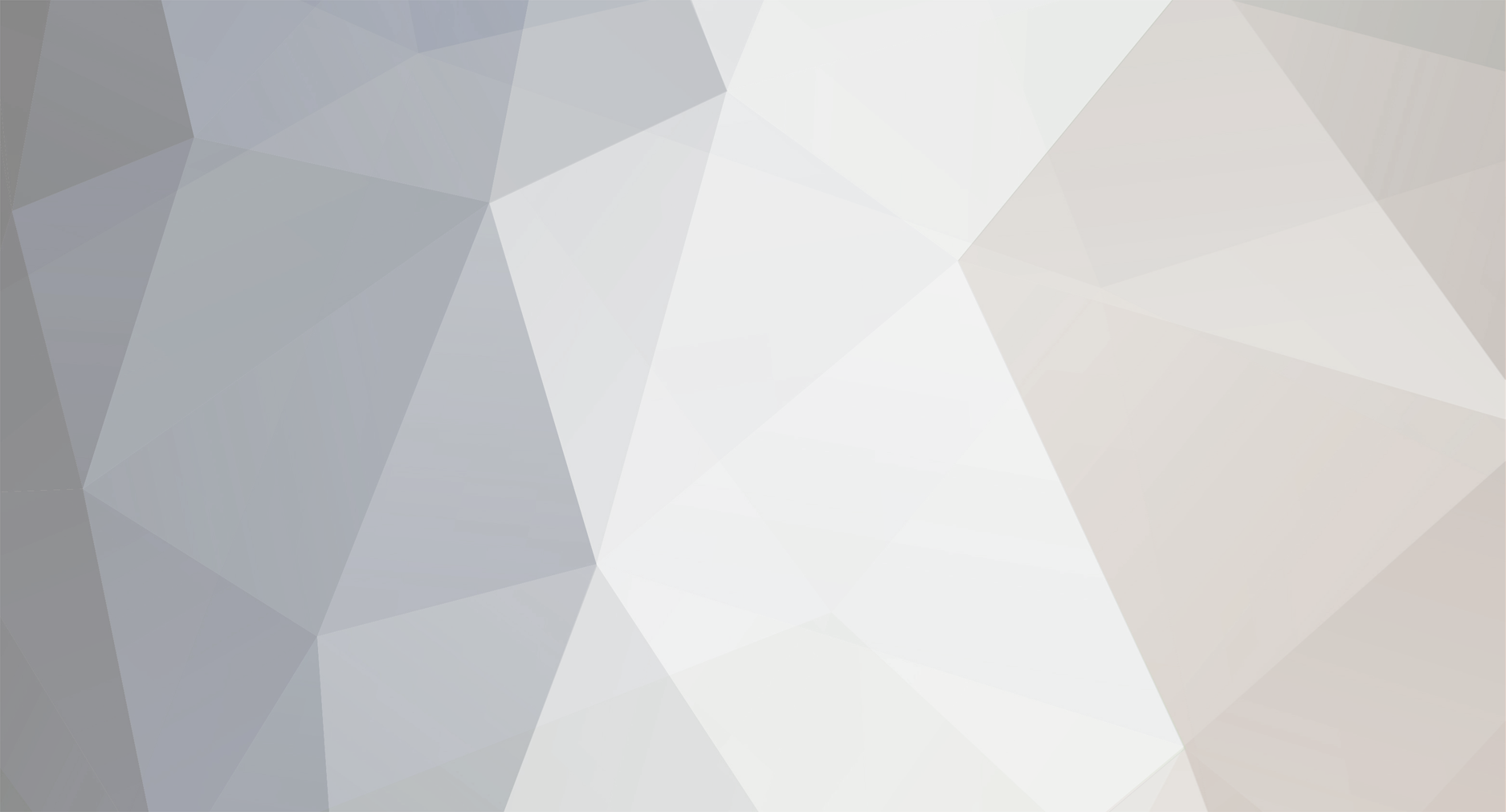
sasa
-
Posts
2,804 -
Joined
-
Last visited
-
Days Won
1
Posts posted by sasa
-
-
try
[code]<html>
<head>
<script language="JavaScript1.3" >
<!--
function row(){
var table=window.document.getElementById("table1");
var newRow = document.createElement("tr");
var newCell=newRow.appendChild(document.createElement("td"));
var input=newCell.appendChild(document.createElement("input"));
input.type="text";
input.name="frend[]";
table.appendChild(newRow);
}
-->
</script>
</head>
<body>
<?php
if ($_POST) {
//for ($x=0;$x<5;$x++) $c["cell".$x]=$_POST['cell'.$x];
print "<pre>";
print_r($_POST);
print "</pre>";
}
?>
<form method="POST" action="">
<input type="button" value="AdRow" onclick="row()">
<input type="submit"><br />
<table id="table1" border="3px" width="20%" align="left" >
<tr>
<td width="20%" align="center">FREND</td>
</tr>
</table><br />
</form>
</body>
</html>[/code] -
use two queries
1st [code]SELECT loginid,SUM(week1+week2) AS weekSum FROM submit1 WHERE username='$username' GROUP BY loginid ORDER BY weekSUM DESC
[/code] 2nd from position[code]SELECT COUNT(*)+1, SUM(week1+week2) AS weekSum FROM submit1 WHERE weekSum > $weeksum GROUP BY loginid ORDER BY weekSUM DESC[/code] -
try[code]<?php
$data = array(
array(id => 1, 'name' => 'asd'),
array(id => 2, 'name' => 'qwe'),
array(id => 3, 'name' => 'xcv'),
array(id => 4, 'name' => 'mko')
);
echo '<form method="POST">';
foreach ($data as $a) echo '<input type="checkbox" name="ch['.$a['id'].']" value="'.$a['name'].'">'.$a['name'].'<br />';
echo '<input type="submit"></form>';
if(isset($_POST['ch'])) foreach ($_POST['ch'] as $key => $value) echo "ch box no $key with value $value is selected<br />";
?>[/code] -
change <select name=\"title$n1\">"; to <select name=\"title[$formfield]\">";
in result page try[code]
foreach ($_POST['title'] as $key => $value) echo "In $key is selected $value<br />";
[/code] -
put your data in array and try[code]<?php
$data = array(
array('Paul', 'Edinburgh', '1st'),
array('Jo','Glasgow', '2nd'),
array('Nicky', 'Edinburgh', '2nd'),
array('James', 'Leeds', '2nd')
);
function grup($data, $key){
foreach ($data as $a) {
$b = $a[$key];
unset($a[$key]);
$out[$b][] = $a;
}
return $out;
}
function pr($a) {
foreach ($a as $k => $b) {
echo $k,"<br />\n";
foreach ($b as $c) {
echo "- ";
echo implode(' => ',$c);
}
echo "\n<br />\n";
}
echo "<br />\n";
}
pr(grup($data,1));
echo "<hr />\n";
pr(grup($data,2));
?>[/code] -
is it what you want
[code] .... AND date_sent < DATE_SUB( CURDATE(), INTERVAL clients.payment_terms DAY)[/code]
you try to compare date_sent with (date_sent - 20 days). It is newer thrue -
is it possible?
date_sent < date_sent - same days -
try[code]<html>
<head>
<title>Mailing Data From a File</title>
</head>
<body>
<?php
$line = file("Grades.txt");
$i = 0;
$num = 0;
$cnt = 0;
while ($line[$i] != -2) {
$first = $line[$i];
$i++;
$last = $line[$i];
$i++;
while ($line[$i] != -1) {
$num = $num + $line[$i];
$cnt++;
$i++;
}
$num = $cnt > 0 ? $num / $cnt : 0;
echo "$last, $first, $num<br />";
$i++;
$cnt = 0;
$num = 0;
}
?>
</body>
</html>[/code] -
[code]<HTML>
<HEAD>
<STYLE>
BODY
{font-family:arial;}
.error {font-weight:bold; color:#ff0000;}
</STYLE>
</HEAD>
<BODY>
<h2>Edit details:</h2>
<?php
// connect to database
include 'db.php';
// has form been submitted?
if ($_POST)
{
foreach
($_POST as $K => $V)
{$v = trim($V) ;$$K = $v;}
// Build UPDATE query
$update = "UPDATE details SET user='$fn', model='$ln', engine='$en',
bhp='$bn' WHERE Id=$id";
// execute query and check for success
if (!mysqli_query($link, $update))
{
$msg = "Error updating data";
}
else
{
$msg = "Record Successfully updated:";
//write table row confirming data
$table_row =
"<TR>
<TD>$fn</TD>
<TD>$ln</TD>
<TD>$en</TD>
<TD>$bn</TD>
</TR>";
}
//if not posted, check that an id has been passed via the url
}
else
{
if (!isset($_GET['id']))
{
$msg = "No user selected";
}
else
{
$id = $_GET['id'];
// Build and execute the query
$select = "SELECT user, model,
engine, bhp FROM details WHERE id=$id";
$result = mysqli_query($link, $select);
// check that the record exits
if (mysqli_num_rows($result)<1)
{
$msg = "No user with that ID found!";
}
else
{
// set vars for the form code
$form_start = "<FORM METHOD=\"post\" ACTION= \"" . $_SERVER['PHP_SELF'] . "\">";
$form_end =
"<TR>
<TD COLSPAN=\"2\">INPUT TYPE=\"submit\"
VALUE=\"submit changes\" /></TD>
<TD COLSPAN=\"2\"><INPUT TYPE=\"reset\"
VALUE=\"Cancel\" /></TD>
</TR></form>";
// assign the results to an array
while ($row = mysqli_fetch_array($result))
{
$fn = $row['user'];
$ln = $row['model'];
$en = $row['engine'];
$bn = $row['bhp'];
//write table row with form fields
$table_row .=
"<TR>
<TD><INPUT TYPE=\"text\" NAME=\"fn\"
VALUE=$fn SIZE=\"127\"/></TD>
<TD><INPUT TYPE=\"text\" NAME=\"ln\"
VALUE=$ln SIZE=\"127\"/></TD>
<TD><INPUT TYPE=\"text\" NAME=\"en\"
VALUE=$en SIZE=\"127\"/></TD>
<TD><INPUT TYPE=\"text\" NAME=\"bn\"
VALUE=$bn SIZE=\"4\"/></TD>
</TR>";
}
// end if record exists' if
}
// end if ID given in url' if
}
// end if form posted' if
}
// close connection
mysqli_close($link);
// print error/success message
echo (IsSet($msg)) ? "<divclass=\"error\">$msg</div>" : "";
?>
<!-- set up the table-->
<TABLE BORDER="1" CELLPADDING="5">
<!-- Show start-of-frm code if form needed -->
<? echo (IsSet($form_start)) ? $form_start : "";
?>
<INPUT TYPE="HIDDEN" NAME="id" VALUE="<? echo $id ?>" />
<tr>
<th>User Name</th>
<th>Model</th>
<th>Engine</th>
<th>BHP</th>
</tr>
<!-- show appropriate table row code (none set if there errors) -->
<? echo (IsSet($table_row)) ? $table_row : "";
?>
<!-- show end-of-form code if we are displaying the form -->
<? echo (IsSet($form_end)) ? $form_end : "" ; ?>
</TABLE>
<br/> <a href="mod.php">Back to users list</a>
</BODY>
</HTML>
?>[/code] -
you can create short message before you know whole message
move '$short = join(' ', array_slice (explode(' ', $posmes['message']), 0, 4));' in while loop (before echo) -
[code]<?php
$myArray[0][0] = "Autobots";
$myArray[0][1] = "Bumblebee";
$myArray[0][2] = "VW Bug";
$myArray[0][3] = "Yellow";
$myArray[0][4] = "Smog Check";
$myArray[1][0] = "Autobots";
$myArray[1][1] = "Jazz";
$myArray[1][2] = "Porsche";
$myArray[1][3] = "White";
$myArray[1][4] = "Steering Realignment";
$myArray[2][0] = "Autobots";
$myArray[2][1] = "Optimus Prime";
$myArray[2][2] = "Diesel";
$myArray[2][3] = "Red and Blue";
$myArray[2][4] = "Catalytic Converter";
$myArray[3][0] = "Decepticons";
$myArray[3][1] = "Starscream";
$myArray[3][2] = "Flying Machine";
$myArray[3][3] = "Red";
$myArray[3][4] = "Girly Voice";
$myArray[3][0] = "Decepticons";
$myArray[3][1] = "Megatron";
$myArray[3][2] = "Pea Shooter";
$myArray[3][3] = "Purple and Black";
$myArray[3][4] = "Everything";
function gr($group_key,$data_key,$aray) { //reorganize array
if (!is_array($data_key)) $data_key = array($data_key);
foreach ($aray as $a) {
$o1 = array();
foreach ($data_key as $e) $o1[] = $a[$e];
$b[$a[$group_key]][] = $o1;
}
return $b;
}
function out($a) { //output array
foreach ($a as $b => $c) {
$out .= $b."\n".'<table border="3">'."\n";
foreach ($c as $d) {
$out .= '<tr>'."\n";
foreach ($d as $e) $out .= "\t<td width=\"150\">$e</td>\n";
$out .= '</tr>'."\n";
}
$out .= '</table>'."\n<br />\n\n";
}
return $out;
}
$a = array(1,3,4,2);
$b = gr(0,$a,$myArray);
//print_r($b);
$c = out($b);
echo $c;
?>[/code] -
try[code]<?php
$myArray[0][0] = "Autobots";
$myArray[0][1] = "Bumblebee";
$myArray[0][2] = "VW Bug";
$myArray[0][3] = "Yellow";
$myArray[0][4] = "Smog Check";
$myArray[1][0] = "Autobots";
$myArray[1][1] = "Jazz";
$myArray[1][2] = "Porsche";
$myArray[1][3] = "White";
$myArray[1][4] = "Steering Realignment";
$myArray[2][0] = "Autobots";
$myArray[2][1] = "Optimus Prime";
$myArray[2][2] = "Diesel";
$myArray[2][3] = "Red and Blue";
$myArray[2][4] = "Catalytic Converter";
$myArray[3][0] = "Decepticons";
$myArray[3][1] = "Starscream";
$myArray[3][2] = "Flying Machine";
$myArray[3][3] = "Red";
$myArray[3][4] = "Girly Voice";
$myArray[3][0] = "Decepticons";
$myArray[3][1] = "Megatron";
$myArray[3][2] = "Pea Shooter";
$myArray[3][3] = "Purple and Black";
$myArray[3][4] = "Everything";
function gr($group_key,$data_key,$aray) {
foreach ($aray as $a) $b[$a[$group_key]] .= " => $a[$data_key]<br />";
foreach ($b as $c => $d) $output .= "$c<br />------------<br />$d<br />";
return $output;
}
print gr(0,1,$myArray);
?>[/code] -
try[code]<?
/* Account activation script */
// Get database connection
include ( './db.php' );
// Create variables from URL.
// first check if it's already been activated
$sql = mysql_query ( "SELECT COUNT(*) AS total FROM users WHERE userid = '" . mysql_real_escape_string ( $_REQUEST['id'] ) . "' AND password = '" . mysql_real_escape_string ( $_REQUEST['code'] ) . "' AND activated = 1" ) or die ( 'Query Error: ' . mysql_error );
$found = mysql_fetch_assoc ( $sql );
if ( $found['total'] == 0 )
{
$sql = mysql_query ( "UPDATE users SET activated = 1 WHERE userid = '" . mysql_real_escape_string ( $_REQUEST['id'] ) . "' AND password = '" . mysql_real_escape_string ( $_REQUEST['code'] ) . "'" ) or die ( 'Query Error: ' . mysql_error );
if ( mysql_affected_rows ( $sql ) == 0 )
{
echo "<strong><font color='red'>Your account could not be activated, no user found by that id or password!</font></strong>";
die(mysql_error());
}
else
{
echo "<strong>Your account has been activated!</strong> You may login below!<br />";
include ( './login.php' );
}
}
else
{
echo "<strong>You have already activated your account!</strong> You may login below!<br />";
include ( './login.php' );
}
?>[/code] -
OK
your output new has index date_f (mean date formated) -
try[code]$query = "SELECT itemid, title, picture, DATE_FORMAT(date, '%d %M %y') AS date_f FROM news ORDER BY 'date' desc";[/code]
-
use htmlentities()
-
try[code]<?php print str_replace('index.php','http://hanmia8.freehostia.com/index.php', file_get_contents('http://hanmia8.freehostia.com/index.php?option=com_content&task=view&id=5&Itemid=6')); ?>[/code]
-
change $pageprev = $page++; to $pageprev = $page-1;
and $pagenext = $page--; to $pagenext = $page+1; -
mysql_fetch_array($query) extract one row from $query in array
this array have two elements it looks like array(0 => 1, 'ID' => 1)
your output is OK -
try [code]<?php
$a=8;
$b=7;
$c = ' echo "$a + $b = ";
echo $c = $a + $b . "<br />\n";
echo $c + $a . "<br />\n";
for($i = 1; $i < 11; $i++) echo $i . "<br />\n";
';
eval($c);
echo 'New value of $c is: ' . $c .'!!!!'; //$c = $a + $b . "\n";
?>[/code] -
expresion some_field LIKE '%%' is always true
-
line[code]$email2=mysql_result(result,$i,"customers_email_address");[/code]must be[code]$email2=mysql_result($result,$i,"customers_email_address");[/code]
-
is this help [code]<?php
function change_date_format($date) {
$date = explode(' > ',$date);
return implode('-',array_reverse(explode('-',$date[0]))).' > '.$date[1];
}
$date = date("Y-m-d > H:i:s");
echo change_date_format($date)."<br />\n";
?>[/code] -
you dont end your functions.
Move 1st } (just after long line) in php part
and in the end add one more }
Ok 2 questions [SOLVED]
in PHP Coding Help
Posted
$h = date('H');
if ($h < 8 or $h > 20) exit('Not workin');
?>[/code]
2nd[code]$rand_strength = rand(1,20)/1000;[/code]