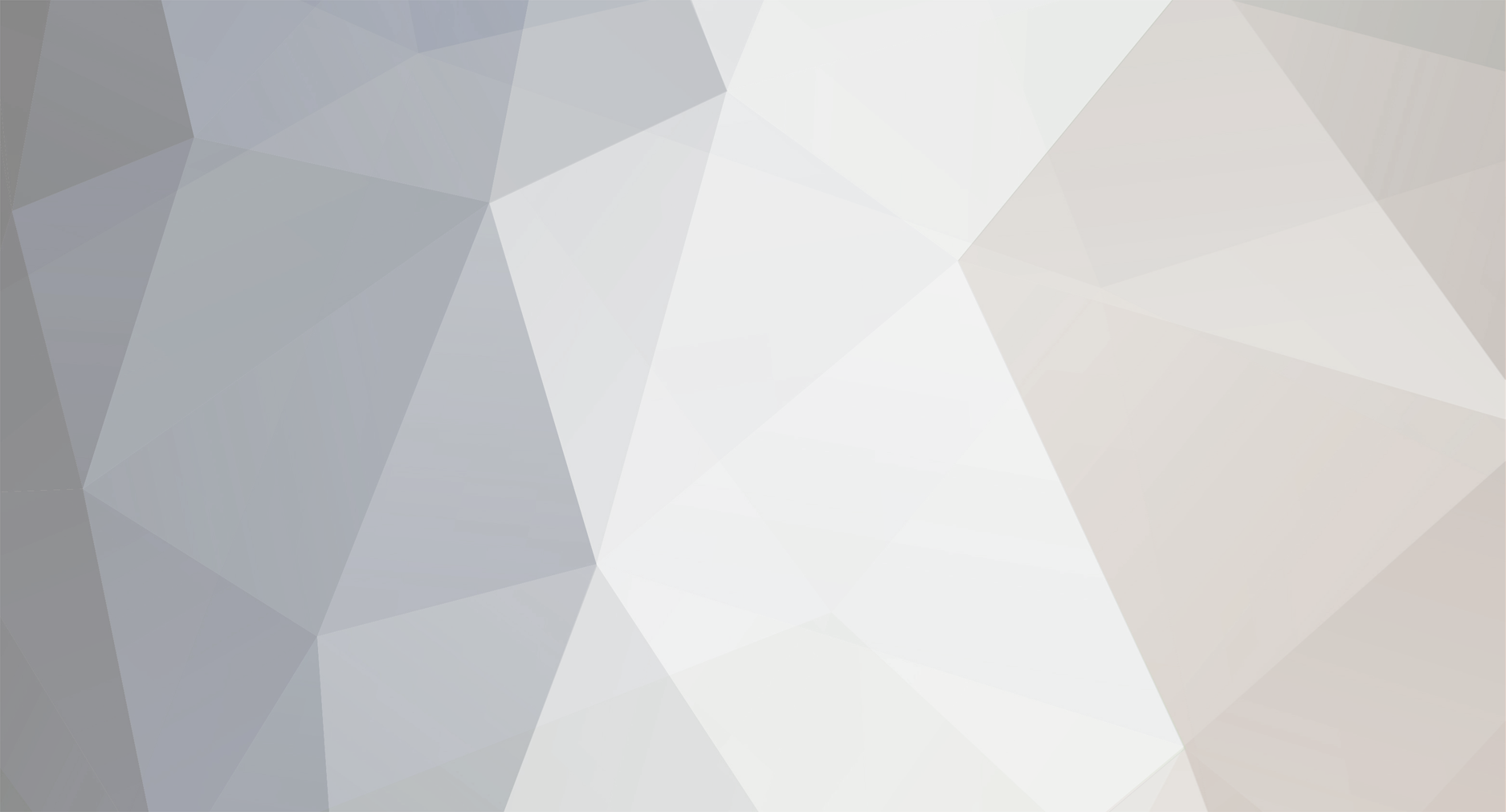
sasa
-
Posts
2,804 -
Joined
-
Last visited
-
Days Won
1
Posts posted by sasa
-
-
$sql = "SELECT * FROM " .$year_arr[$i]. "data WHERE ENGFAM = '$efn' AND EO IN (SELECT max(EO) FROM " .$year_arr[$i]. "data WHERE 1 GROUP BY LEFT(EO,6)) ORDER BY Verified DESC";
-
try
SELECT max(EO) FROM `xxx` WHERE 1 GROUP BY LEFT(EO,6)
-
try
while($r=mysql_fetch_assoc($res)) array_push($chart['chart_data'][0], $r['wght']);
-
sorry
mysql_query($sql) or die(mysql_error());
must be
$query = mysql_query($sql) or die(mysql_error());
-
try
mysql_select_db($database) or die ("Could not select database because ".mysql_error()); $sql="SELECT type.link, type.name, count(hack.id) AS co FROM type LEFT JOIN hack ON hack.typeid=type.typeid GROUP BY type.name ORDER BY type.name ASC"; mysql_query($sql) or die(mysql_error()); while ($row = mysql_fetch_object($query)) { echo "<a href=\"$row->link\" alt=\"$row->name\">$row->name $row->co</a><br />"; }
-
date('20d-m-y');
-
try
$last_admit_date = mysql_result($resultselectadmissionquery,mysql_num_rows($resultselectadmissionquery)-1,'admit_date');
-
try
<?php $result =' [img:868416aced]http://claninfectionist.com/tutorials/photoshop/FireText/untitled18.png[/img:868416aced]'; echo $result1 = preg_replace("[[/]*img:[^\]]+]", "img", $result); echo "\nor"; echo $result2 = preg_replace("[img:[^\]]+]", "img", $result); ?>
-
rename your page to buy.php (you use same php in it)
and
include('index1.php');
-
try
<?php $host="localhost"; // Host name $username="1234"; // Mysql username $password="1234"; // Mysql password $db_name="test"; // Database name $tbl_name="testing2"; // Table name // Connect to server and select databse. $connection=@mysql_connect("$host", "$username", "$password")or die("cannot connect"); mysql_select_db("$db_name")or die("cannot select DB"); $db = @mysql_select_db($db_name, $connection) or die(mysql_error()); $sql="SELECT * FROM $tbl_name ORDER BY id"; $result = mysql_query($sql,$connection) or die(mysql_error()); $y = 0; while ($row=mysql_fetch_array($result)) { $id = $row['id']; $parameter = $row['parameter']; $operator = $row['operator']; $value = $row['value']; if (isset($$parameter)){ $out = "\$test = \$$parameter $operator $value;"; eval($out); echo "Test $id "; if ($test) echo "true<br />\n"; else echo "false<br />\n"; } else echo "Variable \$$parameter not exist <br />\n"; } /* $parameter == $y; eval('?>'.$y.$operator.$value.'<?php '); */ echo "hi"; ?>
-
try
public function set_contacts($member_id) { $sql = ' SELECT * FROM network WHERE member_id = ' . (int)$member_id .' AND contact="Yes"'; $sql = $sql.' OEDER BY name'; if ($rows = $this->Db->get_rows($sql)) { $Member = new Member(); foreach($rows as $row) { $this->contact_list[] = new Member($row['network_member_id']); } } }
-
sorry
i jusr copy your code and i don't see line 5
remove line 5 ($row = mysql_fetch_array($results) or die(mysql_error())
-
try
<?php // run query $sql = "SELECT * FROM subcategories"; $results = mysql_query($sql) or die(mysql_error()); $row = mysql_fetch_array($results) or die(mysql_error()); $totalColumns = 2; $i = 1; echo "<table border='0' cellpadding='2'>"; while ($row = mysql_fetch_array($results)){ if ($i == 1) echo "<tr>"; echo "<td>"; echo "<a href='getcat.php?". $row['subcat_id'] . "'>" . $row['subcat_name'] . "</a>"; echo "</td>"; if ($i == $totalColumns) { echo "</tr>"; $i = 0; } $i++; } echo "</table>"; ?>
-
try to trim variable $item
-
or try
<?php function ms($a, $b) { if ($a['sum'] < $b['sum']) return 1; elseif ($a['sum'] > $b['sum']) return -1; else return 0; } $values = array('ed' => array('current' => 25, 'sum' => 50, 'name' => 'ed'), 'ted' => array('current' => 30, 'sum' => 70, 'name' => 'ted'), 'tom' => array('current' => 28, 'sum' => 60, 'name' => 'tom') ); usort($values, 'ms'); print_r($values); ?>
-
look function mysql_insert_id()
-
Set value of $problem1 to true at start of script
-
edit != Edit
-
try
<?php function uns1($a) { $b = explode(' AND ', $a); $o = ''; for ($i = count($b)-1; $i >= 0; $i--) { $tmp = explode(' OR ', $b[$i]); if ($o){ $q = $o; $o = array(); foreach ($tmp as $x) $o[$x] = $q; } else $o = (count($tmp) == 1) ? $tmp[0] : $tmp; } return $o; } function aa ($a, $b) { foreach ($a as $k => $v) { if (is_array($v)) { //unset($a[$k]); $a[$k]=aa($v, $b); } else { //unset($a[$v]); unset($a[$k]); if (is_array($b) && count($b) == 1) $b=$b[0]; $a[$v] = $b; } } return $a; } function uns2($a) { $j=-1; $i = strpos($a,'('); if ($i === false) return uns1($a); else { $count=1; $j = $i; while ($count > 0) { $i++; if ($a[$i] == '(') $count++; if ($a[$i] == ')') $count--; } if ($j == 0) { $a1 = trim(substr($a, 1, $i-1)); $o1 = trim(substr($a, $i+1, 4)); $a2 = trim(substr($a, $i+5)); $a1 = uns2($a1); if (!is_array($a1)) $a1=array($a1); if ($a2) $a2 = uns2($a2); //else $a2 =array(); if (!is_array($a2)) $a2=array($a2); if ($o1 == 'AND') $out = aa($a1,$a2); else { if ($o1=='OR') $out = array_merge($a1,$a2); else $out = $a1; } } elseif ($j>0) { $a1 = trim(substr($a, 0, $j-4)); $o1 = trim(substr($a, $j-4,4)); $a2 = trim(substr($a,$j)); $a1 = uns2($a1); if (!is_array($a1)) $a1=array($a1); $a2 = uns2($a2); if (!is_array($a2)) $a1=array($a2); if ($o1 == 'AND') $out = aa($a1,$a2); else { if ($o1=='OR') $out = array_merge($a1,$a2); else $out = $a1; } } } return $out; } $d = '(as OR df)'; $b='value1 AND value2 OR (value3 AND value4)'; $a = '(value1 AND value3) OR value4 AND (value5 OR value6) AND value7'; $c = 'value1 AND (value3 OR value4 AND (value5 OR value6) AND value7)'; $x = uns2($a); print_r($x); echo "\n\n"; $x = uns2($c); print_r($x); ?>
-
try
<?php $a1 = 'sasa'; $a2 ='bls'; $a3 = 'asdf'; for ($i=1; $i<4; $i++) echo ${'a'.$i},"<br />\n"; ?>
-
try
<?php $a = 'value1 AND value2 AND (value3 OR value4 AND (value5 OR value6) AND value7)'; $a = str_replace(array('(',')'), '', $a); $b = explode(' AND ', $a); $o = ''; for ($i = count($b)-1; $i >= 0; $i--) { $tmp = explode(' OR ', $b[$i]); if ($o){ $q = $o; $o = array(); foreach ($tmp as $x) $o[$x] = $q; } else $o = $tmp; } print_r($o); ?>
-
try
$check = $_POST[$value_of_parent['qName']][$key_of_child]
-
you forget ) in the end
must be
...
whyjoin VARCHAR(250))")
-
try
<?php session_start(); ?> <form method="POST"> <input type="text" name="id"> <input type="submit" value="add" name="action"> <input type="submit" value="clear cart" name="action"> </form> <?php if ($_POST['action'] == 'clear cart') unset($_SESSION['cart']); if (isset($_POST['action']) and $_POST['action'] == 'add') { $id = $_POST['id']; if ($_SESSION['cart'] and $id) { if (!in_array($id, $_SESSION['cart'])) $_SESSION['cart'][]=$id; else echo 'you already order the book!<br />'; } elseif ($id) $_SESSION['cart']= array($id); else echo 'no book <br />'; } echo 'In your card is: <br />'; if ($_SESSION['cart']) foreach ($_SESSION['cart'] as $a) echo $a,'<br />'; ?>
need help with an active user script.
in PHP Coding Help
Posted