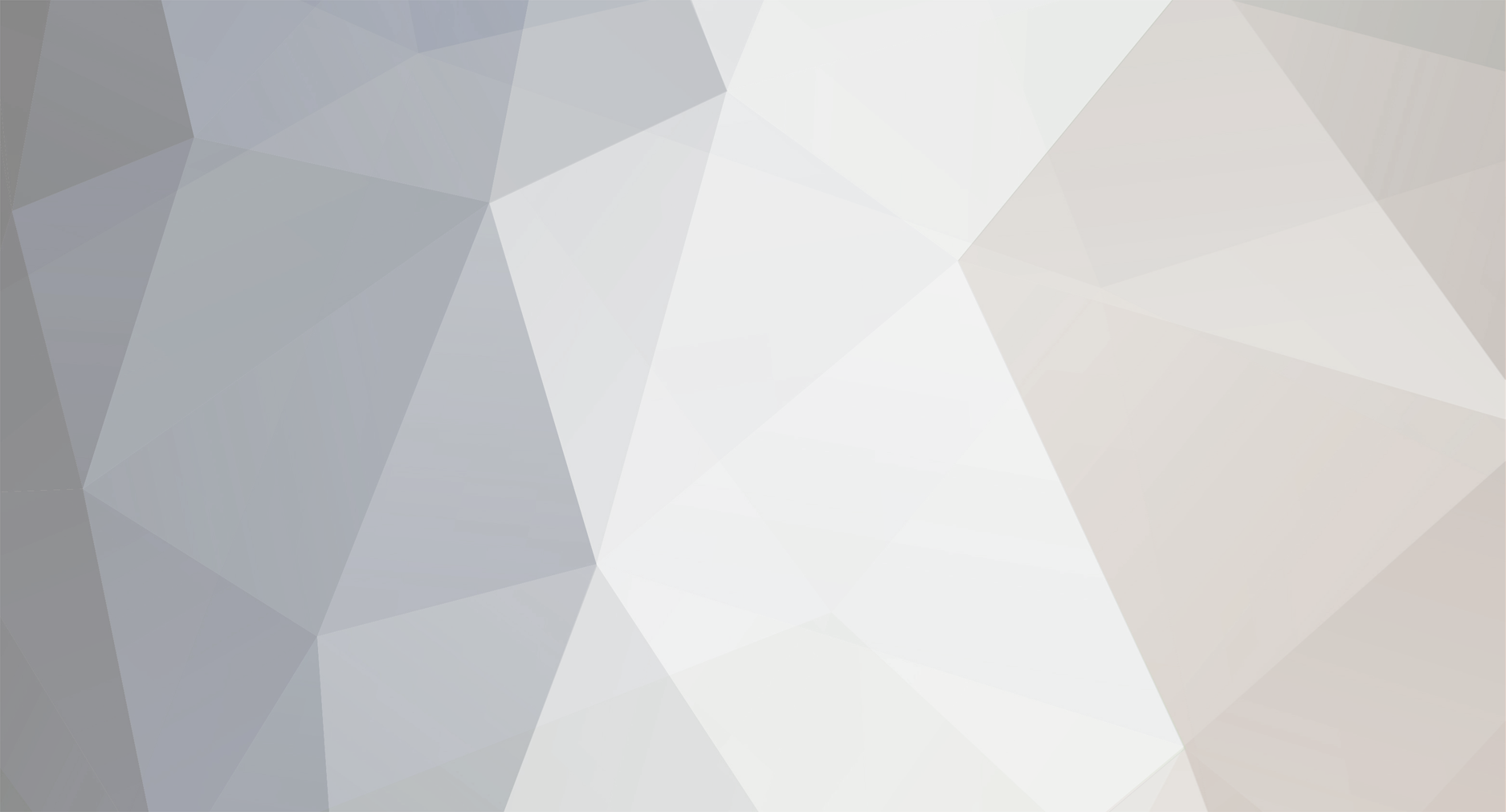
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
Ah the good old Assembler days put all registers on stack modify the registers call the values from stack in reverse order and place them back into their original registers
-
What am I doing wrong that it is not pulling the values of $dbhost, $dbuser, $dbpass from the constants.php file? It doesn't know the variable nor its value because of a differing scope. To get these variables inside of your class you'll need to use the 'global' keyword which I highly discourage. Here's a rewrite of your database class: class Database { protected $_connection = null; protected $_lastResult = null; public function __construct($pass, $name = null, $user = 'root', $host = 'localhost') { $this->openConnection($pass, $user, $host); if (null !== $name) { $this->select($name); } } public function __destruct() { $this->closeConnection(); } public function openConnection($pass, $user = 'root', $host = 'localhost') { $this->_connection = mysql_connect($host, $user, $pass); } public function closeConnection() { if (is_resource($this->_connection)) { mysql_close($this->_connection); $this->_connection = null; } } public function select($name) { return mysql_select_db($name); } public function query($query) { return $this->_lastResult = mysql_query($query, $this->_connection); } public function getRowCount() { return mysql_num_rows($this->_lastResult) or mysql_affected_rows($this->_lastResult);//experimental } }
-
$this->$conn = mysql_connect($dbhost, $dbuser, $dbpass) or die("Could not connect to server."); doesn't work because it doesn't know the values $conn, $dbhost, $dbuser, $dbpass $this->$dbselect = mysql_select_db($dbname, $conn) or die("Could not connect to the database."); should be: $this->$dbselect = mysql_select_db($dbname, $this->$conn) or die("Could not connect to the database."); but again it doesn't know the value of $dbselect and $this->$conn
-
$random = 'DAD' . substr(base64_encode(rand(1000000,9999999)),0,7);
-
Login scrips Members & How to see if they are logged in
ignace replied to gnetuk's topic in PHP Coding Help
sorry told you i was a noob see what a comma can do lol created table now ill let you know if i get it working thankx mate The table is worthless if you use it like that. I assumed you already had a users table (or whatever you want to call it) apparently you do not. The full SQL code would then be: DROP TABLE IF EXISTS users; CREATE TABLE users ( users_id INTEGER NOT NULL AUTO_INCREMENT, users_username VARCHAR(16), users_password VARCHAR(40), users_email_address VARCHAR(96), #optional users_last_click_at DATETIME, users_last_login_at DATETIME, #optional users_registered_at DATETIME, #optional PRIMARY KEY (users_id) ); The lines that have #optional in them can be removed if you don't want to store that information. -
Login scrips Members & How to see if they are logged in
ignace replied to gnetuk's topic in PHP Coding Help
My programming experience involves 4 till 5 years of PHP, SQL, VB.NET, Java, C++, Assembler and COBOL. None programming related experience involves project management. Malevolence already did. <?php include('sesschk.php'); include('dbconnect.php'); $id = $_SESSION['users_id'];//or wherever you get the users id from $q = "UPDATE users SET users_last_click_at = now() WHERE users_id = $id";//replace users and users_last_click_at with your table name and field name $q2 = 'SELECT * FROM users WHERE users_last_click_at BETWEEN now() - 300 AND now()';//same here mysql_query($q) or die('MySQL Error: '.$mysql_error());//remove the 'or die(..)' part once you are uploading it or replace it altogether with or trigger_error('MySQL Error: '.$mysql_error()); $r2 = mysql_query($q2); $u = mysql_num_rows($r2); ?> 'or die()' is a bad practice and you should avoid it use 'or trigger_error()' instead. I'm not going into detail about why? Another member of these forums already did (Daniel0 'or die() must die', http://www.phpfreaks.com/blog/or-die-must-die) -
Login scrips Members & How to see if they are logged in
ignace replied to gnetuk's topic in PHP Coding Help
my sql comes up with a error when i tryy to run this i carnt create the table Ofcourse this is just a part of a table. It indicates the fields you need in your table definition (not necessarily the same field definitions so you may need to adjust them). -
Because you prolly did the print_r() outside of the loop whereby you ask for the children of the last assigned $parentId
-
LOL all I said was LOL and you are already cursing? I didn't say your post is a waste of time. And it's quiet hard to get banned here, so..
-
print_r($categories); print_r($categories[$id]);
-
Login scrips Members & How to see if they are logged in
ignace replied to gnetuk's topic in PHP Coding Help
I don't need to provide the php code for this it's pretty obvious what and where you would use it. I only forgot to add the (obvious) update query which is called on every page request: UPDATE users SET users_last_click_at = now() WHERE users_id = $id Avoid the fuzz. On each page request will the users_last_click_at of a logged in user be updated. Using the above select query will give you all users who clicked between 5 minutes ago and now (someone who is actively clicking (and a member) at your website is IMO online). The only downside on my code is that it will only show online members and will ignore guests. -
Login scrips Members & How to see if they are logged in
ignace replied to gnetuk's topic in PHP Coding Help
CREATE TABLE users ( users_last_click_at DATETIME, ); SELECT * FROM users WHERE users_last_click_at BETWEEN now() - 300 AND now() -
Because it should be: while ($row = mysql_fetch_assoc($result)) mysql_fetch_*() retrieves one record from the result set in differing formats (object(), array(), assoc() (proxy to array($result, MYSQL_ASSOC), row() (proxy to array($result, MYSQL_NUM)
-
html forms: showing sql result in select option list
ignace replied to bobocheez's topic in PHP Coding Help
<select name="subcategory"> <optgroup label="category1"> <option value="subcategory1">Subcategory #1</option> <option value="subcategory2">Subcategory #2</option> </optgroup> </select> Remember that you can't create a optgroup inside a option therefor you can only go as deep as multiple subcategories for a category. Subcategories can not on their turn contain Sub-Subcategories. Note: optgroup is not selectable. -
Wordpress thumbs - need to link to home page and articles...
ignace replied to mr_man000's topic in PHP Coding Help
Same as before post in third-party. http://www.phpfreaks.com/forums/index.php/topic,267179.msg1260255.html#msg1260255 -
Wordpress Gallery - Linking images with story - Please Help!
ignace replied to mr_man000's topic in Applications
Your posting in the wrong section. Post it into third-party you are more likely to get an answer there then here. The peeps in third-party may be familiar with Wordpress. -
I didn't say that. I do think that you may apply some more validation on input like: $sel_sec = $_GET['sec']; can use a good dosage of validation. I could pass anything to sec as your code doesn't check what it contains.
-
If globals are wrong how are we then supposed to program? $var1 = 'hello';//global variable (can be accessed as: $GLOBALS['var1']) $var2 = 'world';//global variable (can be accessed as: $GLOBALS['var2']) I didn't.
-
Do you have these quotes on file? May it only show one same quote during the whole day or show random quotes on each refresh? Random quotes: $lines = file('path/to/quotes/file'); print $lines[array_rand($lines)];
-
MSDN: MySQL: TRUNCATE is faster
-
An even simpler solution would be: if ($array == array_intersect(range(min($array), max($array)), $array))) { //in sequence } This will return true for: $a1= array(1,2,3,4,5,6); and returns false for: $a2= array(3,4,5,12,15);
-
ShopProductWriter writes out your cart items. ShopProduct represents a product. You would use it like: $spw = new ShopProductWriter(); $spw->addProduct(new ShopProduct('Product #1', 'Firstname', 'MainName', 'expensive')); $spw->addProduct(new ShopProduct('Product #2', 'Firstname', 'MainName', 'expensive')); $spw->addProduct(new ShopProduct('Product #3', 'Firstname', 'MainName', 'expensive')); $spw->addProduct(new ShopProduct('Product #4', 'Firstname', 'MainName', 'expensive')); print $spw->write(); I won't recommend using it on a production server (like it allows 'expensive' as a price..) though plus there are many things this class does not make advantage of (__toString(), ..).
-
[SOLVED] List Fields Only If They Have A Value
ignace replied to dwayneparton's topic in PHP Coding Help
print !empty($val) ? $message . trim($field)." = ". trim($val). "\n" : ''; -
I think this should do the trick: function checkSequence($array) { $lowest = min($array); $inSequence = true; $sizeof = sizeof($array); for ($i = 0; $i < $sizeof; ++$i) { if ($lowest !== $array[0]) { $inSequence = false; break; } else if (isset($array[$i + 1]) && $array[$i] > $array[$i + 1]) { $inSequence = false; break; } } } return $inSequence; }