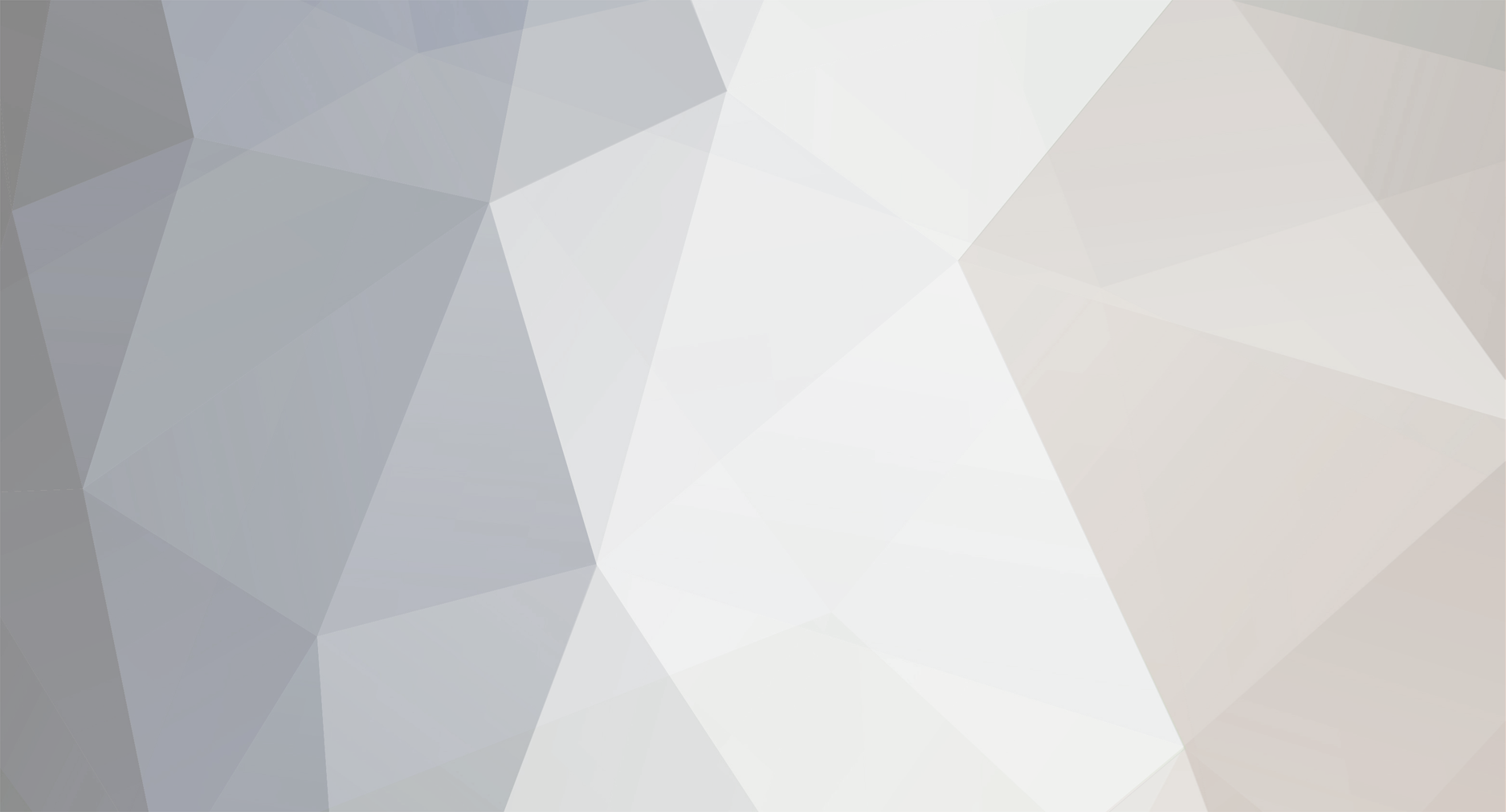
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
So you basically are looking for an ACL (Access-Control List) There are different ways of implementing such behavior if you want to keep the roles or groups known and don't want to allow the end-user to make their own roles you could use something like: class User { const ROLE_GUEST = 'guest'; const ROLE_MEMBER = 'member'; const ROLE_AUTHOR = 'author'; const ROLE_EDITOR = 'editor'; const ROLE_PUBLISHER = 'publisher'; const ROLE_ADMINISTRATOR = 'administrator'; private $role = self::ROLE_GUEST; public function getRole() { return $this->role; } public function setRole($role) { if ($this->getRole() === $role) return; switch ($role) { case self::ROLE_GUEST: case self::ROLE_MEMBER: case self::ROLE_AUTHOR: case self::ROLE_EDITOR: case self::ROLE_PUBLISHER: break; // OK, valid case self::ROLE_ADMINISTRATOR: $this->isAdministrator() or throw new Exception('Only administrators may assign administrator privilege'); break; default: throw new Exception("Invalid role specified '$role'"); break; // break is ignored } $this->role = $role; } public function isGuest() { $this->getRole() === self::ROLE_GUEST; } public function isMember() { $this->getRole() === self::ROLE_MEMBER; } public function isAuthor() { $this->getRole() === self::ROLE_AUTHOR; } public function isEditor() { $this->getRole() === self::ROLE_EDITOR; } public function isPublisher() { $this->getRole() === self::ROLE_PUBLISHER; } public function isAdministrator() { $this->getRole() === self::ROLE_ADMINISTRATOR; } public function save() { /* logic */ } } In your code you would use it like: if ($action === 'edit' && ($u->isEditor() or $u->isAdministrator())) { If you are confused by: $this->isAdministrator() or throw new Exception('Only administrators may assign administrator privilege'); Then the manual says: // The constant false is assigned to $f and then true is ignored // Acts like: (($e = false) or true) $f = false or true; http://www.php.net/manual/en/language.operators.logical.php
-
How would I go about structuring the following scheduler script?
ignace replied to ghurty's topic in PHP Coding Help
I don't understand what you are actually trying to accomplish but I'm guessing that if I would book a conference room I would want all of our staff to be in the same room not spread across many rooms. I also would want to conference for a little longer then 5 minutes usually just discussing the agenda already takes about 20 minutes or so. I would opt for a table structure like: room (id, name, capacity) booking (id, room_id, alias, cost, start, estimated_end, is_paid) Then to tell which conference rooms are available at $time SELECT * FROM room JOIN booking ON room.id = booking.room_id WHERE $time NOT BETWEEN start AND estimated_end+$treshold AND room.capacity >= $capacity -
Where can I find my favourite topics? The old design allowed me to mark topics as favourite or something I kept them as a reference but I can't find them anymore. So, where are they?
-
What CMS will you be using?
-
Your explanation reveals your database has been badly designed. You only need 2 tables: news and news_category. The table news contains any news (sports, politics, ..) and the table news_category contains the categories: sports, politics, .. So if you want all sports related news articles: SELECT * FROM news, news_category WHERE news_category.id = news.category_id AND news_category.name = 'Sports';
-
WHMCS Action Hooks...Arrays & Variables & Migrains Oh My!
ignace replied to Mike Solstice's topic in Applications
Try: $cpanel_user = $vars['params']['username']; -
- Your frontpage is missing a lot like: what is this game about? how does it look? what is in the game? where can I find help/support or a manual? - "If You Forgot Your Password Sent An Email At info@eurogangster.com With Your Username" Sure I'll e-mail you about my lost password for my #1 account
-
Organising hierarchical data in an array (using foreach?)
ignace replied to rpmorrow's topic in PHP Coding Help
Written code only serves as an example and may not fit your current project requirements. The example just gives you a basic idea of what may accomplish your goal. My code has a lot missing and it's up to you to fill in the blanks and properly test it before using it in a production environment. -
Organising hierarchical data in an array (using foreach?)
ignace replied to rpmorrow's topic in PHP Coding Help
Well these only serve the purpose of an example and I am not going to correct any error because you shouldn't be using them in production environments they are not meant to. An alternate solution production ready may be Zend_Navigation. Easy to use http://framework.zend.com/manual/en/zend.navigation.html -
Organising hierarchical data in an array (using foreach?)
ignace replied to rpmorrow's topic in PHP Coding Help
Here's an improved version: $rows = array(); while ($row = mysql_fetch_array($result, MYSQL_ASSOC)) { $pid = intval($row['parent_id']); $rows[$pid][] = $row; } $root = new Menu(); foreach ($rows as $pid => $array) { foreach ($array as $row) { $id = intval($row['id']); if (!isset($rows[$id])) { $root->add(new MenuLeaf($row)); } else { $root->add(new MenuComposite($row)); } } } print $root->render(); abstract class MenuComponent { private $data = array(); public function __construct($data = array()) { $this->data = $data; } abstract public function render(); } class MenuComposite extends MenuComponent { private $components = array(); public function add($key, MenuComponent $item) { $this->components[$key] = $item; } public function render() { if (!sizeof($this->components)) return ''; $html = '<a href="#">' . $this->data['label'] . '</a><ul>'; foreach ($this->components as $component) { $html .= '<li>' . $component->render() . '</li>'; } return $html . '</ul></a>'; } } class Menu extends MenuComposite { public function render() { if (!sizeof($this->components)) return ''; $html = '<ul>'; foreach ($this->components as $component) { $html .= '<li>' . $component->render() . '</li>'; } return $html . '</ul>'; } } class MenuLeaf extends MenuComponent { public function render() { $data = $this->getData(); return '<a href="#">' . $data['label'] . '</a>'; } } -
Organising hierarchical data in an array (using foreach?)
ignace replied to rpmorrow's topic in PHP Coding Help
Use the composite pattern to achieve this: $root = new MenuComposite(); while ($row = mysql_fetch_array($result, MYSQL_ASSOC)) { $mc = new MenuLeaf($row); $root->addItem($mc); } print $root->render(); abstract class MenuComponent { private $_data; public function __construct(array $data) { $this->_data = new ArrayObject($data, ArrayObject::ARRAY_AS_PROPS); } public function getData() { return $this->_data; } public function getId() { return $this->getData()->id; } public function getParentId() { return $this->getData()->parent_id; } public function addItem(MenuComponent $mc) {} public function render() { return ''; } } class MenuComposite extends MenuComponent { private $_items; public function getItems() { if (null === $this->_items) { $this->_items = new ArrayObject(); } return $this->_items; } public function addItem(MenuComponent $mc) { if (0 !== $mc->getParentId()) { // composite $this->getItems()->offsetSet($mc->getId(), $mc); } else if ($this->getItems()->offsetExists($mc->getParentId())) { // leaf $p = $this->getItems()->offsetGet($mc->getParentId()); if ($p instanceof MenuComposite) { $p->addItem($mc); } else { // convert MenuLeaf to MenuComposite $p = new MenuComposite($p->getData()); $p->addItem($mc); $this->getItems()->offsetSet($mc->getParentId(), $p); } } else { throw new Exception('Parent item with id ' . $mc->getParentId() . ' does not exist.'); } } public function render() { $list = '<ul>'; foreach ($this->_items as $id => $mc) { $list .= '<li>' . $mc->render() . '</li>'; } $list .= '<ul>'; return $list; } } class MenuLeaf extends MenuComponent { public function render() { return '<a href="' . $this->getData()->href . '">' . $this->getData()->label . '</a>'; } } -
add a watermark to your images.
-
http://www.amazon.com/Understanding-Search-Engines-Mathematical-Environments/dp/0898714370
-
question about a Reservation database (help)
ignace replied to rogueblade's topic in Application Design
You won't need a customers table because have you ever had to give your full details when you went to a restaurant? No. all required information comes down to a reservation name (which can be anything), date and time of the reservation, and the number of people accompanying you. Possible use-cases: 1. A customer cancels his reservation 2. A customer has a coupon code or some other means (birthday, ..) by which he receives a reduction on his meal 3. The owner wants to find the most popular dish 4. The owner wants to find out if all none cancelled reservations have been paid for this day, this month, .. 5. The owner wants to find the total income (excl VAT) for today, this month, year, .. 6. The owner wants to find out which is the most popular choice of payment among his customers 7. .. menu (id, name, description, price) consumption (menu_id, reservation_id) reservation (id, tabletop_id, for, at, size, cancelled) tabletop (id, name, seats) checkout (id, reservation_id, subtotal, total, paid_by, coupon_code, reduction) This is by no means the end try to find some more use-cases discuss with your teacher, your parents or visit a restaurant. You don't need all information but you should have a good idea of the basic workflow within a restaurant. -
item_1 through item_7 in one table is bad design. Give us some more details and I may help you normalize your database.
-
Site Architecture help - need to define needed DB's
ignace replied to Gabriel_Haukness's topic in PHP Coding Help
The best would have been if you had defined your entire application in clear business rules both in terms of your application as your database. http://en.wikipedia.org/wiki/Business_rule -
For future reference create a binary table: a | b | -a | -b | -a+-b | -a.-b | 0 | 0 | 1 | 1 | 1 | 1 | 0 | 1 | 1 | 0 | 1 | 0 | 1 | 0 | 0 | 1 | 1 | 0 | 1 | 1 | 0 | 0 | 0 | 0 | -a+-b = !a||!b -a.-b = !a&&!b As you can see in the above table it only returns true when both !a & !b return true where !a || !b only returned false when both !a & !b were false
-
Not if you use PHP you can easily determine the active page: <li<?php print isActivePage($href, $_SERVER['REQUEST_URI']) ? ' class="active"' : ''; ?>><a href="<?php print $href; ?>"><?php print $label; ?></a></li>
-
Site Architecture help - need to define needed DB's
ignace replied to Gabriel_Haukness's topic in PHP Coding Help
A great help with any project is textual analysis and an ERD (entity-relationship diagram). Your text after stripping out all none db related material: I got: -- code refers to a 2-letter country code http://www.modemsite.com/56k/_ccodes.asp language (id, name, code) -- polyglot means a person who speaks many languages -- 'speak' indicates how well a person speaks that language 3 out of 5 for example where 5 is perfect or native speaker polyglot (language_id, user_id, speak) user (group_id, employee_of, street_address, ..) group job (language_id, requested_by, assigned_to, deadline) job_type (id, name) -- pph is pay per hour -- i put pph here because depending on the client they may charge more or less per hour invoice (job_id, client_id, hours, pph) -- company where an interpreter works agency (id, name, street_address, ..) -
That's because of how || works. I assume you want to show the not-authorized message whenever someone tries to do something while not an admin nor a staff member. Try: function sessionAuthenticate ( ) { // Are you loged in is admin, when trying to asscess this page if (!isset($_SESSION["admin"]) && !isset($_SESSION["staff"])) { // Set error message $_SESSION["message"] = "<div class='error'>You are not authorised to access the URL: <br> {$_SERVER["REQUEST_URI"]}</div>"; // After error message move to login.php header ("Location: login.php"); exit; } }
-
Just add an additional class attribute to your tag <li class="active"><a href="index.php">Homepage</a></li> And add the CSS: .active a, a:active { background: #F00; color: #000; }
-
Just add an additional class attribute to your tag <label for="username" class="input-mandatory">Username</label> <input type="text" name="username" id="username"> And add the CSS: .input-mandatory:after { content: " *:"; color: #C00; }
-
session_set_cookie_params
-
And you question is?
-
- Invalid CSS & HTML - The video starts playing from the moment you enter the website (let the user control when he wants to hear something) - Almost all important text (logo, Beezag is an invite-only..) is contained in an image (Google can't read images as a result http://www.google.com/search?q=beezag) - No description meta tag - Titles remain the same between page switches (which adds to #3) - When I hit tab the first it selects is your sitemap on the bottom - A login form but no auto-select