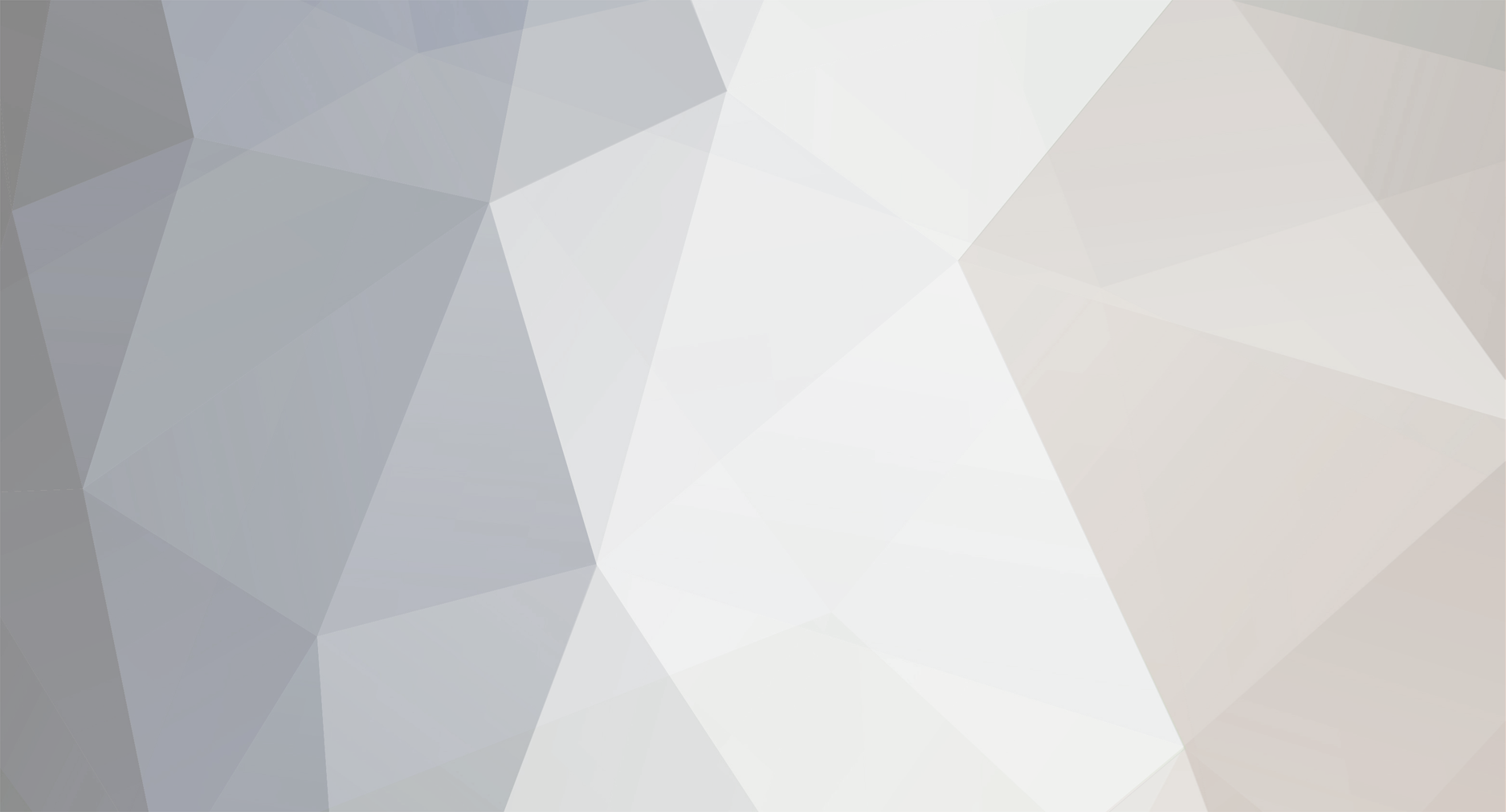
NotionCommotion
Members-
Posts
2,446 -
Joined
-
Last visited
-
Days Won
10
Everything posted by NotionCommotion
-
Matching day of week and time of day
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Well, turns out the FDA will be stopping by later today to audit our 21 CFR Part 11 drug production facility and ... Just kidding, nothing unethical or illegal. -
I wish to find the closest two DateTimes which are within $fillStart and $fillEnd and have the same week and 24 hour times as $gapStart and $gapEnd. For instance, the following results in $fillStartModified and $fillEndModified which meets that criteria. fillStart 2019-07-23 00:15:00 Tue fillEnd 2019-09-23 13:00:00 Mon gapStart 2019-05-23 00:15:00 Thu gapEnd 2019-06-23 13:00:00 Sun fillStartModified 2019-07-25 00:15:00 Thu fillEndModified 2019-08-25 13:00:00 Sun I came up with the following which seems to work, but it is kind of complicated and I am not positive it will meet all edge conditions. Any recommendations? Thanks function getFill(\DateTimeImmutable $fillStart, \DateTimeImmutable $fillEnd, \DateTimeInterface $gapStart, \DateTimeInterface $gapEnd) { //$gapInterval = $gapStart->diff($gapEnd); // Doesn't work $gapInterval = new \DateInterval('PT'.($gapEnd->getTimestamp() - $gapStart->getTimestamp()).'S'); if($gapStart > $fillStart) { //The fill is older than the gap so make the fill's endTime match the gap's endTime $fillEndModified = $fillEnd ->modify('previous '.$gapEnd->format('D')) ->setTime(...explode('.', $gapEnd->format('H.i.s.u'))); if($fillEndModified->diff($fillEnd)->format('%a') >= 7) { $fillEndModified = $fillEndModified->add(new \DateInterval('P7D')); } $fillStartModified = $fillEndModified->sub($gapInterval); if($fillStartModified < $fillStart) { $fillStartModified=null; $fillEndModified=null; } } else { //The fill is newer than the gap so make the fill's startTime match the gap's startTime $fillStartModified = $fillStart ->modify('next '.$gapStart->format('D')) ->setTime(...explode('.', $gapStart->format('H.i.s.u'))); if($fillStart->diff($fillStartModified)->format('%a') >= 7) { $fillStartModified = $fillStartModified->sub(new \DateInterval('P7D')); } $fillEndModified = $fillStartModified->add($gapInterval); if($fillEndModified > $fillEnd) { $fillStartModified=null; $fillEndModified=null; } } return [$fillStartModified, $fillEndModified]; }
-
Steven, See below. Also, you probably want to make a HTTP POST request instead of GET. if(isset($_GET['post'])){ // bla bla bla $row=mysqli_fetch_array($result); } ?> <!-- What if $_GET['post'] wasn't set? Will $row be defined? --> <content> <?php echo $row['Website']?> <?php echo $row['UcasPointsRequired']?> <?php echo $row['OverallScore']?> </content>
-
How to compare two DateIntervals
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Agree that some DateIntervals' duration are a function of time and therefore the difference between two is as well, and it is kind of misleading if PHP reported the value. That being said, some times it is good information to have. How long is a month or a year? Easy enough, however, to add my own method to estimate it, so not a problem. Do you know whether I was correct and that PHP once implemented the ability to use comparison operators on DateIntervals? Not that I want to do so, and just wondering whether I just imagined it. -
How to compare two DateIntervals
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
I am pretty sure I was able to use comparison operators on a DateInterval using some previous PHP version, but can no longer do so with PHP7.4. Probably best to always add to some date, and will do so. Related https://bugs.php.net/bug.php?id=49914 -
I thought I was able to determine which DateInterval is greater the same as done with DateTime, however, either this is not true or I am doing something wrong. Please advise. Thanks! private function compareDateInterval(\DateInterval $dateInterval1, \DateInterval $dateInterval2):bool { $greaterThan = $dateInterval1 > $dateInterval2?'true':'false'; echo("test1: dateInterval1 > dateInterval2: $greaterThan\n"); $dateTimeNow = new \DateTimeImmutable; $dateTime1 = $dateTimeNow->add($dateInterval1); $dateTime2 = $dateTimeNow->add($dateInterval2); $greaterThan = $dateTime1 > $dateTime2?'true':'false'; echo("test2: dateTime1 > dateTime2: $greaterThan\n"); $timestampNow = $dateTimeNow->getTimestamp(); $timestamp1 = $dateTime1->getTimestamp(); $timestamp2 = $dateTime2->getTimestamp(); $greaterThan = $timestamp1 > $timestamp2?'true':'false'; echo("test3: timestamp1 > timestamp2: $greaterThan\n"); echo('$dateTimeNow => '.json_encode($dateTimeNow).PHP_EOL); echo('$dateInterval1 => '.json_encode($dateInterval1).PHP_EOL); echo('$dateInterval2 => '.json_encode($dateInterval2).PHP_EOL); echo("timestampNow: $timestampNow, timestamp1: $timestamp1, timestamp2: $timestamp2\n"); } <b>Warning</b>: Cannot compare DateInterval objects in <b>/var/www/app/src/Tools/PointTransitionHistory.php</b> on line <b>136</b><br /> test1: dateInterval1 > dateInterval2: false test2: dateTime1 > dateTime2: true test3: timestamp1 > timestamp2: true $dateTimeNow => {"date":"2020-08-23 15:06:24.454702","timezone_type":3,"timezone":"UTC"} $dateInterval1 => {"y":0,"m":0,"d":2,"h":14,"i":0,"s":0,"f":0,"weekday":0,"weekday_behavior":0,"first_last_day_of":0,"invert":1,"days":2,"special_type":0,"special_amount":0,"have_weekday_relative":0,"have_special_relative":0} $dateInterval2 => {"y":0,"m":0,"d":11,"h":15,"i":0,"s":0,"f":0,"weekday":0,"weekday_behavior":0,"first_last_day_of":0,"invert":1,"days":11,"special_type":0,"special_amount":0,"have_weekday_relative":0,"have_special_relative":0} timestampNow: 1598195184, timestamp1: 1597971984, timestamp2: 1597190784
-
Thanks gw1500se, Before posting this question, I did look at several sources such as https://www.timeanddate.com/time/zones/z which described it as military time. Both your and my reference state that it is the same as UTC, but as seen by the output of my initial script, they have different timezone types and I didn't know what implications that might have.
-
What are the differences and implications of UTC time and Zulu time? <?php function getArr(string $time):array { $dateTime = new \DateTime($time); return [ 'time'=>$time, 'timestamp'=> $dateTime->getTimestamp(), 'dateTime' => $dateTime ]; } $arr = getArr('2020-08-05'); $arr_z = getArr('2020-08-05T00:00:00Z'); print_r($arr); print_r($arr_z); echo('equal timestamps: '.($arr['timestamp'] === $arr['timestamp']?'true':'false')); Array ( [time] => 2020-08-05 [timestamp] => 1596585600 [dateTime] => DateTime Object ( [date] => 2020-08-05 00:00:00.000000 [timezone_type] => 3 [timezone] => UTC ) ) Array ( [time] => 2020-08-05T00:00:00Z [timestamp] => 1596585600 [dateTime] => DateTime Object ( [date] => 2020-08-05 00:00:00.000000 [timezone_type] => 2 [timezone] => Z ) ) equal timestamps: true
-
Persisting an object over multiple requests
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Thanks requnix, All your replies makes sense. -
Persisting an object over multiple requests
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Okay, I see how a redis or memcached server can be used instead of saving content in a DB or flat file. Appears that for new projects, redis is likely preferred. There was much content available describing how to set it up and storing strings, etc in it, but not much regarding persisting an PHP object with methods. Appears that I will likely want to create a second class which will be injected with the serialized JSON object and implements a common interface to the original creator, true? Any thoughts on how to best spawn a new process. Is http://gearman.org/ worth considering for the forking of a PHP process, or maybe some other application is more relevant? Or maybe just run it in the background and have it write to redis/memcached when complete? Looks like both redis and memcached require some unique key to identify the content. Any concerns with using the web client's session cookie string? Does my client polling strategy make sense to determine whether the object is available make sense? Thanks! -
Persisting an object over multiple requests
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Thanks, will check it out. -
I have an object which is very expensive to create, and is fairly large but by no means enormous. The object has two tasks: Display to the user what can be changed in a database. Make some or all of those changes based on user input. Instead of creating it first to perform the first task, I would like to serialize it and store it somewhere and then restore it to perform the second task to reduce user wait time. Communication of both tasks is as follows where the web client first makes a XMLHttpRequest and then cURL is used for the remaining: Web Client -> Web Server -> REST API Server -> Time Historian Application (and then back in the same order) In addition, both of these tasks take significantly longer than 30 seconds resulting in cURL error 28. I certainly can investigate to determine which of the requests are causing this error, however, feel that the solution to persisting the object might solve this issue as well. I am thinking of making the REST API server responsible for temporarily storing the object, and am considering the following: Web client makes XMLHttpRequest to web server and passes session cookie. Web server makes cURL request to REST API server and passes that same cookie (maybe a bad idea?). REST API server initiates the time historian application, spawns some new process, and replies to the web server maybe with some expected wait time duration, and web server in turn responds to web client. Spanned process when object is complete serializes the object's content and saves it as JSON using the session cookie as the filename. Web client periodically makes requests to web server which in turn make requests to REST API server and when the JSON file is available, recreates the object, executes the applicable method, and replies with the applicable content. Web client sends user data to the web server and in turn to the REST server to initiate the second task. Web client similarly periodically makes requests to web server which in turn make requests to REST API server to check if complete and if so the JSON object file is deleted. If request has not been fulfilled within 24 hours or so, JSON object file is deleted. I would appreciate any general feedback or recommendations how best to accomplish this, and whether using some 3rd party framework such as Gearman, ReactPHP, redis, etc might simplify matters. Thank you
-
Feeding MultipleIterator a generator
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Apr 28 15:00:51 stage kernel: php-fpm[29337]: segfault at 60600000001 ip 00007fd51efcd270 sp 00007ffc86605580 error 6 in dbg-php-7.0.so[7fd51efb5000+2c000] Based on the above, it was the debugger that crashed and not PHP, true? New debugger firmware cured the issue. Thanks -
Feeding MultipleIterator a generator
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
No watches/conditions. For the script I posted, it happens the second time $iterator = new \MultipleIterator(\MultipleIterator::MIT_NEED_ALL|\MultipleIterator::MIT_KEYS_ASSOC); is called. But for the below script, it happens on the second generator on the foreach($iterator as $v) line. <?php ini_set('display_errors', 1); class A { public function test($iterator1, $iterator2) { $iterator = new \MultipleIterator(\MultipleIterator::MIT_NEED_ALL|\MultipleIterator::MIT_KEYS_ASSOC); $iterator->attachIterator($iterator1, 'iterator1'); $iterator->attachIterator($iterator2, 'iterator2'); $values = []; foreach($iterator as $v) { $values[] = $v['iterator2'] - $v['iterator1']; } return $values; } public function getArrayIterator($x) { $a=[]; foreach([0,1,2,3,4] as $i) { $a[]=$x * $i; } return new ArrayIterator($a);; } public function getGeneratorIterator($x) { for($i=0; $i < 5; $i++) { yield $x*$i; } } } $a1 = new A(); $a2 = new A(); $o=$a1->test($a1->getArrayIterator(1), $a1->getArrayIterator(2)); print_r($o); $o=$a1->test($a1->getArrayIterator(3), $a1->getArrayIterator(4)); print_r($o); $o=$a2->test($a2->getArrayIterator(1), $a2->getArrayIterator(2)); print_r($o); $o=$a2->test($a2->getArrayIterator(3), $a2->getArrayIterator(4)); print_r($o); $o=$a1->test($a1->getGeneratorIterator(1), $a1->getGeneratorIterator(2)); print_r($o); //Script gets where without segfault $o=$a2->test($a2->getGeneratorIterator(1), $a2->getGeneratorIterator(2)); print_r($o); -
The following script causes a segfault during the second $a2->test() pass. I then disabled my debugger (phped) and it works as desired. If I replace the generator with an ArrayIterator, I don't get the errors even with the debugger enabled. Is there something wrong with my script or is there something wrong with the debugger? Thanks <?php ini_set('display_errors', 1); class A { public function test() { $iterator = new \MultipleIterator(\MultipleIterator::MIT_NEED_ALL|\MultipleIterator::MIT_KEYS_ASSOC); $iterator1 = $this->getIterator(1); $iterator2 = $this->getIterator(2); $iterator->attachIterator($iterator1, 'iterator1'); $iterator->attachIterator($iterator2, 'iterator2'); $values = []; foreach($iterator as $v) { $values[] = $v['iterator2'] - $v['iterator1']; } return $values; } public function getIterator($x) { for($index=0; $index < 10; $index++) { yield $x*$index; } } } $a1 = new A(); $o1=$a1->test(); print_r($o1); $a2 = new A(); $o2=$a2->test(); print_r($o2);
-
Is there anything different between a response and a responder? My thought is maybe a response is something that one application gives to another, and a responder is used only by a single application to create a response. interface TrendInterface { public function getResponse($blabla):TrendResponse; public function getResponder($blabla):TrendResponder; }
-
Retreive float from PDO and PostgreSQL
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Thanks requinix, You mean one of these two solutions, right? Oh, never mind, I was confused by "Or instead of fighting the language you can let PHP cast them as needed" and thought doing so was one of the two versions of casting. Off topic. I used to always let PHP do what it thought was right regarding casting but now am going maybe too extreme the other way. Do you have any rules of thumb regarding type declaring primitive variables? $string='123.4'; $float=floatval($string); $float=(float)$string; -
The following returns strings instead of floats. Am I able to retrieve floats directly from PDO and/or PostgreSQL or must I manually type cast them afterwards using PHP? Thanks $sql='WITH RECURSIVE t AS (bla bla bla) SELECT id, CAST(SUM(slope*value+intercept) AS FLOAT) "value", SUM(slope*prev_value+intercept)::FLOAT "prevValue" FROM t WHERE type=\'physical\' GROUP BY id'; $stmt = $this->pdo->prepare($sql); $stmt->execute($ids); $arr = $stmt->fetchAll(\PDO::FETCH_UNIQUE); //returns [123=>['value'=>'123.456', 'prevValue'=>'122.234'], ...]
-
Changing array keys using another array
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Are you assuming starting with the $keys and $arrayValues in my original post? $arr1 = array_fill_keys($keys, []); echo(json_encode($arr1).PHP_EOL); $arr2 = array_fill_keys($keys, $arrayValues); echo(json_encode($arr2).PHP_EOL); $values = array_map(function($values) use($keys, $arr1, $arr2) { //$arr=array_combine($arr1, $values); //Array to string conversion //$arr=array_combine($arr2, $values); //Array to string conversion $arr=array_combine($keys, $values); return $arr; }, $arrayValues); echo(json_encode($values).PHP_EOL); { "time": [], "system1_typeA": [], "system2_typeA": [], "system1_typeB": [], "system3_typeC": [], "system2_typeB": [], "system1_typeC": [] } { "time": [["2020-06-28 08:46:34", 1.71, 6.29, 6.32, 5.55, 1.25, 8.81], ["2020-06-28 08:47:45", 1.88, 8.57, 8.62, 9.98, 8.33, 8.89], ["2020-06-28 08:48:10", 5.75, 4.36, 3.54, 7.93, 8.13, 1.43], ["2020-06-28 08:49:15", 7.14, 9.54, 5.39, 4.58, 5.77, 4.72], ["2020-06-28 08:50:00", 9.42, 7.44, 1.47, 1.87, 1.23, 4.79]], "system1_typeA": [["2020-06-28 08:46:34", 1.71, 6.29, 6.32, 5.55, 1.25, 8.81], ["2020-06-28 08:47:45", 1.88, 8.57, 8.62, 9.98, 8.33, 8.89], ["2020-06-28 08:48:10", 5.75, 4.36, 3.54, 7.93, 8.13, 1.43], ["2020-06-28 08:49:15", 7.14, 9.54, 5.39, 4.58, 5.77, 4.72], ["2020-06-28 08:50:00", 9.42, 7.44, 1.47, 1.87, 1.23, 4.79]], "system2_typeA": [["2020-06-28 08:46:34", 1.71, 6.29, 6.32, 5.55, 1.25, 8.81], ["2020-06-28 08:47:45", 1.88, 8.57, 8.62, 9.98, 8.33, 8.89], ["2020-06-28 08:48:10", 5.75, 4.36, 3.54, 7.93, 8.13, 1.43], ["2020-06-28 08:49:15", 7.14, 9.54, 5.39, 4.58, 5.77, 4.72], ["2020-06-28 08:50:00", 9.42, 7.44, 1.47, 1.87, 1.23, 4.79]], "system1_typeB": [["2020-06-28 08:46:34", 1.71, 6.29, 6.32, 5.55, 1.25, 8.81], ["2020-06-28 08:47:45", 1.88, 8.57, 8.62, 9.98, 8.33, 8.89], ["2020-06-28 08:48:10", 5.75, 4.36, 3.54, 7.93, 8.13, 1.43], ["2020-06-28 08:49:15", 7.14, 9.54, 5.39, 4.58, 5.77, 4.72], ["2020-06-28 08:50:00", 9.42, 7.44, 1.47, 1.87, 1.23, 4.79]], "system3_typeC": [["2020-06-28 08:46:34", 1.71, 6.29, 6.32, 5.55, 1.25, 8.81], ["2020-06-28 08:47:45", 1.88, 8.57, 8.62, 9.98, 8.33, 8.89], ["2020-06-28 08:48:10", 5.75, 4.36, 3.54, 7.93, 8.13, 1.43], ["2020-06-28 08:49:15", 7.14, 9.54, 5.39, 4.58, 5.77, 4.72], ["2020-06-28 08:50:00", 9.42, 7.44, 1.47, 1.87, 1.23, 4.79]], "system2_typeB": [["2020-06-28 08:46:34", 1.71, 6.29, 6.32, 5.55, 1.25, 8.81], ["2020-06-28 08:47:45", 1.88, 8.57, 8.62, 9.98, 8.33, 8.89], ["2020-06-28 08:48:10", 5.75, 4.36, 3.54, 7.93, 8.13, 1.43], ["2020-06-28 08:49:15", 7.14, 9.54, 5.39, 4.58, 5.77, 4.72], ["2020-06-28 08:50:00", 9.42, 7.44, 1.47, 1.87, 1.23, 4.79]], "system1_typeC": [["2020-06-28 08:46:34", 1.71, 6.29, 6.32, 5.55, 1.25, 8.81], ["2020-06-28 08:47:45", 1.88, 8.57, 8.62, 9.98, 8.33, 8.89], ["2020-06-28 08:48:10", 5.75, 4.36, 3.54, 7.93, 8.13, 1.43], ["2020-06-28 08:49:15", 7.14, 9.54, 5.39, 4.58, 5.77, 4.72], ["2020-06-28 08:50:00", 9.42, 7.44, 1.47, 1.87, 1.23, 4.79]] } [{ "time": "2020-06-28 08:46:34", "system1_typeA": 1.71, "system2_typeA": 6.29, "system1_typeB": 6.32, "system3_typeC": 5.55, "system2_typeB": 1.25, "system1_typeC": 8.81 }, { "time": "2020-06-28 08:47:45", "system1_typeA": 1.88, "system2_typeA": 8.57, "system1_typeB": 8.62, "system3_typeC": 9.98, "system2_typeB": 8.33, "system1_typeC": 8.89 }, { "time": "2020-06-28 08:48:10", "system1_typeA": 5.75, "system2_typeA": 4.36, "system1_typeB": 3.54, "system3_typeC": 7.93, "system2_typeB": 8.13, "system1_typeC": 1.43 }, { "time": "2020-06-28 08:49:15", "system1_typeA": 7.14, "system2_typeA": 9.54, "system1_typeB": 5.39, "system3_typeC": 4.58, "system2_typeB": 5.77, "system1_typeC": 4.72 }, { "time": "2020-06-28 08:50:00", "system1_typeA": 9.42, "system2_typeA": 7.44, "system1_typeB": 1.47, "system3_typeC": 1.87, "system2_typeB": 1.23, "system1_typeC": 4.79 } ] -
Are you sure you are configured to display errors? Consider adding the following at the top of the page. error_reporting(E_ALL); ini_set('display_startup_errors', 1); ini_set('display_errors', 1); You also have calls to a bunch of functions which are not declared in your script. Are they being imported elsewhere? When I get stuck, I put little traps in the code (either echo, exit, or syslog) to pinpoint where the error is.
-
Changing array keys using another array
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Is array_map fed the keys and not the arrayValues? $map = array_map(function($v) { return explode('_',$v); }, $keys); Is array_fill_keys being passed an array for the second argument? Maybe another small (or big) hint? -
I have a sequential multidimensional array with many outer rows where all the inner arrays have the same number of elements as another sequential array which represents metadata of the first array's inner arrrays. $keys: ["time","system1_typeA","system2_typeA","system1_typeB","system3_typeC","system2_typeB","system1_typeC"] $arrayValues: [ ["2020-06-27 07:40:06",2.16,9.25,9.44,7.76,5.43,1.12], ["2020-06-27 07:40:23",2.66,8.93,4.31,6.59,8.44,3.42], ["2020-06-27 07:41:24",8.71,2.78,8.92,6.58,3.65,4.38], ["2020-06-27 07:41:58",1.86,6.36,2.65,3.99,7.25,3.32], ["2020-06-27 07:42:33",8.71,3.66,8.82,8.54,8.22,4.51] ] My desired output is: { "time": ["2020-06-27 07:40:06", "2020-06-27 07:40:23", "2020-06-27 07:41:24", "2020-06-27 07:41:58", "2020-06-27 07:42:33"], "system1": { "typeA": [2.16, 2.66, 8.71, 1.86, 8.71], "typeB": [9.44, 4.31, 8.92, 2.65, 8.82], "typeC": [1.12, 3.42, 4.38, 3.32, 4.51] }, "system2": { "typeA": [9.25, 8.93, 2.78, 6.36, 3.66], "typeB": [5.43, 8.44, 3.65, 7.25, 8.22] }, "system3": { "typeC": [7.76, 6.59, 6.58, 3.99, 8.54] } } Is there much faster way to do so than the following? I would like to use a built in function like array_combine() if possible, but grouping by system and type creates an issue. function transform(array $keys, array $arrayValues):array { $map=[]; foreach ($keys as $key) { $map[] = explode('_',$key); } $count=count($keys); $newValues=[]; foreach ($arrayValues as $i=>$arrayValue) { $newValues['time'][]=$arrayValue[0]; for ($i = 1; $i < $count; $i++) { $newValues[$map[$i][0]][$map[$i][1]][]=$arrayValue[$i]; } } return $newValues; }
-
Thanks kicken, Yes, I see why addValue() might take a little time. I know performance optimization is often foolhardy, but think this is a good time to do so. Regarding the my responder question, I definitely don't need to spend any more time dwelling on it and just pick one, and will likely go with the one you suggested.
-
Thanks kicken, Well, that is significant. ColumnData was applied on the short loop so there were few of them which contained large arrays? I would not expect significant difference in time. Guess that is why testing is always good! I know I am a little off topic, but still hope to get your thoughts. Say I had a class RawData which was injected with either a stream or some data array, and needed the ability to export the data in several different formatted versions. Would you any of the following approaches or some other approach? $rawData=new RawData($stream); $outputV1 = $rawData->createOutput(new OneOfServeralOutputFormatters()); $outputV2 = (new OneOfServeralOutputFormatters($rawData))->createOutput(); $outputV3 = (new OneOfServeralOutputFormatters())->createOutput($rawData);