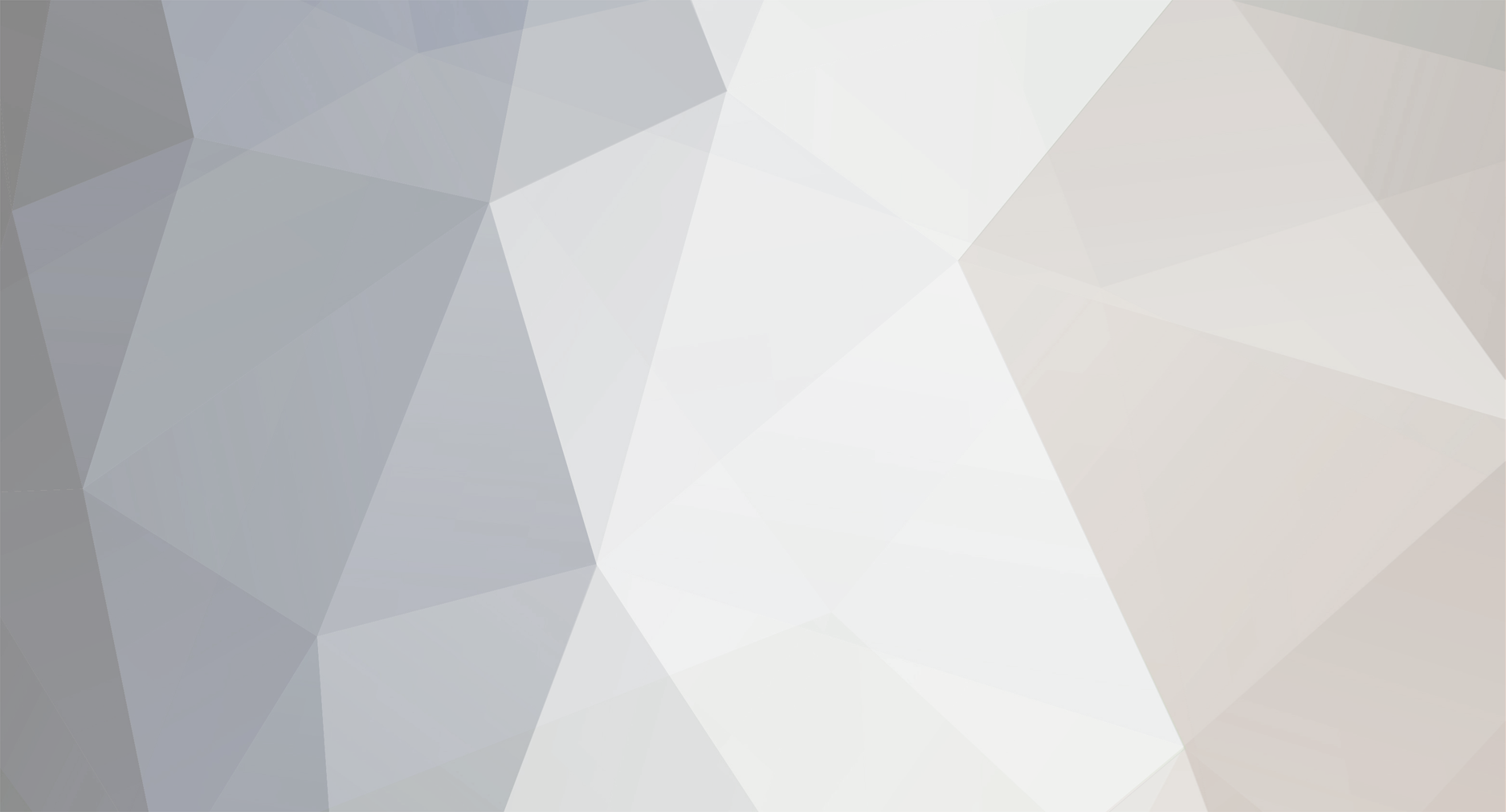
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
[HOW] Auto Delete a Folder content by Cron Job after no of days!!!
ignace replied to natasha_thomas's topic in Miscellaneous
I have dived into Linux permissions and to remove a file/directory you need the write permission. The below script should do the job and report if something isn't correct. $directoryPath = realpath('path/to/directory'); if (!is_dir($directoryPath)) { error_log("Directory $directoryPath does not exist."); exit(0); } function cleanDirectory($directoryPath, $recursive = FALSE) { if (!is_readable($directoryPath) || !is_writable($directoryPath)) { error_log("Can't delete directory $directoryPath as you don't have the correct permissions."); return FALSE; } $folderEmpty = TRUE; foreach (scandir($directoryPath) as $file) { $filePath = realpath("$directoryPath/$file"); if (is_dir($filePath)) { if (!cleanDirectory($filePath, $recursive)) { $folderEmpty = FALSE; } continue; } if (!deleteFile($filePath)) { $folderEmpty = FALSE; } } return $folderEmpty ? @rmdir($directoryPath) : FALSE; } function deleteFile($filePath) { if (!is_writable($filePath)) { error_log("Can't delete file $filePath as you don't have the correct permissions."); return FALSE; } return @unlink($filePath); } -
And what do you think I calculated?
-
I think it's quite obvious that he wants a specific image to show up (therefor the ID parameter) instead of only the first one always? Why use the LIMIT-clause, if ID is a PK it will only be present once as the PK is a unique field. And why use while (..) if you just LIMIT-ed to 1 and evaluated mysql_num_rows($run) == 1? $image = mysql_fetch_assoc($run); header('Content-type:image/' . mime_content_type($image['image'])); readfile('PATH_TO_IMAGES/' . $image['image']); Would suffice.
-
@James session_start()? header("Content-type:image/jpeg");? What if the content came from a GIF?
-
No, you put: <div class="picture"><img src="photo.php?id=.."></div> And in photo.php you decide on the appropriate header and echo out the data, something like: header('Content-Type: ' . $row['mime']); echo $row['photo'];
-
It won't work.
-
some questions just for my own knowledge
ignace replied to chaiwei's topic in Other Programming Languages
1. What is the use of iterator SPL since we can use while loop, foreach and for loop? I can't find its useful usage for me. can you give me an example? class MyIterator implements Iterator, Countable { private $key = 0; private $count = 0; private $data = array(); public function __construct($data) { $this->data = $data; $this->count = sizeof($data); } public function rewind() { $this->key = 0; } public function valid() { return $this->key < $this->count; } public function next() { ++$this->key; } public function current() { return $this->data[$this->key]; } public function key() { return $this->key; } public function count() { return $this->count; } } 2. Mysql is opensource? if I used it to create a system using php and mysql and sell it to others. Do I need to pay for that? Yes. No. 3. Stored procedure is better? I have look in the sourcecode and I found that my friend was using stored procedure. As they told me stored procedure is save server memory for running millions of query. but the cons is they are hard to maintain. Is that true? A stored procedure will not save server memory as when it returns a result it still has to be loaded in to memory for PHP to process it. 4. My friends was using prostgre sql integrate with c language. he said that will be faster compare to just use php and mysql. Is that true? Is there any way to integrate mysql with c? or I can use php integrate with c. will it faster compare to postgresql with c? C is indeed faster then PHP, PHP is build on top of C so it's slightly slower. 5. what is the best/stable free c/c++ compiler and can use with apache, php and mysql server? g++? G++ is a language not a compiler. Here is a list of compilers http://www.thefreecountry.com/compilers/cpp.shtml, please note you should use the appropriate compiler for your operating system. -
Set the correct character set.
-
[WARNING] Question requires ULTRA PRO GODLIKE MYSQL MASTER![/WARNING]
ignace replied to this.user's topic in MySQL Help
SELECT, MySQL is optimized for SELECT queries. -
Euhm that's not how you calculate row size, something similar to this would be more correct (although I'm no expert in this): id + search + count = int + varchar + int = 4 bytes + 255 bytes + 4 bytes = 263 bytes (assuming you use varchar(255)) 263 bytes * 1M records = 263 000 000 = 263 M 263 bytes * 1G records = 263 000 000 000 = 263 G If your website is popular you may reach 1M records (which I doubt you'll ever get to). A more realistic number would be: id + search + count = mediumint + varchar(32) + mediumint = 3 bytes + 32 bytes + 3 bytes = 40 bytes 40 bytes * 1M = 40M 40 bytes * 1G = 40G
-
Damn, can't believe I didn't notice that.
-
Try if (empty($argv[1])) { echo 'Please provide a search term.'; exit(0); } $dom = new DomDocument(); if (!$dom->load('TVGuide.xml')) { echo 'Failed to load TVGuide.xml'; exit(0); } foreach ($dom->getElementsByTagName('Series') as $serie) { if ($argv[1] == $serie->nodeValue) { foreach ($serie->parentNode->childNodes as $childNode) { echo $childNode->nodeName, ': ', $childNode->nodeValue, PHP_EOL; } echo PHP_EOL; } } Should create something like: Start: 2001-07-05T20:00:00 Duration: PT30M Series: Brookside Title: Start: 2001-07-05T20:30:00 Duration: PT30M Series: Brookside Title:
-
try mysql_query($SQL) or die('Query failed: ' . $SQL . ' (' . mysql_error() . ')');
-
Split your script into smaller chunks and process it one at a time.
-
May this be what you are looking for http://code.google.com/intl/nl/apis/ajaxlanguage/
-
Zend_Controller_Dispatcher_Exception: Invalid controller specified (error)
ignace replied to ethereal1m's topic in Frameworks
It has nothing to do with the version difference. And it's best to not disable the error handler as you otherwise will not be notified of any occured error's returning a blank page. And yes you should incorporate the ErrorController, it will tell you what the error is. -
You need a Paginator like: class Paginator implements Iterator { private $key = 0; private $sizeOf = 0; private $itemsPerPage; private $data = array(); private $activePage = 1; public function __construct($array, $itemsPerPage) { $this->data = $array; $this->sizeOf = sizeof($array); $this->itemsPerPage = abs((int)$itemsPerPage); } public function getItemsForPage($pageNumber) { $pageNumber = $pageNumber > 0 ? $pageNumber : 1; $pageNumber = $pageNumber < $this->getNumberOfPages() ? $pageNumber : $this->getNumberOfPages(); $this->activePage = $pageNumber; $this->key = $this->calculateOffset($pageNumber); return $this; } public function getActivePage() { return $this->activePage; } public function getNumberOfPages() { return ceil($this->sizeOf / $this->itemsPerPage); } public function valid() { return $this->key < $this->calculateOffset($this->getActivePage() + 1) && $this->key < $this->sizeOf; } public function key() { return $this->key; } public function next() { $this->key++; } public function rewind() { //$this->key = 0; } public function current() { return new ArrayObject($this->data[$this->key], ArrayObject::ARRAY_AS_PROPS); } private function calculateOffset($pageNumber) { return ($pageNumber - 1) * $this->itemsPerPage; } public function hasNextPage() { return $this->getActivePage() < $this->getNumberOfPages(); } public function hasPreviousPage() { return $this->getActivePage() > 1; } } Use it like: $rows = $db->execute('SELECT * FROM table')->fetchAll(); $paginator = new Paginator($rows, 20);//20=items per page $offset = $_GET['page_number']; $items = $paginator->getItemsForPage($offset); if ($paginator->hasNextPage()) { echo "has next"; } else { echo "doesn't have next"; } if ($paginator->hasPreviousPage()) { echo "has previous"; } else { echo "doesn't have previous"; } foreach ($items as $item) { print_r($item); }
-
Most probably they contact an interface that communicates with the version control system and returns the updated files since your release version.
-
Zend_Controller_Dispatcher_Exception: Invalid controller specified (error)
ignace replied to ethereal1m's topic in Frameworks
Whenever you get an error (read Exception) your dispatcher will catch this otherwise uncaught Exception (to remain functional towards your end-users) and create an error handler (Zend_Controller_Plugin_ErrorHandler) object, add it to the internal messaging system, sets the active controller to ErrorController, and signals the dispatcher the request is yet unhandled (isDispatched(false)). So your dispatcher tries to find and execute ErrorController which it can't find and therefor throws an Exception that isn't caught as the Dispatcher itself doesn't handle it's own Exception's. In order to work with the Zend framework you need a deep understanding of it's internal workings. I suggest you familiarize yourself before digging any deeper as it's only getting more and more complex with each new release. -
I wouldn't do that and display a CAPTCHA after 3 times instead. The reason for this is that my name is quite popular apparently and I always have to come up with different variations on my name which means that every time I login I have to go over multiple possibilities before I get it right to actually login.
-
[WARNING] Question requires ULTRA PRO GODLIKE MYSQL MASTER![/WARNING]
ignace replied to this.user's topic in MySQL Help
I don't think so, if you are using MyISAM as an engine and performing a count(*) instantly returns the number of records as MyISAM stores the number of records as-is and thus only has to retrieve the variable value without even having to go over the table and count each record. -
Yeah like end which you clearly don't know either
-
Zend_Controller_Dispatcher_Exception: Invalid controller specified (error)
ignace replied to ethereal1m's topic in Frameworks
You have an error and it's trying to dispatch ErrorController which you are missing. class ErrorController extends Zend_Controller_Action { public function errorAction() { $errorHandler = $this->_getParam('error_handler'); var_dump($errorHandler);//does this contain an exception property? print $errorHandler->exception->getMessage(); } } -
basename
-
Depends how does WP do it's update? Automatic? Or does it just tell you there is a new update and refers you to the download site?