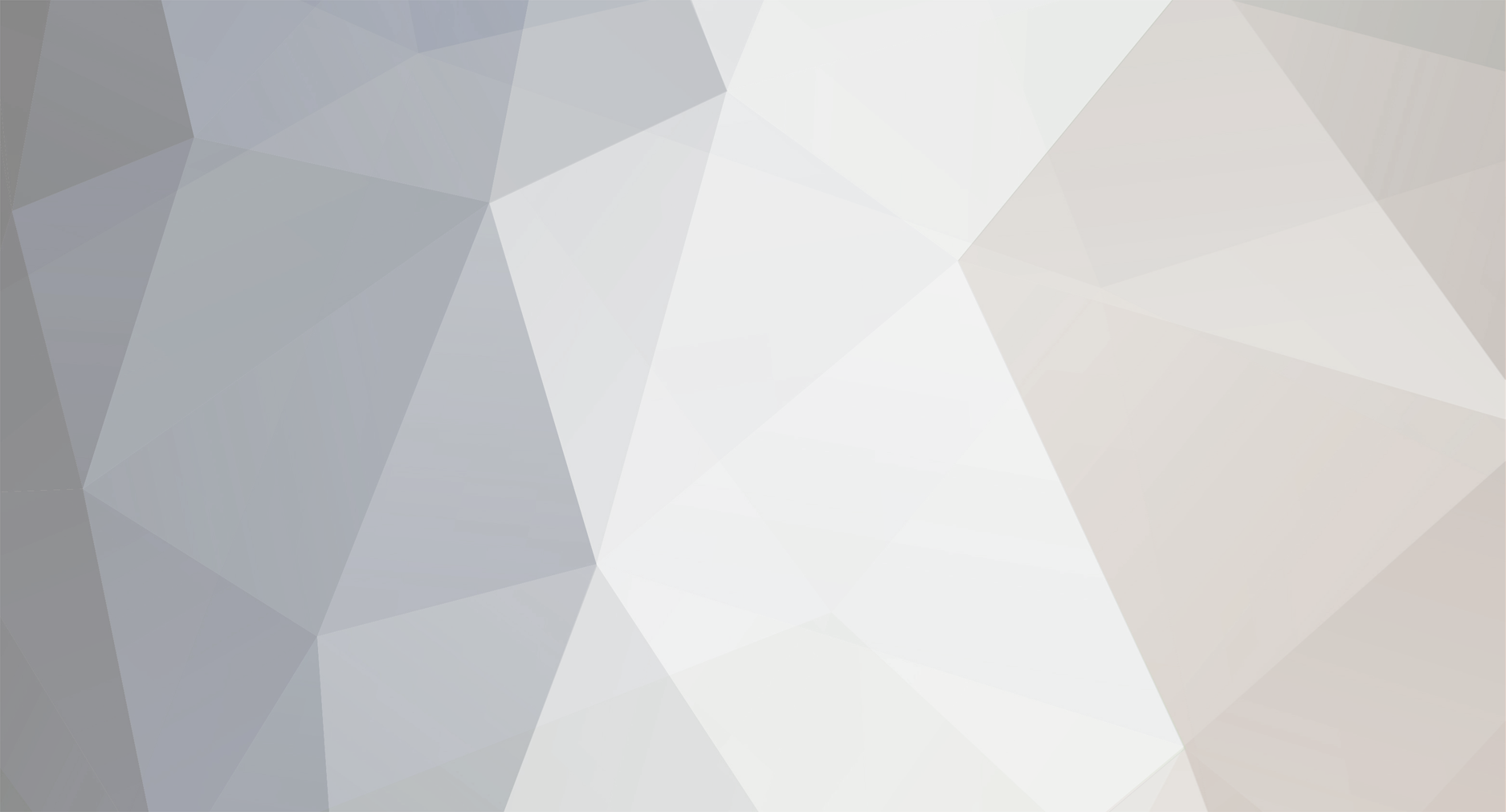
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
I fail to see how this seems challenging? Use the host address to query the DB for the website details SELECT * FROM websites WHERE domain = 'website.com' and the content SELECT * FROM content WHERE domain_id = 1. Sure there are a few caveats (www.website.com versus website.com being just one).
-
Does the below produce what you want? And if not then copy-paste (or link) the result. $sql = ' SELECT hungergames_users.id, hungergames_records.user_id, hungergames_records.victories, hungergames_records.biggest_kill_streak, hungergames_records.most_chests_opened, hungergames_records.total_chests_opened, hungergames_records.lastlogin, hungergames_records.longest_lifespan, hungergames_records.total_lifespan, hungergames_records.total_points, hungergames_records.most_points, hungergames_users.donor, hungergames_users.user FROM hungergames_records JOIN hungergames_users ON hungergames_users.id = hungergames_records.user_id ORDER BY hungergames_records.total_points DESC '; $result = mysql_query($sql, $mysql_link); if ($result && mysql_num_rows($result)) { $rank = 1; while ($row = mysql_fetch_array($result)) { echo ($rank++), ' ', $row['id'], ' ', $data['user'], '<br>', PHP_EOL; } }
-
Read http://www.codinghorror.com/blog/2012/04/speed-hashing.html
-
If you are using shared hosting then it will be unlikely for you to install them. You can try contacting the host provider and ask them if they could install the extension but it's also unlikely they will. An other option you may have is finding the poisson distribution in pure PHP like: http://www.phpmath.com/build02/PDL/docs/package.php?view=6c892fdf713fd5c15ff0cdd31daf79463531ceef Which uses PEAR (quite likely to be installed on your host). A full index of pure PHP mathematical functions can be found at: http://www.phpmath.com/build02/
-
Do not write your PHP code in your HTML, but organize them in functions and use those in your HTML. This separates your core app functionality from your HTML and makes it easier to edit/re-use later on. functions.php function get_monthy_sales_for_year($year, $mysql_link) { $sql = 'SELECT * FROM MonthlySales WHERE Year = %d'; $sql = sprintf($sql, $year); return mysql_fetch_all($sql, $mysql_link, MYSQL_ASSOC); } function get_monthly_sales($mysql_link) { return mysql_fetch_all('SELECT * FROM MonthlySales', $mysql_link, MYSQL_ASSOC); } function mysql_fetch_all($sql, $mysql_link, $mode = MYSQL_ASSOC, $callback = null) { $rows = array(); $result = mysql_query($sql, $mysql_link); if ($result && mysql_num_rows($result)) { while ($row = mysql_fetch_array($result, $mode)) { if (is_callable($callback) && ($crow = call_user_func($callback, $row))) { $row = $crow; } $rows[] = $row; } } return $rows; } /** * @param array|ArrayAccess $array */ function get_param($array, $name, $default = null) { if (!isset($array[$name])) { return $default; } return $array[$name]; } function get_year_range() { // this may be used at several places but changing the actual range is done here. return range(2000/*start*/, 2001/*end*/); } As you can see this looks much cleaner than your example. The below code assumes you have $link = mysql_connect('localhost', 'root', 'root'); somewhere. monthly_sales.php <?php include 'functions.php'; ?> <form action="<?php /*leave empty if processed on same page*/ ?>" method="post" name="form_filter"> <select name="year"> <option value="all">All</option> <?php foreach (get_year_range() as $year): ?> <option value="<?php print $year; ?>"><?php print $year; ?></option> <?php endforeach; ?> </select> <input type="submit" value="Filter"> </form> <table> <?php if (!get_param($_POST, 'year')) { <?php foreach (get_monthly_sales($link) as $sale): ?> <tr> <td><?php print $sale['Id']; ?></td> <td><?php print $sale['ProductCode']; ?></td> <td><?php print $sale['Month']; ?></td> <td><?php print $sale['Year']; ?></td> <td><?php print $sale['SalesVolume']; ?></td> </tr> <?php endforeach; ?> <?php else: ?> <?php foreach (get_month_sales_for_year(get_param($_POST, 'year'), $link) as $sale): ?> <tr> <td><?php print $sale['Id']; ?></td> <td><?php print $sale['ProductCode']; ?></td> <td><?php print $sale['Month']; ?></td> <td><?php print $sale['Year']; ?></td> <td><?php print $sale['SalesVolume']; ?></td> </tr> <?php endforeach; ?> <?php endif; ?> </table>
-
What is the technical term used for this feature?
ignace replied to usman07's topic in PHP Coding Help
The code I provided will only work for the type=detached, you will have to write the other functions to handle semi-detached, etc... If you do not feel comfortable writing PHP then either: 1) go out and buy a book and read until you feel comfortable with the language or 2) pay someone to do it for you (or teach you, having someone to ask questions directly is often easier). -
What is the technical term used for this feature?
ignace replied to usman07's topic in PHP Coding Help
You can make this as simple/straightforward as: function get_detached_houses($mysql) { $sql = 'SELECT * FROM real_estate WHERE type_id = 5'; // 5 = Detached House $rows = mysql_fetch_all($sql, $mysql, MYSQL_ASSOC); return $rows; } You can check what the user asked for by creating URL's like: estate.php?type=semi-detached estate.php?type=detached .. In your code you would use the type to display the correct properties: $mysql = mysql_connect(/*...*/); if (get('type') === 'detached') { $estate = get_detached_houses($mysql); } else if (get('type') === 'semi-detached') { // .. } else { $estate = get_houses(); } mysql_fetch_all and get are custom functions: function get($name, $default = null) { return array_key_exists($name, $_GET) ? $_GET[$name] : $default; } // executes a query and returns a result set function mysql_fetch_all($sql, $mysql, $mode = MYSQL_BOTH) { $rows = array(); $res = mysql_query($sql, $mysql); if ($res && mysql_num_rows($res)) { while ($row = mysql_fetch_array($res, $mode)) { $rows[] = $row; } } return $rows; } -
It should be noted though that these will not be unique. As an example I already had a duplicate after generating 960 serials. I hope this was just an exercise and you didn't wanted to use these for software licensing or something?
-
One line of code (without indentiation) to create the serial. print implode('-', // concatenate each group with '-' str_split( // split them into lenghts of 4 implode('', // concatenate them to a string array_rand( // pick out 12 elements array_flip( // array_rand reads keys, not values array_merge( // merge ranges range(0, 9), // 0 - 9 range('A', 'Z') // A - Z ) ), 12) ), 4) );
-
How Does PHPFreaks Feel About the Hate PHP Often Gets as a Language?
ignace replied to Masna's topic in Miscellaneous
You underestimate us! We can be really neutral on these subjects... For we are enlightened. -
What do you have so far?
-
I second that. There is no such thing as /home/users on Windows.
-
How Does PHPFreaks Feel About the Hate PHP Often Gets as a Language?
ignace replied to Masna's topic in Miscellaneous
Try Scheme. -
How Does PHPFreaks Feel About the Hate PHP Often Gets as a Language?
ignace replied to Masna's topic in Miscellaneous
The fun part about PHPWTF is that the website is written in PHP. Makes me think the owner is like: Choice of language is just that.. You don't like it, move on! Go program Java, boy! That said you shouldn't be limiting yourself to one language anyway. -
There really is no need to create your own Time object as PHP has a built-in equivalent DateTime
-
The solution to your problem can be found here!
-
It's humor, Jim. But not as we know it!
-
$query_handle->bindParam($i, $value[$i]); should be: $query_handle->bindParam($i, $binds[$i]); The reason you are not getting any errors is because you don't have error reporting set to On (at the very least you would have gotten something like `Undefined index` since $value is empty) or display errors have been turned Off. error_reporting(E_ALL); ini_set('display_errors', 1); For best effect these should be turned on in your php.ini
-
And you shouldn't. At the very least export these rows to an archive table.
-
Without having some demo data it's hard to write this query off the top of my head, but could something like this work? ( -- invoice_numbers marked as inactive should be considered for re-use SELECT invoice_number AS `Missing From`, invoice_number AS `To` FROM payments WHERE active=0 ) UNION ( -- find unused invoice_numbers -- Source: http://www.artfulsoftware.com/infotree/queries.php#71 SELECT a.invoice_number + 1 AS `Missing From`, MIN(b.invoice_number) - 1 AS `To` FROM payments a, payments b WHERE a.invoice_number < b.invoice_number GROUP BY a.invoice_number HAVING `Missing From` < MIN(b.invoice_number) )
-
OOP - Recommended Template/Theme/Page Design Structure
ignace replied to Bladescope's topic in PHP Coding Help
your centos server should be fine. -
1) The while is unnecessary. while ( $a = mysql_fetch_assoc($r) ) { global $siteName, $siteMotto, $siteIco; $siteName = $a['site_name']; $siteMotto = $a['site_motto']; $siteIco = $a['site_ico']; } Instead just write: $a = mysql_fetch_assoc($r); global $siteName, $siteMotto, $siteIco; $siteName = $a['site_name']; $siteMotto = $a['site_motto']; $siteIco = $a['site_ico']; 2) PHP has a built-in data-access layer called PHP Data Objects (PDO), use it! There are plans to ditch the mysql extension in favor of mysqli, so avoid it, if possible or use PDO. That said use PDO using the mysqli driver. 3) Create classes to group related functionality. abstract class DataStoreService { private $pdo; public function __construct(PDO $pdo) { $this->pdo = $pdo; } protected function query($sql) { $stmt = $this->pdo->query($sql); if (false === $stmt) { throw new ErrorException($this->pdo->errorInfo(), $this->pdo->errorCode()); } return $stmt; } } class Site {/*represents the site*/} class SiteSettings extends DataStoreService { public function getSiteData() { $stmt = $this->query('SELECT * FROM siteSettings ORDER BY id DESC LIMIT 1'); if ($stmt->rowCount()) { return $stmt->fetchObject( 'Site' ); } return null; // no site data available! bad? throw an exception if it is. } } class Article {/*represents an article*/} class Articles extends DataStoreService { public function getLatest() { $stmt = $this->query('SELECT * FROM testDB ORDER BY created DESC LIMIT 4'); if ($stmt->rowCount()) { return $stmt->fetchAll( PDO::FETCH_CLASS, 'Article' ); } return array(); // nothing here! } 4) Avoid the use of the global keyword. You don't need it! Read up on Depedency Injection and Service Containers
-
OOP - Recommended Template/Theme/Page Design Structure
ignace replied to Bladescope's topic in PHP Coding Help
There aren't that many IDE's out there that fully support the new v5.4 features like traits. -
OOP - Recommended Template/Theme/Page Design Structure
ignace replied to Bladescope's topic in PHP Coding Help
Yup. Hands down the best IDE out there for PHP development. The current beta (EAP) version fully supports PHP v5.4 and almost all of Subversion 1.7. They recently added support for {@inheritDoc} and they show code coverage for all lines in your files. And that's just scratching the surface. When I looked at all the supported features, my first thought was: IT'S OVER 9000!!! -
Something like this? http://www.jankoatwarpspeed.com/post/2008/05/22/CSS-Message-Boxes-for-different-message-types.aspx