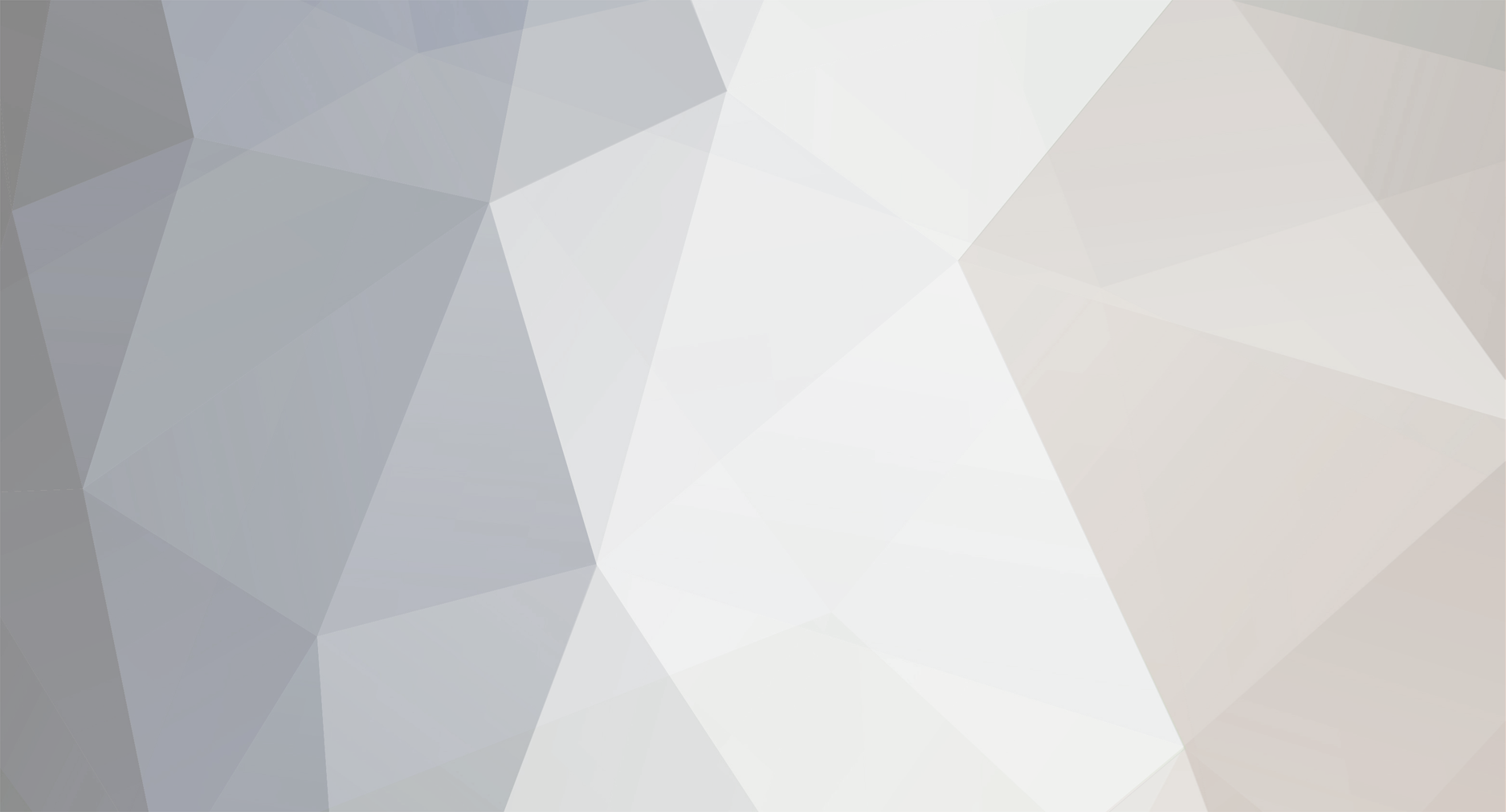
sasa
-
Posts
2,804 -
Joined
-
Last visited
-
Days Won
1
Posts posted by sasa
-
-
before echo category just check is it diferent thet last one
look mjdamato's example
-
for class='last' just count items i while loop and compare it with num_options
-
direct (no warping edge) distance from x1 to x2 is abs(x1 - x2) and it must be less then d
if edge distance is less then d that direct distance is greater than d complement (499 - d)
same for y
sorry i'm not good in english
-
maybe
<?php $x = $_GET['x']; $y = $_GET['y']; $d = $_GET['distance']; $d1 = 499 - $d; $condition = " WHERE (ABS(`x`-$x)<=$d OR ABS(`x`-$x)>=$d1) AND (ABS(`y`-$y)<=$d OR ABS(`y`-$y)>=$d1)"; ?>
ups i have two extra (
-
try
<?php function get_cat_selectlist($current_cat_id = 0) { mysql_connect('localhost','root','root'); mysql_select_db('sre'); $sql = 'SELECT cat_id, parent ,cat_name from `tblcatagory1` where parent = '.$current_cat_id; $get_options = mysql_query($sql); $num_options = mysql_num_rows($get_options); $out = ''; if ($num_options > 0) { // start ul tag $out = "<ul>\n"; while (list($cat_id, $parent, $cat_name) = mysql_fetch_row($get_options)) { $out .= '<li>'. $cat_name.'</li>'."\n"; $out .= get_cat_selectlist($cat_id); } $out .= "</ul>\n"; }//if greter the zero return $out; }//function echo get_cat_selectlist(); ?>
-
ups i make mistake
syntaks is table.field
so change 'school_district.hood' to 'hood.school_district'
-
(<color\s*="[0-9A-Za-z]+?"\s*>.*</color>)
or use htmlspecialchars_decode() function before regex
-
try
<?php $teststring = "2010-03-14,74527,645,New,7417417,Toll,2,74527417,32,202,7452741741,ghtgbwt,gtgwtgwtfgwtrg,2010-03-14,857527527,645,New,7417418,Toll,7,74527418,32,202,527527,hjm,djmdmdjmdjmdgjm,2010-03-14,85285,645,New,7417419,Toll,2,74527419,32,202,750757,jryjkryjkfryk,yjmyjyjtyjhm"; //$teststring1 = preg_replace("/([a-zA-Z0-9_\-]+?),/" , "\"$1\",",$teststring); //$teststring2 = preg_replace("/\,{13}/" , "],[",$teststring1); $a = array_chunk(explode(',',$teststring),13); foreach ($a as $k => $b) $a[$k] = '"'.implode('","', $b).'"'; $teststring2 = implode('],[',$a); var_dump($teststring2); ?>
-
try
<?php $test = 'blah #123 blah blah #4567 #sasa'; $out = preg_replace('/#[^ ]+/', '<a href=\'$0\'>$0</a>', $test); echo $out; ?>
-
it's php error
you miss some ; in the end of line
-
change line
input.name = 'distance[]' + lastRow;
to
input.name = 'distance[' + lastRow + ']';
and so on
-
"SELECT table1.* , table2.* FROM table1
LEFT JOIN table2 ON some_field.table1=field.table2
WHERE table1.id=".$_GET['id']
-
you try to open NOT exist picture and resize it
check that file exist
-
change line
<td colspan="2" style="padding-top:5px;"><?php echo $bbcode->parse($blogoutput); ?></td>
to
<td colspan="2" style="padding-top:5px;"><?php echo $bbcode->parse($row_Blogs['Content']); ?></td>
-
try
<html> <head> <title>Halo Custom Edition BC Kill Log</title> </head> <body> <?php $lines = file('halo.log'); $teams = array(); foreach($lines as $line) { if(preg_match('/PCR_PLAYER/',$line)) { $teams[] = $line; } } //$teamnames = array(); $teamscore = array(); foreach($teams as $team) { $teamarr = split(chr(9),$team); if(trim($teamarr[1]) == 'PCR_PLAYER' && preg_match("/»ßÇ«/", $teamarr[3], $matches)) { $name = $teamarr[3]; $name = preg_replace("/Player [0-9]*/", "", $name); $name = str_replace('"', '', $name); if (isset($teamscore[$name])) $teamscore[$name] += $teamarr[5]; else $teamscore[$name] = $teamarr[5]; } } ?> <table> <tr> <th>team</th> <th>score</th> </tr> <?php foreach($teamscore as $name => $score) { echo '<tr><td>'.$name.'</td><td>'.$score.'</td></tr>'; } ?> </table> </body> </html>
-
try
require("settings.php"); $extract = mysql_query("SELECT * FROM users"); $owerall = 0; while ($row = mysql_fetch_assoc($extract)) $overall += $row['frpcash']; echo "Total FrP cash: ". $overall ."";
-
change
<form method='post' action='test.php'>
to
<form method='post' action='test.php' style='display:inline;'>
-
| means or in regex
-
try to add
<style type="text/css"> a, span { float:right; margin-right:8; } </style>
to your script
-
try
<?php $test = "Along with the tutorials, the developers on the forum will be able to help you ... This covers updating your Dev server to recent versions of PHP and MySQL, ..."; $srch = "php mysql forum"; $srch = trim($srch); $srch = preg_replace('/\s+/','|',$srch); //replace spaces with | $test = preg_replace('/('.$srch.')/i', '<b>$1</b>', $test); echo $test; ?>
-
remove 'var'
-
-
you don't close else block opened in line 58
-
try to change your data array
<script> function addRow() { var tbl = document.getElementById('thetable'); var lastRow = tbl.rows.length; var row = tbl.insertRow(lastRow); var cell1 = row.insertCell(0); var input = document.createElement('input'); input.name = 'data[' + lastRow + '][date]'; input.id = 'date' + lastRow; cell1.appendChild(input); var cell2 = row.insertCell(1); input = document.createElement('input'); input.name = 'data[' + lastRow + '][am]'; input.id = 'am' + lastRow; cell2.appendChild(input); var cell3 = row.insertCell(2); input = document.createElement('input'); input.name = 'data[' + lastRow + '][pm]'; input.id = 'pm' + lastRow; cell3.appendChild(input); var cell4 = row.insertCell(3); input = document.createElement('input'); input.name = 'data[' + lastRow + '][comment]'; input.id = 'comment' + lastRow; cell4.appendChild(input); } function delRow() { var tbl = document.getElementById('thetable'); var lastRow = tbl.rows.length; if (lastRow > 2) tbl.deleteRow(lastRow - 1); } </script> <form action="javascript:get(document.getElementById('myform'));" name="myform" id="myform"> <table style="WIDTH: 100%; BORDER-COLLAPSE: collapse" id="thetable"> <tbody> <tr> <td> <p align="center">Date</p></td> <td> <p align="center">Am</p></td> <td> <p align="center">Pm</p></td> <td> <p align="center">Comment</p></td> </tr> <tr> <td> <input name="data[1][date]" /> </td> <td> <input name="data[1][am]" /> </td> <td> <input name="data[1][pm]" /></td> <td> <input name="data[1][comment]" /></td> </tr> </tbody> </table> <table style="WIDTH: 100%; BORDER-COLLAPSE: collapse"> <tr> <td> <p align="center"> <input type="button" value="Add Row" onclick="addRow()" /> <input type="button" value="Subtract Row" onclick="delRow()" /> </p> </td> </tr> </table>
Reordering table after delete
in PHP Coding Help
Posted
before delete pull position of deleted category then delete it and then