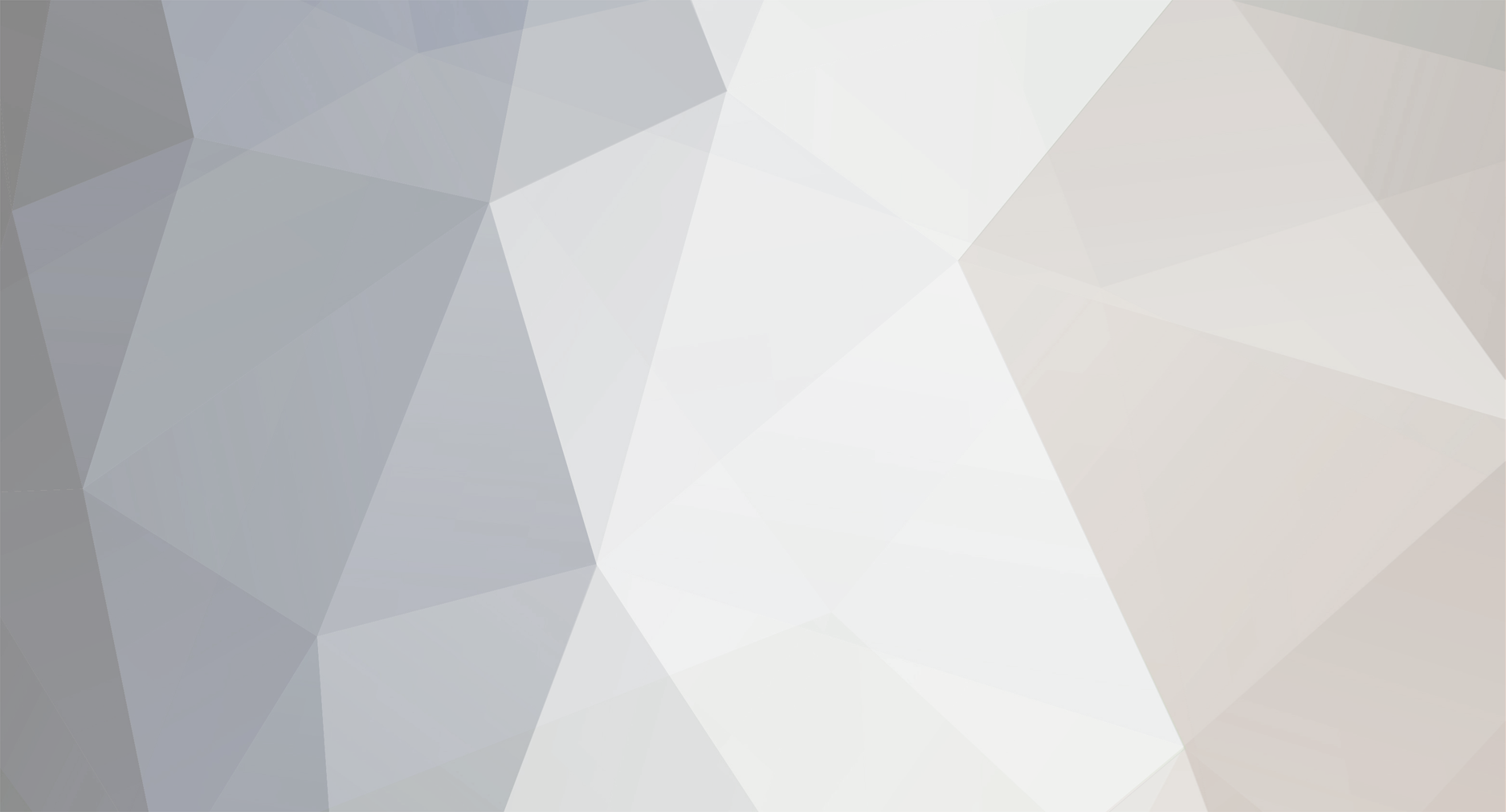
sasa
-
Posts
2,804 -
Joined
-
Last visited
-
Days Won
1
Posts posted by sasa
-
-
SELECT *, COOUNT(*) AS num FROM (SELECT * FROM `hits`GROUP BY ip) a GROUP BY cullumn_name_with_numbers_784_and_so_on
-
yes if start is number
-
<?php $titles_list = array(1,2,3); $prices_list = array(11,22,33); $services_list = array(111,222,333); reset($titles_list); reset($prices_list); reset($services_list); $title = current($titles_list); $price = current($prices_list); $service_id = current($services_list); while ($title and $price and $service_id){ mysql_query("INSERT INTO product_invoices (name, invoice_id, price, quantity, date_time, service_id) VALUES('$title', '$invoice_id', '$price', '$today_slashes', '$service_id')") or die(mysql_error()); $title = next($titles_list); $price = next($prices_list); $service_id = next($services_list); } ?>
-
$page_name = $_SERVER["REQUEST_URI"]; $page_name = preg_replace('/&?limit=\d+/','',$page_name);
-
try
<?php $hierarchy=array(); $hierarchy[]=array('id' => 1, 'parent_id' => 0, 'name' => 'root1'); $hierarchy[] = array('id' => 2, 'parent_id' => 0, 'name' => 'root2'); $hierarchy[] = array('id' => 3, 'parent_id' => 1, 'name' => 'root1-1'); $hierarchy[] = array('id' => 4, 'parent_id' => 1, 'name' => 'root1-2'); $hierarchy[] = array('id' => 5, 'parent_id' => 3, 'name' => 'root1-1-1'); $hierarchy[] = array('id' => 6, 'parent_id' => 2, 'name' => 'root2-1'); function find_pat($a, $n, $key='name' ){ $out = array(); foreach ($a as $r){ if ($r[$key] == $n){ $out = find_pat($a, $r['parent_id'],'id'); $out[]=$r; } } return $out; } $a = find_pat($hierarchy, 'root1-1-1');] print_r($a); ?>
-
SELECT group_concat(if((select count(b.id) from table b where b.id <= a.id and a.grup = b.grup)=1, a.name, '') separator '') name_1, group_concat(if((select count(b.id) from table b where b.id <= a.id and a.grup = b.grup)=2, a.name, '') separator '') name_2, group_concat(if((select count(b.id) from table b where b.id <= a.id and a.grup = b.grup)=3, a.name, '') separator '') name_3, group_concat(if((select count(b.id) from table b where b.id <= a.id and a.grup = b.grup)=4, a.name, '') separator '') name_4, group_concat(if((select count(b.id) from table b where b.id <= a.id and a.grup = b.grup)=5, a.name, '') separator '') name_5 FROM table a group by a.grup ORDER BY a.id
-
you can use GROUP and GROUP_CONCAT in your query
-
$result = mysql_query("select * from staff'"); $i=0: echo "<table border='0' cellpadding='10' cellspacing='0'>"; //echo "<tr>"; while($row = mysql_fetch_array($result)) { if($i == 0) echo '<tr>'; $i++; echo "<td><img src=../upload/".$row['Pic']." border='0' width='140' height='105' /></td>"; echo "<td>".$row['Name']."</br><b>".$row['Role']."</b></td>"; if($i==2){echo '</tr>'; $i=0;} } if($i>0)echo "<td> </td></tr>"; echo "</table>";
-
add names of fields that you want to select after SELECT command
-
you look for $_SERVER[REQUEST_URI]
-
use different variables for your results
-
change your function to
function somefunction($vaiable) { $gers = mysql_query(sprintf("SELECT emp_id, lastname FROM info WHERE active='1' AND oid='$oid' AND pidnumber='4'")) or die(mysql_error()); $out =''; while ($gerow = mysql_fetch_array($gers)) { $out .= "ci.attorney='$lastnamey' OR "; } return $out; }[>/code]
-
try
if(isset($_GET['lang'])) { switch($_GET['lang']) { case 'zh-hans': include (TEMPLATEPATH . '/branches-cn.php'); //branches pages contains the translated images break; case 'zh-hant': include (TEMPLATEPATH . '/branches-tcn.php'); break; default: include (TEMPLATEPATH . '/branches.php'); //This is my english page break; } } else include (TEMPLATEPATH . '/branches.php');
-
or
<?php $test ='"STEAM_0:0:18130940" { "admin" "(Console)" "unban" "1274151724" "time" "1273546924" "name" "-=SAS=- Death Master511" "reason" "Gcombat on spawn/Server Crash Attempts 1 week appeal at halania.com" } "STEAM_0:1:6428933" { "time" 1273619777 "unban" 1273706177 "admin" "TornadoChas3r(STEAM_0:0:19768725)" "name" ".:T.¥:. TRÅÑŒ (CRYSTAL)" "reason" "RDM/ 1 day Ban \"You had Enough Warnings\" / :" } '; $test = preg_replace('/"(STEAM_[^"]+)"/', '[$1]', $test); $test = preg_replace('/\{|}/', '', $test); $test = preg_replace('/"([^"]+)"\t+([^ ])/S', '$1=$2', $test); $test = parse_ini_string($test, 1); print_r($test); ?>
-
try
<?php $string_parts = 4; $max = 10000; $arr = array(); $i = 0; $start = memory_get_usage(); $ar = array_pad(array(), 4, "A"); for(;$i<$max;$i++){ //$arr[] = array_pad(array(), 4, "A"); $arr[] = $ar; } $end = memory_get_usage(); printf("%d iterations, %d bytes in array\n", $max, $end-$start); $arr2 = array(); $i = 0; $ar = array("A", "A", "A", "A"); $start2 = memory_get_usage(); for(;$i<$max;$i++){ $arr2[] = $ar; //$arr2[] = array('A', 'A', 'A', 'A'); //$arr2[] = array_fill(0,4,'A'); } $end2 = memory_get_usage(); printf("%d iterations, %d bytes in array (%.2f%%)\n", $max, $end2-$start2, ($end2-$start2)/($end-$start)*100); ?> f/code]
-
try
<?php $time = 200; $each_block = 60; $total_time = 86400; $no_blocks = $total_time / $each_block; $curr_bl = ceil($time / $each_block); $to_end_of_block = $curr_bl * $each_block - $time; $left = $no_blocks - $curr_bl; echo "You are on block num $curr_bl, to the end of this block left $to_end_of_block sec and to the end of the day left $left blocks." ?>
-
try
for($i = 0; $i < $num_course; $i++) // num_course is the number of arrays (course0,course1) that are recieved { $test = "course".$i; for($x = 0; $x < sizeof($$test); $x++) //ad one more $ { echo($$test[$x]); //same here } }
-
try
<?php $downloadscount = mysql_query("SELECT SUM(timesdownloaded) FROM sheets WHERE timesdownloaded>='0'"); //$totaldownloads = mysql_num_rows($downloadscount); $totaldownloads = mysql_result($downloadscount, 0, 0); ?>
-
<?php $test = '000000001718989'; $test = ltrim($test, '0'); echo $test; ?>
-
use mysql_data_seek($result, 0); before inside loop to move index to start of $result
-
try
<?php $test = '[quote="AdRock"]just testing the error[/quote] Another test'; $test = html_entity_decode($test, ENT_QUOTES); preg_match_all('/\[\s*QUOTE="([^"]*)"/s', $test, $out); print_r($out[1]); ?>
-
try
<?php $food = ""; $drink = "beer"; $price = "five dollars"; $test = array($food, $drink, $price); foreach ($test as $key => $v ) if (!$v) unset ($test[$key]); $test = implode(', ', $test); echo $test; ?>
-
WHERE CFBP.Game_ID = CFBG.ID AND ((CFBP.Pick =1 AND CFBP.Pick = CFBG.Away) OR (CFBP.Pick =2 AND CFBP.Pick = CFBG.Home)) AND CFBP.User_ID = $user;
-
try to change $row['promo.title'] to $row['title']
sort array by group
in PHP Coding Help
Posted
try to change
to
not tested