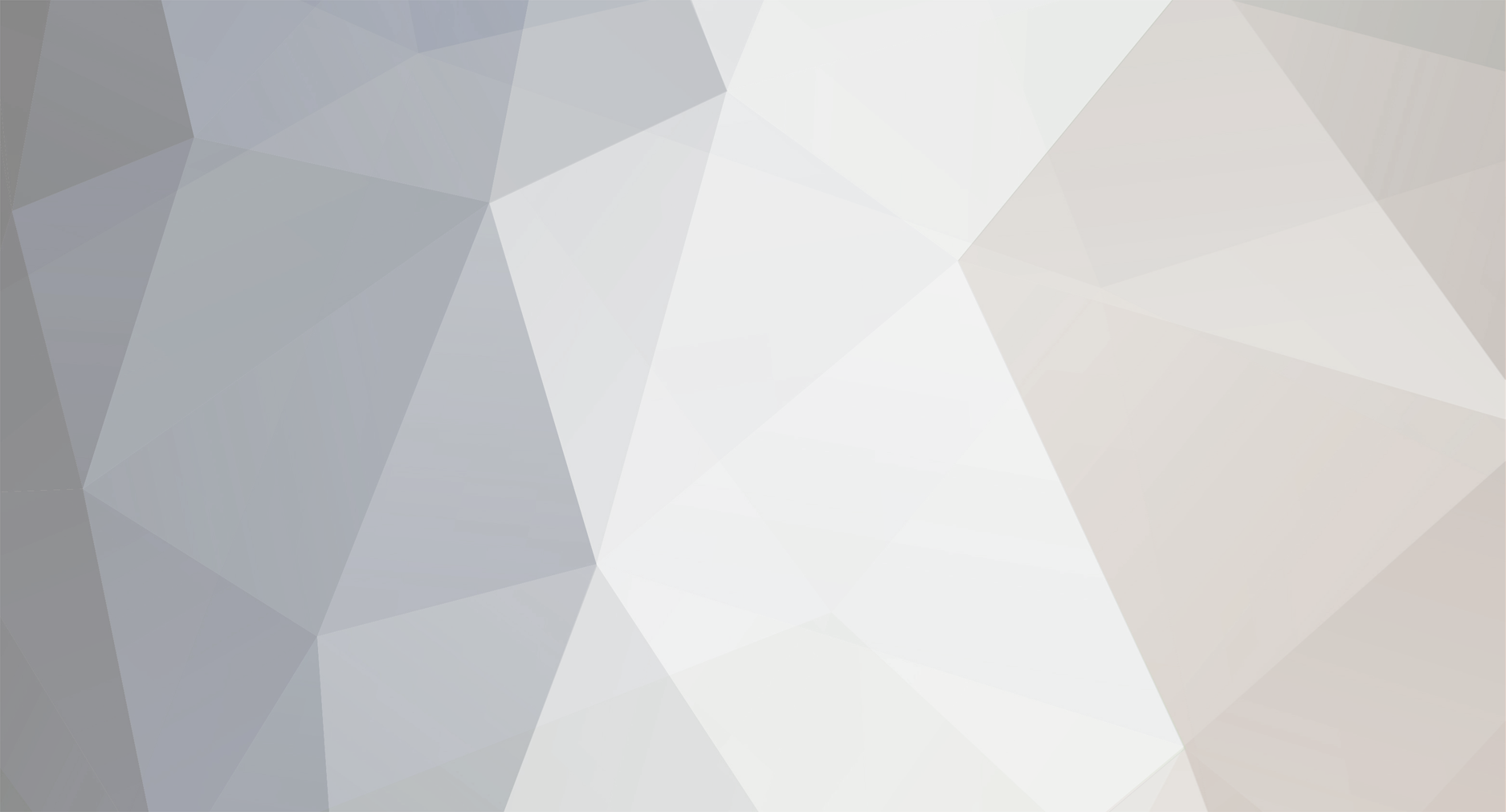
DBookatay
-
Posts
334 -
Joined
-
Last visited
Posts posted by DBookatay
-
-
// Nav Tabs $query = "select DISTINCT YEAR(sold_date) FROM Sold"; $result = mysql_query($query); $numrows = mysql_num_rows($result); while($row = mysql_fetch_array($result)){ $year = substr($row['sold_date'], 0, -6); $navTab .= '<a class="miniTabOn" href="index.php?date='.$year.'">'.$year.'</a>'; }
I am trying to create a link for each year of items in my database, yet the text is not showing up...
The "nav tab" is, because I can see the background image from the class="miniTabOn".
Can anyone spot an error?
-
Upon further review, the first position for substr() is 1.
I'm sorry but I don't understand
-
What you posted should work. However YEAR() would be clearer and probably faster -
SELECT * FROM Sold WHERE YEAR(sold_date) = 2010
My code didn't work, yours did though.
Thank you...
-
SELECT * FROM Sold WHERE substr(sold_date, 0, 4) = 2010
What I am trying to do is select all rows from the database from 2010, from the "sold_date" field.
How is this accomplished with date values? (ex: "2010-08-11", "2009-01-15")
-
Worked like a charm, thank you!
-
There's definitely a better way. You are doing almost the exact same code in each option. This is an obvious application for a function. Try something like this:
<?php function check_warr($term,$date_sold,$mileage) { list($len_text,$miles_text) = explode(' / ',$term); list($len,$type) = explode(' ',$len_text); list($miles) = explode(' ',$miles_text); $miles = str_replace(',',$miles); // get rid of comma $type = ($type == 'month')?'months':$type; $warr_date = strtotime($date_sold . '+' . $len . ' ' . $type); $warr_ext = array(date('m-d-Y',$warr_date),number_format($mileage + $miles) . ' miles'); $warr_text = ($warr_date > time())?'Expires':'<span class="red">Expired</span>'; $warr_ext[] = $warr_text; return ($warr_ext); } list($warr_Ext_Exp_Date, $warr_Ext_Exp_Miles, $warr_Ext_Exp_Text) = check_warr($row['warr_Ext_Term'],$date_sold,$row['mileage']); ?>
Note: not tested, may contain syntax errors.
Ken
WOW! This works MUCH better!
Thank you very much!!!
There is one error: "Wrong parameter count for str_replace() on line 225"
-
This is the entire code, with all the different options.
There has to be a better way!
switch($row['warr_Ext_Term']) { case '90 days / 4,500 miles': $day90 = date('Y-m-d',strtotime($date_sold . '+90 days')); $warr_Ext_Exp_Date = substr($day90, 5, 2).'-'.substr($day90, 8, 2).'-'.substr($day90, 2, 2); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 4500).' miles'; $today = date('Y-m-d'); if ($day90 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '180 days / 7,500 miles': $day180 = date('Y-m-d',strtotime($date_sold . '+180 days')); $warr_Ext_Exp_Date = substr($day180, 5, 2).'-'.substr($day180, 8, 2).'-'.substr($day180, 2, 2); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 7500).' miles'; $today = date('Y-m-d'); if ($day180 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '6 month / 6,000 miles': $month6 = date('Y-m-d',strtotime($date_sold . '+6 months')); $warr_Ext_Exp_Date = substr($month6, 5, 2).'-'.substr($month6, 8, 2).'-'.substr($month6, 2, 2); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 6000).' miles'; $today = date('Y-m-d'); if ($month6 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '12 month / 12,000 miles': $month12 = date('Y-m-d',strtotime($date_sold . '+12 months')); $warr_Ext_Exp_Date = substr($month12, 5, 2).'-'.substr($month12, 8, 2).'-'.substr($month12, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 12000).' miles'; $today = date('Y-m-d'); if ($month12 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '12 month / 14,500 miles': $month12 = date('Y-m-d',strtotime($date_sold . '+12 months')); $warr_Ext_Exp_Date = substr($month12, 5, 2).'-'.substr($month12, 8, 2).'-'.substr($month12, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 14500).' miles'; $today = date('Y-m-d'); if ($month12 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '12 month / 15,000 miles': $month12 = date('Y-m-d',strtotime($date_sold . '+12 months')); $warr_Ext_Exp_Date = substr($month12, 5, 2).'-'.substr($month12, 8, 2).'-'.substr($month12, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 15000).' miles'; $today = date('Y-m-d'); if ($month12 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '13 month / 13,000 miles': $month13 = date('Y-m-d',strtotime($date_sold . '+13 months')); $warr_Ext_Exp_Date = substr($month13, 5, 2).'-'.substr($month13, 8, 2).'-'.substr($month13, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 13000).' miles'; $today = date('Y-m-d'); if ($month13 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '14 month / 14,000 miles': $month14 = date('Y-m-d',strtotime($date_sold . '+14 months')); $warr_Ext_Exp_Date = substr($month14, 5, 2).'-'.substr($month14, 8, 2).'-'.substr($month14, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 14000).' miles'; $today = date('Y-m-d'); if ($month14 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '15 month / 15,000 miles': $month15 = date('Y-m-d',strtotime($date_sold . '+15 months')); $warr_Ext_Exp_Date = substr($month15, 5, 2).'-'.substr($month15, 8, 2).'-'.substr($month15, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 15000).' miles'; $today = date('Y-m-d'); if ($month15 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '16 month / 16,000 miles': $month16 = date('Y-m-d',strtotime($date_sold . '+16 months')); $warr_Ext_Exp_Date = substr($month16, 5, 2).'-'.substr($month16, 8, 2).'-'.substr($month16, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 16000).' miles'; $today = date('Y-m-d'); if ($month16 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '17 month / 17,000 miles': $month17 = date('Y-m-d',strtotime($date_sold . '+17 months')); $warr_Ext_Exp_Date = substr($month17, 5, 2).'-'.substr($month17, 8, 2).'-'.substr($month17, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 17000).' miles'; $today = date('Y-m-d'); if ($month17 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '18 month / 18,000 miles': $month18 = date('Y-m-d',strtotime($date_sold . '+18 months')); $warr_Ext_Exp_Date = substr($month18, 5, 2).'-'.substr($month18, 8, 2).'-'.substr($month18, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 18000).' miles'; $today = date('Y-m-d'); if ($month18 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '19 month / 19,000 miles': $month19 = date('Y-m-d',strtotime($date_sold . '+19 months')); $warr_Ext_Exp_Date = substr($month19, 5, 2).'-'.substr($month19, 8, 2).'-'.substr($month19, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 19000).' miles'; $today = date('Y-m-d'); if ($month19 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '20 month / 20,000 miles': $month20 = date('Y-m-d',strtotime($date_sold . '+20 months')); $warr_Ext_Exp_Date = substr($month20, 5, 2).'-'.substr($month20, 8, 2).'-'.substr($month20, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 20000).' miles'; $today = date('Y-m-d'); if ($month20 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '21 month / 21,000 miles': $month21 = date('Y-m-d',strtotime($date_sold . '+21 months')); $warr_Ext_Exp_Date = substr($month21, 5, 2).'-'.substr($month21, 8, 2).'-'.substr($month21, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 21000).' miles'; $today = date('Y-m-d'); if ($month21 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '22 month / 22,000 miles': $month22 = date('Y-m-d',strtotime($date_sold . '+22 months')); $warr_Ext_Exp_Date = substr($month22, 5, 2).'-'.substr($month22, 8, 2).'-'.substr($month22, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 22000).' miles'; $today = date('Y-m-d'); if ($month22 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '23 month / 23,000 miles': $month23 = date('Y-m-d',strtotime($date_sold . '+23 months')); $warr_Ext_Exp_Date = substr($month23, 5, 2).'-'.substr($month23, 8, 2).'-'.substr($month23, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 23000).' miles'; $today = date('Y-m-d'); if ($month23 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '24 month / 24,000 miles': $month24 = date('Y-m-d',strtotime($date_sold . '+24 months')); $warr_Ext_Exp_Date = substr($month24, 5, 2).'-'.substr($month24, 8, 2).'-'.substr($month24, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 24000).' miles'; $today = date('Y-m-d'); if ($month24 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '24 month / 26,500 miles': $month24 = date('Y-m-d',strtotime($date_sold . '+24 months')); $warr_Ext_Exp_Date = substr($month24, 5, 2).'-'.substr($month24, 8, 2).'-'.substr($month24, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 26500).' miles'; $today = date('Y-m-d'); if ($month24 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '24 month / 30,000 miles': $month24 = date('Y-m-d',strtotime($date_sold . '+24 months')); $warr_Ext_Exp_Date = substr($month24, 5, 2).'-'.substr($month24, 8, 2).'-'.substr($month24, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 30000).' miles'; $today = date('Y-m-d'); if ($month24 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '25 month / 25,000 miles': $month25 = date('Y-m-d',strtotime($date_sold . '+25 months')); $warr_Ext_Exp_Date = substr($month25, 5, 2).'-'.substr($month25, 8, 2).'-'.substr($month25, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 25000).' miles'; $today = date('Y-m-d'); if ($month25 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '26 month / 26,000 miles': $month26 = date('Y-m-d',strtotime($date_sold . '+26 months')); $warr_Ext_Exp_Date = substr($month26, 5, 2).'-'.substr($month26, 8, 2).'-'.substr($month26, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 26000).' miles'; $today = date('Y-m-d'); if ($month26 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '27 month / 27,000 miles': $month27 = date('Y-m-d',strtotime($date_sold . '+27 months')); $warr_Ext_Exp_Date = substr($month27, 5, 2).'-'.substr($month27, 8, 2).'-'.substr($month27, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 27000).' miles'; $today = date('Y-m-d'); if ($month27 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '28 month / 28,000 miles': $month28 = date('Y-m-d',strtotime($date_sold . '+28 months')); $warr_Ext_Exp_Date = substr($month28, 5, 2).'-'.substr($month28, 8, 2).'-'.substr($month28, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 28000).' miles'; $today = date('Y-m-d'); if ($month28 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '29 month / 29,000 miles': $month29 = date('Y-m-d',strtotime($date_sold . '+29 months')); $warr_Ext_Exp_Date = substr($month29, 5, 2).'-'.substr($month29, 8, 2).'-'.substr($month29, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 29000).' miles'; $today = date('Y-m-d'); if ($month29 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '30 month / 30,000 miles': $month30 = date('Y-m-d',strtotime($date_sold . '+30 months')); $warr_Ext_Exp_Date = substr($month30, 5, 2).'-'.substr($month30, 8, 2).'-'.substr($month30, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 30000).' miles'; $today = date('Y-m-d'); if ($month30 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '31 month / 31,000 miles': $month31 = date('Y-m-d',strtotime($date_sold . '+31 months')); $warr_Ext_Exp_Date = substr($month31, 5, 2).'-'.substr($month31, 8, 2).'-'.substr($month31, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 31000).' miles'; $today = date('Y-m-d'); if ($month31 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '32 month / 32,000 miles': $month32 = date('Y-m-d',strtotime($date_sold . '+32 months')); $warr_Ext_Exp_Date = substr($month32, 5, 2).'-'.substr($month32, 8, 2).'-'.substr($month32, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 32000).' miles'; $today = date('Y-m-d'); if ($month32 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '33 month / 33,000 miles': $month33 = date('Y-m-d',strtotime($date_sold . '+33 months')); $warr_Ext_Exp_Date = substr($month33, 5, 2).'-'.substr($month33, 8, 2).'-'.substr($month33, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 33000).' miles'; $today = date('Y-m-d'); if ($month33 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '34 month / 34,000 miles': $month34 = date('Y-m-d',strtotime($date_sold . '+34 months')); $warr_Ext_Exp_Date = substr($month34, 5, 2).'-'.substr($month34, 8, 2).'-'.substr($month34, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 34000).' miles'; $today = date('Y-m-d'); if ($month34 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '35 month / 35,000 miles': $month35 = date('Y-m-d',strtotime($date_sold . '+35 months')); $warr_Ext_Exp_Date = substr($month35, 5, 2).'-'.substr($month35, 8, 2).'-'.substr($month35, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 35000).' miles'; $today = date('Y-m-d'); if ($month35 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '36 month / 36,000 miles': $month36 = date('Y-m-d',strtotime($date_sold . '+36 months')); $warr_Ext_Exp_Date = substr($month36, 5, 2).'-'.substr($month36, 8, 2).'-'.substr($month36, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 36000).' miles'; $today = date('Y-m-d'); if ($month36 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '36 month / 38,500 miles': $month36 = date('Y-m-d',strtotime($date_sold . '+36 months')); $warr_Ext_Exp_Date = substr($month36, 5, 2).'-'.substr($month36, 8, 2).'-'.substr($month36, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 38500).' miles'; $today = date('Y-m-d'); if ($month36 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '36 month / 45,000 miles': $month36 = date('Y-m-d',strtotime($date_sold . '+36 months')); $warr_Ext_Exp_Date = substr($month36, 5, 2).'-'.substr($month36, 8, 2).'-'.substr($month36, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 45000).' miles'; $today = date('Y-m-d'); if ($month36 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '37 month / 37,000 miles': $month37 = date('Y-m-d',strtotime($date_sold . '+37 months')); $warr_Ext_Exp_Date = substr($month37, 5, 2).'-'.substr($month37, 8, 2).'-'.substr($month37, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 37000).' miles'; $today = date('Y-m-d'); if ($month37 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '38 month / 38,000 miles': $month38 = date('Y-m-d',strtotime($date_sold . '+38 months')); $warr_Ext_Exp_Date = substr($month38, 5, 2).'-'.substr($month38, 8, 2).'-'.substr($month38, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 38000).' miles'; $today = date('Y-m-d'); if ($month38 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '39 month / 39,000 miles': $month39 = date('Y-m-d',strtotime($date_sold . '+39 months')); $warr_Ext_Exp_Date = substr($month39, 5, 2).'-'.substr($month39, 8, 2).'-'.substr($month39, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 39000).' miles'; $today = date('Y-m-d'); if ($month39 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '40 month / 40,000 miles': $month40 = date('Y-m-d',strtotime($date_sold . '+40 months')); $warr_Ext_Exp_Date = substr($month40, 5, 2).'-'.substr($month40, 8, 2).'-'.substr($month40, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 40000).' miles'; $today = date('Y-m-d'); if ($month40 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '41 month / 41,000 miles': $month41 = date('Y-m-d',strtotime($date_sold . '+41 months')); $warr_Ext_Exp_Date = substr($month41, 5, 2).'-'.substr($month41, 8, 2).'-'.substr($month41, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 41000).' miles'; $today = date('Y-m-d'); if ($month48 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '42 month / 42,000 miles': $month42 = date('Y-m-d',strtotime($date_sold . '+42 months')); $warr_Ext_Exp_Date = substr($month42, 5, 2).'-'.substr($month42, 8, 2).'-'.substr($month42, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 42000).' miles'; $today = date('Y-m-d'); if ($month48 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '43 month / 43,000 miles': $month43 = date('Y-m-d',strtotime($date_sold . '+43 months')); $warr_Ext_Exp_Date = substr($month43, 5, 2).'-'.substr($month43, 8, 2).'-'.substr($month43, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 43000).' miles'; $today = date('Y-m-d'); if ($month43 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '44 month / 44,000 miles': $month44 = date('Y-m-d',strtotime($date_sold . '+44 months')); $warr_Ext_Exp_Date = substr($month44, 5, 2).'-'.substr($month44, 8, 2).'-'.substr($month44, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 44000).' miles'; $today = date('Y-m-d'); if ($month44 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '45 month / 45,000 miles': $month45 = date('Y-m-d',strtotime($date_sold . '+45 months')); $warr_Ext_Exp_Date = substr($month45, 5, 2).'-'.substr($month45, 8, 2).'-'.substr($month45, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 45000).' miles'; $today = date('Y-m-d'); if ($month45 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '46 month / 46,000 miles': $month46 = date('Y-m-d',strtotime($date_sold . '+46 months')); $warr_Ext_Exp_Date = substr($month46, 5, 2).'-'.substr($month46, 8, 2).'-'.substr($month46, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 46000).' miles'; $today = date('Y-m-d'); if ($month46 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '47 month / 47,000 miles': $month47 = date('Y-m-d',strtotime($date_sold . '+47 months')); $warr_Ext_Exp_Date = substr($month47, 5, 2).'-'.substr($month47, 8, 2).'-'.substr($month47, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 47000).' miles'; $today = date('Y-m-d'); if ($month47 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '48 month / 48,000 miles': $month48 = date('Y-m-d',strtotime($date_sold . '+48 months')); $warr_Ext_Exp_Date = substr($month48, 5, 2).'-'.substr($month48, 8, 2).'-'.substr($month48, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 48000).' miles'; $today = date('Y-m-d'); if ($month48 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '48 month / 50,000 miles': $month48 = date('Y-m-d',strtotime($date_sold . '+48 months')); $warr_Ext_Exp_Date = substr($month48, 5, 2).'-'.substr($month48, 8, 2).'-'.substr($month48, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 50000).' miles'; $today = date('Y-m-d'); if ($month48 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '48 month / 75,000 miles': $month48 = date('Y-m-d',strtotime($date_sold . '+48 months')); $warr_Ext_Exp_Date = substr($month48, 5, 2).'-'.substr($month48, 8, 2).'-'.substr($month48, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 75000).' miles'; $today = date('Y-m-d'); if ($month48 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '49 month / 49,000 miles': $month49 = date('Y-m-d',strtotime($date_sold . '+49 months')); $warr_Ext_Exp_Date = substr($month49, 5, 2).'-'.substr($month49, 8, 2).'-'.substr($month49, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 49000).' miles'; $today = date('Y-m-d'); if ($month49 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '50 month / 50,000 miles': $month50 = date('Y-m-d',strtotime($date_sold . '+50 months')); $warr_Ext_Exp_Date = substr($month50, 5, 2).'-'.substr($month50, 8, 2).'-'.substr($month50, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 50000).' miles'; $today = date('Y-m-d'); if ($month50 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '51 month / 51,000 miles': $month51 = date('Y-m-d',strtotime($date_sold . '+51 months')); $warr_Ext_Exp_Date = substr($month51, 5, 2).'-'.substr($month51, 8, 2).'-'.substr($month51, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 51000).' miles'; $today = date('Y-m-d'); if ($month51 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '52 month / 52,000 miles': $month52 = date('Y-m-d',strtotime($date_sold . '+52 months')); $warr_Ext_Exp_Date = substr($month52, 5, 2).'-'.substr($month52, 8, 2).'-'.substr($month52, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 52000).' miles'; $today = date('Y-m-d'); if ($month52 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '53 month / 53,000 miles': $month53 = date('Y-m-d',strtotime($date_sold . '+53 months')); $warr_Ext_Exp_Date = substr($month53, 5, 2).'-'.substr($month53, 8, 2).'-'.substr($month53, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 53000).' miles'; $today = date('Y-m-d'); if ($month51 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '54 month / 54,000 miles': $month54 = date('Y-m-d',strtotime($date_sold . '+54 months')); $warr_Ext_Exp_Date = substr($month54, 5, 2).'-'.substr($month54, 8, 2).'-'.substr($month54, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 54000).' miles'; $today = date('Y-m-d'); if ($month54 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '55 month / 55,000 miles': $month55 = date('Y-m-d',strtotime($date_sold . '+55 months')); $warr_Ext_Exp_Date = substr($month55, 5, 2).'-'.substr($month55, 8, 2).'-'.substr($month55, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 55000).' miles'; $today = date('Y-m-d'); if ($month55 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '56 month / 56,000 miles': $month56 = date('Y-m-d',strtotime($date_sold . '+56 months')); $warr_Ext_Exp_Date = substr($month56, 5, 2).'-'.substr($month56, 8, 2).'-'.substr($month56, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 56000).' miles'; $today = date('Y-m-d'); if ($month56 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '57 month / 57,000 miles': $month57 = date('Y-m-d',strtotime($date_sold . '+57 months')); $warr_Ext_Exp_Date = substr($month57, 5, 2).'-'.substr($month57, 8, 2).'-'.substr($month57, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 57000).' miles'; $today = date('Y-m-d'); if ($month57 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '60 month / 60,000 miles': $month60 = date('Y-m-d',strtotime($date_sold . '+60 months')); $warr_Ext_Exp_Date = substr($month60, 5, 2).'-'.substr($month60, 8, 2).'-'.substr($month60, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 60000).' miles'; $today = date('Y-m-d'); if ($month60 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '60 month / 64,000 miles': $month60 = date('Y-m-d',strtotime($date_sold . '+60 months')); $warr_Ext_Exp_Date = substr($month60, 5, 2).'-'.substr($month60, 8, 2).'-'.substr($month60, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 64000).' miles'; $today = date('Y-m-d'); if ($month60 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '60 month / 70,000 miles': $month60 = date('Y-m-d',strtotime($date_sold . '+60 months')); $warr_Ext_Exp_Date = substr($month60, 5, 2).'-'.substr($month60, 8, 2).'-'.substr($month60, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 70000).' miles'; $today = date('Y-m-d'); if ($month60 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '60 month / 100,000 miles': $month60 = date('Y-m-d',strtotime($date_sold . '+60 months')); $warr_Ext_Exp_Date = substr($month60, 5, 2).'-'.substr($month60, 8, 2).'-'.substr($month60, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 100000).' miles'; $today = date('Y-m-d'); if ($month60 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '72 month / 70,000 miles': $month72 = date('Y-m-d',strtotime($date_sold . '+72 months')); $warr_Ext_Exp_Date = substr($month72, 5, 2).'-'.substr($month72, 8, 2).'-'.substr($month72, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 70000).' miles'; $today = date('Y-m-d'); if ($month72 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '72 month / 90,000 miles': $month72 = date('Y-m-d',strtotime($date_sold . '+72 months')); $warr_Ext_Exp_Date = substr($month72, 5, 2).'-'.substr($month72, 8, 2).'-'.substr($month72, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 90000).' miles'; $today = date('Y-m-d'); if ($month72 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '84 month / 100,000 miles': $month84 = date('Y-m-d',strtotime($date_sold . '+84 months')); $warr_Ext_Exp_Date = substr($month84, 5, 2).'-'.substr($month84, 8, 2).'-'.substr($month84, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 100000).' miles'; $today = date('Y-m-d'); if ($month84 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; case '120 month / 100,000 miles': $month120 = date('Y-m-d',strtotime($date_sold . '+120 months')); $warr_Ext_Exp_Date = substr($month120, 5, 2).'-'.substr($month120, 8, 2).'-'.substr($month120, 0, 4); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 100000).' miles'; $today = date('Y-m-d'); if ($month120 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; }
-
I assume you are using a database for this. If so, why not use a ID for each warranty period, ie 1 = 9 days/4500 miles. Inside that row, also store the days and miles, and you can use that column data as your variable to do computations against. That should allow you to remove the entire Switch block.
Because the database is spanning back 7 years worth of information.
-
I have a "switch statement" that is a bit ridiculous
switch($row['warr_Ext_Term']) { case '24 month / 24,000 miles': $month24 = date('Y-m-d',strtotime($date_sold . '+730 days')); $warr_Ext_Exp_Date = substr($month24, 5, 2).'-'.substr($month24, 8, 2).'-'.substr($month24, 2, 2); $warr_Ext_Exp_Miles = number_format($row['mileage'] + 24000).' miles'; $today = date('Y-m-d'); if ($month24 > $today) { $warr_Ext_Exp_Text = 'Expires'; } else { $class = 'red'; $warr_Ext_Exp_Text = '<span class="'.$class.'">Expired</span>'; } break; } $warr_Ext_Cmpy = ' <li><label>Extended</label>'.$row['warr_Ext_Cmpy'].'</li> <li><label>Term</label>'.$row['warr_Ext_Term'].'</li> <li><label>Contract #</label>'.$row['warr_Ext_Num'].'</li> <li><label>'.$warr_Ext_Exp_Text.'</label><span class="'.$class.'">'.$warr_Ext_Exp_Date.'</span><br><label> </label><span class="'.$class.'">'.$warr_Ext_Exp_Miles.'</span></li>' ;
What it does is determines when a customers warranty expires, or when it did expire based on the miles that they bought the vehicle at, and based on today's date.
The problem is that I sell numerous warranties from 9 days, 4,500 miles up to 120 months, 100,000 miles.
Is there a better way to write this, based on the info pulled from the "$row['warr_Ext_Term']" row?
Any help would be greatly appreciated...
-
I have a SQL row that has a date field: ex: 2010-11-01.
When a car is sold there either is a 30 day warranty, a 60 day warranty, or 0 day warranty.
What I'm trying to do is display when the vehicles warranty expires, based on the date it was sold, or when did it expire based on the same sold date pulled from the database.
Example using last months date: 2010-10-01
60 day: "Expires 11-30-10"
30 day: "Expired 11-01-10"
I can not seem to use the date function properly...
Any help would be greatly appreciated.
-
The query would look something like (test it first on a sample of your data to be sure) -
UPDATE your_table SET sold_date = CONCAT('20',sold_date3,'-',sold_date1,'-',sold_date2);
2 follow up questions, and forgive me because I'm still learning.
1. This database was started in 1998, so how do I add
CONCAT('19',sold_date3,'-',sold_date1,'-',sold_date2);
if it's a 1998 or 1999 and then a
CONCAT('20',sold_date3,'-',sold_date1,'-',sold_date2);
if it's year 2000 and up.
2. If there already is the proper date in the "sold_date" field, how do I exclude those. Meaning I only want to alter the rows that have "sold_date" as "0000-00-00" AND data in the "sold_date1", "sold_date2" and "sold_date3" fields.
-
I want the SQL code that will pull the values from sold_date1, sold_date2, and sold_date3 and put them combined into "sold_date".
Then I can delete the rows and content of sold_date1, sold_date2, and sold_date3.
-
I first created my database several years ago, and have since been trying to make changes to it that makes it "proper."
I have 3 char(2) rows: sold_date1, sold_date2 and sold_date3, with values such as "10", "12", "09".
How do I take the values from those rows and convert and update them so that they get inserted into a new row "sold_date" with the "date" type?
Example "2009-10-12"
-
The example you are using relies on a very old feature/bug called register globals. You still have undefined variables.
Where is $price_asking, $vin and $stock defined? You use them in your query but don't define them anywhere.
The data your looking to use within your query will be found within the $_POST array.
Instead of looking around for examples of simple code (and finding terrible examples at that) why not try learning PHP yourself? Scripts like this are really quite straight forward once you know the basics.
That is how I have been learning, buy looking at working examples... Do you know of a good working example?
-
The error says it all. $Submit isn't defined anywhere in your script.
Change it to $_POST['Submit']
I did this:
<?php if($_POST['Submit']){ for($i=0;$i<$count;$i++){ $sql1="UPDATE Inventory SET vin='$vin[$i]', price_asking='$price_asking[$i]' WHERE stock='$stock[$i]'"; $result1=mysql_query($sql1); } if($result1){header("location:test.php");} } mysql_close(); ?>
and it deleted every row in the dB. (Good thing I had it saved)
Does anyone have a better working example than the one I found?
-
Anyone?
-
-
I added your ini_set code and got the following errors:
Notice: Undefined variable: Submit in /home/.phillipina/dbookatay/login.carcityofdanbury.com/passed/New/test.php on line 49
Notice: Undefined variable: result1 in /home/.phillipina/dbookatay/login.carcityofdanbury.com/passed/New/test.php on line 62
-
I found a script online to upload multiple rows at one time, but can not get the thing to work...
Anyone spot any errors?
<?php $host="xxxxxxxxxxxxxxxxxxxx"; // Host name $username="xxxxxx"; // Mysql username $password="xxxxx"; // Mysql password $db_name="xxxxxx"; // Database name // Connect to server and select databse. mysql_connect("$host", "$username", "$password")or die("cannot connect"); mysql_select_db("$db_name")or die("cannot select DB"); $sql="SELECT * FROM Inventory ORDER BY year DESC"; $result=mysql_query($sql); // Count table rows $count=mysql_num_rows($result); ?> <table> <form name="form1" method="post" action="test.php"> <tr> <td> <table> <tr> <td>Stock</td> <td>VIN</td> <td>Asking</td> </tr> <? while($rows=mysql_fetch_array($result)){ ?> <tr> <td><? $id[]=$rows['stock']; ?><? echo $rows['stock']; ?></td> <td><input size="19" name="vin[]" type="text" id="vin" value="<? echo $rows['vin']; ?>"></td> <td><input size="3" name="price_asking[]" type="text" id="price_asking" value="<? echo $rows['price_asking']; ?>"></td> </tr> <? } ?> <tr><td colspan="9" align="right"><input type="submit" name="Submit" value="Submit"></td></tr> </table> </td> </tr> </form> </table> <?php // Check if button name "Submit" is active, do this if($Submit){ for($i=0;$i<$count;$i++){ $sql1="UPDATE Inventory SET vin='$vin[$i]', price_asking='$price_asking[$i]' WHERE stock='$id[$i]'"; $result1=mysql_query($sql1); } } if($result1){ header("location:test.php"); } mysql_close(); ?>
-
Thank you!
-
Well there are quite a few:
#1
$time = strtotime($row['date']); print date('r', $time);
#2
list($year, $month, $day) = explode('-', $row['date']); print date('r', mktime(0, 0, 0, $month, $day, $year));
Ok, thanks.
This is how I had it:
$month = substr($row['date'], 5, 2); switch($month){ case '01': $month = 'January'; break; case '02': $month = 'Febuary'; break; case '03': $month = 'March'; break; case '04': $month = 'April'; break; case '05': $month = 'May'; break; case '06': $month = 'June'; break; case '07': $month = 'July'; break; case '08': $month = 'August'; break; case '09': $month = 'September'; break; case '10': $month = 'October'; break; case '11': $month = 'November'; break; case '12': $month = 'December'; break; } $day = substr($row['date'], 8, 2); switch($day){ case '01': $day = '1st'; break; case '02': $day = '2nd'; break; case '03': $day = '3rd'; break; case '04': $day = '4th'; break; case '05': $day = '5th'; break; case '06': $day = '6th'; break; case '07': $day = '7th'; break; case '08': $day = '8th'; break; case '09': $day = '9th'; break; case '10': $day = '10th'; break; case '11': $day = '11th'; break; case '12': $day = '12th'; break; case '13': $day = '13th'; break; case '14': $day = '14th'; break; case '15': $day = '15th'; break; case '16': $day = '16th'; break; case '17': $day = '17th'; break; case '18': $day = '18th'; break; case '19': $day = '19th'; break; case '20': $day = '20th'; break; case '21': $day = '21st'; break; case '22': $day = '22nd'; break; case '23': $day = '23rd'; break; case '24': $day = '24th'; break; case '25': $day = '25th'; break; case '26': $day = '26th'; break; case '27': $day = '27th'; break; case '28': $day = '28th'; break; case '29': $day = '29th'; break; case '30': $day = '30th'; break; } $year = substr($row['date'], 0, 4); $date = $month.' '.$day.', '.$year;
but figured there had to be an easier way of doing it...
-
How do I convert the value of date row "2007-05-19", it something that displays like: May 19th, 2007?
I've tried every possible scenerio that I can think of with no luck...
-
Anyone have any suggestions?
I solved it myself...
$date1 = date("Y").'-'.date(d).'-'.date("m"); ----- should be ----- $date1 = date("Y").'-'.date(m).'-'.date("d");
-
Anyone have any suggestions?
Can not figure out this DATE query
in PHP Coding Help
Posted
Would you mind explaining? I dont understand...