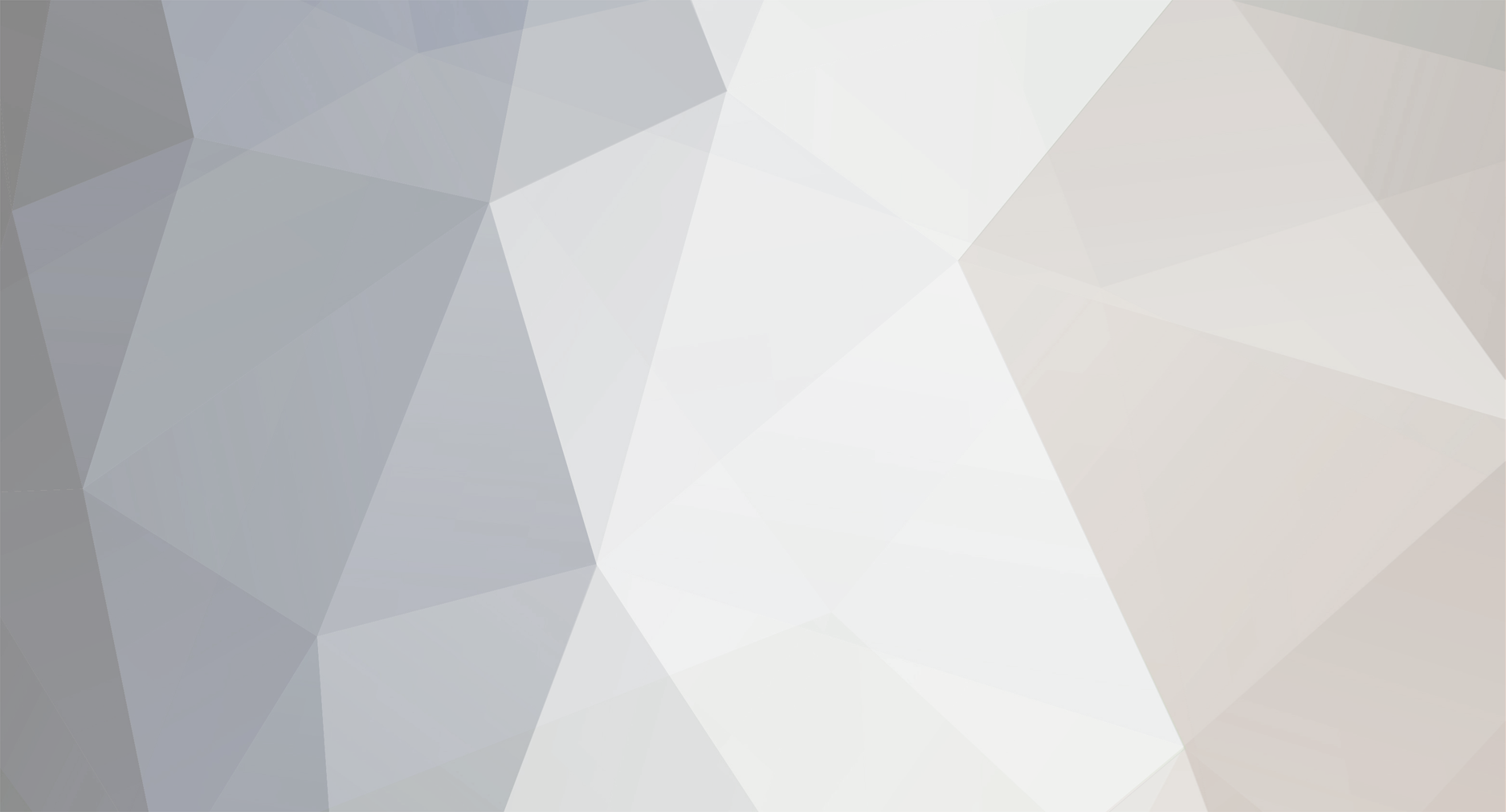
Canman2005
-
Posts
669 -
Joined
-
Last visited
Never
Posts posted by Canman2005
-
-
Fixed it
-
Hi all
I have the following QUERY
SELECT * FROM `products` WHERE ((`title` LIKE '%Apple%') || (`title` LIKE '%Google%') || (`title` LIKE '%Microsoft%'))
Which all works super.
But I am adding a form onto the front end which allows people to enter a "keyword" into a form field, I am asking them to seperate keywords with commas, when they submit the form, it will create a variable called
$words
so that value could be something like
$words = "apple, microsoft, google, ebay";
or
$words = "iphone, ipod";
my question is, how can I get PHP to turn that variable into something like
((`title` LIKE '%Apple%') || (`title` LIKE '%Google%') || (`title` LIKE '%Microsoft%'))
so that I can use it within my query.
I did have a thought though, and that was about people adding a comma at the start or end, so the variable could look like
$words = ",iphone, ipod";
or
$words = "iphone, ipod,";
or
$words = ",iphone, ipod,";
So I guess I would need to strip any spaces and commas at the start and end of the variable
I have been trying something like
str_replace(',','%') || (`title` LIKE '%',$words);
but I cannot seem to tweak it so that it works correctly
Anyone have any ideas? Maybe my way is going to be too complex
Thanks
Dave
-
thank you very much
-
Hi all
I have a content loading script
function ajaxLoader(url,id) { if (document.getElementById) { var x = (window.ActiveXObject) ? new ActiveXObject("Microsoft.XMLHTTP") : new XMLHttpRequest(); } if (x) { x.onreadystatechange = function() { el = document.getElementById(id); el.innerHTML = ""; if (x.readyState == 4 && x.status == 200) { el = document.getElementById(id); el.innerHTML = x.responseText; } } x.open("GET", url, true); x.send(null); } }
which works great.
I then have a drop down HTML list which looks like
<select name="products" onChange="ajaxLoader('product_list.php?value=(this.value)','contentarea');"> <option value="1">Apple</option> <option value="2">Dell</option> <option value="3">Logitech</option> </select>
I then have a SPAN area
<span id="contentarea"></span>
in which "product_list.php" loads in.
all I want to do, is pass the value of whatever option was selected
So if "Dell" was selected, the call would be
onChange="ajaxLoader('product_list.php?value=2','contentarea');"
and if "Apple" was selected, the call would be
onChange="ajaxLoader('product_list.php?value=3','contentarea');"
but using
(this.value)
doesn't seem to be passing the selected value in the list
can anyone help? I'm totally stumpted
Thanks
Dave
-
thanks dude, worked a charm
-
Hi all
I have the following PHP
$q = mysql_query("SELECT * FROM `people` WHERE `type` = 1"); while($r = mysql_fetch_array($q)) { echo $r['product']; echo "<br>"; }
This produces
1
3
6
13
which works great.
But i'm trying to create an array containing those values, so I get something like
array(1, 3, 6, 13)
How can I achive this?
I've tried
$q = mysql_query("SELECT * FROM `people` WHERE `type` = 1"); while($r = mysql_fetch_array($q)) { $ray = $ray. array($r['product'].','); }
but that doesn't seem to work and no matter how I tweak it, it doesn't seem to work.
Can anyone help?
Thanks very much
Dave
-
It's just that I want to show some rows at the top of the list.
I guess if it's not easy i'll have to think of another route
-
Hi all
I have the following database table
ID VALUE
1 Apple
2 Microsoft
3 Mobiles
4 MP3s
5 Laptops
I use a "SELECT" query in my PHP to get those results.
Is it possible to "ORDER" my results by
ID number 2 first
ID number 1 second
and then the remainder of the results in a A-Z order
so the above would look like
ID VALUE
2 Microsoft
1 Apple
5 Laptops
3 Mobiles
4 MP3s
I tried
ORDER BY (CASE WHEN `id` = 2 THEN 0 WHEN `id` = 1 THEN 2 END), `value` ASC
But that doesn't seem to work no matter how much I tweak it around
Any help would be great
Thanks
Ed
-
Hi all
I have the following code
<?php
$array = array(1,2,3,4,5,6,7,8,9,10,11,12,13);
$cols = 3;
$count = count($array);
if($count%$cols > 0){
for($i=0;$i<($cols-$count%$cols);$i++){
$array[] = ' ';
}
}
echo "<table border=\"1\">\r\n";
foreach($array as $key => $td){
if($key%$cols == 0) echo "<tr>\r\n";
echo "<td>$td</td>\r\n";
if($key%$cols == ($cols - 1)) echo "</tr>\r\n";
}
echo "</table>";
?>
which works great and returns something like
1 2 3 4
5 6 7 8
9 10 11 12
13
but is it possible to change this so that it orders the results like
1 5 9 13
2 6 10
3 7 11
4 8 12
any help would be great
Thanks
-
Hi all
Wonder if someone can help
I have the following code
// declare a global XMLHTTP Request object var XmlHttpObj; function CreateXmlHttpObj() { try { XmlHttpObj = new ActiveXObject("Msxml2.XMLHTTP"); } catch(e) { try { XmlHttpObj = new ActiveXObject("Microsoft.XMLHTTP"); } catch(oc) { XmlHttpObj = null; } } if(!XmlHttpObj && typeof XMLHttpRequest != "undefined") { XmlHttpObj = new XMLHttpRequest(); } } function ListOnChange() { var county = document.getElementById("county"); var selectedContinent = county.options[county.selectedIndex].value; var requestUrl; requestUrl = "code/xml.php" + "?filter=" + encodeURIComponent(selectedContinent); CreateXmlHttpObj(); if(XmlHttpObj) { XmlHttpObj.onreadystatechange = StateChangeHandler; XmlHttpObj.open("GET", requestUrl, true); XmlHttpObj.send(null); } } function StateChangeHandler() { if(XmlHttpObj.readyState == 4) { if(XmlHttpObj.status == 200) { PopulateCountyList(XmlHttpObj.responseXML.documentElement); } else { alert("problem retrieving data from the server, status code: " + XmlHttpObj.status); } } } function PopulateCountyList(countyNode) { var region = document.getElementById("region"); for (var count = region.options.length-1; count >-1; count--) { region.options[count] = null; } var countyNodes = countyNode.getElementsByTagName('county'); var idValue; var textValue; var optionItem; for (var count = 0; count < countyNodes.length; count++) { textValue = GetInnerText(countyNodes[count]); idValue = countyNodes[count].getAttribute("id"); optionItem = new Option( textValue, textValue, false, false); region.options[region.length] = optionItem; } } function GetInnerText (node) { return (node.textContent || node.innerText || node.text) ; }
which is stored as a Javascript file, I then have this HTML
<select name="county" id="county" onChange="return ContinentListOnChange()">
<option value="London">London</option>
<option value="New York">New York</option>
<option value="Paris">Paris</option>
</select>
So when one of the options is selected (ie: Paris), a new HTML select list is loaded below containing options based on what has been selected.
The code for the HTML list which loads is
<select name="region" id="region"> <option value="">Select county</option> </select>
This all works fine, but I wonder if anyone can help with my questions.
Basically I have a PHP session stored on the page called
$_SESSION['county']
which can store the name of one of the options, so like
$_SESSION['county'] = 'Paris';
or
$_SESSION['county'] = 'London';
So my question is, how can I provide the javascript a default "county" to load data for?
Kind of a default option.
I wonder if it's something to do with doing an "onload" within the <body> tag
Any help would be ace, i've tried to many things and cannot get it to work
Thanks
Dave
-
thats guy, i'm basically tying to split up my array so I can do a "SELECT" query from the database.
All works great, thanks
-
thats guy, i'm basically tying to split up my array so I can do a "SELECT" query from the database.
All works great, thanks
-
coooool, thanks for that everyone
I wonder if you could help me further
Is it possible to pull a single value from an array?
Let me explain.
My array currently looks like
( [1] => 4 [45] => 2 )
so with the above
45 is linked to 2 ([45] => 2)
and
1 is liked to 4 ([1] => 4)
so is it possible for example, to supply var such as
$var = 45
and run something so that it grabs the value
2
or if I supplied
$var = 1
then it would allow me to grab value
4
any help would be fab
thanks all
-
Hi all
I have an array set under
$_SESSION['items']
so if I do a
print_r($_SESSION['items']);
then I get
( [1] => 4 [45] => 2 )
what I want to do is implode that array so that I get an output that looks like
1,45
but I cannot figure it out.
If I use
print implode(",", $_SESSION['items']);
then that outputs
4,2
but I want it to output
1,45
instead
any ideas?
thanks
ed
-
thanks guys
-
Hi all
I need to a count on my array, i'm wondering if this is the best way to do it
foreach($_SESSION['basket'] as $key => $value) { $values = $values + $value; } print $values
Thanks
-
Nope, it could change and there could be more
So it could be
results.php?q=iphone&type1[]=hardware&type1[]=software&type2[]=blue&type2[]=white&type2[]=red
or
results.php?q=iphone&type1[]=hardware&type2[]=blue&type2[]=white
any help would be great
thanks
-
Hi all
I have a small shopping cart system using an array to store product id numbers in.
It stores it like
2,34,55,55,67,121
Within my cart I have the following, which allows the quantity of a particular product ID to be increased
if ($cart) { $newcart = ''; foreach ($_POST as $key=>$value) { if (stristr($key,'qty')) { $id = str_replace('qty','',$key); $items = ($newcart != '') ? explode(',',$newcart) : explode(',',$cart); $newcart = ''; foreach ($items as $item) { if ($id != $item) { if ($newcart != '') { $newcart .= ','.$item; } else { $newcart = $item; } } } for ($i=1;$i<=$value;$i++) { if ($newcart != '') { $newcart .= ','.$id; } else { $newcart = $id; } } } } } $cart = $newcart;
To use the above, I have a input field next to each item which looks like
<input type="text" name="qty23" value="" />
How can I adapt the above code so that if I pass in my URL
?action=update&id=23
then it would reduce the quantity of 23 by 1
so with an array which looked like
10,10,23,23,30
after passing
?action=update&id=23
it would then look like
10,10,23,30
any help would be great, been trying to crack this but with no luck
thanks in advance
ed
-
hi
anyone got any ideas on this?
-
Hi all
I wonder if anyone can tell me how to set the ROOT folder for my entire site?
My site is running locally and is at the URL
http://localhost/Site 233/www/
and I need to set that as the ROOT folder for the site.
Only because when I use links such as
<a href="/contact.php">contact</a>
it set that URL as
and I need it to be
http://localhost/Site 233/www/contact.php
thanks
ed
-
Hi everyone
I am trying to ReWrite a URL and this is my first time trying to do something like this.
Basically I have the following URL
www.domain.com/results.php?q=iphone&type1[]=hardware&type1[]=software&type2[]=blue&type2[]=white&type2[]=red
that I want to rewrite to
www.domain.com/find/iphone/type1/hardware/software/type2/blue/white/red/
i've been playing around with
RewriteRule ^find/([^/]+)/([^/]+)/ results.php?type1[]=$1&type2[]=$2 [QSA,L]
but i'm just making it worse and cannot seem to find the correct way of doing it.
thanks very much
ed
-
Just one question, if I pass the `class` ID numbers in my URL string such as
class[]=2&class[]=1&class[]=33
How can I count the total number of
class[]
passed in the URL string, so in the above example, it would be "3" in total
It's just to that I can calculate the number for
$num = 2
Or is there a better way to pass those ID numbers in the URL string?
Thansk again
Ed
-
Thanks everyone, I didn't see a 2nd page of comments
It seems that PFMaBiSmAd suggestion to use
$num = 2; $query = "SELECT s.name, count(*) as cnt FROM staff s JOIN classes c ON s.id = c.staff WHERE c.class IN (1,2) GROUP BY c.staff HAVING cnt = $num";
Works fantastic
, i'm not 100% until I run all the tests, but it seems to be working.
Thanks to PFMaBiSmAd and everyone else
-
Hi
I'm sure i'm making sound harder than it is.
Let me try and explain a bit better
Okay, so I have 2 tables, one is called `staff` and it contains a list of staff (ie: Dave, Sarah).
I also have another table called `classes` which contain a list of ID numbers for classes attended (that field is called `class`) and ID numbers of staff runing the class (that field is called `staff`).
So if in my QUERY, I pass ID number 1, I want it to look in the `classes` table, and grab a list of `staff` ID numbers which attend `class` ID number 1 ONLY, so it would get just;
But if in my QUERY, I pass ID numbers 2 and 3, then it would get just;
But if in my QUERY, I pass ID numbers 1, 2 and 3, it would get nothing, as there are no staff that attend all classes 1,2 and 3, so it would get just;
Does that make it anymore clear for anyone?
Thanks so much everyone for your help
Ed
Updating multiple records
in PHP Coding Help
Posted
Hi all
I have some PHP code which basically outputs the following form
when I submit the form, I want to run an update QUERY for everything on the form, using the following QUERY
So it's almost like a need to run 3 updates scripts
Is this possible?
I thought maybe with a
foreach()
but i've no idea where to start.
any ideas anyone?
thanks all
dave