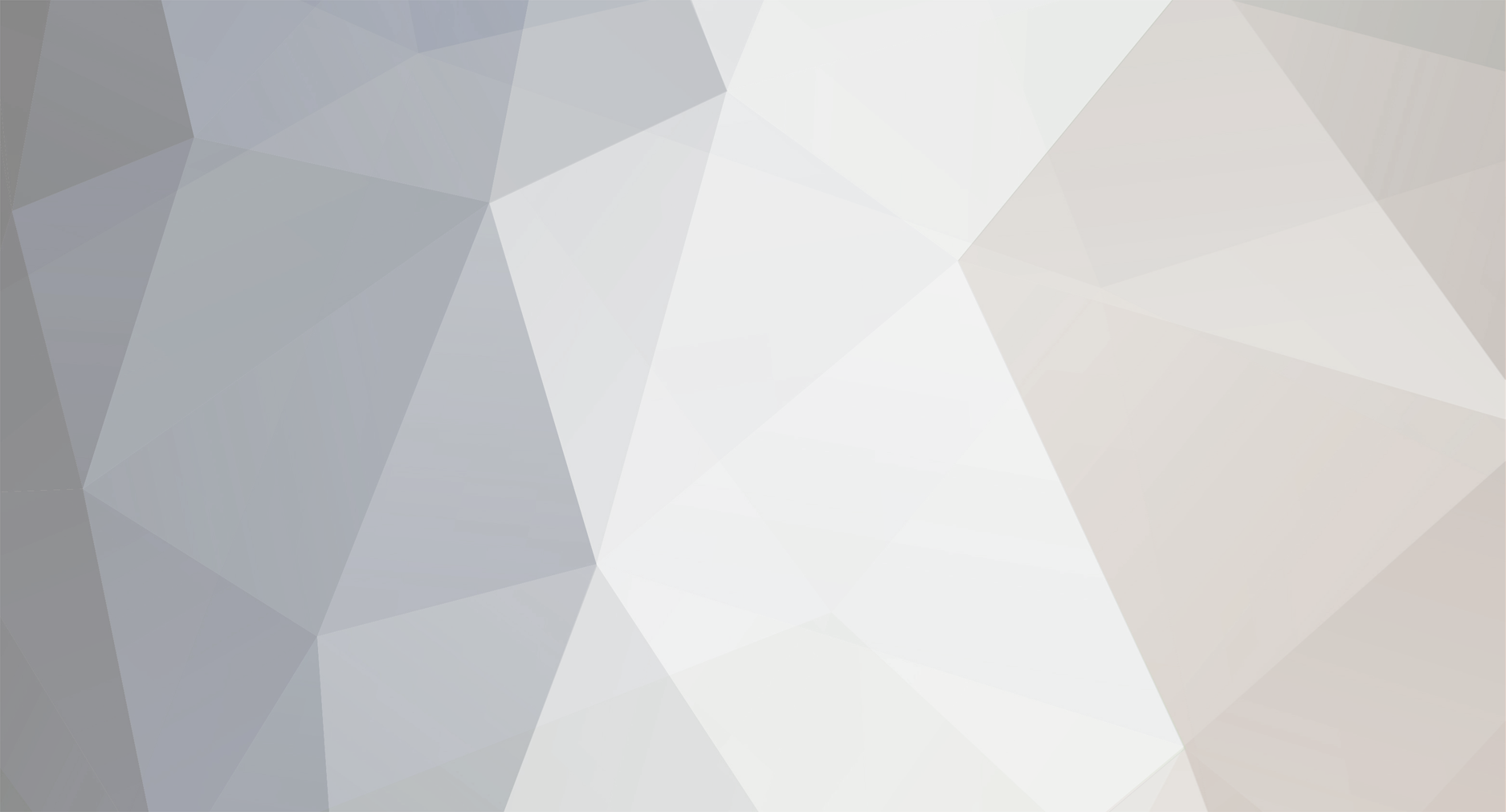
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
Just like you learned QuickBasic: practice. You have a webserver so you don't need to install one locally. Go and download Netbeans (the PHP version) and write a PHP script, Netbeans will assist you in code completion and what not, but most important, it tells you when it's valid. Once it's done and valid, upload it to your webserver (you can configure Netbeans to do this upon saving) and access it through your browser. Do this long enough and instead of copy-pasting the scripts from the internet you'll be writing these scripts yourself with ease
-
Simple OOP question: best practice for site-wide constants
ignace replied to johnsmith153's topic in PHP Coding Help
Not a valid argument since I can do the same thing with simple objects and a clever architecture. -
Simple OOP question: best practice for site-wide constants
ignace replied to johnsmith153's topic in PHP Coding Help
I still fail to see why you keep insisting on using a Singleton/Multiton design at all for something that does not need it. Use objects, not classes, it's Object-Oriented after all not Class-Oriented -
Simple OOP question: best practice for site-wide constants
ignace replied to johnsmith153's topic in PHP Coding Help
The correct term would then be Multiton not Singleton. IMO you should avoid static methods when not necessarily needed, they create a not so easy to replace dependency on your code. -
accessing methods of outer class from methods in inner class...
ignace replied to Hall of Famer's topic in PHP Coding Help
Like? -
Simple OOP question: best practice for site-wide constants
ignace replied to johnsmith153's topic in PHP Coding Help
Why do you insist on using constants? Why not a configuration file, like the one below: <?php return array( 'company_name' => 'My Company Name', 'company_slogan' => 'My Company Slogan', .. ); Through an admin (or installer) interface you can now change these values and write them back to the file: // example, don't copy and use in your production scripts file_put_contents('config.php', '<?php' . PHP_EOL . PHP_EOL . 'return array(' . PHP_EOL . toArrayKeyValNotation($config) . PHP_EOL . ');'); This has the advantage of everything being in one place and it's easier to modify and write back because there is no function for example that gives you only your own declared constants, if you wanted to write these to a file, for example. -
Simple OOP question: best practice for site-wide constants
ignace replied to johnsmith153's topic in PHP Coding Help
Just wrap you site-wide configuration settings in a configuration object (or an array) and pass that to your company object using Dependency Injection. Something like: class Company { private $_name; public function __construct($cfg) { $this->_name = $cfg['company_name']; } } An example DI container can be found here: https://github.com/fabpot/Pimple A use-case can be found here: https://github.com/fabpot/Silex/blob/master/src/Silex/Application.php -
How do you handle system objects used in almost every other class?
ignace replied to Hall of Famer's topic in PHP Coding Help
I like Pimple as a DI container: https://github.com/fabpot/Pimple A use-case can be found at: https://github.com/fabpot/Silex/blob/master/src/Silex/Application.php -
accessing methods of outer class from methods in inner class...
ignace replied to Hall of Famer's topic in PHP Coding Help
I don't get why you have a UserProfile at all... It has no id, so it's not an entity. It wraps data which I feel should belong to the User object, and it's only responsibilities are formatting. IMO, at best, your UserProfile IS a View. But since this formatting is part of your domain these should also be in your User class. Just like the below function would: public function getFullName() { return $this->getTitle() . '. ' . $this->getFirstname() . ' (' . $this->getAlias() . ') ' . $this->getLastName(); } If you have a requirement that states that you always should address the user with it's full name. Admin and Mod are Special Case's of User so these should inherit from User (but since PHP does not support method overloading, it's better to make these responsibilities also part of User and just check with isModerator() and isAdmin() of some sort). I also fail to see how Friend and Banned could be Decorators? Banned could be a Value Object that tells how the user was banned (perm, temp) and how long (in case of temp) but not a Decorator. And Friend should be just an instance of a User as element in a _friends property array. $user1->isFriendsWith($user2); //implementation public function isFriendsWith(User $u) { Assert::true(!$this->equals($u)); return $this->_friends->contains($u); } -
Restricting database records based on allowed "cities" or "counties" fields
ignace replied to vestport's topic in MySQL Help
So now you have payed $200 to find out you still need to learn to program... -
The script appeared to hang and returned nothing. Still don't quite understand though why it works outside of the loop without the escapeshellarg() and not inside the loop. Other people have reported the same problem but they didn't have the same case as me (passed the var as second parameter) hopefully this post will help someone in the future who made the same silly mistake
-
Phew. Found it I just needed to add escapeshellarg Can't believe I didn't try it in the first place, since it's on the PHP exec() function manual..
-
This code works outside of the while, but not inside while ($row = mysql_fetch_assoc($res)) { $host = $row['host']; $cmd = exec("mysql -h$host -e \"show status like 'wsrep_cluster_size'\""); echo $cmd; } I have found nothing on google, stackoverflow or the php manual that explains why this does not appear to work inside a loop?
-
It's possible that Google SE reads HTML and executes JS just like a browser does (except there will be no UI). In most cases this also means it follows the correct path (fallback to href when onclick does not return something usable for example).
-
Design/Architecture: code for matching shows from filenames
ignace replied to huddy's topic in PHP Coding Help
You can always at a later point refactor your code and create re-usable components out of it. For example: class tvfilename extends RecursiveIteratorIterator { When a string is provided we assume it's a directory path: public function __construct($input, $filter = null) { if (is_string($input)) { parent::__construct(new RecursiveDirectoryIterator($input, $filter)); } else { parent::__construct($input); } } When traversing you would pass the filename/whatever to a parser: public function current() { return $this->_parser->parse(parent::current()); } So at this point you should get an StdClass from current() with properties, if you want it to be of a certain class then you should also supply a factory to your parser: class EpisodeParser { private $_factory; public function __construct(Factory $factory = null) { $this->_factory = $factory; } public function parse($string) { // parsing if (!is_null($this->_factory)) { $parsed = $this->_factory->make($parsed); } return $parsed; } } All the responsibilities divvid up You would use it like this: $tv = new tvfilename\tvfilename('/path/to/dir', array('*.txt', '*.db', '*.url')); foreach ($tv as $episode) { echo 'SEASON: ', $episode->season, "\r\n", 'EPISODE: ', $episode->episode, "\r\n" 'SERIE: ', $episode->serie; } A simple intuitive interface -
Even simpler then: switch (strtolower($_GET['brand'])) { case 'compaq': echo '<img src=".." ..'; break; }
-
Be sure to donate some of that money you just made with some help from us
-
The Egg of Columbus: http://jsfiddle.net/BJ9XP/2/
-
Just change the src="" of the <img/> and point it to the new location (you may need to append something like ?_=Math.rand() to the image filename for it to work).
-
Design/Architecture: code for matching shows from filenames
ignace replied to huddy's topic in PHP Coding Help
Well we can reasonably assume it will only be used to traverse directories, so i see no need to divving up the responsibilities. In what other ways are you planning on using it? Why have you started this project in the first place? -
Design/Architecture: code for matching shows from filenames
ignace replied to huddy's topic in PHP Coding Help
You should add more responsibilities to your object, currently it does nearly nothing: $tv = new tvfilename\tvfilename('/path/to/dir', array('*.txt', '*.db', '*.url')); foreach ($tv as $episode) { echo 'SEASON: ', $episode->season, "\r\n", 'EPISODE: ', $episode->episode, "\r\n" 'SERIE: ', $episode->serie; } You could abstract what it traverses and get something like: $tv = new tvfilename\tvfilename(new tvfilename\RecursiveDirectoryFilterIterator('/path/to/dir', array('*.txt', '*.db', '*.url'))); Or in case of a DB: $tv = new tvfilename\tvfilename(new tvfilename\DbRecordIterator($dbAdapter)); -
wigwambam already showed you:
-
Design/Architecture: code for matching shows from filenames
ignace replied to huddy's topic in PHP Coding Help
Now only if those pirates would just name those files consistently... -
ROFLMFAO ... you just started writing JS or something? *Creative* solution Do as jesirose said: window.location.href = "informationPage.html?desc=" + desc; And what scootsah said: <textarea disabled id="infoArea"><?php echo htmlentities(strip_tags($_GET['desc'])); ?></textarea> But it's quite possible the .html has to be .php otherwise the PHP will not get parsed (until you tell it to parse .html as well of course).