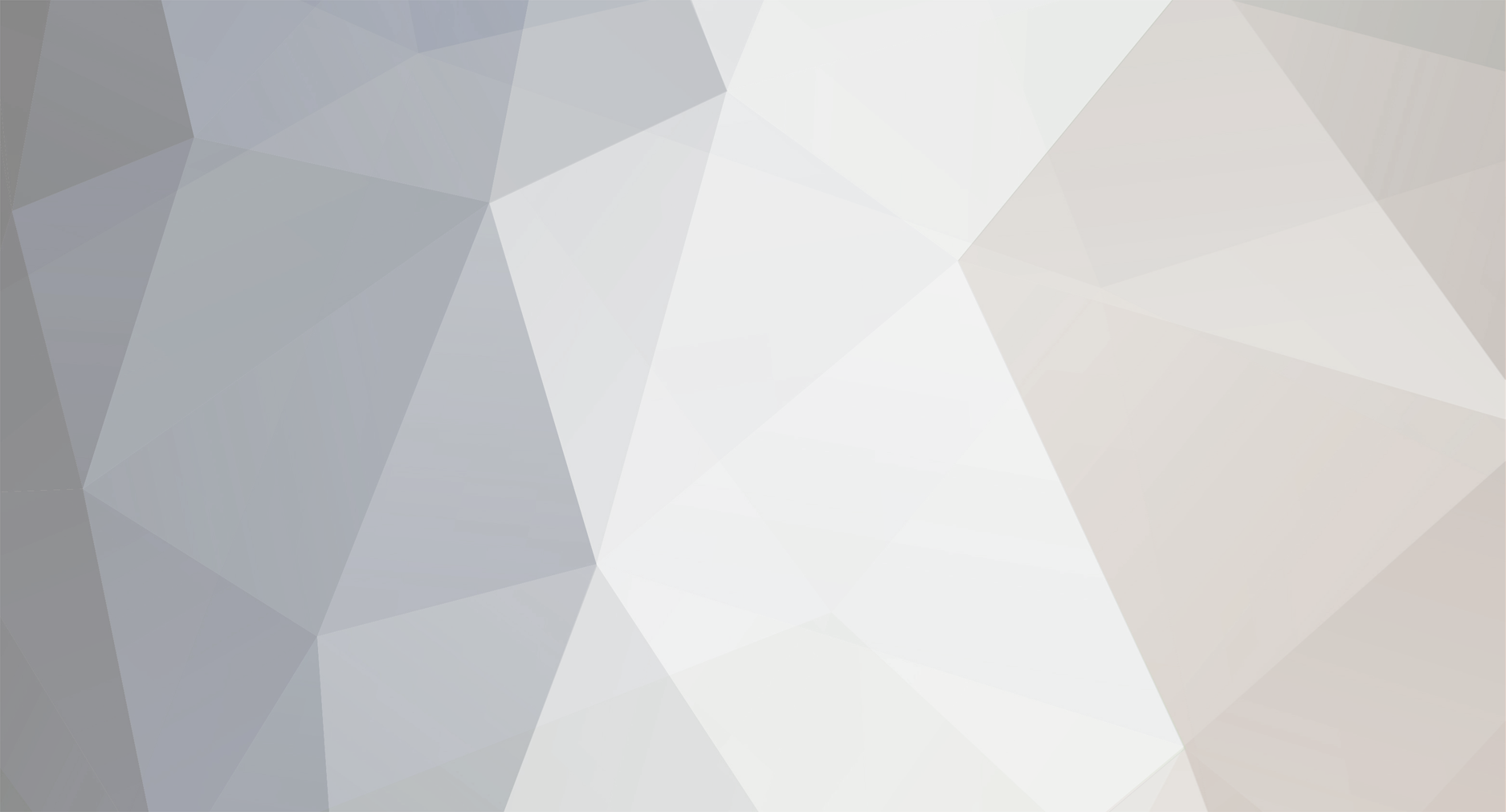
sasa
-
Posts
2,804 -
Joined
-
Last visited
-
Days Won
1
Posts posted by sasa
-
-
try[code]
<?php
$usernames = array("chris", "bob", "pete");
$passwords = array("piss", "knob", "feet");
$username = $_POST['username'];
$password = $_POST['password'];
$log_in = 'not logged in';
for($x = 0; $x < count($usernames); $x++) {
if(@$username == $usernames[$x] && $password == $passwords[$x]):
$log_in = 'loged in';//echo("logged in");
//else:
//echo("not logged in");
endif;
}
echo $log_in;
?>
[/code] -
try[code]
function userExists($a){
global $conn;
$q = "select username from users where username = '$a'";
$result = mysql_query($q,$conn);
return (mysql_numrows($result) > 0);
}
[/code] -
try[code]
<?php
mysql_connect("", "", "");
mysql_select_db("");
$seed = (isset($_GET['seed']) and $_GET['seed'] >= 1) ? intval($_GET['seed']) : rand(1,10000);
$page = (isset($_GET['page']) and $_GET['page'] >= 1) ? intval($_GET['page']) : 1;
$per_page = 3;
$total = mysql_result(mysql_query('select count(*) from notes'),0,0);
$t_pages = ceil($total/$per_page);
$page = $page > $t_pages ? $t_pages : $page;
$start = ($page - 1) * $per_page;
$result = mysql_query("
SELECT
*
FROM
notes
ORDER BY RAND($seed)
LIMIT $start, $per_page
") or die(mysql_error());
while ($row = mysql_fetch_array($result)) {
$text = markerconvert($row[content]);
$result_array[] = "<div id=\"".$row[ID]."\" class=\"notecontent\" style=\"border: 1px ".$row[bclr]." solid; background-color:".$row[bgclr]."; font-size:10; width:160px; height:100px;\">".$text."</div>";
}
mysql_free_result($result);
foreach ($result_array as $category_link) {
if ($counter == $number_of_categories_in_row) {
$counter = 1;
$result_final .= "\n</tr>\n<tr>\n";
} else $counter++;
$result_final .= "\t<td>" . $category_link . "</td>\n";
}
if ($counter) {
if ($number_of_categories_in_row - $counter)
$result_final .= "\t<td colspan='" .
($number_of_categories_in_row - $counter) . "'> </td>\n";
}
index_footer($result_final);
if ($page > 1) echo '<a href="?page='.($page-1).'&seed='.$seed.'">PREV </a>'; else echo 'PREV ';
for ($i = 1; $i<=$t_pages;$i++) if ($i != $page) echo '<a href="?page='.$i.'&seed='.$seed.'">| '.$i.' </a>';
else echo "| $i ";
if ($page < $t_pages) echo '<a href="?page='.($page+1).'&seed='.$seed.'">| NEXT</a>';else echo '| NEXT';
page_footer();
?>
[/code] -
change line [code]$content = $_REQUEST['view'];[/code]to[code]$viev = $_REQUEST['view'];[/code]
-
it work for me[code]
<?php
$a=array ( 0 => 'hey.txt', 1 => 'logo.gif', 'Wow' => array ( 0 => 'cool.gif', 1 => 'Saved Game', 2 => 'OK!' ,), );
$b= array ( 0 => 'logo.gif', 'Wow' => array ( 0 => 'cool.gif', 1 => 'Saved Game' ), );
// a -b
function rem ($a, $b) {
foreach ($a as $k => $v) {
if (is_array($v)) {
if (key_exists($k, $b)) {
$c= rem($v, $b[$k]);
if (count($c)) $out[$k] = $c;
}
} elseif (!in_array($v, $b)) $out[$k] = $v; //or $out[] = $v
}
return $out;
}
$c = rem($a,$b);
print_r($c);
?>[/code] -
1. in join_warp.php you have input name="family_name" and you look for $_POST['last_name'] in register.php
2. you don't set up $word = $_POST['word'] in register.php
3. you must chck if $word is OK this is first part of join_warp.php (16 lines)
-
try[code]
<?php
$result=mysql_query($sql);
while ($a=mysql_fetch_array($result)) $rows[]=$a;
$keys= array_rand($rows,count($rows));
foreach ($keys as $key){
$row=$rows[$key];
// you code
}
?>
[/code] -
function addNewTable($username){
$q = "CREATE TABLE IF NOT EXISTS ".$username."('to' varchar(30) not null, 'from' varchar(30) not null, 'amount' int not null, 'date' date not null primary key, 'for' varchar(250))";
return mysql_query($q, $this->connection);
} -
try[code]
<?php
function money($cash) {
$knuts = $cash % 29;
if ($knuts) $knuts = "$knuts Knut" . (($knuts == 1) ? '':'s'); else $knuts = '';
$cash = intval($cash / 29);
$sickles = $cash % 17;
if ($sickles) $sickles = "$sickles Sickle" . (($sickles == 1) ? '':'s').', '; else $sickles = '';
$galon = intval($cash / 17);
if ($galon) $galon = "$galon Galon" . (($galon == 1) ? '':'s').', '; else $galon = '';
return $galon.$sickles.$knuts;
}
echo money(123456789);
?>
[/code] -
try[code]
<form name="form1" method="GET" action="categories.php">
<input type="hidden" name="c" value="0">
<?php
$query = 'select pd_id, pd_name FROM tbl_product group by pd_name desc';
$result = @mysql_query($query) or die('<p>Sorry, no data to edit...</p>');
?>
<select name="p" id="select4" onchange="submit()">
<option value="0">---</option>
<?php
while($row=mysql_fetch_assoc($result)) {
echo "\t<option value=\"" . $row['pd_id'] . '">' . $row['pd_name'] . ' </option>';
}
?>
<input type="submit"></form>
[/code] -
or maybe[code]
<?php
function dec2frac ($n) {
list ($int, $dec) = explode ('.', "$n");
if (!$dec) return "$int";
$p = strlen ($dec);
$den = pow (10, $p);
$a = $den; //Euclidean algorithm
$b = $dec;
$c = $a % $b;
while ($c){ $a = $b; $b = $c; $c = $a % $b; }
//$comval = $b;
$dec /= $b;
$den /= $b;
if (!$int) $int = ''; else $int = "$int "; // no leading 0
return "$int$dec/$den";
}
$a = array (0.5,'5.0', 1.75, 2.625, 4.997); // test data (ie that data for which the program works)
foreach ($a as $n) echo "$n -> ". dec2frac ($n) . "<br />\n";
?>
[/code] -
[quote author=Barand link=topic=103913.msg414307#msg414307 date=1155343993]
@sasa,
I'm playing devil's advocate again. Try
echo flo_to_fract(7.625);
[/quote]i change code[code]
<?php
function flo_to_fract($a){
if ($a==0) return '0';
$a1=floor($a);
if ($a1==0) $a1=''; else $a1 ="$a1 ";
$b=$a-$a1;
$c=strlen("$b")-2;
$c=round("1E+$c");
$b=round($b * $c);
if ($b==0) return $a1;
while ((($b%2)==0) and (($c%2)==0)) {
$b=$b/2;
$c=$c/2;
}
while ((($b%5)==0) and (($c%5)==0)) {
$b=$b/5;
$c=$c/5;
}
$a1.="$b/$c";
return $a1;
}
echo flo_to_fract(7.625);
?>
[/code]thanks for debug Barand -
try[code]
<?php
function flo_to_fract($a){
if ($a==0) return '0';
$a1=floor($a);
if ($a1==0) $a1=''; else $a1 ="$a1 ";
$b=$a-$a1;
$c=strlen("$b")-2;
$c=round("1E+$c");
$b=round($b * $c);
if ($b==0) return $a1;
while (($b%2)==0) {
$b=$b/2;
$c=$c/2;
}
while (($b%5)==0) {
$b=$b/5;
$c=$c/5;
}
$a1.="$b/$c";
return $a1;
}
echo flo_to_fract(7.125);
?>
[/code] -
[quote author=harville85 link=topic=103925.msg414219#msg414219 date=1155336416]
Thanks, I'll see if I can make this work. While I do that, here's an example of what I have now that works, but it doesn't list the actual category in my email.
$text_body = $_POST['contact_name']. "<br>";
$text_body .= $_POST['city']. "<br>";
$text_body .= $_POST['state']. "<br>";
$text_body .= $_POST['zip_code']. "<br>";
$text_body .= $_POST['phone']. "<br>";
$text_body .= $_POST['fax']. "<br>";
$text_body .= $_POST['email']. "<br>";
[/quote]change in[code]
$text_body = "Name: ".$_POST['contact_name']. "<br>";
$text_body .= "City: ".$_POST['city']. "<br>";
etc.
[/code] -
$body="
Name: $name\n
Address: $address\n
...
" -
you need pagination
one row per page
google for same tutorial -
try for debug[code]
if ($parentId == 0) {
$categories[$id] = array('name' => $name, 'children' => array());
} else {
if(isset($categories[$parentID]['name'])) {
echo "Data error for line $id name: $name. ParentID $parentID is NOT main cat.";exit;
}
$categories[$parentId]['children'][] = array('id' => $id, 'name' => $name);
}
[/code] -
mysql_result($sql10,$x,'store_id') return from resorce $sql10 row: $x and field: 'store_id'
when $x=0 it returns 1st row etc. -
line 4 must be $id = $_GET['id']; not ==
-
you require("add.php"); in add.php
-
use mysql_data_seek($myQry,0); before while loop
-
i make mistake
i echo field 'store' not 'store_id' in line [code]for ($x=0;$x<5;$x++) echo "store #: ".mysql_result($sql10,$x,'store');[/code]
corr to [code]for ($x=0;$x<5;$x++) echo "store #: ".mysql_result($sql10,$x,'store_id');[/code]
$x is number and you can do any statmens
-
try[code]
$storesql = "SELECT store_id
FROM store
WHERE store_open_closed = 'o'
ORDER BY store_id";
$sql10 = run_sql($storesql) or die(mysql_error());
$num_of_stores=mysql_num_rows($sql10);
for ($x=0;$x<5;$x++) echo "store #: ".mysql_result($sql10,$x,'store');[/code]
-
try[code]
<?php
include"connect.php";
$sql="SELECT * FROM southport_categories";
$query=mysql_query($sql);
while ($row=mysql_fetch_array($query)) $output[$row['Category']][]=$row['subcat'];
foreach ($output as $cat => $subcats){
echo "<p>$cat";
foreach ($subcats as $subcat) echo " -> $subcat";
echo "</p>";
}
?>
[/code]
Splitting text into teaser and main part
in PHP Coding Help
Posted
<?php
$p = array('.', '!', '?');
$max = 350;
$x = min($max, strlen($content));
while (!in_array($content[--$x], $p) and $x >= 0);
if ($x >= 0) $out=substr($content, 0, $x+1);
else $out = 'Err'; // error
echo $out;
?>
[/code]