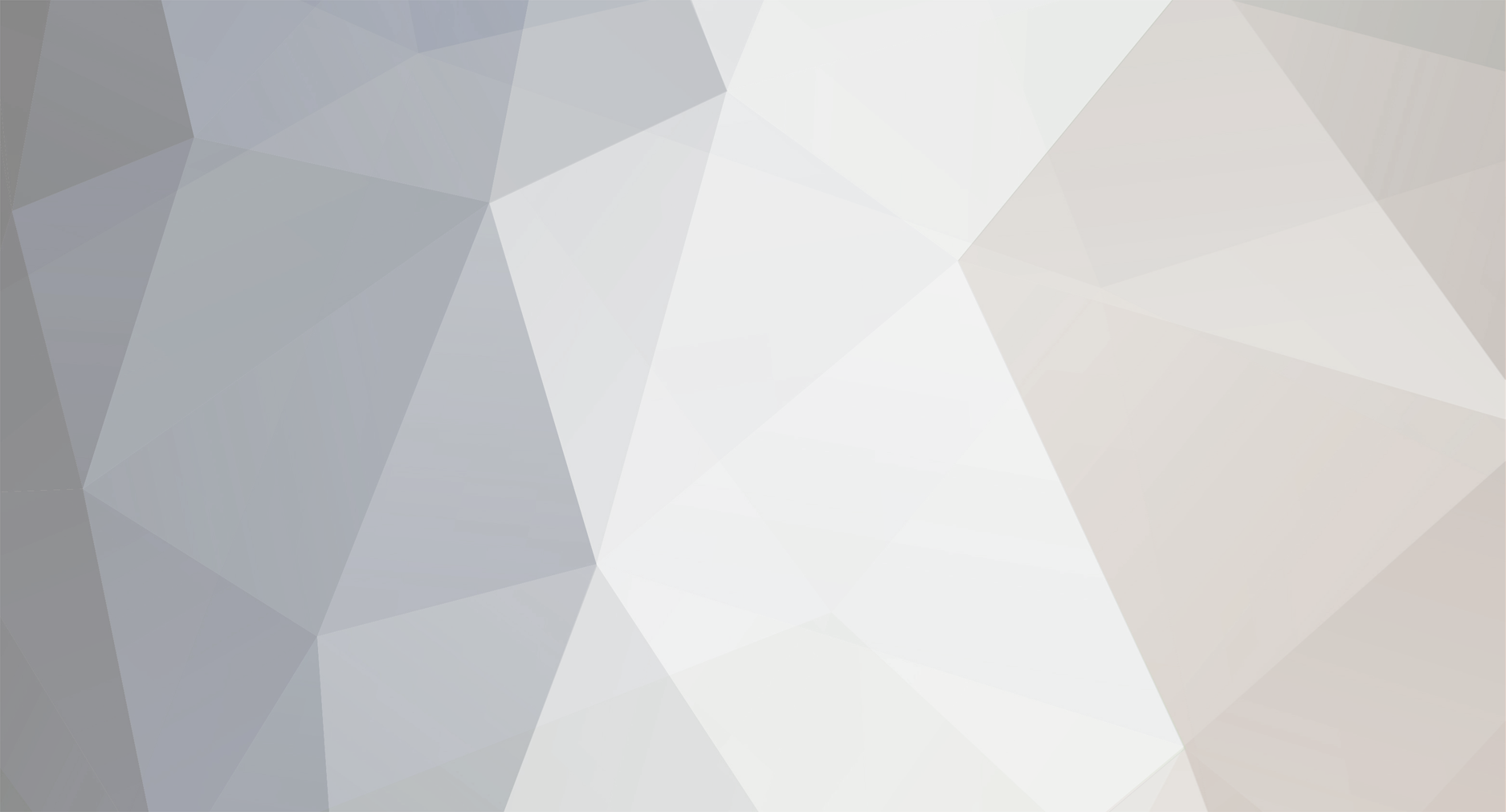
NotionCommotion
Members-
Posts
2,446 -
Joined
-
Last visited
-
Days Won
10
Everything posted by NotionCommotion
-
Good news is usually PHP provides more guidance regarding errors. Bad news is when you are just starting off, you will get a bunch of these. At first, try to keep your echo's small and don't mix single and double quotes. I always "just because" put parenthesis around the stuff I want to echo. Know that a semicolon is "mostly" end of command, and keep an eye out for them. Use a dot (.) to join stuff. <?php $myname = 'Noxin'; echo '<p>This is PHP</p>'. "<p>My name is $myname</p>". '<p>My name in another notation is still '.$myname.'</p>'; ?> PS. See http://php.net/manual/en/language.types.string.php Also, I would probably use the following even though the curly brackets are not required: echo("<p>This is PHP</p><p>My name is {$myname}</p><p>My name in another notation is still {$myname}</p>");
-
Risks of allowing users to upload files to the server
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
What might allow this to happen? You mean not to use the user-provided filename to store it on the server, or not to display this name when someone wants to download it? If the former, why pick an extension at all, and just use a random name without an extension (and revert to the applicable filename with extension when downloaded via headers)? If the later, way? Again, what might make the webserver execute one of these file/scripts? Proper PHP extensions? I don't, but of course use escapeshellarg() and the like. Please explain. -
My server is Linux/Apache/PHP. When a file is uploaded, I use PHP's finfo_open to confirm that the file have the correct file extension matches and delete them if it doesn't match. I also which file mimi types and size could be uploaded. Things I do with the files include: Upload user's files and store them in some public directory (/var/www/html/users_public_directory/), and allow other users to directly download them. Upload user's files and store them in some private directory (/var/www/users_private_directory/), and allow other users to download them using X-Sendfile. Upload user's ZIP files and convert them to PDF files (unzip the ZIP file, and uses Libreoffice and Imagemagick's convert to convert them to PDFs). From the server's prospective, what are the risks of allowing users to upload files? Are there some file types which are more dangerous to the server? Could they be executed on the server, and if so, how could this be prevented?
-
Edit Microsoft Word file on website
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Thaanks kicken, makes sense. -
Edit Microsoft Word file on website
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Maybe Libreoffice? I currently use it headless to convert word/excel/powerpoint to PDF. -
Other than downloading the file, editing it on the local PC, saving it, and uploading it back to the server using some applicable script, any ideas?
-
Various development and configuration tools. Currently, I have a bunch of scripts such as delete the database and rebuild it from scratch. Once in production, I will obviously not want that one! Other scrips are to modify the database to add a new page to the application. Currently, I have the scripts located in a non-public directory, and have a publicly accessible directory protected with an Apache password with contains PHP files with a single require('/var/www/private/some_file.php'). I want to clean it up and have one location to access all of them. Yes, I cold move them out of the web root, but don't want to currently. And I agree it should be robust enough so no one could do anything with it even if they found it, but what if I miss something? Adding a little to hiding it just seemed like an easy way to add some extra insurance.
-
Definitely quick and easy, however, it doesn't hide the page.
-
I have a particular PHP file which is publicly located, however, I don't want anyone but me to access. Below are my thoughts how to do so. Please comment. Use an uncommon name, and definitely not index.php. Either include a file called index.html in the same directory, or set up Apache not to show them using Options -Indexes, or maybe both for good measure. Require some variable to be set to a given value in either the GET or POST array, and if not set, throw a 404 header and display the 404 missing file HTML. If user accesses page and is not logged on as determined by a session value, display a log-on page. Prevent indexing by either putting <meta name="robots" content="none" /> in the HTML, and using header("X-Robots-Tag: noindex, nofollow", true); in the PHP, or maybe both for good measure. Seem reasonable? Anything else? Thanks
-
Detect whether the page was refreshed
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Set it to what? A serialized GET or something? I am not concerned whether they have ever been there, only that it was the last page. -
Detect whether the page was refreshed
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
This seems to work if(($_SERVER['REQUEST_METHOD']==='POST') || !isset($_SERVER['HTTP_CACHE_CONTROL']) || $_SERVER['HTTP_CACHE_CONTROL'] !== 'max-age=0'){ //store } -
Detect whether the page was refreshed
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
I have a class that uses sessions to remember the browsers immediate previous state. I use it to validate when JS is not available (no, I do not validate using JS, but POST the data using Ajax and if no errors, redirect client side to the next page). Upon a non-ajax post request, I validate data, and if an error, store the error and redirect the page back to the form page. Going to another page will clear the history as desired, but I don't wish to clear it if it is just refreshed with F5 or equal. -
How can I detect if the page was refreshed? I thought the following worked, however, after much head scratching, discovered it does not work for a POST request using the Chrome browser. Thanks <?php $refresh=isset($_SERVER['HTTP_CACHE_CONTROL']) && $_SERVER['HTTP_CACHE_CONTROL'] === 'max-age=0'; echo(($refresh?'refresh':'not refresh').'<br>'); echo('$_GET<pre>'.print_r($_GET,1).'</pre>'); echo('$_POST<pre>'.print_r($_POST,1).'</pre>'); ?> <ul> <li><a href="refresh.php?id=1">one</a></li> <li><a href="refresh.php?id=2">two</a></li> <li><a href="refresh.php?id=3">three</a></li> </ul> <form method="post" action="<?php echo $_SERVER['PHP_SELF']; ?>"> <input type="text" name="text" value="hello"> <input type="submit" name="submit" value="submit"> </form>
-
Needles, haystacks, and arrays
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
I am currently not positive. I am sure you know, and while in hindsight it is obvious, array('a','b') does not have equality (and obviously not identity) to array('b','a'). -
What happens if two people submit at the same time?
NotionCommotion replied to greenace92's topic in PHP Coding Help
Is an auto-increment column really necessary? What if a random PK was desired? Wouldn't a unique constraint provide the same results? -
Needles, haystacks, and arrays
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Let me give a working example. Is this really the best way to accomplish this, or is there a better way? <?php function test($map,$array1) { $value=false; foreach($map as $elem) { if(array_diff($array1, $elem['options']) === array_diff($elem['options'], $array1)) { $value=$elem['name']; break; } } return $value; } $map=array( array('name'=>'nothing','options'=>array()), array('name'=>'aaa','options'=>array('a')), array('name'=>'bbb','options'=>array('b')), array('name'=>'ccc','options'=>array('c')), array('name'=>'aaa and bbb','options'=>array('a','b')), array('name'=>'aaa and ccc','options'=>array('a','c')), array('name'=>'bbb and ccc','options'=>array('b','c')), array('name'=>'aaa, bbb, and ccc','options'=>array('a','b','c')) ); echo(test($map,array()).'<br>'); echo(test($map,array('c')).'<br>'); echo(test($map,array('b','c')).'<br>'); echo(test($map,array('a','b','c')).'<br>'); ?> -
Query table and return sub-arrays
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Thanks Barand, but wouldn't this return: Array ( [1] => Array ( [2] => Array ( [0] => 4 [1] => 5 [2] => 8 ) ) ) where I was looking for: Array ( [a] => 1 => 2 [c] => Array ( [0] => 4 [1] => 5 [2] => 8 ) ) -
I have the following mapping array: Given array(), I would like to return 'nothing', given array('c'), I would like to return 'ccc', given array('b','c'), I would like to return 'bbb and ccc', given array('a','b','c'), I would like to return 'aaa, bbb, and ccc', etc. How is this best accomplished? As an alternate solution, instead of being given the above arrays, I could be given the following strings "", "c", "b~c", "a~b~c" and I can change the above mapping array to include these strings instead of the arrays I show. Please reference http://forums.phpfreaks.com/topic/292921-query-table-and-return-sub-arrays/ for history. Would this be a better approach? How would I ensure the order of the JOINed values were always in the correct order for my mapping array?
-
Given the following query, SELECT t1.a, t1.b, t2.c FROM t1 INNER JOIN t2 ON t2.id=t1.t2_id WHERE t1.pk=123; I get the following three records: array( array('a'=>1,'b'=>2,'c'=>4), array('a'=>1,'b'=>2,'c'=>5), array('a'=>1,'b'=>2,'c'=> ) What would be the best way to get just one record such as the following? array('a'=>1,'b'=>2, 'c'=>array(4,5,) My thoughts were to use MySQL's GROUP_CONCAT, and then use implode() to turn it into an array, but didn't know if there was a better way. Thanks
-
I am sending emails using PHPMailer. My configuration is below. When adding a link to the message, should I use anchor tags? Both seem to work. What is the implication to recipients who are not using HTML email? On a side note, if anything below seems wrong, please let me know. Thanks $msg='<p>Hello</p><p>Link: http://phpmailer.worxware.com/</p><p>Link: http://phpmailer.worxware.com</p><a href="http://phpmailer.worxware.com">http://phpmailer.worxware.com</a>'; $mail = new myPHPMailer(true); $mail->AddReplyTo('myEmail@xxx.com', 'Michael Reed'); $mail->SetFrom('myEmail@xxx.com', 'Michael Reed'); $mail->AddAddress('johndoe@xxx.com', 'John Doe'); $mail->Subject = "Here is your email"; //AltBody property need not be sent since PHPMailer will create an alternate automatically $mail->MsgHTML($message); return ($mail->Send()); class myPHPMailer extends PHPMailer { public function __construct($allow_exceptions=false){ $this->isSMTP(); $this->SMTPDebug = 0; $this->Host = "smtp.gmail.com"; $this->Port = 587; $this->SMTPSecure='tls'; // sets the prefix to the server. Used for gmail only. $this->SMTPAuth = true; $this->Username = 'xxx'; $this->Password = 'xxx'; } }
-
allowing user choose from multiple rows
NotionCommotion replied to Cryotech's topic in PHP Coding Help
If you want to do it with just PHP, you will need a form. You could put the form tags around each of your submit buttons, but I would recommend putting the form tags around all of them. If you go with the later, then you need each of your input buttons to send something unique. Instead of using an input tag, use a button tag with the submit attribute, and include a unique value for each. When your server gets hit, look at the value and do what you need to do. -
Prevent named sessions form overwriting each other
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
I got rid of changing the session id and having multiple session_starts, and I don't have the out of sequence phenomena. I am curious and hope someone could explain what might have been happening. Note that there is an iframe involved as well, but I don't think it was the cause as it doesn't happen anymore. As an alternative, I came up with the following. Please comment (I have a feeling it will not be received very well). It would have been nice not to have to actually change the global $_SESSION variable, but I didn't know how to decode the session file without using session_decode(), and as far as I know session_decode() doesn't have any options not to change the global $_SESSION variable. Also, I am concerned that the session file prefix (sess_) might change in the future or based on PHP installation configuration. public function getOtherSession($session_id_other) { $session_current=$_SESSION; if($session_other=file_get_contents(session_save_path().'/sess_'.$session_id_other)) { session_decode($session_other); $session_other=$_SESSION; $_SESSION=$session_current; } else {$session_other=false;} return $session_other; } -
Prevent named sessions form overwriting each other
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Well, this is odd. I am still messing around with accessing a session, then getting the session id from another session cookie and accessing that session. It appears that my server is being hit one time more than I would expect. Also, a few things appear to be going out of sequence. I have all these sys_log triggers, and the one at the end is not the last one logged, but one related to where I am accessing another session. I've checked for __destruct and register_shutdown_function causing this, but they are not. Am I going crazy, or can headers or something cause this? -
Reason I wasn't sure is that https://example.com/page1/controller1 is rewritten to https://example.com?page=page1&controller=controller1, so it would seem https://example.com/page1/controller1?var=1&var2=foo would be rewritten to https://example.com?page=page1&controller=controller1?var=1&var2=foo (two question marks) PS. When posting messages on this forum, how do you prevent the URLs from being displayed as links?
-
Prevent named sessions form overwriting each other
NotionCommotion replied to NotionCommotion's topic in PHP Coding Help
Thanks Kicken, Good point about all those headers. Don't know the impact, but suspect it can't be ideal. I'll check out session_handler_class but would like to first pursue different options. Could I (and probably more importantly should I) attempt to access the session file directly? I am just reading and not writing, I know the filename (i.e. the session ID), and will be performing this operation fairly rarely. Or maybe I try doing this differently. My script1 is responsible to set something when the user logs in. Script2 needs to ensure the user logged on under script1, and if so, do something with the users_id. Instead of using sessions, I could have script1 set two cookies (the users_id and some hash), and then script2 could deal with it as needed. The risk factor of script2 getting spoofed is fairly low. Thanks for your help