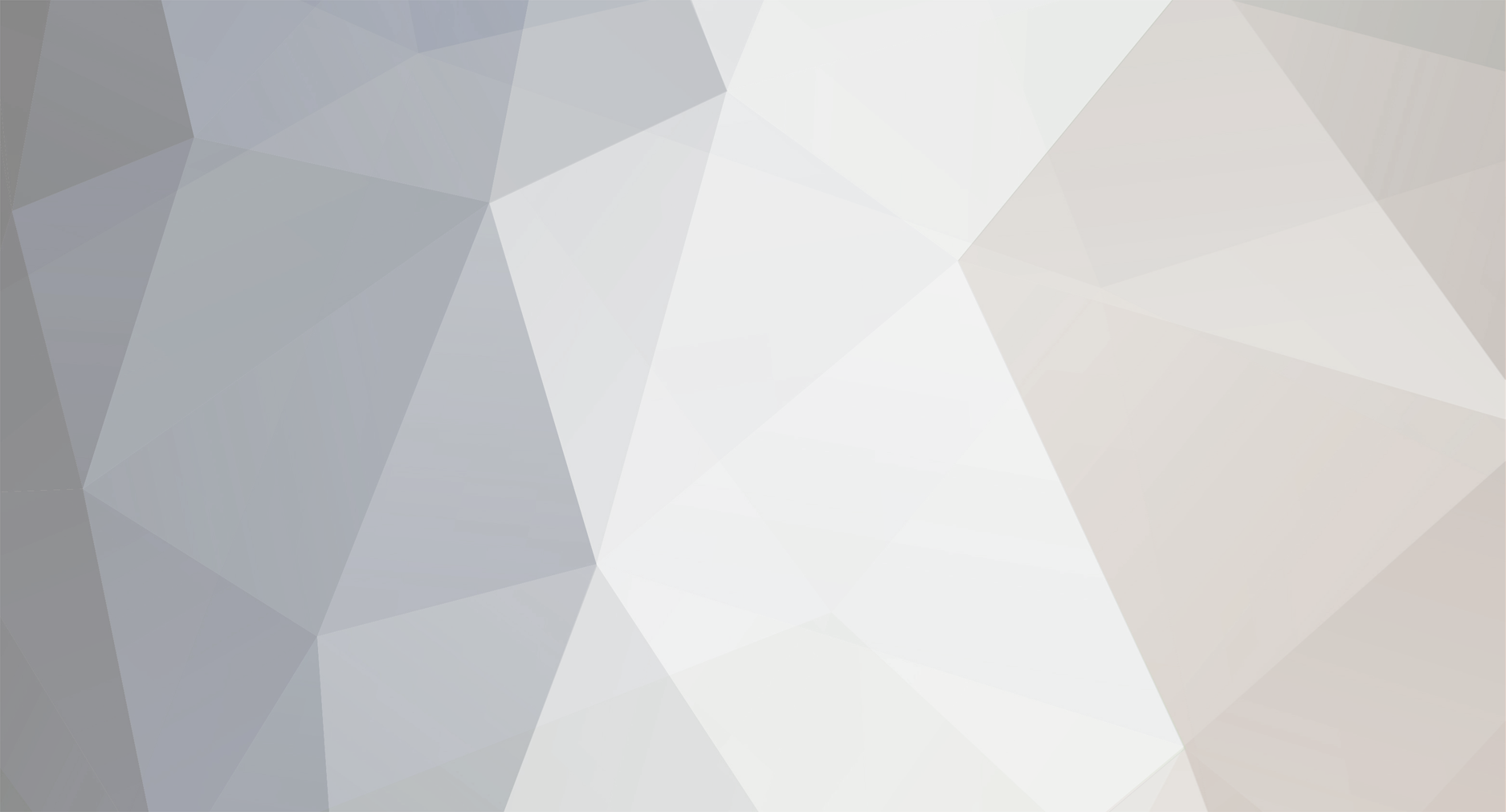
webdeveloper123
Members-
Posts
437 -
Joined
-
Last visited
-
Days Won
1
Everything posted by webdeveloper123
-
using for loop to display elements from array
webdeveloper123 replied to webdeveloper123's topic in PHP Coding Help
sorry that should read: and I am getting the data from $newdata but getting illegal offset errors all over the page for the other array. even though apparently they should all be in 1 array now ($table). Plus the data from $newdata shows all records under Row Number 4 -
using for loop to display elements from array
webdeveloper123 replied to webdeveloper123's topic in PHP Coding Help
Hey Barand, yes I used foreach before on this array sucessfully but then came across this for loop and thought it look interesting and so I gave it a try. I'm Using: foreach ($table as $row => $data) { echo "<p><b>Row number $row</b></p>"; echo "<ul>"; foreach ($data as $col) { echo "<li>$col[fname]</li>"; echo "<li>$col[lname]</li>"; echo "<li>$col[age]</li>"; } echo "</ul>"; } and I am getting the data from $newdata but getting illegal offset errors all over the page for the other array. Plus the data from $newdata shows all records under Row Number 4 but apparently they should all be in 1 array now ($table) -
Hi, I've got a for loop which displays elements from an array. The array looks like this: $table = array ( 0 => array ( 'fname' => 'Peter', 'lname' => 'Smith', 'age' => '37' ), 1 => array ( 'fname' => 'Paul', 'lname' => 'Hartley', 'age' => '48' ), 2 => array ( 'fname' => 'Mary', 'lname' => 'Baker', 'age' => '42' ), 3 => array ( 'fname' => 'Jane', 'lname' => 'Doe', 'age' => '51' ) ); The for loop looks like this: for ($row = 0; $row < 4; $row++) { echo "<p><b>Row number $row</b></p>"; echo "<ul>"; for ($col = 0; $col < 1; $col++) { echo "<li>".$table[$row]['fname']."</li>"; echo "<li>".$table[$row]['lname']."</li>"; echo "<li>".$table[$row]['age']."</li>"; } echo "</ul>"; } Now this code is working fine. Then I set about adding an element to the array using array_push like this: $newdata = array ( 'fname' => 'Lionel', 'lname' => 'Messi', 'age' => '36' ); array_push($table,$newdata); And I displayed it (sucessfully) using this: for ($row = 0; $row < 5; $row++) { echo "<p><b>Row number $row</b></p>"; echo "<ul>"; for ($col = 0; $col < 1; $col++) { echo "<li>".$table[$row]['fname']."</li>"; echo "<li>".$table[$row]['lname']."</li>"; echo "<li>".$table[$row]['age']."</li>"; } echo "</ul>"; } Note: The only difference between the above 2 for loops is I changed $row < 4 to $row < 5 Then I wanted to add more than one element to the array so I used this: $newdata = array ( 4 => array ( 'fname' => 'Jon', 'lname' => 'Atkins', 'age' => '27' ), 5 => array ( 'fname' => 'Phil', 'lname' => 'Jones', 'age' => '14' ), 6 => array ( 'fname' => 'Frank', 'lname' => 'Lampard', 'age' => '48' ), 7 => array ( 'fname' => 'Toney', 'lname' => 'Brentford', 'age' => '25' ) ); array_push($table,$newdata); But the weird baffling part is I am using the same forloop: for ($row = 0; $row < 8; $row++) { echo "<p><b>Row number $row</b></p>"; echo "<ul>"; for ($col = 0; $col < 1; $col++) { echo "<li>".$table[$row]['fname']."</li>"; echo "<li>".$table[$row]['lname']."</li>"; echo "<li>".$table[$row]['age']."</li>"; } echo "</ul>"; } Note : This time I have changed $row < 8 but I get this error : Row number 4 Notice: Undefined index: fname Undefined index: lname Undefined index: age And errors like : Row number 5 Notice: Undefined offset: 5 in on line 86 Notice: Trying to access array offset on value of type null in on line 86 Notice: Undefined offset: 5 in on line 87 Notice: Trying to access array offset on value of type null in on line 87 Notice: Undefined offset: 5 in on line 88 Notice: Trying to access array offset on value of type null in on line 88 And I get the same errors as above for row 5 but for rows 6 and 7. Btw, I have row numbers for each element in the array. So Row Number 0 displays the First Name, last name and age for the element at index 0 etc. And I have done a print_r($table) and get this: Array ( [0] => Array ( [fname] => Peter [lname] => Smith [age] => 37 ) [1] => Array ( [fname] => Paul [lname] => Hartley [age] => 48 ) [2] => Array ( [fname] => Mary [lname] => Baker [age] => 42 ) [3] => Array ( [fname] => Jane [lname] => Doe [age] => 51 ) [4] => Array ( [4] => Array ( [fname] => Jon [lname] => Atkins [age] => 27 ) [5] => Array ( [fname] => Phil [lname] => Jones [age] => 14 ) [6] => Array ( [fname] => Frank [lname] => Lampard [age] => 48 ) [7] => Array ( [fname] => Toney [lname] => Brentford [age] => 25 ) ) ) It seems there are two [4] in the array. I would ideally like to get the rows automatically adjusted so I don't have to keep changing the code when I add new elements in the array. I used $arrayLength = count($table); And changed it to: (only showing relevant code here, the rest is same as above for loops): $row < $arrayLength; and I get these errors: Notice: Undefined index: fname on line 86 Notice: Undefined index: lname on line 87 Notice: Undefined index: age on line 88 But the offset errors disappear Sorry for long post Many thanks
-
figured it out. anyone who is interested it's this: document.getElementById("addNew").onclick = function () { addOne(); } document.getElementById("sList").ondblclick = function () { deleteList(); } function addOne() { var a = document.getElementById("addItem").value; var li = document.createElement("li"); li.appendChild(document.createTextNode(a)); document.getElementById("sList").appendChild(li); } function deleteList() { const list = document.getElementById("sList"); list.removeChild(list.firstElementChild); } and HTML same as above
-
Hi, I am fairly new to JS and need some advice/logic. I am trying to create a Shopping list using DOM, which adds an item to a list and then when I want to remove it, it should remove on double click. Problem is when I double-click, it deletes all the items, not just the one I double clicked on. I'm pretty sure I have to generate an array of DOM elements and go from there, But I am stuck as what to do next. Here is my code: document.getElementById("addNew").onclick = function () { addOne(); } document.getElementById("sList").ondblclick = function () { deleteList(); } function addOne() { var a = document.getElementById("addItem").value; var li = document.createElement("li"); li.appendChild(document.createTextNode(a)); document.getElementById("sList").appendChild(li); } function deleteList() { const element = document.getElementById("sList"); element.remove(); } And the HTML: <div id="message">JavaScript Shopping list</div> <div> <input type="text" id="addItem"> <input type="button" id="addNew" value="Add to List"> </div> <div id="output"> <h1>Shopping List</h1> <ol id="sList"> </ol> </div> Many thanks
-
echo javascript inside anchor element
webdeveloper123 replied to webdeveloper123's topic in PHP Coding Help
that did it barand! Many thanks to you both! -
Hey, sorry guys I know this is a easy one but I've been at it all day and can't seem to figure it out. I want to include this: onclick="return confirm('Are you sure?')" Inside this line of code: <td> <?php echo("<a href='delete.php?user_id=" . $d_row["FormId"] . "'>Delete</a>"); ?></td> I am deleting a record, I have all the SQL, it's all fine, but just this annoying little problem. I have tried many things , including escaping the double quotes but I have got no where. I have tried the following but none of them work: <td> <?php echo("<a href='delete.php?user_id=" . $d_row["FormId"] . "' onclick="return confirm('Are you sure?')">Delete</a>"); ?></td> <td> <?php echo("<a href='delete.php?user_id=" . $d_row["FormId"] . "onclick=\"return confirm('Are you sure?')\"'>Delete</a>"); ?></td> <td> <?php echo("<a href='delete.php?user_id=" . $d_row["FormId"] . " \"onclick=\"return confirm('Are you sure?')\"'>Delete</a>"); ?></td> Thanks
-
deriving age from someone's birthday
webdeveloper123 replied to webdeveloper123's topic in PHP Coding Help
thanks guys! -
deriving age from someone's birthday
webdeveloper123 replied to webdeveloper123's topic in PHP Coding Help
@Barand...….genius! I should have tried that......popped into my head a few days ago but for some reason I never tried to combine them! Yes it was from the same book....believe it or not ( I know it doesn't show on these forums) but I got a 2.1 in Software engineering at Uni. Just didn't code for 14 years after that. -
deriving age from someone's birthday
webdeveloper123 replied to webdeveloper123's topic in PHP Coding Help
Come on barand, look i'll explain. This is what I have: $query = "SELECT Form.FormId, Form.FirstName, Form.LastName, Form.Email, Form.Age, Form.Birthdate, Form.FavLanguage, GROUP_CONCAT(Vehicle.VehSelection SEPARATOR ', ') AS VehSelection FROM Vehicle RIGHT JOIN Form ON Form.FormId = Vehicle.FormId GROUP BY Form.FormId"; $result = mysqli_query($link, $query); $agequery = "SELECT timestampdiff(year, Birthdate, curdate()) as age2 FROM Form"; $resultage = mysqli_query($link, $agequery); The first $query puts all the VehSelections on one line. Then I save that into $result. Then I do another query called $agequery and save it into $resultage. Then I fetch the $result and save it to the $table array. $table = []; while ($row = mysqli_fetch_assoc($result)) { $table[] = $row; } So then can I save both results ($result and $resultage) into the same $table array? Because I'm not sure if you can do that. I tried but it didn't work(maybe i'm doing something wrong. So instead I create a new table array, called $tableage: $tableage = []; while ($rowage = mysqli_fetch_assoc($resultage)) { $tableage[] = $rowage; } So moving on from there, If I have $table and $tableage, I got the derived ages in $tableage and the rest of the data in $table. So when I loop round to print the records from the database I use: <?php if ($table) { foreach ($table as $d_row) { ?> <tr> <td><?php echo($d_row["FormId"]); ?></td> <td width="10"> </td> <td><?php echo($d_row["FirstName"]); ?></td> <td width="10"> </td> <td><?php echo($d_row["LastName"]); ?></td> <td width="10"> </td> <td><?php echo($d_row["Email"]); ?></td> <td width="10"> </td> <td><?php echo($d_row["Age"]); ?></td> <td width="10"> </td> <td><?php echo($d_row["Birthdate"]); ?></td> <td width="10"> </td> <td><?php echo($d_row["FavLanguage"]); ?></td> <td width="10"> </td> <td><?php echo($d_row["VehSelection"]); ?></td> <td width="10"> </td> <td><?php echo("<a href='edit3.php?user_id=" . $d_row["FormId"] . "'>Edit</a>"); ?></td> <td width="10"> </td> <td> <?php echo("<a href='delete_feedback.php?user_id=" . $d_row["FormId"] . "'>Delete</a>"); ?></td> </tr> <?php } } ?> But because the foreach says $table, I can't use $tableage in there as well. So I start a new foreach: <?php if ($tableage) { foreach ($tableage as $d_row1) { ?> <td><strong>new Age</strong></td> <td width="10"> </td> <td><?php echo($d_row1["age2"]); ?></td> <?php }} ?> My question is how do I combine the printed out results into one? I would like to replace <td><?php echo($d_row["Age"]); ?></td> with <td><?php echo($d_row1["age2"]); ?></td> And have the printed results in one foreach, not two. -
deriving age from someone's birthday
webdeveloper123 replied to webdeveloper123's topic in PHP Coding Help
I have2 separate queries -
deriving age from someone's birthday
webdeveloper123 replied to webdeveloper123's topic in PHP Coding Help
I can't do that because age2 is not inside $table -
deriving age from someone's birthday
webdeveloper123 replied to webdeveloper123's topic in PHP Coding Help
I guess the real question was how do I get the derived ages into my View page? Because I had the SQL and it was working, all the guys who posted here posted code that derived the age correctly but I couldn't get it into my View page which looks like this: <?php if ($table) { foreach ($table as $d_row) { ?> <tr> <td><?php echo($d_row["FormId"]); ?></td> <td width="10"> </td> <td><?php echo($d_row["FirstName"]); ?></td> <td width="10"> </td> <td><?php echo($d_row["LastName"]); ?></td> <td width="10"> </td> <td><?php echo($d_row["Email"]); ?></td> <td width="10"> </td> <td><?php echo $diff->format("%y years"); ?></td> <td width="10"> </td> <td><?php echo($d_row["Birthdate"]); ?></td> <td width="10"> </td> <td><?php echo($d_row["FavLanguage"]); ?></td> <td width="10"> </td> <td><?php echo($d_row["VehSelection"]); ?></td> <td width="10"> </td> <td><?php echo("<a href='edit3.php?user_id=" . $d_row["FormId"] . "'>Edit</a>"); ?></td> <td width="10"> </td> <td> <?php echo("<a href='delete_feedback.php?user_id=" . $d_row["FormId"] . "'>Delete</a>"); ?></td> </tr> <?php } } ?> That was the problem from the start, as before I posted I had the correct sql but couldn't manage to get it into my View page, which displays all records from the database. I was doing this: <?php if ($table) { foreach ($table as $d_row) { ?> <tr> <td><?php echo($d_row["FormId"]); ?></td> <td width="10"> </td> <td><?php echo($d_row["FirstName"]); ?></td> <td width="10"> </td> <td><?php echo($d_row["LastName"]); ?></td> <td width="10"> </td> <td><?php echo($d_row["Email"]); ?></td> <td width="10"> </td> <?php } } ?> <?php foreach ($resultage as $row) { ?> <td><?php echo " {$row['age2']} <br>"; ?></td> <?php } ?> <?php foreach ($table as $d_row) { ?> <td width="10"> </td> <td><?php echo($d_row["Birthdate"]); ?></td> <td width="10"> </td> <td><?php echo($d_row["FavLanguage"]); ?></td> <td width="10"> </td> <td><?php echo($d_row["VehSelection"]); ?></td> <td width="10"> </td> <td><?php echo("<a href='edit3.php?user_id=" . $d_row["FormId"] . "'>Edit</a>"); ?></td> <td width="10"> </td> <td> <?php echo("<a href='delete_feedback.php?user_id=" . $d_row["FormId"] . "'>Delete</a>"); ?></td> </tr> <?php } ?> But when I loaded the page it looked a total mess. So I'm using Barands code at the moment which is this: $agequery = "SELECT timestampdiff(year, Birthdate, curdate()) as age2 FROM Form"; $resultage = mysqli_query($link, $agequery); foreach ($resultage as $row) { echo " {$row['age2']} <br>"; } Now how do I get echo " {$row['age2']} <br>"; into the top block of code in this post, replacing <td><?php echo $diff->format("%y years"); ?></td> ? -
deriving age from someone's birthday
webdeveloper123 replied to webdeveloper123's topic in PHP Coding Help
Yes, thanks barand -
deriving age from someone's birthday
webdeveloper123 replied to webdeveloper123's topic in PHP Coding Help
I just thought it would take each FormId, 1 by 1, and calculate the age for each record and print it out I understand what your saying about variables. -
deriving age from someone's birthday
webdeveloper123 replied to webdeveloper123's topic in PHP Coding Help
I didn't know it was only querying one record, I thought all the FormId's from the database were stored in there, as I've done similar sql code like that before and it worked -
deriving age from someone's birthday
webdeveloper123 replied to webdeveloper123's topic in PHP Coding Help
That's why I'm looking into doing Zend courses, as it is a much better level of teaching -
deriving age from someone's birthday
webdeveloper123 replied to webdeveloper123's topic in PHP Coding Help
Tbh, the book I bought to learn php was really bad. That's why my code is not that good, as when I learnt it, it was a very bad book. -
deriving age from someone's birthday
webdeveloper123 replied to webdeveloper123's topic in PHP Coding Help
ahh you guys are looping around the result set and not the $table array, I spotted that in ginerjm commented code. Is that a better way of doing it? -
deriving age from someone's birthday
webdeveloper123 replied to webdeveloper123's topic in PHP Coding Help
maybe I should have mentioned that earlier, lol -
deriving age from someone's birthday
webdeveloper123 replied to webdeveloper123's topic in PHP Coding Help
Thanks to both of your replies. That's what I did Barand, I used: SELECT TIMESTAMPDIFF (YEAR, Birthdate, CURDATE()) AS AGE FROM Form; It gave me correct results for all the records, but when I tried to put it into my code I did this: SELECT TIMESTAMPDIFF (YEAR, Birthdate, CURDATE()) AS Age1 FROM Form WHERE Form.FormId = '$FormId'"; But when I tried to loop round it and output the records I only ever got result for first record. I'll try out solutions from above. Thanks -
deriving age from someone's birthday
webdeveloper123 replied to webdeveloper123's topic in PHP Coding Help
I tried this as well: foreach ($table as $value){ $date1=date_create("now"); $date2=date_create(($value["Birthdate"])); $diff=date_diff($date1,$date2); } And I tried both of them without the loop as well