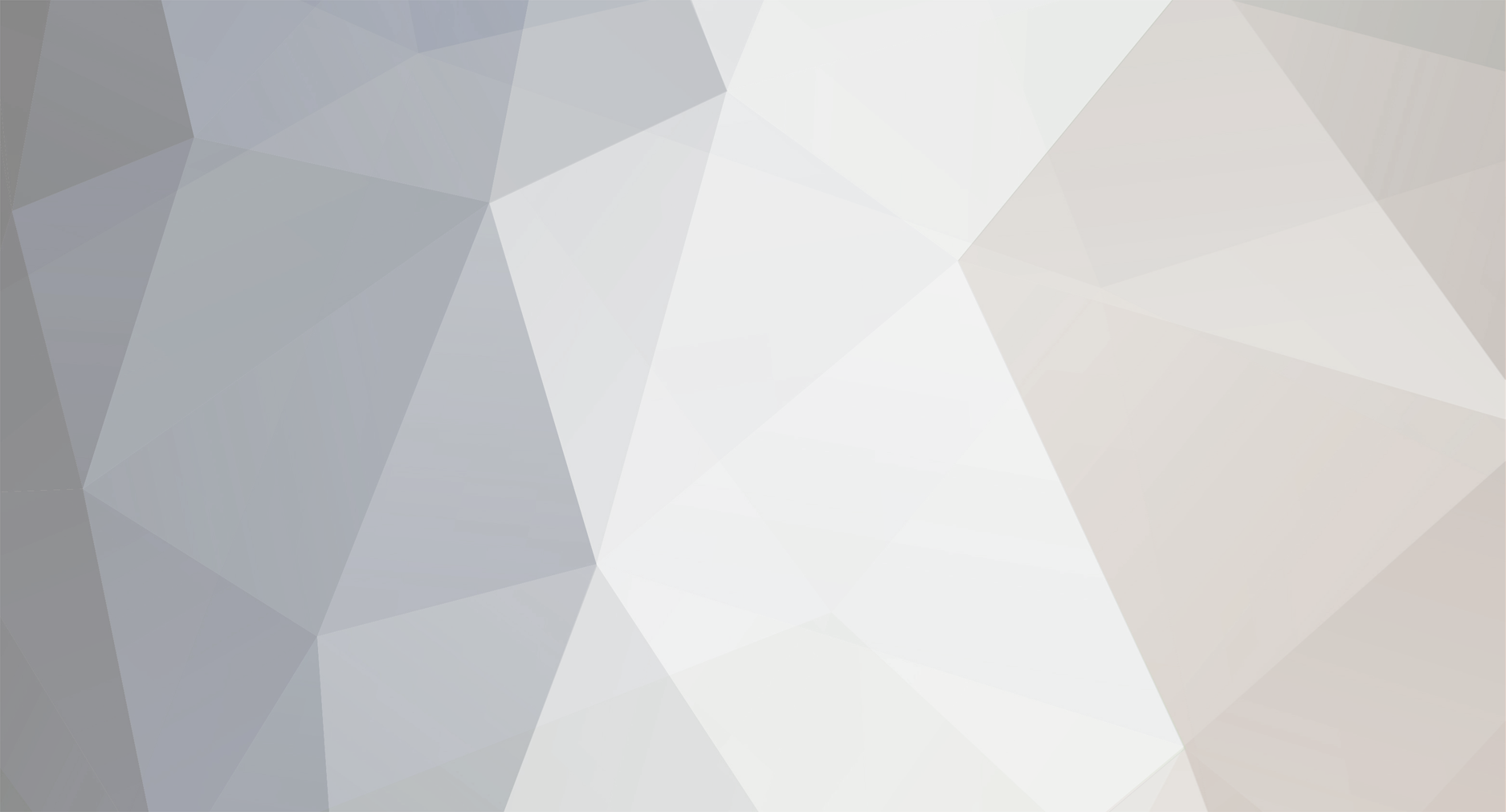
johnska7
Members-
Posts
27 -
Joined
-
Last visited
Never
Everything posted by johnska7
-
passing variables between forms on the same page
johnska7 replied to cljones81's topic in PHP Coding Help
You would have to look into a javascript solution, as PHP can only pass variables when a page is submitted to the server. -
I don't know why it's doing that, but you could always run a chmod command after creating the folder to change the permissions
-
Those are variables being passed to the next page. Anytime you see a '.php?', anything after that '?' is going to be a variable name, and then it's value. In this case, it is saying that the variable "red" is equal to "5". What you do with this is, on the next page, you have a piece of code that does a $_GET['red']. This will read the variable red and then let you do comparisons or whatever you need to do. I think in your case what you want to do is the following: Have a page that reads all entries in a table and display them into an HTML table. In each table, you'll have a link that gets the "ID" fields, or some other unique identifier, to put in as a url variable. Then, on your "download" page, you'll get that ID from the url, do another database query to get all the info about that item (including the title) and place all that info on the page, along with a link to download whatever the item is.
-
http://us.php.net/sort
-
Sounds like PHP isn't executing... are you sure that it is installed and active on your server?
-
O.k., so I made some advances today. I decided that I'm going to handle the bulk of the conversion with a perl script, since it seems to act a little better when doing multiple commands. I scripted out a PHP page that simply does: exec("convertvideo -source_movie=mtg_2.mov -final_name=mtg_2 > /dev/null &"); Problem is, it seems that php will still stop executing before this is done converting. I was trying to move away from PHP because I don't want to have to change my max_execution time... is this something that popen() can handle? Or do I have to find another way of pushing the process away from PHP? I or should I just suck it up and change my max_execution time?
-
assign a string to a variable - newbe problem
johnska7 replied to orbitalnets's topic in PHP Coding Help
You need concatenation, and quotes only need to be escaped (\") inside of other quotes So what you want is: $link = "<p>".$row_show_portfolio['url']."</p>"; If you wanted to have quotes in your string, then you'd need to do: $link = "<p>He said:\"I'm a nice guy!\" ".$row_show_portfolio['url']."</p>"; the above would print out (to the $link variable): "I'm a nice guy" your_row_return_here -
You also don't have a form, so right now this page should do nothing. You have two options: PHP or Javascript. The javascript method will allow you to check right then-and-there to see if any of the radio buttons is checked, and would be something to the effect of getting the element by id and seeing if it's value is something other than null. You would run this with an onClick function on your button. The PHP method would have you put a check at the top of your processing form that sees if the $_POST['something'] equals anything other than NULL. If it equals NULL, echo out an error message or redirect back to your menu page.
-
Here is a complete working copy of what it seems you'd like <?php if (isset($_POST['Submit'])== "Update Entry") { $missing = array(); foreach($_POST as $key=>$value) { if(empty($_POST[$key])) { array_push($missing, $key); } } if(!empty($missing)) { $missing = implode(", ", $missing); echo "<p class='special'>You must suppy a value for: ".$missing.".</p>"; } else { echo "<script type=\"text/javascript\">window.location=\"addform.php\"</script>"; } } ?> <form action="" method="post" enctype="application/x-www-form-urlencoded"> <div class="content">First Name: <input type="text" maxlength="20" name="Fname" id="Fname" size="15" value="<?php if (isset($_POST['Fname'])) echo $_POST['Fname'] ?>" /></div> <div class="content">Last Name: <input type="text" maxlength="20" name="LName" id="LName" size="15" value="<?php if (isset($_POST['LName'])) echo $_POST['LName'] ?>" /></div> <div class="content">Address: <input type="text" maxlength="20" name="Address" id="Address" size="15" value="<?php if (isset($_POST['Address'])) echo $_POST['Address'] ?>" /></div> <div class="content">City: <input type="text" maxlength="20" name="City" id="City" size="15" value="<?php if (isset($_POST['City'])) echo $_POST['City'] ?>" /></div> <div class="content">State: <input type="text" maxlength="20" name="State" id="State" size="15" value="<?php if (isset($_POST['State'])) echo $_POST['State'] ?>" /></div> <div class="content">Zip: <input type="text" maxlength="20" name="Zip" id="Zip" size="15" value="<?php if (isset($_POST['Zip'])) echo $_POST['Zip'] ?>" /></div> <div class="content">Phone: <input type="text" maxlength="20" name="Phone" id="Phone" size="15" value="<?php if (isset($_POST['Phone'])) echo $_POST['Phone'] ?>" /></div> <div class="submit"><input type="submit" name="Submit" id="Submit" namevalue="Update Entry" /></div> </form> The extra array code at the top will see what the person is missing from their submission and tell them exactly what fields they still need to fill out and it's dynamic, so if you add more fields, it will pick them up.
-
Gotta have it all wrapped around and If then else, like so <?php if (isset($_POST['Submit'])== "Update Entry") { if (empty($_POST['FName']) || empty($_POST['LName']) || empty($_POST['Address']) || empty($_POST['City']) || empty($_POST['State']) || empty($_POST['Zip']) || empty($_POST['Phone'])) { echo "<p class='special'>You must complete the form in full.</p>"; } } else { ?> <form action="" method="post" enctype="application/x-www-form-urlencoded"> <div class="content">First Name: <input type="text" maxlength="20" name="Fname" id="Fname" size="15" value="<?php if (empty($_POST['Fname'])) echo $_POST['Fname'] ?>" /></div> <div class="content">Last Name: <input type="text" maxlength="20" name="LName" id="LName" size="15" value="<?php if (empty($_POST['LName'])) echo $_POST['LName'] ?>" /></div> <div class="content">Address: <input type="text" maxlength="20" name="Address" id="Address" size="15" value="<?php if (empty($_POST['Address'])) echo $_POST['Address'] ?>" /></div> <div class="content">City: <input type="text" maxlength="20" name="City" id="City" size="15" value="<?php if (empty($_POST['City'])) echo $_POST['City'] ?>" /></div> <div class="content">State: <input type="text" maxlength="20" name="State" id="State" size="15" value="<?php if (empty($_POST['State'])) echo $_POST['State'] ?>" /></div> <div class="content">Zip: <input type="text" maxlength="20" name="Zip" id="Zip" size="15" value="<?php if (empty($_POST['Zip'])) echo $_POST['Zip'] ?>" /></div> <div class="content">Phone: <input type="text" maxlength="20" name="Phone" id="Phone" size="15" value="<?php if (empty($_POST['Phone'])) echo $_POST['Phone'] ?>" /></div> <div class="submit"><input type="submit" name="Submit" id="Submit" namevalue="Update Entry" /></div> </form> <?php } ?> What this winds up saying is "If the form has been submitted, do the check and print out the error messages ELSE print out the form to be filled out." If you need it to put a message at the top of the form when fields are missing but still print out the form, that's something else.
-
You should wrap the top part into a check to see if the form has been submitted in general, ie. <? if($_POST['Submit'] == "Update Entry") { if (empty($_GET['FName'])) { echo "<p class='special'>You must complete the form in full.</p>"; } } else { ?> <form action="AddForm.php" method="get" enctype="application/x-www-form-urlencoded"> <div class="content">First Name: <input type="text" maxlength="20" name="Fname" id="Fname" size="15" value="<?php if (empty($_GET['Fname'])) echo $_GET['Fname'] ?>" /></div> <div class="content">Last Name: <input type="text" maxlength="20" name="LName" id="LName" size="15" value="<?php if (empty($_GET['LName'])) echo $_GET['LName'] ?>" /></div> <div class="content">Address: <input type="text" maxlength="20" name="Address" id="Address" size="15" value="<?php if (empty($_GET['Address'])) echo $_GET['Address'] ?>" /></div> <div class="content">City: <input type="text" maxlength="20" name="City" id="City" size="15" value="<?php if (empty($_GET['City'])) echo $_GET['City'] ?>" /></div> <div class="content">State: <input type="text" maxlength="20" name="State" id="State" size="15" value="<?php if (empty($_GET['State'])) echo $_GET['State'] ?>" /></div> <div class="content">Zip: <input type="text" maxlength="20" name="Zip" id="Zip" size="15" value="<?php if (empty($_GET['Zip'])) echo $_GET['Zip'] ?>" /></div> <div class="content">Phone: <input type="text" maxlength="20" name="Phone" id="Phone" size="15" value="<?php if (empty($_GET['Phone'])) echo $_GET['Phone'] ?>" /></div> <div class="submit"><input type="submit" name="Submit" value="Update Entry" /></div> </form> <? } ?>
-
O.k., so I was able to get the process to work directly with exec("ffmpeg... >/dev/null &"); So my next question is, is it possible to have it alert me (email, etc) when it's done? Or stack commands, so I can encode, then launch an injector process, then copy? I know I can probably create a whole php file for it and then do a exec("php converter.php?action=convert"), etc for the actions, but how can I queue them up so they happen one after the other?
-
Amazing! Thank you very much.
-
Use the task scheduler in windows
-
Hello everyone, I'm trying to set up a workflow for converting uploaded videos from flv, creating the screenshot, injecting metadata and then copying to the proper folder. I can do all of that stuff, but what I'm trying to figure out is, if I want to have a "queue" area (most of these files are over 1 gig, so they won't upload directly through PHP), I'll have the user select their movie and say "Convert", but is there a way to make it so that when PHP does the exec command to have it run as a different process, or escape from running it in the browser? A normal video conversion can take over 20 minutes, so I don't want to have the page sitting there loading or even timing out. I'd rather have it display a message that the conversion is happening and that an email will be sent to the person when it's all finished. Any help would be greatly appreciated!
-
MySQL Permission - Can create tables, but not edit rows...
johnska7 replied to johnska7's topic in MySQL Help
Just to make sure, I also just created a new user and did "GRANT ALL on database_name.* TO user_name". Same error happens. I can create tables, but I can't enter data into the rows once they're created. -
MySQL Permission - Can create tables, but not edit rows...
johnska7 replied to johnska7's topic in MySQL Help
I'm clicking on the cell below the column header, where I should be able to enter a value. I should probably also say that I have a working setup on a Mac server at work, so I'm not really sure why I can't get this to work. It's MySQL 5.0 on an Ubuntu box. -
This is going to drive me nuts. I'm trying to set up a dev environment in which the root user will be able to access every table in every database I have. Currently, I can edit the mysql and information_scheme tables, and any tables that I have restored from a backup. But every time I add a new table to any database, I can't edit any of the rows. I'm currently using the MySQL Query Browser on OS X and the "edit" button stays grayed out on any of these new tables that are created. Can anyone help me walk though some troubleshooting steps so I can move forward on this? Thanks!
-
Actually, I think I may have found a way. I think I can use Insertion.after, along with some PHP and javascript trickery. Please let me know if you have any ideas still, but I'm going to try this out at the same time.
-
What I have so far looks like this (rendered from the original source): <div id="content"> <form action="" name="new" method="post"> <input name="title" type="text"><label for="area">Area</label> <input name="tutorial_area" id="tutorial_area" value="2" onclick="updateArea('program', '2')" type="radio"> 2D<br> <input name="tutorial_area" id="tutorial_area" value="1" onclick="updateArea('program', '1')" type="radio"> 3D<br> <input name="tutorial_area" id="tutorial_area" value="3" onclick="updateArea('program', '3')" type="radio"> Layout<br> <input name="tutorial_area" id="tutorial_area" value="4" onclick="updateArea('program', '4')" type="radio"> Web Dev<br> <div id="program"><br><label for="program">Program</label> <input name="tutorial_program" id="tutorial_program" value="4" onclick="updateArea('subject', '4')" type="radio"> 3D Studio Max<br> <input name="tutorial_program" id="tutorial_program" value="1" onclick="updateArea('subject', '1')" type="radio"> Maya<br> <input name="tutorial_program" id="tutorial_program" value="3" onclick="updateArea('subject', '3')" type="radio"> XSI<br> </div> <br> <div id="subject"><label for="subject">Subject</label> <input name="tutorial_subject" id="tutorial_subject" value="1" onclick="addStep();" type="radio"> modeling </div> <div id="step_1" style="display: none;"></div> <input name="Submit" value="Submit" type="submit"></form> </div> I haven't optimized the code yet, so I know there are XHTML errors and such. What I'm trying to figure out is how to keep adding divs to the page without have to define them first, as there can (theoretically) be an unlimited number of steps. BTW, I'm using PHP to generate most of this, if that helps. The first three options are made with the following call: function updateArea(db, ID) { new Ajax.Updater(db, 'includes/ajax.php', { method: 'get', parameters: { action: 'updateArea', type: db, id: ID} }); if(db != "subject") { new Ajax.Updater('subject', 'includes/ajax.php', { method: 'get', parameters: { action: 'updateArea', type: 'subjectRemove' } }); } } So I'm not sure if I'm going about it the right way yet or not. I did look at the site you posted, tom, yesterday when I was trying to figure some of this out but it's a bit confusing. Thanks for the quick response!
-
Hello all, I'm trying to use ajax (via prototype) to add elements to a page. What I have so far is: Person picks an area, which adds a program option to the page. They choose a program and it adds a subject option to the page. When they pick the subject, I want it to bring in the ability to enter text and then have a link to add another element of text under it, which has a link to add another element of text...and so on. I'm trying to figure out how I can use inner.html, but I'm not having much luck, so if anyone has a tutorial or can help guide me through all of this, I'd be really appreciative. Thanks!
-
Can you post the following lines from your config file? $cfg['Servers'][$i]['host'] $cfg['Servers'][$i]['port'] $cfg['Servers'][$i]['socket'] $cfg['Servers'][$i]['connect_type'] $cfg['Servers'][$i]['auth_type']
-
Hey all, I have a nice little script that exports the contents of my database to an excel file. I have everything working (even the headers so that it pops open a window for opening or saving the file) but what I'm left with is a blank white screen behind the window...the page is accessed via a link, not a form, and everything is done between my function file and the top of the page that does an include on the function file. My question is, how can I make it so that the link that I click off of stay on the screen, or reload the screen, after clicking the link so that the people who are trying to get the excel file can still be in the regular area and not have to hit the back button.
-
Perfect! I need to figure out how to get it to flow with what I already have, but it's great. And I figured out why it wasn't showing before...I was selecting the wrong table...TGIF :)
-
O.k., Huggie, your idea semi worked...I can see the results when I execute that line in mysql admin, but when I try to execute your code (and other iterations) in a web page, I just get a blank screen. Also, I checked to see how many rows were being returned in the fetch and only 5 were returned...I have well over 3000 rows in the database, and about 13 rows that it shows for "fields" when I execute the SHOW in mysql admin...any idea what might be going on?