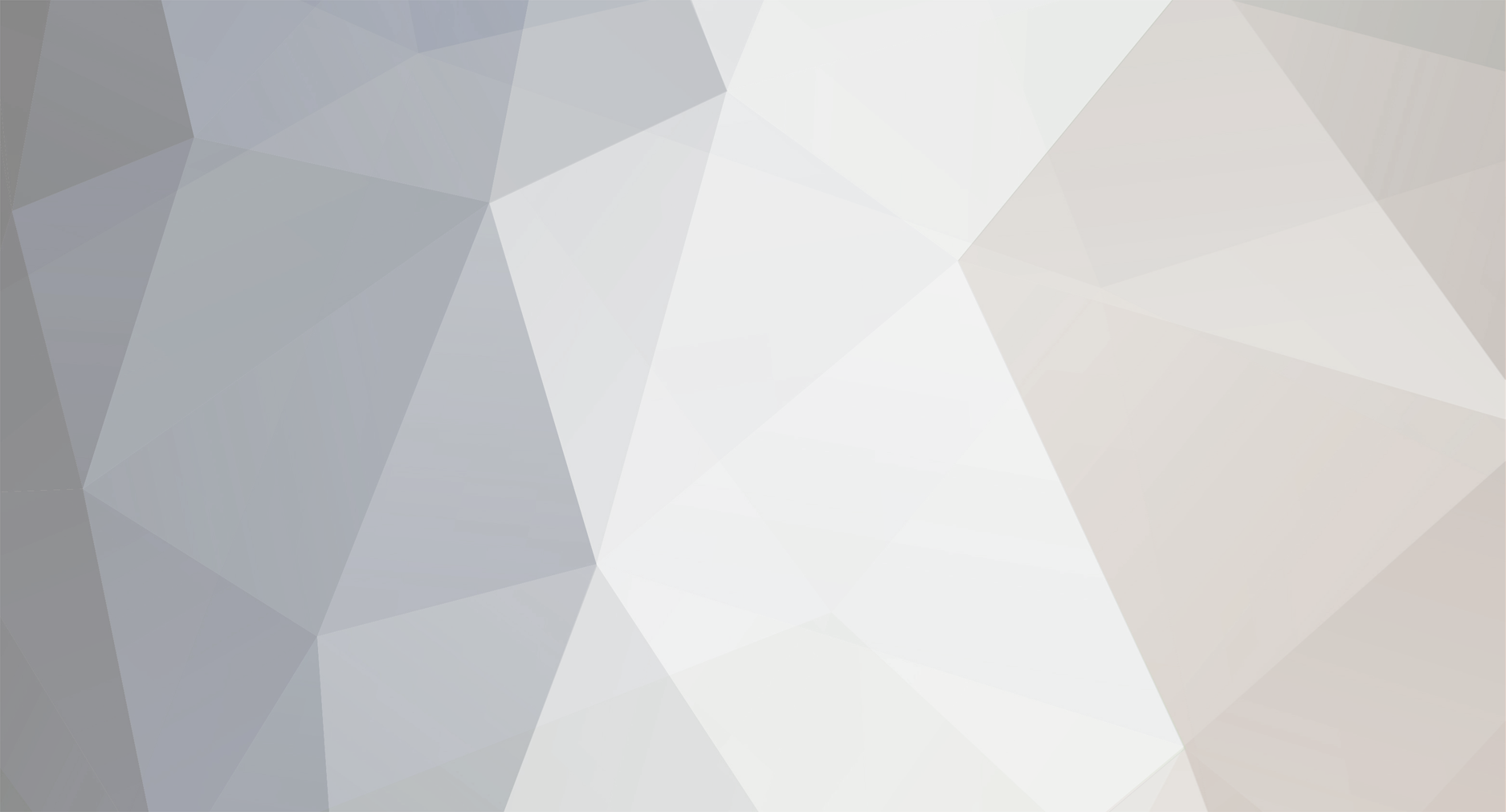
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
Your bad word filter is going to be a little more complex. You would want to catch for example sh*t (which is not caught by your regular filter which searched for shit), you can do that with preg_match('/sh?t/', $badWord) you also would want to replace all regularly used symbols for their letter counterparts eg $h*t which you would replace with sh*t and then replace ? with any symbol that does not have a proper letter counterpart. You would also want to remove symbols that are used to fool these filters eg s.h.i.t. etc..
-
function getFilesByExtension($directory, $validExtensions) { $files = array(); $directory = rtrim($directory, '\/') . DIRECTORY_SEPARATOR; $dh = opendir($imagesFolder); while (false != ($file = readdir($dh))) { $extension = pathinfo($file, PATHINFO_EXTENSION); $extension = strtolower($extension); if (in_array($extension, $validExtensions)) { $files[] = $directory . $file; } } closedir($dh); return $files; } function usort_sortFilesByLastModifiedTimeHelper($file1, $file2) { $mtime1 = filemtime($file1); $mtime2 = filemtime($file2); if ($mtime1 === $mtime2) { return 0; } return $mtime1 < $mtime2 ? -1 : 1; } $images = getFilesByExtension($imagesFolder, array('jpg', 'jpeg', 'gif', 'png')); usort($images, 'usort_sortFilesByLastModifiedTimeHelper'); print_r($images);
-
Sure, but what if you would delete a picture?
-
[Help] How to change variable increments during while statement
ignace replied to cinos11's topic in Third Party Scripts
I re-wrote your code to a simpler, properly indented example. if (!empty($_POST)) { $answers = array( ''/*!important*/, 'a', 'b', 'b', 'a', 'a', 'b', 'c', 'a', 'd', 'a', 'b', 'd', 'a', 'e', 'b', 'c', 'd', 'a', 'b', 'd'); $score = 0; foreach ($answers as $key => $value) { if (isset($_POST['q' . $key])) { if ($value === $_POST['q' . $key]) { $score += 1; } } } $sizeof = sizeof($answers) - 1; if ($sizeof === $score) { echo 'Congratulations, you got a perfect score.'; } else if (floor($sizeof / 2) < $score) {//11+ echo 'Not bad, you scored average.'; } else { echo 'You failed the test.'; } } -
SELECT avg(column) FROM table
-
colors (id, user_id, red, green, blue) If you use my script I provided you earlier, you can insert them like: $user = $_SESSION['id']; foreach ($_POST['red'] as $key => $value) { $red = $value; $green = $_POST['green'][$key]; $blue = $_POST['blue'][$key]; $query = "INSERT INTO colors (user_id, red, green, blue) VALUES ('$user', '$red', '$green', '$blue')"; }
-
Extracting form input parameters from form post
ignace replied to elitegosu's topic in PHP Coding Help
You can't retrieve those values if you didn't submit the form yourself. And cURL can't submit a form, it can however provide the POST data generated by a form to the processing script. -
http://www.phpfreaks.com/forums/index.php/topic,218322.msg999863.html#msg999863
-
You don't need to set static ip's, the ip's won't change in this session. And it depends how old is your "old computer"? Otherwise it's possible you can't use a straight cable and will need a cross-over cable to connect both computers.
-
Show Google Search Term That Brought Visitor to my Website?
ignace replied to cromagnon's topic in PHP Coding Help
Sure, but you need to have them installed. And you need to know where your users are from so can you switch the locale to theirs. -
http://www.php.net/manual/en/class.domdocument.php http://www.php.net/manual/en/class.domxpath.php In the left sidebar you can find more XML-related classes. You can learn XPath here: http://w3schools.com/xpath/default.asp
-
Plus I provided a checkbox solution, maybe read my reply in it's entirety? If you want to limit it to 16 side-by-side you can do that by using CSS: float all <li>'s and put a height on the <ul>.
-
If you would have a more clear look you would have noticed it's 1600px wide.
-
The implementation of indexOf() depends on the object you are calling, like haku pointed out. When it's a string it'll search for sub-strings, when it's a nodelist it will return the index of a node.
-
A URL doesn't need the WWW-part (at least in most cases where the server has been put up properly) eg http://phpfreaks.com/forums/ works too.
-
Show Google Search Term That Brought Visitor to my Website?
ignace replied to cromagnon's topic in PHP Coding Help
Does your server host Windows? If so, then try: setlocale(LC_ALL, ''); Otherwise you can try: setlocale(LC_ALL, 'se_SE'); -
libxml_use_internal_errors(true); $dom = new DomDocument(); if ($dom->load('today.xml')) { $xpath = new DomXPath($dom); foreach ($xpath->query('//item/title') as $node) { echo $node->nodeValue, "<br>\n"; } }
-
.indexOf()?
-
How about updating the visitCount each time a user visits the page?
-
How about? It's that simple or am I missing something? $valueCount = array_count_values($array); foreach ($array as $key => $value) { if ($valueCount[$value] < 3 || $valueCount[$value] > 3) { unset($array[$key]); } } Edit: I tested it using the array of MrAdam and got Array ( [ 0 ] => value 1 [ 1 ] => value 1 [ 2 ] => value 1 [ 3 ] => value 2 [ 4 ] => value 2 [ 5 ] => value 2 )
-
Show Google Search Term That Brought Visitor to my Website?
ignace replied to cromagnon's topic in PHP Coding Help
How does it show up in your HTML source? FF&IE: View > Source -
SELECT * FROM categories AS c, (SELECT * FROM products WHERE category_id = c.id LIMIT 10) AS p WHERE c.id = p.category_id Something like this?
-
fields (field_id, field_log_id, field_name, field_type) logs (log_id, log_name) fields_values (value_field_id, value_value) insert into logs (1, 'Walk The Dog'); insert into fields (1, 1, 'Location', 'String[200]'), (2, 1, 'BathroomStops', 'Integer'), (3, 1, 'FirehydrantsSeen', 'Integer'), (4, 1, 'GoodExercise', 'Boolean'); insert into fields_values (1, 'Pittsburgh'), #Location (2, '3'), #BathroomStops (3, '15'), #FirehydrantsSeen (4, 'False'); #GoodExercise Your application would handle String[200], Integer, and Boolean.
-
I thought it was fun to do: function createHexColorList($name, $callback) { if (!function_exists($callback)) { trigger_error("Function '$callback' does not exist.", E_USER_ERROR); return; } $return = ''; for ($i = 0; $i < 256; ++$i) { $return = $return . $callback($name, dechex($i)); } return $return; } function htmlInput($name, $value) { $value = strtoupper($value); return '<li><label><input type="radio" name="' . $name . '" value="' . $value . '"> 0x' . $value . '</label></li>'; } echo '<form action="#" method="POST">', '<ul id="red">', createHexColorList('red', 'htmlInput'), '</ul>', '<ul id="green">', createHexColorList('green', 'htmlInput'), '</ul>', '<ul id="blue">', createHexColorList('blue', 'htmlInput'), '</ul>', '</form>'; if (!empty($_POST)) { $red = $_POST['red']; $green = $_POST['green']; $blue = $_POST['blue']; echo 'You selected: <span style="background-color: #' . $red . $green . $blue . '">' . $red . $green . $blue . '</span>'; } To allow for multiple colors to be selected: function createHexColorList($name, $callback) { if (!function_exists($callback)) { trigger_error("Function '$callback' does not exist.", E_USER_ERROR); return; } $return = ''; for ($i = 0; $i < 256; ++$i) { $return = $return . $callback($name, dechex($i)); } return $return; } function htmlInputCheckbox($name, $value) { $value = strtoupper($value); return '<li><label><input type="checkbox" name="' . $name . '[]" value="' . $value . '"> 0x' . $value . '</label></li>'; } echo '<form action="#" method="POST">', '<ul id="red">', createHexColorList('red', 'htmlInputCheckbox'), '</ul>', '<ul id="green">', createHexColorList('green', 'htmlInputCheckbox'), '</ul>', '<ul id="blue">', createHexColorList('blue', 'htmlInputCheckbox'), '</ul>', '</form>'; if (!empty($_POST)) { $red = $_POST['red']; $green = $_POST['green']; $blue = $_POST['blue']; foreach ($red as $key => $colorCode) { print 'You selected: <span style="background-color: #' . $red[$key] . $green[$key] . $blue[$key] . '">' . $red[$key] . $green[$key] . $blue[$key] . '</span><br>'; } }
-
http://usceshoppingcenter.com/static/graphics/jun/custom-doc-bg.jpg Download Firefox, install Firebug.