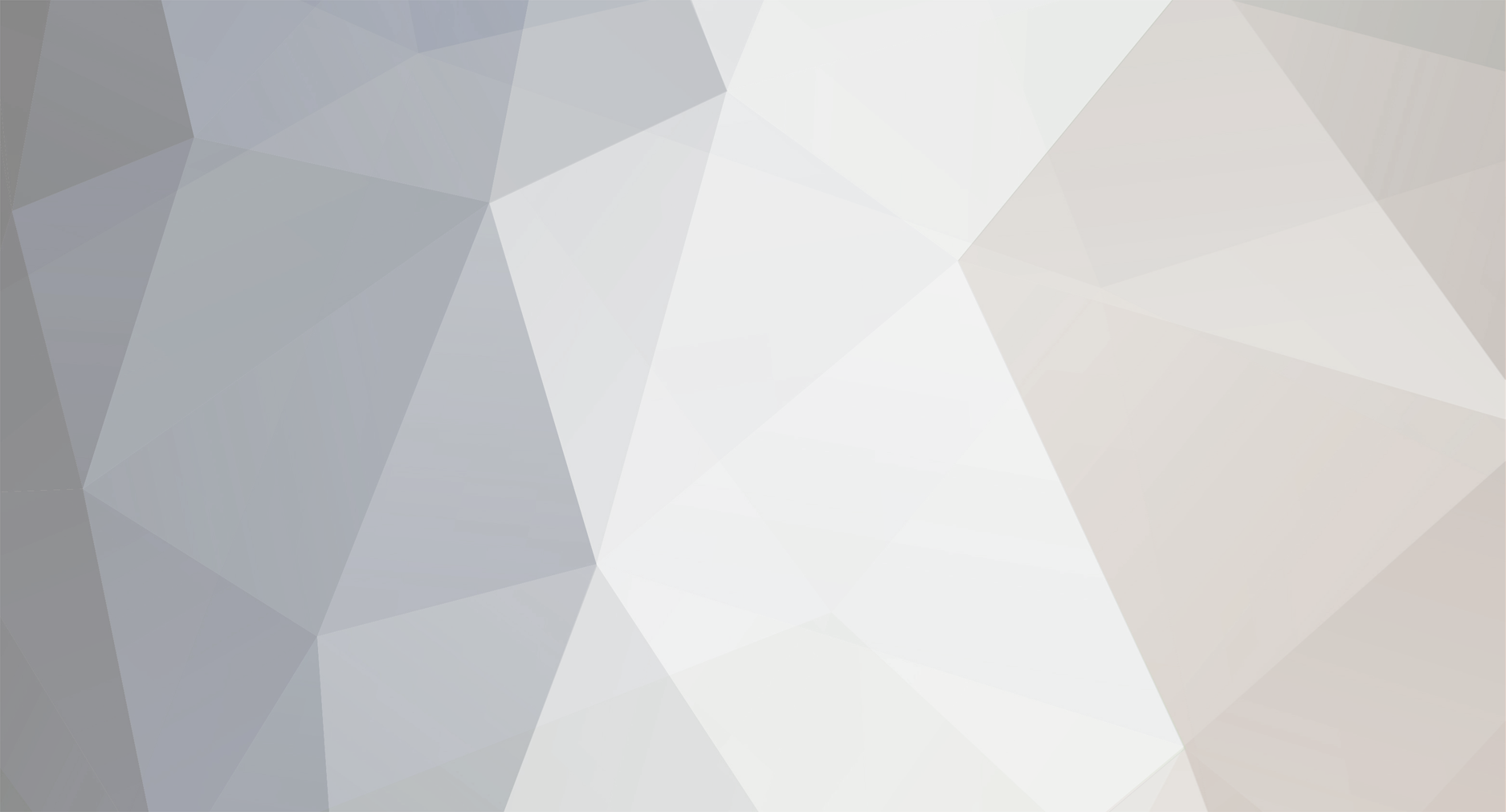
kenrbnsn
-
Posts
8,234 -
Joined
-
Last visited
Posts posted by kenrbnsn
-
-
First, when posting code on this forum, please put the code between
tags.
Next, Please describe your problem better.
Ken
-
Your server probably has runtime magic quotes turned on. If you have access to the php.ini file, turn them off. If you don't have access, get your hosting company to turn them off. Until that happens use stripslashes on the data before displaying it.
Ken
-
That's different. You need to put the column name in the select clause, not the value.
Plus you shouldn't use addslashes when adding data into the database, use mysql_real_escape_string which escapes many more problematic characters.
If you explain what you're trying to do with the retrieved data & how you're doing it, we can help you.
Ken
-
The img link uses $info['img'] which is different than $info['username']
Just because it works for one, doesn't mean it works for the other.
Ken
-
Is $info['username'] set to anything?
Put
<?php echo '<pre>' . print_r($info,true) . '</pre>'; ?>
at the start of your while loop. This will tell you what's being returned by the query.
Ken
-
This topic has been moved to Miscellaneous.
-
Either
<?php $query1 = "SELECT distinct link_id, url, title, description, " . stripslashes($fulltxt) . ", size FROM ".$mysql_table_prefix."links WHERE link_id in ($inlist)"; $result = mysql_query($query1); echo mysql_error(); ?>
or
<?php $fulltxt = stripslashes($fulltxt); $query1 = "SELECT distinct link_id, url, title, description, $fulltxt, size FROM ".$mysql_table_prefix."links WHERE link_id in ($inlist)"; $result = mysql_query($query1); echo mysql_error(); ?>
Ken
-
Or
<?php $amessage ="<a href='freindacept.php?username={$info['username']}'>Has sent you a friend request </a>'"; ?>
Ken
-
Do
<?php echo "<a href='viewprofile.php?username={$info['username']}'><img src='http://datenight.netne.net/images/{$info['img']}' title='{$info['username']}' width='50' height='50'></a>"; ?>
No escaped double quotes. Much cleaner IMHO.
Ken
-
You never close your validate_input function, plus there are many other syntax errors in your code. Like "<?php" & "?>" tags in the middle of the HEREDOC blocks.
Ken
-
If you use the explode method, you can use the little used third parameter to explode to limit the number of parts returned:
<?php $strs = array('1. item number 1', '12. item number 12', '101. item number 101'); foreach ($strs as $str) { list (,$str) = explode('.',$str,2); echo ltrim($str) . "<br>\n"; } ?>
Ken
-
The $_REQUEST array is populated via information on the URL or from a form. You really should use the $_GET array if the values are coming from the URL (or a form using the "get" method) or the $_POST array if the values come from a form with using the "post" method.
At the top of your script, put:
<?php if (isset($_POST)) { echo '<pre>$_POST: ' . print_r($_POST, true) . '</pre>'; echo '<pre>$_GET: ' . print_r($_GET,true) . '</pre>'; ?>
This will dump to the screen these arrays in a readable way.
To get around your problem, do something like:
<?php if(isset($_REQUEST['username']) && $_REQUEST['username'] == "rotten" && isset($_REQUEST['password']) && $_REQUEST['password'] == "1212"){ $_SESSION['username'] = "rotten"; $_SESSION['password'] = "1212"; header("Location: home.php ".$_SESSION['pageredirect']); } ?>
The isset function checks to see if the array entry is there before checking the value.
Ken
-
How is this code being invoked?
When posting code to this forum, please put it between
tags.
Ken
-
You can also write it like
<?php $image = "<img src='images/flags/{$row_rsPilots['country']}.gif' alt='' name='Flag' width='20' height='20' />"; $link = "http://www.domain.com/{$row_rsPilots['country']}"; if (!empty($rsPilots['country'])) { echo "<a href='$link'>$image</a>"; } ?>
which gets rid of the escaped double quotes and looks cleaner (IMHO).
Ken
-
Instead of doing this
<?php $concertDates = array(); ?>
at the start of your script, initialize the array with the values and remove all other lines that populate the array:
<?php $concertDates = array('201105071'=>'May 7, 2011 (9:00am - 12:00pm)', '201105072'=>'May 7, 2011 (1:00pm - 4:00pm)', '201105141'=>'May 14, 2011 (9:00am - 12:00pm)'); ?>
Ken
-
<?php echo $concertDates[$concertDate]; ?>
or
<?php $var = $concertDates[$concertDate]; echo $var; ?>
Ken
-
And your problem is?
-
Can you post the code where you set the cookie?
Ken
-
You're returning the totalcount from the function, but not doing anything with it when you call the function recursively. Try something like:
<?php include 'config.php'; // Connect database function leftcount($node) //Function to calculate leftcount { $sql = "SELECT lchild,rchild FROM tree WHERE parent = '$node'"; $execsql = mysql_query($sql); $array = mysql_fetch_array($execsql); if(!empty($array['lchild'])) { $count += leftcount($array['lchild']); } if(!empty($array['rchild'])) { $count += leftcount($array['rchild']); } $totalcount = 1 + $count; return $totalcount; } $parent = "2"; $left = leftcount($parent); echo $left; ?>
Ken
-
-
Did you try it?
It works fine.
Ken
-
This is most likely done with mod-rewrite in Apache.
Ken
-
It should echo "123"
Try
<?php $array = array("1","2","3"); foreach ($array as $value){ echo $value . '<br>'; } ?>
Another way to do this is
<?php $array = array("1","2","3"); echo implode('<br>',$array); ?>
Ken
-
Using a slash at the start of a path like that, say to start at the root of the whole file system, which is not what you want.
You want to do something like
<?php require_once $_SERVER['DOCUMENT_ROOT'] . '/config.inc.php'; ?>
If your file is in the webroot.
Ken
Extractign a Substring...
in PHP Coding Help
Posted
If the URL is always going to look similar to that example, you can do something like
Ken