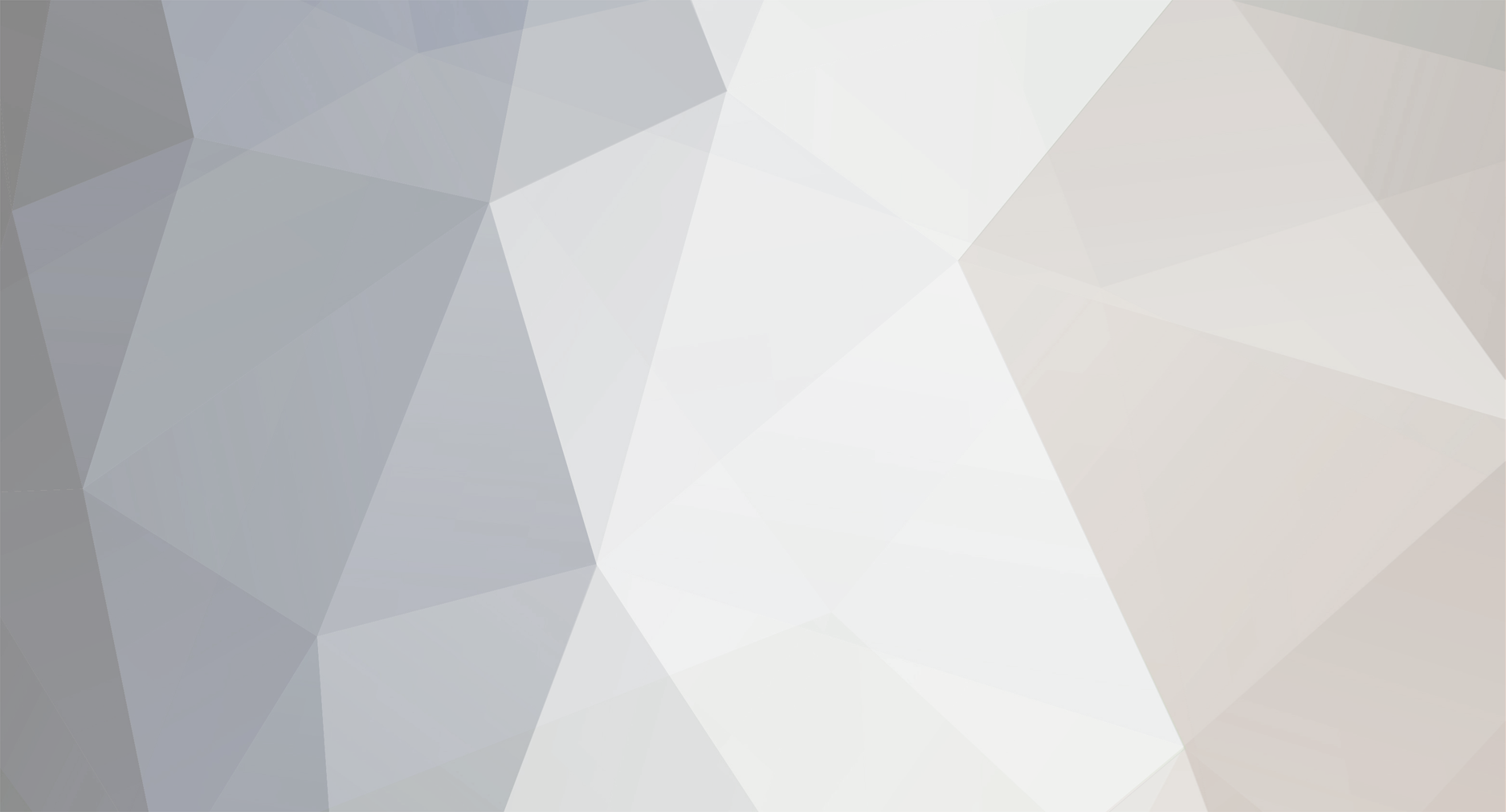
Canman2005
-
Posts
669 -
Joined
-
Last visited
Never
Posts posted by Canman2005
-
-
Hi all
I have the following function
<?php function size_hum_read($size){ $i=0; $iec = array("B", "KB", "MB", "GB", "TB", "PB", "EB", "ZB", "YB"); while (($size/1024)>1) { $size=$size/1024; $i++; } return substr($size,0,strpos($size,'.')+4).$iec[$i]; } ?>
Basically it reads a file on the server and returns the size of the file.
I pull the function using
<?php print size_hum_read(filesize('image.jpg')); ?>
It returns something like
23.213KB
The problem im coming across is that the last part of the filesize is always 3 digits long, for example
234.432KB
2.328MB
Would anyone know how I could round up the last 3 digits into 2 digits?
Basically
234.432KB
would become
234.43KB
and
2.328MB
would become
2.33MB
Can this be done?
Thanks
Ed
-
its still returning 2, anyone got any bright ideas?
-
Yep, 100%, the whole query code is
$total = mysql_result(mysql_query("SELECT COUNT(*) as Num FROM files GROUP BY categoryid"),0); print $total;
and my database looks like
id categoryid 223 9 224 9 225 10 226 10 227 10 228 11 229 11 230 11 231 12
Can anyone see anything that is wrong?
Thanks
Ed
-
Hi all
I have the following query
SELECT COUNT(*) as Num FROM files GROUP BY categoryid
The problem is that if I run this query in phpmyadmin, I get a total of 4, which is right. But if I run this query within a php page, I only get 2 as a result.
Can anyone tell me why I get a different result?
Any advice would be great
Thanks
Ed
-
Problem is that there is 20,000 records and changing them would be out of the picture, is there a solution with my method?
-
Use the following function
<?php function limitString($string, $maxlength) { if (strlen($string) > $maxlength) { print nl2br(substr($string, 0, $maxlength)."..."); } else { print $string; } } ?>
and then on the text you want to limit, use
<?php limitString($text, 150); ?>
$text being your variable and the 150 marks the limit for the characters
Hope this helps
Ed
-
You can use GROUP BY xx (xx being the field name) if you want to show just one of each and not show any duplicates
-
Hi all
I have a database which holds news, with each news article it has two fields start_date and end_date, these dates are stored as
2007-01-02 (YYYY-MM-DD)
I currently use the following query for my main news page
SELECT * FROM mynews WHERE area='1' AND live = '1' ORDER BY id DESC
What I want to do is to write a new page where it can show old news, this would only show news that has the end_date which is past todays current date. Additionally to this, I want to show just the last 2 months worth of news.
How can I ask it to just query rows with an end_date which has past, but only for 2 months.
Does that make sense?
Can anyone help?
Thanks
Ed
-
Hi prozente
The server wont allow such a function, sadly I cant change any of the servers config and its all passworded up
Should I give up?
-
Hi
mime_content_type doesnt work on my server & im not able to install anything on it.
Is there no way around it, even with a complex function script?
I've hunted online but not found a script which works yet
Any advice anyone?
Thanks
-
With that one, I get
Fatal error: Call to undefined function finfo_open() in
Any ideas?
Thanks so far everyone
-
Nope, there files already stored on my server, this is for internal use so there is no security issue
-
Hi
If I try that, I get the error
Fatal error: Call to undefined function mime_content_type() in C:\www\nagc\members\elements\download.php on line 3
Is there anymore options or what do I need to adjust or add?
Thankd
-
Hi all
I have the following;
$file = $_GET['filename'];
How can I print on screen, the mine type for this file?
Is this easy?
Thanks
Ed
-
cool, thanks
-
thanks, worked great
-
Hi all
Something strange is happening.
I have an if statement, it looks like
if($_GET['folder'] != 4) { $sql = "UPDATE `messages` SET `read` = 1 WHERE `id` = ".$_GET['id'].""; $query = mysql_query($sql); exit(); }
So it should run that query if the url looks like
page.php?folder=1
and if the url looks like
page.php?folder=4
then it shouldnt run, as the ?folder= value in the url is 4
But for some reason, this rule isnt happening and if the url contains ?folder=4
Does anyone know why this isnt working correcly?
Any help would be great
Thanks
Dave
-
russell@2fl.co.uk ??
russell@twofl.co.uk ??
Hi all
I have a sql table which holds events for my website, in each row there is the following fields
id (auto increase)
title (text)
date_from (dates are stored as YYYY-MM-DD)
date_end (dates are stored as YYYY-MM-DD)
I then have a query which looks in that table and returns all rows that have todays date inside the "date_from" field, the query looks like
<?php $meventssql = "SELECT * FROM events WHERE datefrom = '".date('Y-m-d')."'"; $meventsquery = @mysql_query($meventssql,$connection) or die(mysql_error()); while ($meventsrow = mysql_fetch_array($meventsquery)) { ?> <?php print $meventsrow['title']; ?><br> <?php } ?>
How can I re-write the query, so that it uses the date_from & date_end and returns all rows which fall in between those dates, so a row with a date_from set to "2007-01-01" and the date_end set to "2007-01-03", it would then return that row on
2007-01-01
2007-01-02
2007-01-03
Does that make sense?
Been having a hunt around, but cant seem to find anything which would do this.
Can anyone help me?
Thanks in advance
Ed
-
nice one
-
Hi all
I have two varibles, one called
$cycle_through_the_month
and another called
$display_month.
"$cycle_through_the_month" displays a date, such as "5" for the 5th day, "18" for the 18th day and so on
"$display_month" displays a month and year, such as "MARCH 2007" or "NOVEMBER 2007".
Combined together like "$cycle_through_the_month $display_month" produces something like 2 APRIL 2007 or 26 JUNE 2007.
What I want to do is to format these so that they print out like
02/12/2007
or
29/11/2007
Is this possible? Can anyone help?
Thanks in advance
Ed
-
Hi all
I have a insert query which looks like
if (isset($_GET['action']) == 'addmsg') { $forumsgaddsql = "INSERT INTO `forum` SET type = 4, message = '".$_GET['message']."'"; $forumsgaddquery = mysql_query($forumsgaddsql); print"<script>document.location='index.php'; </script>"; exit(); }
This inserts some data into my table 'forum'.
I then have another table called "swear", a sample of this table is
words < FIELD NAME
------
test1
test2
test3
What I want to do, is before the INSERT query runs, check the output of any of the words in $_GET['message'] against all the words in the "swear" table and then return a COUNT for the total number of words matched.
Can this be done? I'm sure i've seen it done before, but I cannot remember how to do this.
Any help would be great
Thanks
Ed
-
Hi all
I have the following varibles
<?
$percent = 20;
$total = 200;
?>
Basically $percent is the percentage, such as 20%
The total is the amount, such as 200
How can I deduct in php $percent from $total, so it would remove 20% from 200?
Is this easy?
Thanks in advance
Dave
-
I get
18/03/2007
1970 01 01
-
thanks, I tried that previously and it worked fine, just seems to be an issue with my code, not sure what it is
Altering a filesize function
in PHP Coding Help
Posted
thanks, but if I do a round then it removes the KB, MB etc off the end of the filesize