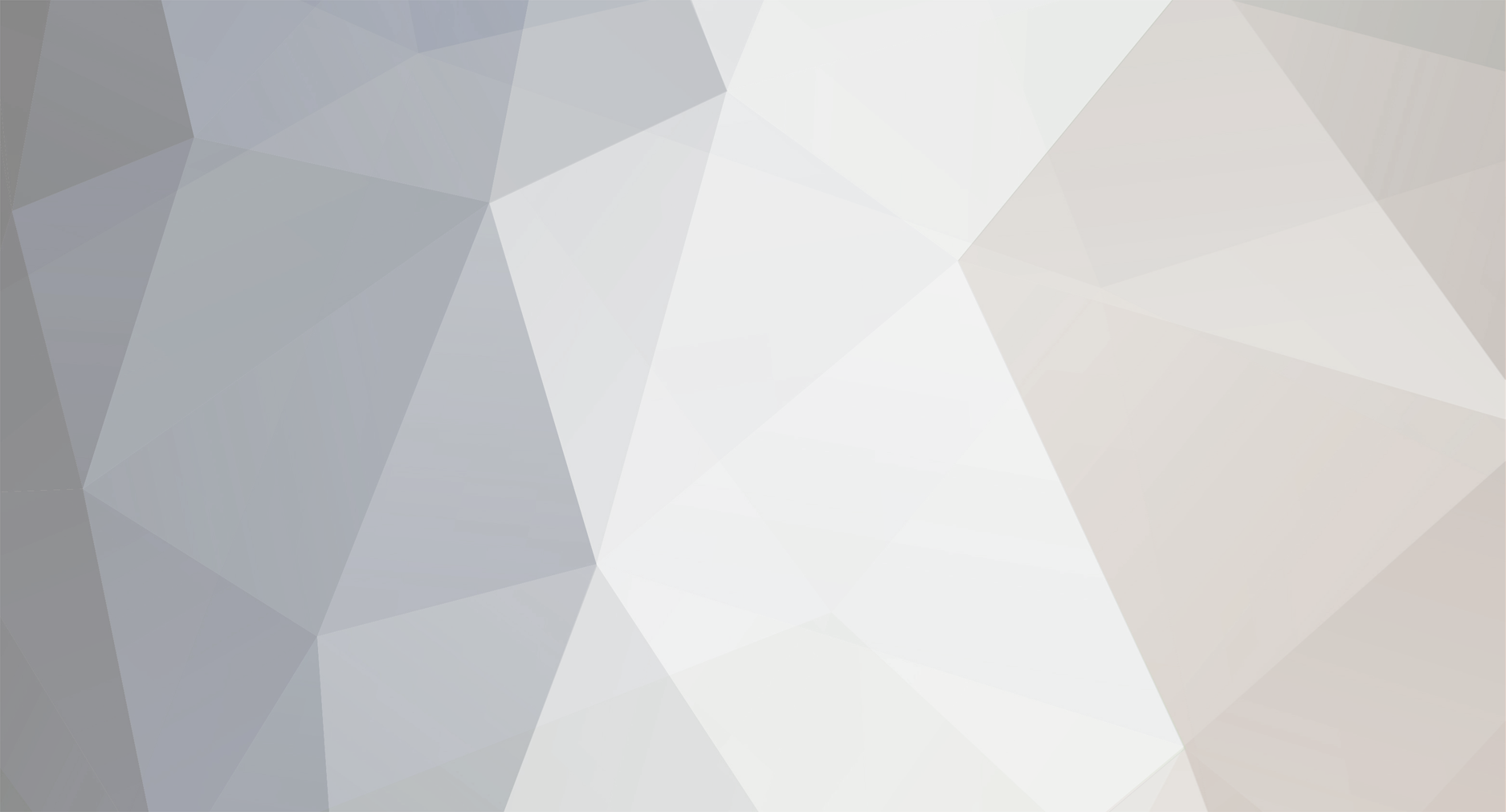
jworisek
Members-
Posts
112 -
Joined
-
Last visited
Never
Everything posted by jworisek
-
when you are designing a relational database (like I suggested) it really isn't a big deal if you make effective use of indexes. In the database I work on we have about a years worth of data in the new structure I moved us to and some of the relational tables have over 45,000 rows in them. I do this for work... my company has around 1600 products in the relational database and we use it to track all of our orders, do invoices and all that kinds of stuff. I have had no problems with speed, although it will really come down to a matter of how well your database is optimized... both with respect to your system (memory allowances and such) and indexes. I use a lot of explain queries to test and refine my queries. You really want to make sure that when you run a multi-table query and each table has 30,000+ rows that it isn't checking any more rows than absolutely necessary ;)
-
Pulling The Ten Highest Integers From A Mysql Field
jworisek replied to sdat1333's topic in MySQL Help
I was working under the assumption that it was 10 highest unique integers and all the IDs for those integers. If its 10 integers and you will get more than 10 IDs, go with my code, otherwise go with wildbugs -
you know, it is quite helpful if you actually tell us some information. You know, like what it should do and what it is doing wrong... maybe tell us what error you are getting?
-
have a table that stores users Users [table][tr][td] user_id, username, etc. Items [table][tr][td] item_id, item_name, etc. Combined [table][tr][td] combined_id, user_id, item_id then just link them together. You may want to add additionaly fields to the combined table if you want to say group items that a user entered on a certain day.
-
Pulling The Ten Highest Integers From A Mysql Field
jworisek replied to sdat1333's topic in MySQL Help
is there going to be duplicate values in the column? you can do something like this to get the top ten values: [code] SELECT var FROM table_name ORDER BY var ASC LIMIT 10 [/code] and then you can use another query to grab the ID [code] SELECT var_id FROM table_name where var='$var' [/code] You can do this in one query with subselects but I think its easier to understand if you are new by using multiple queries. -
it would really help if you could post exactly what the error you are getting is. can you access mysql through console? I don't think you need to move the dll's, but you do need to uncomment them in you php.ini file. I have a similiar setup and when I had a hard time getting both mysql.dll and mysqli.dll to function properly together so I use the mysqli exclusively now.
-
well you can use this code immediately after submitting the data... [code] "SELECT last_insert_id() as id_number from table_name"; [/code] and then save that ID in either a hidden form field or a session variable.
-
I think you mean this right? [code] Select title, A.category, order from TableA A INNER JOIN TableB B ON (B.category=A.category) order by title ASC //or it can also be written as: Select title, A.category, order from TableA A, TableB B WHERE A.category=B.category order by title ASC [/code]
-
try this... [code] SELECT * FROM table_name WHERE LENGTH(column_name)<=30; [/code] [!--quoteo(post=389369:date=Jun 29 2006, 01:07 PM:name=shai1)--][div class=\'quotetop\']QUOTE(shai1 @ Jun 29 2006, 01:07 PM) [snapback]389369[/snapback][/div][div class=\'quotemain\'][!--quotec--] Is there a way I can do a select that will return the records with a character count less then a specific number? i.e. select * from table where character count of textField<=30 [/quote]
-
well you could do something like: [code] select id from tablename were col1 like %something% or col2 like %something% or col3........ etc. [/code] I would suggest you rethink your database layout though... If you have to search every single column then you do not have it designed properly.
-
well if you just want to open a new window with a link, you can use: [code] <a href="" target="_blank"></a> [/code] not sure if that will work with what you have.
-
the problem is with : [code] if($logout = true){ $_SESSION['loginid'] = NULL; } [/code] you are setting $logout to true instead of comparing and therefore making the if statement true and setting $_SESSION['loginid'] = NULL... Did you mean to say: [code] if($logout == "true"){ $_SESSION['loginid'] = NULL; } [/code] [!--quoteo(post=387271:date=Jun 23 2006, 01:45 PM:name=jfee1212)--][div class=\'quotetop\']QUOTE(jfee1212 @ Jun 23 2006, 01:45 PM) [snapback]387271[/snapback][/div][div class=\'quotemain\'][!--quotec--] I have this code included in index.php (which is the only accessible file... it includes all of the other pages) [code]<?php session_start(); if(!$validation){ die('You do not have the proper privileges to view this page'); } $mysql_user = "XXXXX"; $mysql_password = "XXXXXX"; $mysql_server = "XXXXXX"; $mysql_db = "XXXXXX"; $connect = mysql_connect($mysql_server, $mysql_user, $mysql_password); $select = mysql_select_db($mysql_db); if($logout = true){ $_SESSION['loginid'] = NULL; } if($_POST['login']){ if($_POST['username'] && $_POST['password']){ $username = stripslashes($_POST['username']); $password = stripslashes($_POST['password']); $userid = mysql_query("SELECT userid FROM users WHERE username='$username' AND password=PASSWORD('".$password."')"); $valid = mysql_num_rows($userid); $login_userid = mysql_fetch_array($userid); if($valid==1){ $userid = $userid[0]; $_SESSION['loginid'] = $login_userid[0]; }else{ echo 'Incorrect login info'; } }else{ echo 'You did not fill in the fields'; } } if(isset($_SESSION['loginid'])){ $user_loginid = $_SESSION['loginid']; $login_get_realname = mysql_query("SELECT realname FROM users WHERE userid='$user_loginid'"); $login_get_username = mysql_query("SELECT username FROM users WHERE userid='$user_loginid'"); $user_realname = mysql_fetch_array($login_get_realname); $user_username = mysql_fetch_array($login_get_username); $user_realname = $user_realname[0]; $user_username = $user_username[0]; $user_logged_in = true; } $home = "users"; ?>[/code] I use $user_logged_in on other pages to verify that the session is there and validated, however when i echo $_SESSION['loginid'] it returns null. When you first log on, the "Welcome..." iinfo is displayed properly, but when you click on a link it loses the logged in status. The link is [a href=\"http://www.jafsitedesign.com/collegeartstore\" target=\"_blank\"]http://www.jafsitedesign.com/collegeartstore[/a] Thanks a bunch [/quote]
-
so that code is everything on your addevent.php page? I tried that same code on my server and it worked fine. [!--quoteo(post=387260:date=Jun 23 2006, 01:02 PM:name=DaveLinger)--][div class=\'quotetop\']QUOTE(DaveLinger @ Jun 23 2006, 01:02 PM) [snapback]387260[/snapback][/div][div class=\'quotemain\'][!--quotec--] well, here's my code: [code] <?php session_start(); if(!isset($_SESSION['loggedIn']) || !$_SESSION['loggedIn'] == 1) { die("Please login you are not authorised to access this page"); } ?> <html> <head> <title>PCritics.com</title> <?php include('../../header.php'); ?> //the rest of my code here[/code] and when I access the file directly, it acts like before, like the code isnt there, lets me right in without starting the session =/ actually scratch that it works, but how do I close the session when they're done? [/quote]
-
you must have session_start before any headers are sent... place it at the very start of your script
-
try something like this: heres a quote from PHP Cookbook [!--quoteo--][div class=\'quotetop\']QUOTE[/div][div class=\'quotemain\'][!--quotec--] Also, unless specified all values being passed into and out of a function are passed by value, not reference. This means PHP makes a copy of the value and provides you with that copy to access and manipulate. Therefore, any changes you make to your copy don't alter the original value. [/quote] so unless you specifically return the values, only copies are modified then lost when not returned from the function. [code] /*$pages = array();*/ /* No need to declare it in php */ function addMenuHeader($name) { $pages[] = array($name); return $pages; /* need the return to get the variables out of the function */ } function addMenuItem($name,$pages) { /* need to pass it the pages variable for it to use it. */ $n = sizeof($pages); $pages[$n-1][] = $name; return $pages; } [/code]
-
[code]<?php session_start(); if(!isset($_SESSION['loggedIn']) || !$_SESSION['loggedIn'] == 1) { die("Please login you are not authorised to access this page"); } // rest of code here [/code] missing a paren after SESSION['loggedIn']
-
using javascript in a php file to update menus based on selections
jworisek replied to jworisek's topic in Javascript Help
that should be able to work... the problem is that I don't really have a clue how to adapt that script to use ids instead of names. Can you give me a hand with that? [!--quoteo(post=387215:date=Jun 23 2006, 11:20 AM:name=nogray)--][div class=\'quotetop\']QUOTE(nogray @ Jun 23 2006, 11:20 AM) [snapback]387215[/snapback][/div][div class=\'quotemain\'][!--quotec--] You can give the select an ID and access the select object using that [code] <select name="product_id[]" id="product_id"> [/code] Javascript will be like [code] document.getElementById('product_id') [/code] [/quote] -
this may sound fairly simple, but why don't you just ADD the http:// after words? you can have an input something like: [code] http:// <input type="text" name="url" /> // or simply just put http:// already in the input <input type="text" name="url" value="http://" /> [/code] It just seems kinda silly and probably very frustrating for the users to have to put the http:// in themselves. [!--quoteo(post=387208:date=Jun 23 2006, 11:04 AM:name=lpxxfaintxx)--][div class=\'quotetop\']QUOTE(lpxxfaintxx @ Jun 23 2006, 11:04 AM) [snapback]387208[/snapback][/div][div class=\'quotemain\'][!--quotec--] Hello, As I am close to launching my tutorial site, I almost forgot something very important. Form Validation. I got most of them to work, except for one major one, the URL. I want the user to enter the url with "http://" in the front. Unfortunately, a lot of users were submitting URL's with "www." in front, instead of "http://." That is bad, because it would then link to "www.mysite.com/page.phpwww.tutorialurl.com/tut1.php" etc. So far, I tried to do the following: if (!eregi ('^((http|https|ftp)://)?([[:alnum:]\-\.])+(\.)([[:alnum:]]){2,4}([[:alnum:]/+=%&_\.~?\-]*)$', stripslashes(trim($_POST['url'])))) { $problem = TRUE; $message .= '<p>Please enter a valid URL.</p>'; } It did not work for the "http://" part, but it did work for the ".com" part.. Does anyone know a way to require a "http://" in front of the post? Thanks [/quote]
-
I have a form where the user inputs multiple line items at once and I am currently passing this to the next page using: [code] <select name="blah[]"><option value="">option #1</option>.....</select> [/code] so its it a nice array. I have about 8 inputs per line item so this makes it easy to loop through everything and reconstruct it after submitting since I can use the same index in each option per line item. What I would like to do though is to be able to dynamically update one based on another. for example, lets say we have products and sizes Product A: Sizes (Small, Medium, Xlarge) Product B: Sizes (Xsmall, Large) Product C: Sizes (Xsmall, Medium, Large, Xlarge) So I want to have the user select the product and then dynamically update the sizes dropdown menu to only the applicable values. I can do this with javascript for one line item, but it fails when I try to use the [] to put the data into arrays. Does anyone have any experience in this sort of thing and have suggestions? I left a message in the javascript forums but no one has posted yet.
-
I'll say up front that I am a bit of an idiot when it comes to javascript... With that out of the way, I already have a script that updates on dropdown menu based on the users input for a different menu on the same page. Here is what I want to do: lets say we have products and sizes Product A: Sizes (Small, Medium, Xlarge) Product B: Sizes (Xsmall, Large) Product C: Sizes (Xsmall, Medium, Large, Xlarge) right now, in php I place all the values into an array for submitting to the next page [code] <select name="product_id[]"><option value=""></option>....</select> [/code] The base code that I am currently using is this (NOTE: I build the arrays in php beforehand): [code]<script LANGUAGE="JavaScript"> <!-- Original: Jerome Caron ([email protected]) --> <!-- This script and many more are available free online at --> <!-- The JavaScript Source!! http://javascript.internet.com --> colors = new Array( <? echo $color_array; ?> ); function fillSelectFromArray(selectCtrl, itemArray, goodPrompt, badPrompt, defaultItem) { var i, j; var prompt; // empty existing items for (i = selectCtrl.options.length; i >= 0; i--) { selectCtrl.options[i] = null; } prompt = (itemArray != null) ? goodPrompt : badPrompt; if (prompt == null) { j = 0; } else { selectCtrl.options[0] = new Option(prompt); j = 1; } if (itemArray != null) { // add new items for (i = 0; i < itemArray.length; i++) { selectCtrl.options[j] = new Option(itemArray[i][0]); if (itemArray[i][1] != null) { selectCtrl.options[j].value = itemArray[i][1]; } j++; } // select first item (prompt) for sub list selectCtrl.options[0].selected = true; } } // End --> </script>[/code] [code]<form method="POST" action="two.php" name="Main"> <h1>New Order</h1> <h2>Process</h2> <SELECT NAME="process_id" onChange="fillSelectFromArray(this.form.color_id, ((this.selectedIndex == -1) ? null : colors[this.selectedIndex-1]));"> <OPTION VALUE="-1">Select Process</option> <? echo $process_list; ?> </SELECT> <h2>Enhancement</h2> <SELECT NAME="color_id"> <option value="0">Select Enhancement</option> </SELECT> <input type="submit" value="submit" name="submit" /> </form>[/code] In this example, changing the process updates the color list to only colors associated with that process. This is exactly like what I want to do with the products/sizes but the product/sizes has multiple line items and has to be passed as an array. When I try to work the hard brackets into the name being called with this script it simply ignores it. Any ideas?
-
In my code the most I would have is 7 iterations whereas yours will always have 70 iterations... The one I posted was different from my first thought where I might have to go back through and take out the weekends afterwords. Instead I figured it was easiest just to step through one day at a time and ignore weekends as I go. I could be wrong but that seems a lot more efficient than always pulling the max amount of days to check.
-
well I have something like: [code] <? $today=mktime(); //todays date in seconds $one_day=24*60*60; //one day in seconds $target_days=5; //5 work days $days_used=0; while ($days_used<$target_days){ $day=date("w",$today); if (($day==0)||($day==6)){ $today=$today+$one_day; } else{ $today=$today+$one_day; $days_used++; } } ?> [/code] It will work, but I'm sure there is a more efficient way.
-
I'm looking into a way to computerize deadlines for our orders. At the moment a user manually enters the date by checking a cheat sheet and using a calendar to figure out when the deadline is. My problem is that I only want to use weekdays in the calculation. For instance we might get an order we can ship in 3 days.. if we get it on a friday, I want to be able to say we will ship on the following wednesday. Some may have up to 2 month deadlines. At the moment all I can think of is doing a rough calculation including weekends then going back through and taking out the weekends and adding that time back on... Although that will get tricky as well since I will have to loop through that possibly 3 times. Anybody have any ideas on an easier way?
-
that page does exactly what you programmed it to... nowhere in your programming is a call to check inside the given 'series'. You should put code for checking the series hits inside an if... [code] if ($_GET[series]!=""){ /* Note you should actually test what is given against actual values to verify that a user didn't make up a series to gain access to your query.... */ $sql= select ..... from layouts where series='$_GET[series]'"; ...etc } else{} [/code]
-
[!--quoteo(post=378495:date=May 30 2006, 03:01 PM:name=snapper64)--][div class=\'quotetop\']QUOTE(snapper64 @ May 30 2006, 03:01 PM) [snapback]378495[/snapback][/div][div class=\'quotemain\'][!--quotec--] [code] $sql = 'UPDATE `users`' . ' SET `status` = `1` . ' WHERE `password` = `$md5`' AND `timestamp` = `$base`; [/code] [/quote] I could be wrong, but that doesn't look right... try this: [code] $sql ="UPDATE users SET status = '1' WHERE password = '$md5' AND timestamp = '$base'"; [/code]