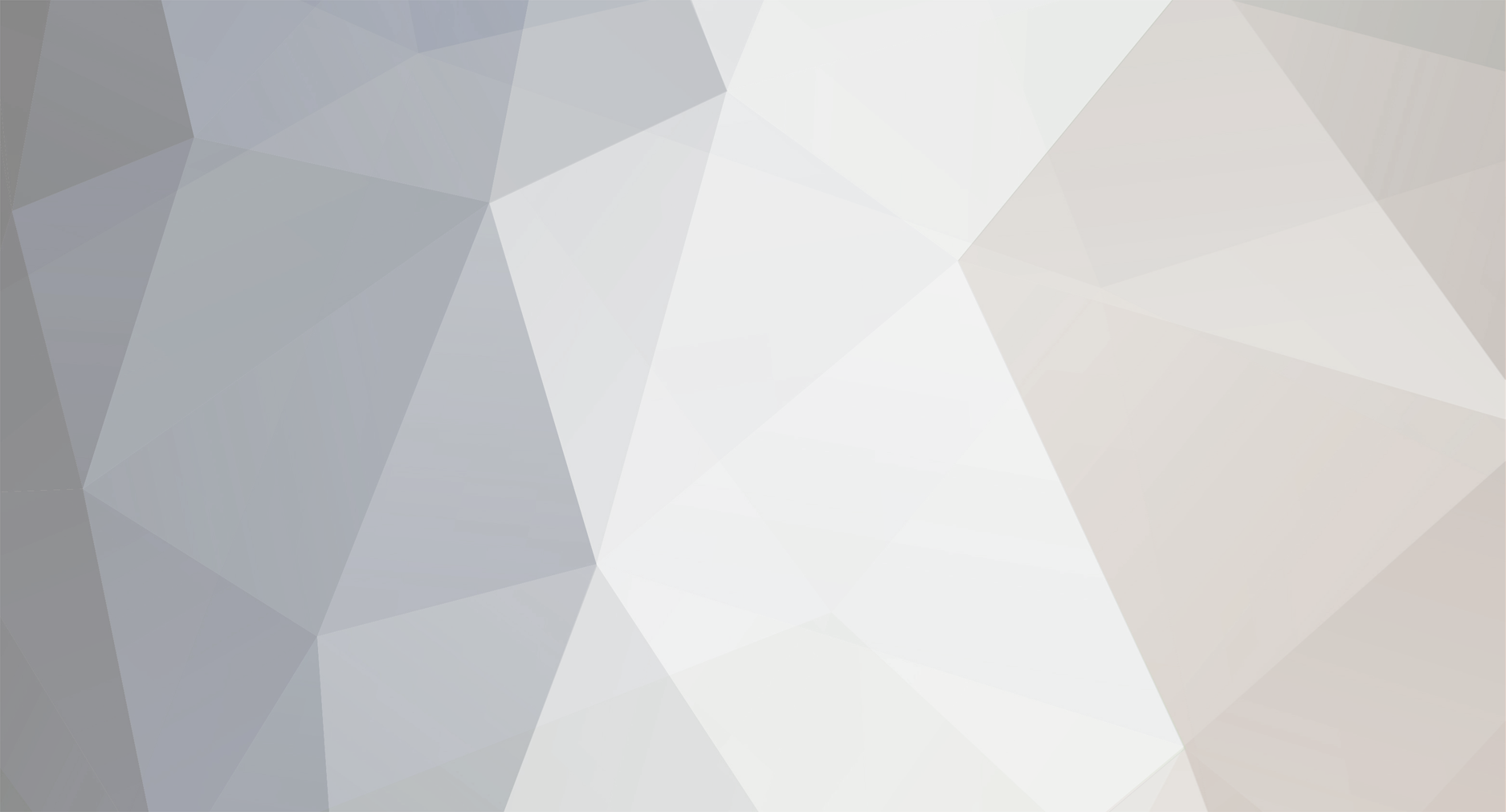
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
You can validate your JSON output on: http://www.jsonlint.com/ You can also include JSON-P, which allows you to: http://service.domain.top/bands.php?apikey=XXX&callback=jsFunction Which would return the JSON wrapped in the passed callback (P in JSON-P, or padding) jsFunction(/*JSON code here*/);
-
As so: $result = mysql_query( "SELECT due_owner, employee, pacts, dock, name, lname FROM psrinfo WHERE employee='Jane Doe' AND $trigger_date BETWEEN due_owner - INTERVAL 6 DAY AND due_owner" );
-
getimagesize()
-
$trigger_date BETWEEN due_owner - INTERVAL 6 DAY AND due_owner
-
PHP Script - Extract temperatures from another website
ignace replied to michael.davis's topic in PHP Coding Help
Changing The water temperature is ([\d]{1,2}.[\d]{1,2}) degrees to <span id="atemp">([\d]{2}) for example would get you the degrees of the second link. These should be good examples to get you started. -
PHP Script - Extract temperatures from another website
ignace replied to michael.davis's topic in PHP Coding Help
I'm not going to write all the code for you. The example I showed was to point you in the right direction. I'm glad to help if you are stuck, though. Look up web scraping, and get comfortable writing RegEx. -
PHP Script - Extract temperatures from another website
ignace replied to michael.davis's topic in PHP Coding Help
$html = file_get_contents('http://www.lrn.usace.army.mil/pao/lakeinfo/CEN.htm'); preg_match('~The water temperature is ([\d]{1,2}.[\d]{1,2}) degrees~', $html, $match); echo $match[1]; Something like that should work. -
Welcome Consider your problems a thing of the past!
-
You could make this as simple as: http://service.domain.top/bands.php Returning whatever you fancy. Of course this will be very specific to your needs, so if you are planning on letting other people use this API you will probably have to add some formats like XML and JSON, or maybe even the good old CSV http://service.domain.top/bands.xml Would also be valid, updating it overnight with a CRON.
-
Math is the foundation of a computer and thus programming. OO has laws (or principles, if you like) just like Math does. Understanding it's laws helps you to apply it correctly. Robert C. Martin identified the core principles as S.O.L.I.D., and by another author(anyone?) Tell, Don't Ask (Command-Query Separation helps you to achieve Tell, Don't Ask), Writing code is an iterative process due to changing requirements or a better understanding of the domain. For this reason you'll find a lot of people talking about re-factoring, a good book on the subject is the one by Martin Fowler or Pro PHP Refactoring. Separating your code into discrete functions helps you to make your software more flexible and thus easier to change. I could for example change the implementation of getXML() to inject the dependency instead of hard-coding cURL, and use a Factory to create the XML object.
-
Here's an example of your class in correct OO: class Scripture { const USE_CURL = TRUE; private $_userAgent = ''; private $_url = ''; public function __construct($url, $userAgent = 'Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US; rv:1.8.1.1) Gecko/20061204 Firefox/2.0.0.1') { $this->_url = $url; $this->_userAgent = $userAgent; } public function getScripture($format = '%s<br><b>%s</b>') { $xml = $this->getXML(); $script1 = $xml->channel->item->description; $script2 = $xml->channel->item->title; return sprintf($format, $script1, $script2); } private function getXML() { $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $this->_url); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); curl_setopt($ch, CURLOPT_USERAGENT, $this->_userAgent); curl_setopt($ch, CURLOPT_TIMEOUT ,3); $data = curl_exec($ch); curl_close($ch); return new SimpleXMLElement($data); } } You could further re-factor this code and remove cURL so that the technique used can vary, file_get_contents() with stream_context_get_default() instead of cURL (Factory pattern).
-
Welcome PS My browser is tired of being a fat client, so no cookies for him!
-
No need for the extra code: SELECT ... FROM Departments JOIN Department_Members USING(Dept_ID) JOIN Members USING(MemberID) You don't need the DM_ID, Dept_ID & Member_ID already make a unique value.
-
You should have a table named departments and a members table that holds a foreign key to the department or if one member can be part of many departments, create an extra table that holds a reference to both department and member. You avoid duplication and ease maintenance and querying. Removing a department is as easy as just removing the record. INNODB even allows you to set certain rules on foreign keys, when you do. And moving members to a different department is as easy as just performing an UPDATE-statement.
-
Your path should read: C:\xampp and zend\xampp\php\PEAR\Zend For it to work. Zend is not part of PEAR.
-
The query of jcbones works. If it didn't means your information is wrong. Here's a test-case for you to try out: create temporary table t1 (user CHAR(5) NOT NULL, code CHAR(5) NOT NULL PRIMARY KEY); create temporary table t2 (code CHAR(5) NOT NULL PRIMARY KEY, hits TINYINT NOT NULL); insert into t1 values ('user1', 'code1'), ('user1', 'code2'), ('user2', 'code3'), ('user3', 'code4'); insert into t2 values ('code1', 100), ('code2', 50), ('code3', 40), ('code4', 80); select sum(t2.hits) from t1 join t2 using(code) where t1.user='user1'; Running this, returns 150
-
Symfony2, Zend framework 2.0, .. Look for a framework that does not constrain your modeling in any way. To give an example in CI & CI2 you need to extend Model and call it through their Loader interface for it to work. That's a BIG no-no
-
They don't. That said, it's possible they manually check it, or if all images have the same "look" they could scan the image to make sure it's an actual skin image, or ..
-
$field will never be empty. $value however will. if(!empty($value)) { You can filter all empty values from $_GET using array_filter() You also have code duplication, I suggest you wrap that inside a function.
-
Can you post your code? money.Debit, to MySQL this means the same, to PHP Debit and debit are 2 different indices. I also noted INNNER. Add these 2 lines at the start of your script: error_reporting(E_ALL); ini_set('display_errors', 1); While you are developing.
-
Write each textarea line as a new row in database
ignace replied to perky416's topic in PHP Coding Help
Doesn't mean it won't work. It's as thorpe stated, quoting the wrong value and no error catching. if (isset($_POST['submit'])) { $lines = split("\r\n", $_POST['textarea_name']); if(!empty($lines)) { foreach($lines as $line) { mysql_query("INSERT INTO table_name(field_name) VALUES('".mysql_real_escape_string($line)."')") or trigger_error('MySQL: '.mysql_error(), E_USER_ERROR); } } } -
Scratch that. The example is quiet lame, but the intention should be clear. Remove the dependency between either objects (Model:User and Infrastructure:Email).
-
It makes your query valid as you are now building it like: SELECT * FROM fans WHERE 1 AND $field = '$value' AND $field = '$value' .. You could also just write TRUE instead of 1, it won't make any difference. Everything else should be self-explanatory.
-
//If nothing was submitted (or if the url is completely blank) if ($_GET == array ()) { //Define a variable that will be used to query the members; in this case, it would select all members $query = "SELECT * FROM fans"; } else { //If the user typed at least one thing (in the form OR the url) if (count ($_GET) == 1) { //If what they typed is only for one criteria, define a variable that creates a query for only ONE criteria to search for $query = "SELECT * FROM fans WHERE 1"; foreach ($_GET as $field => $value) { $query .= " AND $field = '$value'"; } //If the user has typed in more than one field and hits search } else { //Define a variable for a query that selects members based off each criteria $query = "SELECT * FROM fans WHERE 1"; foreach ($_GET as $field => $value) { $query .= " AND $field LIKE '%$value%'"; } } }