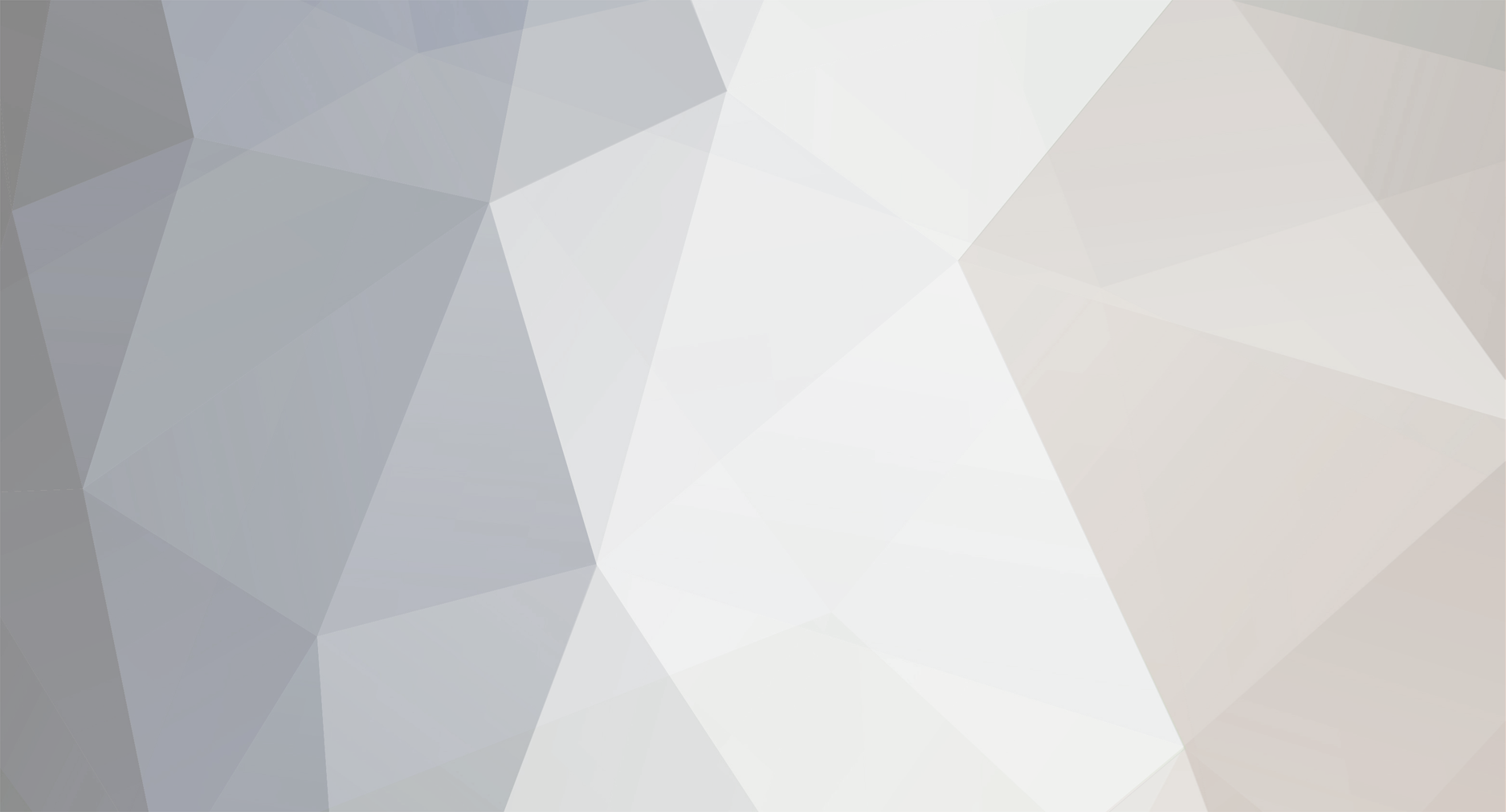
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
I see you are already using objects to handle it, however it's design is very inflexible (which is why you get these headaches). class Cart { private $items = array(); public function addItem(CartItem $item) { $object = $this->findItemByName($item->getName()); if (null === $object) { $this->items[] = $object; } } public function findItemByName($name) { foreach ($this->items as $item) { if (0 === strcasecmp($name, $item->getName())) { return $item; } } return NULL; } } class CartItem { private $id = 0; private $name = ''; private $quantity = 0; private $price = 0.0; private $image = ''; public function getName() { return $this->name; } public function addQuantity($quantity) { if (!ctype_digit($quantity)) { throw new Exception(''); } if (0 > (int) $quantity) { throw new Exception(''); } $this->quantity += (int) $quantity; return $this; } public function equals(CartItem $item) {} } $cart = Cart::fromArray($_SESSION['cart']); .. if (array_key_exists(array('id', 'name', 'quantity', 'price', 'image'), $_POST)) { $id = $_POST['id']; $name = $_POST['name']; .. $temp = new CartItem(); $temp->setId($id); $temp->setName($name); $temp->setQuantity($quantity); .. $item = $cart->findItemByName($temp->getName());//or $cart->findItemById($temp->getId()); if (NULL === $item) {//does not yet exists $cart->addItem($temp); } else { $item->addQuantity(1); } $_SESSION['cart'] = $cart->toArray();//store }
-
I never understood why you would sanitize input at all? Really, you are only helping the attacker in his efforts. If the data isn't what you expected like a piece of text where you expected a number or a negative where you expected a positive number, then just reject it. The end-user is messing with the variables, shut him up.
-
Can anyone please explain me basic structure of a simple forum?
ignace replied to angel1987's topic in Application Design
Something like: CREATE TABLE forum ( id smallint NOT NULL AUTO_INCREMENT, parent_id smallint, .. INDEX forum_parent_id_FK (parent_id), FOREIGN KEY forum_parent_id_FK (parent_id) REFERENCES forum (id) ON UPDATE NO ACTION ON DELETE NO ACTION, PRIMARY KEY (id) ) ENGINE = InnoDB; CREATE TABLE forum_reply ( id integer NOT NULL AUTO_INCREMENT, replyto_id integer NOT NULL, forum_id smallint NOT NULL, .. INDEX forum_reply_replyto_id_FK (replyto_id), FOREIGN KEY forum_reply_replyto_id_FK (replyto_id) REFERENCES forum_reply (id) ON UPDATE NO ACTION ON DELETE NO ACTION, INDEX forum_reply_forum_id_FK (forum_id), FOREIGN KEY forum_reply_forum_id_FK (forum_id) REFERENCES forum (id) ON UPDATE NO ACTION ON DELETE NO ACTION, PRIMARY KEY (id) ) ENGINE = InnoDB; You would create a forum and add sub-forums by specifying the forum.parent_id. When someone creates a thread you would specify to which a user replies by using forum_reply.replyto_id. -
$curl = curl_init(); curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_RETURN_TRANSFER, FALSE); curl_exec($curl); $url = curl_getinfo($curl, CURLINFO_EFFECTIVE_URL); $query = parse_url($url, PHP_URL_QUERY);
-
You can't, embed is not part of both (X)HTML and will therefor not validate. I don't know of any alternative, HTML5 maybe?
-
Post your entire script as it is because if the script is as you posted it above then you already know what the problem is.
-
javascript prompt box then store input on database
ignace replied to violinrocker's topic in PHP Coding Help
You can't modify the default behavior of a prompt but you can create your own (modal) and apply Ajax calls afterwards to store the input. -
<answer<?php print ($row['correct'] === $row['answer1']) ? ' correct="y"' : ''; ?>><?php print $row['answer1']; ?></answer>
-
session_set_cookie_params(3600); session_start(); session_set_cookie_params must be called before session_start.
-
Getting Values From MySQL to display them on PHP
ignace replied to PascalNouma's topic in PHP Coding Help
I'm probably one of the few who thought of the Decorator pattern when they looked at your attached image. -
smashing magazine - http://www.smashingmagazine.com/ six revisions - http://www.sixrevisions.com/ script & style - http://scriptandstyle.com/ nettuts - http://net.tuts.com/ css-tricks - http://css-tricks.com/ content with style - http://www.contentwithstyle.co.uk/ a list apart - http://www.alistapart.com/
-
even any number i input or even with no input on the track page, would still run the database. $correct_tracking_numbers=array(22333); if(in_array(22333,$correct_tracking_numbers)){ Is also always true. Like -Karl- said what do you want it to do?
-
curl_setopt($curl, CURLOPT_RETURNTRANSFER, FALSE); curl_exec($curl);
-
Attaching a DIV to your mouse cursor may have some nasty effects on your design.
-
Formatting number to currency with the (commas etc)
ignace replied to elabuwa's topic in Javascript Help
Why not just use money_format. For those systems that do not have the function, you can find one in the comments. -
$curl = curl_init(); curl_setopt($curl, CURLOPT_URL, $url); curl_exec(); $url = curl_getinfo($curl, CURLINFO_EFFECTIVE_URL); $query = parse_url($url, PHP_URL_QUERY);
-
Yes. Or change your doctype to XHTML
-
You also have intval, floatval, doubleval, and strval.
-
You are using the incorrect DTD. You are using the HTML 4.01 DTD and defining an XML namespace which is only implemented as of XHTML 1.0. Remove the xmlns.
-
Continuing on this, I would extend it's functionality a little further and define the relationship status between 2 users (friends, lovers, haters, ..). Although you may not need the functionality now, it may well in the future.
-
Please help me in starting multiple streams of a file.
ignace replied to rigy73's topic in PHP Coding Help
You want to let people (who do not have a premium account) download through your script using a stored premium account? -
if (Get_QString('Post') == "True") { $Date_Day = GetPost('Day'); $Date_Month = GetPost('Month'); $Date_Year = GetPost('Year'); echo date('l', mktime(0, 0, 0, $Date_Month, $Date_Day, $Date_Year)); }
-
You can't. You can however create your own image and change the default: cursor: url(my-cursor.cur);
-
set_time_limit(0); class Crawler implements IteratorAggregate { private $dom = null; private $urlList = null; public function __construct() { $this->dom = new DomDocument(); $this->urlList = new ArrayObject(); } public function getUrlList() { return $this->urlList; } public function getIterator() { return $this->urlList->getIterator(); } public function crawl($url) { $this->urlList->append($url); if ($dom->loadHtmlFile($url)) { foreach ($dom->getElementsByTagName('a') as $a) { $href = $a->attributes->getNamedItem('href'); if (!$this->_isUrl($href)) continue;//trail ends here $this->crawl($href); } } } private function _isUrl($url) { return FALSE !== parse_url($url); } } Let's hope none lead to external sources or this script may run forever.