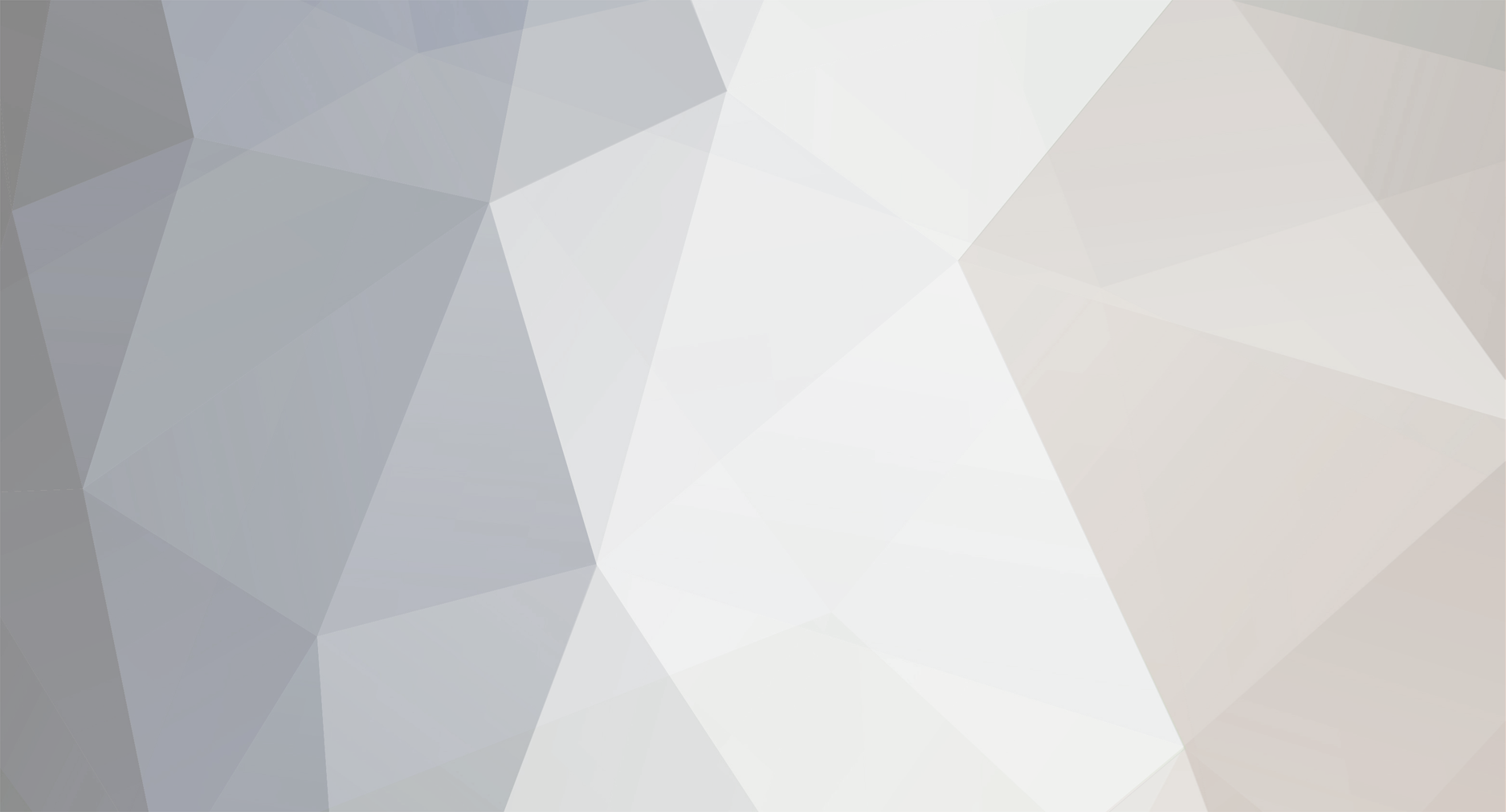
KrisNz
Members-
Posts
271 -
Joined
-
Last visited
Everything posted by KrisNz
-
This just means that you aren't running any mailserver software. The easiest thing to do is to open up php.ini and change the SMTP setting from localhost to your isp's smtp server, i.e smtp.myisp.tld. Then restart apache.
-
1. The name field. 2. CURLOPT_COOKIEJAR should be set to the name of a file where curl can store all cookie information. You also need to set CURLOPT_COOKIEFILE to the same path so it can be retrieved. The login form posts to... https://zarkov.shop.o2.co.uk/login/mblogin Thats the url you want to post to. You need to look at the form and make sure you account for every input in that form - such as 'ACTION', 'dest' and 'fu'. Manual page - look for an example from Michael Sky 3. I hope I just did.
-
Simple 3 code modification needed (random to sequential)
KrisNz replied to djpurpose's topic in PHP Coding Help
Thinking about it, serializing it is overkill for such a simple value, its really more useful for objects and arrays. Here's a really simple example you could expand on... $var = 0; //save value in file file_put_contents("myvar",$var);//this is a php5 function $newvar = file_get_contents("myvar"); //read value out of file $newvar++; echo "$newvar"; //outputs 1 -
Simple 3 code modification needed (random to sequential)
KrisNz replied to djpurpose's topic in PHP Coding Help
I also wrote this which is a bit different to what I described above but works out the same. <?php $usr[] = "bob"; $usr[] = "john"; $usr[] = "lisa"; $usr[] = "roy"; $usr[] = "alice"; $usr[] = "sarah"; $usr[] = "nick"; $usr[] = "berry"; $usr[] = "chuck"; $usr[] = "charlie"; $usr[] = "the very last user"; $thisDir = dirname(__FILE__); define("DB_USR_TRACK_DIR","$thisDir".DIRECTORY_SEPARATOR.'counter'.DIRECTORY_SEPARATOR); //save path for 'tracking' file $size = count($usr); $matches = glob(DB_USR_TRACK_DIR."[0-9]*"); //look for files with a numeric name $new = 0; if (empty($matches)) { touch(DB_USR_TRACK_DIR.$new); //create file } else { $current = basename($matches[0]); //get current index $new = $current + 1;//grab next index if ($new == $size) { //bounds check $new = 0; //restart } rename(DB_USR_TRACK_DIR.$current,DB_USR_TRACK_DIR.$new); //rename current to new } echo $usr[$new]; ?> -
Simple 3 code modification needed (random to sequential)
KrisNz replied to djpurpose's topic in PHP Coding Help
The problem is that a web app is stateless. Your values aren't going to persist between requests. A basic solution would be to 1. set the current user number. 1. serialize() the current_user_number. 2. on the next request, unserialize() the current_user_number. 3. ++current_user_number 4. return to 1 You will of course want to check that incrementing the current_user_number doesn't take you out of the bounds of your array. If it does, reset it 0. Serialize -
take the @ off session_start(). See if you get any error messages.
-
You just need a condition that checks whether the record is 'old' or not and output accordingly... <?php if ($record == 'old') : ?> <option value='Rescheduled'<?PHP echo (@$regstatus == "Rescheduled")?" selected=\"selected\"":""; ?>>Rescheduled <?php endif; ?> There's a disabled attribute for the option tag, but IE doesn't seem to support it. If you wanted to show it without it being selectable, I think you'd need to search for a javascript solution.
-
[SOLVED] call me dumb but can someone please help me out with this
KrisNz replied to kannu's topic in PHP Coding Help
if you just want to see if one string is contained in another string, strpos() is the fastest way. strpos manual page -
[SOLVED] PHP Heredoc Inside A Function - Not Working
KrisNz replied to jadedknight's topic in PHP Coding Help
There mustn't be any white space or any other character for that matter on either side of the end delimiter for a heredoc string. -
[SOLVED] please help... this is really bugging me now
KrisNz replied to joshgarrod's topic in PHP Coding Help
to answer your first question: var_dump(). The reason it doesn't update is because the code sets $welcomeText to be the current value from the database. Your update code uses that same variable instead of reading the new one out of $_POST. -
[SOLVED] please help... this is really bugging me now
KrisNz replied to joshgarrod's topic in PHP Coding Help
this works for me as far as displaying the message, note that I changed the db settings... <?php $usr = "root"; $pwd = ""; $db = "test"; $host = "localhost"; # connect to database $cid = mysql_connect($host,$usr,$pwd); mysql_selectdb($db); if (!$cid) { echo("ERROR: " . mysql_error() . "\n"); } ?> </font></p> <TITLE></TITLE> <P>Update welcome message</P> <font face="Arial, Helvetica, sans-serif"> <?php $query = "SELECT * FROM `Welcome`"; $result = mysql_query($query); var_dump($result); $Title=mysql_result($result,0,"Title"); $WelcomeText=mysql_result($result,0,"WelcomeText"); # this is processed when the form is submitted # back on to this page (POST METHOD) if ($REQUEST_METHOD=="POST") { # double-up apostrophes $Title = str_replace("'","''",$Title); $WelcomeText = str_replace("'","''",$WelcomeText); # setup SQL statement $SQL = "UPDATE `Welcome` SET `Title` = '$Title',`WelcomeText` = '$WelcomeText' WHERE `Welcome`.`ID` =1 ;"; #execute SQL statement $result = mysql_db_query($db,"$SQL",$cid); $ID=mysql_insert_id(); # check for error if (!$result) { echo("ERROR: " . mysql_error() . "\n$SQL\n"); } echo ("<P>Welcome message updated</P>\n"); } ?> </font> <FORM NAME="fa" ACTION="index.php?page=welcomeMessage" METHOD="POST"> <TABLE> <TR> <TD><font face="Arial, Helvetica, sans-serif"><B>Title: </B> </font></TD><TD><font face="Arial, Helvetica, sans-serif"> <INPUT NAME="Title" TYPE="text" id="Title" value="<?php echo $Title; ?>" SIZE=40> </font></TD></TR> <TR> <TD height="96" VALIGN=TOP><font face="Arial, Helvetica, sans-serif"><B>Welcome message : </B> </font></TD><TD><p><font face="Arial, Helvetica, sans-serif"><textarea name="WelcomeText" cols=100 rows=50 id="WelcomeText"><?php echo $WelcomeText; ?></textarea> </font></p> </TD> </TR> <TR> <TH COLSPAN=2><font face="Arial, Helvetica, sans-serif"> <input name="submit" type="submit" value="Update message" /> </font></TH> </TR> </TABLE> <p align="center"> </p> </FORM> <font face="Arial, Helvetica, sans-serif"> <?php mysql_close($cid); ?> -
I mean this <?php class A { public static function myClassName() { return __CLASS__; } } class B extends A { public static function myClassName() { return __CLASS__; } } class C extends B { public static function myClassName() { return __CLASS__; } } ?>
-
[SOLVED] please help... this is really bugging me now
KrisNz replied to joshgarrod's topic in PHP Coding Help
you type 0. I just mean that the variable is pointless. -
[SOLVED] please help... this is really bugging me now
KrisNz replied to joshgarrod's topic in PHP Coding Help
you don't need this loop... while ($i < $num) { $Title=mysql_result($result,$i,"Title"); $WelcomeText=mysql_result($result,$i,"WelcomeText"); } Remove the loop and replace the $i with 0. -
the MyClassName method should technically be marked static if you want to access it without an instance and all child classes will need to override that method.
-
[SOLVED] call to undefined function, gotta be an easy fix
KrisNz replied to php_ninja's topic in PHP Coding Help
class methods are accessed with -> i.e $form_email->display_form_text(); -
modulo - thats the remainder of a division of two numbers.
-
Komodo! Especially 4.x versions where the project stays in sync with the file system, that was a major pain in 3.x versions. The auto-complete is really good and it supports a lot of languages.
-
You're going to need to read all your db rows into an array before you start trying to draw your table because of the way you're displaying your data. This example is pretty basic and inflexible but hopefully it will point you in the right direction. <?php $rows[] = "row 1"; $rows[] = "row 2"; $rows[] = "row 3"; $rows[] = "row 4"; $rows[] = "row 5"; $rows[] = "row 6"; $rows[] = "row 7"; $rows[] = "row 8"; $rows[] = "row 9"; $rows[] = "row 10"; $rows[] = "row 11"; $rows[] = "row 12"; $rows[] = "row 13"; $rows[] = "row 14"; $rows[] = "row 15"; $numColumns = 3; $rowCount = count($rows); $numRows = (int) $rowCount /$numColumns; ?> <table> <tr> <th>col 1</th> <th>col 2</th> <th>col 3</th> </tr> <?php $j = 0; for ($i=0; $i<$numRows ; $i++) : ?> <tr> <td> <?php echo $rows[$j] ?> </td> <td> <?php echo $rows[$j+5] ?> </td> <td> <?php echo $rows[$j+10] ?> </td> </tr> <?php $j++ ; endfor; ?> </table>
-
$dt is an object that cant be converted to a string. you probably want something like echo $dt->format("Y-m-d H:i:s");
-
strip_tags lets you pass a string of allowable tags and then it will only strip ones you don't want. You could then compare the original post to the stripped one and if they were different you'd know the user had submitted dodgy content. Obviously that won't help with the seven words you cant say on tv....
-
No, that would be illogical. If you need the original one in other sections of code, make a copy of the array in the appropriate script and operate on that.
-
Something like <?php $page = "main"; if (isset($_GET['page']) and !empty($_GET['page'])) { $page = $_GET['page']; $page = preg_replace("/[^a-zA-Z0-9_\-]/","_",$page); //remove invalid characters } if (file_exists("/path/to/my/$page.htm")) { //check that the file lives on my server in the folder I expect it to include_once("/path/to/my/$page.htm"); } else { include_once("/path/to/my/404.htm"); //show a "page not found" } ?> Basically I'm saying that when you're dealing with data that you don't have complete control over, it's good practice to check that said data is what you expect it to be.
-
You need to retrieve the value of page from the $_GET array. e.g <?php //create a default value $page = "main"; if (isset($_GET['page']) and !empty($_GET['page'])) { $page = $_GET['page']; } ?> Note that the code I've shown is blindly trusting the user which you should never do. I could potentially open another website inside your one, depending on how php is configured. You'll need to do some appropriate checks of $page to make sure its value isn't malicious.