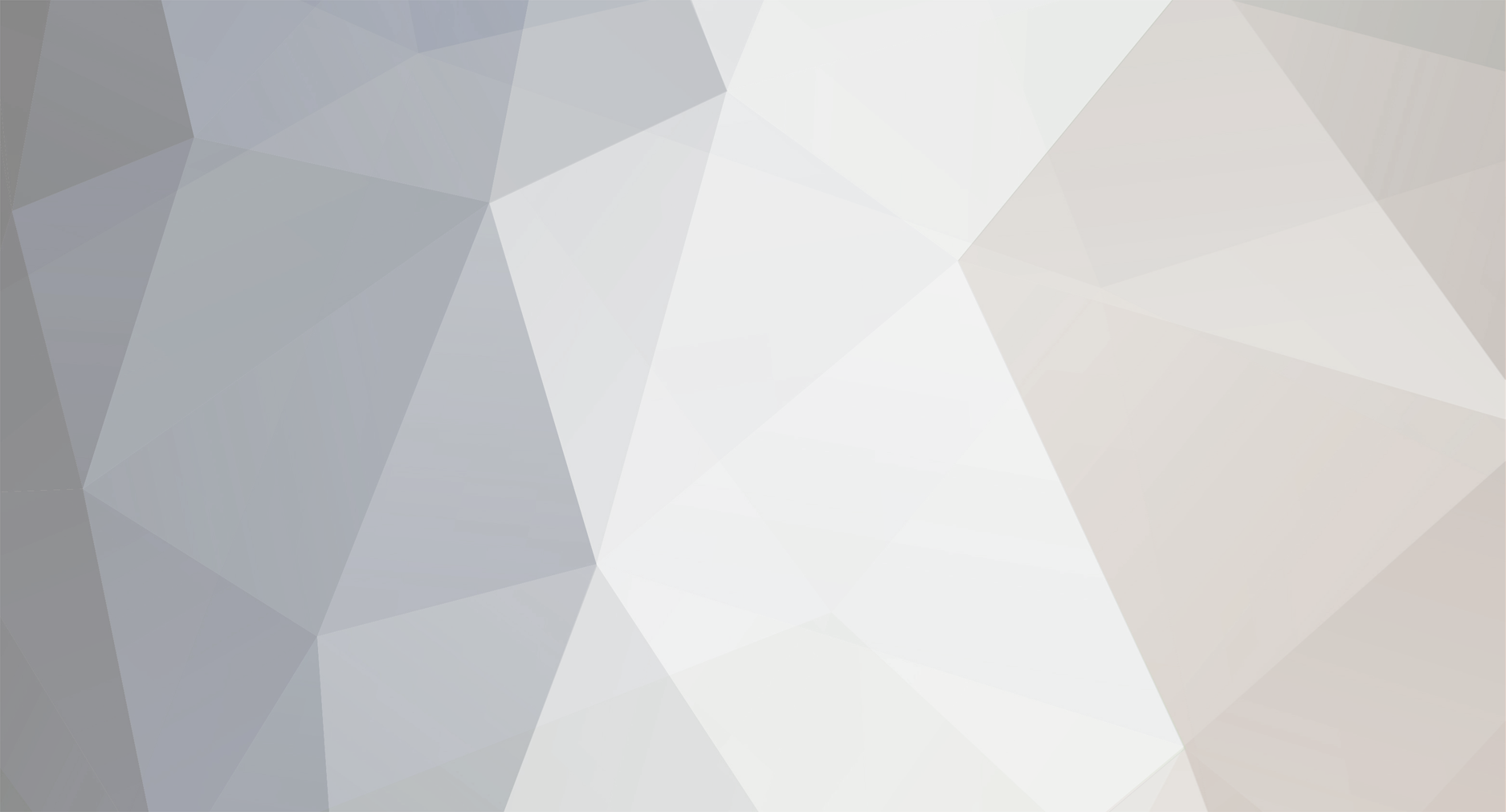
mem0ri
Members-
Posts
110 -
Joined
-
Last visited
Never
Everything posted by mem0ri
-
Grabbing a variable from within the same file
mem0ri replied to monkeybidz's topic in PHP Coding Help
Sounds like you need to be working in JavaScript. PHP won't allow you to dynamically manipulate code like that...once it's run through, it's run through until the page is refreshed. -
You can try to set the file permissions in the PHP script just to make sure with chmod() function. It may also be a simple case of not setting sub-folder permissions correctly. Often, if you set the permissions for a 'main' folder and then create a sub-folder later...the subfolder will still be created WITHOUT the desired permissions. You may need to go in and change the sub-folder perms.
-
Use the mysql_real_escape_string function :-)
-
Can you help us out by posting the actual code that you're running? It's impossible to debug something when we can't see it.
-
You're missing the final step of taking the $result-set object and fetching each row from it as an array...try something like: $result = mysql_query($sql, $GLOBALS[connectionID]); while ($row = mysql_fetch_array($result) $db[] = $row; Your $db variable will then look like: $db = array(0=>array('field1'=>$value1...), 1=>array('field1'=>$value2)...); ...where each array-value in $db is an array that contains an entire row of the database.
-
The page naming is actually: http://www.mysite.com/index.php?page=2 It's called "dynamic" paging...and basically you're just sending at $_GET variable to the index page over and over again to tell it which .php (or .html or other) file to load for content.
-
More help needed :( PHP Form and SQL query to add info to database
mem0ri replied to Jax2's topic in PHP Coding Help
You want to sanitize all of your strings for the database so that the information you attempt to INSERT doesn't cut off or error due to ' or other symbols. Remember also that SQL-injection hacks can occur when you don't sanitize your strings. Try passing all of your $_POST variables through: mysql_escape_string() -
You'll want to add: $row = mysql_fetch_array($result); echo($row[0]); You need to capture the result array from the result-object...and then display that result.
-
You need to switch your $this->getTitle() with $view->getTitle() . As far as class-optimization goes, you might want to take a look at the __get and __set methods for setting/getting variables within an object.
-
I'm not sure exactly how the interaction with your file works from the user-interface, but the ampersand is a special character in a URL and can result in incomplete url-requests if you're expecting to include the ampersand character in the URL name. You will want to use rawurlencode / rawurldecode in order to get around that difficulty.
-
You're going to have to give us more information than just those two lines of very simple code... ...a headers-already-sent error means that you've already established header-elements on the page and therefore cannot modify them further. You will need to change the location of your code-block to occur before headers are sent.
-
I'd probably do the "show four" in PHP myself as I like to run a single DB query and then organize the information display via PHP. I especially wouldn't want to be running 100 different SELECT statements limited to 4 results each if I had 100 projects...especially since the innoDB engine for MySQL can really only handle about 200 qps.
-
Get rid of the ?> at the end of your form (on the </form> line) and the <? immediately following. It's possibily your PHP.ini file isn't setup to handle 'short php tags' and that's messing thigns up--plus, you don't need to end PHP code and start PHP code again right in the middle of PHP code.
-
I would keep your 100 pages in a session-variable array... $arr = array('pagename', 'pagename', pagename'....)...giving you key-values 0-99 for all 100 pages... ...then...when someone initiates a new page-load to one of these random pages... $y = count($arr) -1; $val = rand(0, $y); //load $arr[$val]; unset($arr[$val]); That should work.
-
You're definitely going to want to use AJAX / XML importing. You may want to research FLEX and MXML (Adobe's solution for passing information to and from Flash).
-
I've got a situation where...in the one case I'm setting a bitwise value $x: $x = (2 << $y); And another where I need to instead 'discover' the value of $y if $x is known instead... $y = 2 ???? $x; I could run a while loop.... while(($x/2) != 2) $z++; But I'm wondering if someone might know another, more 'efficient' way of working out the equation? It SEEMS like there must be an inverse relationship with << and >> that would allow you to figure how many bit's a number is shifted... BTW...I'm using only factorial values in this area of the app...so all numbers would come out as 'clean' numbers like 2, 4, 8, 16, 32, etc.
-
Change date to an INT...I go with INT 11 ...then just insert time() anytime you run an update.
-
[SOLVED] while + insert after every second run?
mem0ri replied to blueman378's topic in PHP Coding Help
You're going to want to run an incrementation on your while loop (like an $x++) and check every loop through of whether the remainder (x % 2) == 1. If so, </td></tr>...else just </td>...conversely, you'll have to switch between <tr><td> and just <td> at the start of each while loop. It's easily done...just did it earlier today actually...just can't pull up the code at the moment. -
My initial thought is that your $_SESSION variable isn't necessarily wrong, but that other things could be... Just a quick note: In your $query, you're selecting both "username" and "valid" where username = $username and valid = 1...you can get rid of selecting "username" and "valid", 'cause you already have values for them ($username and 1). So...just select end_date WHERE username = '$username' AND psword = '$psword' AND valid = '1'"; Also...if valid is going to be a numeric (I'm GUESSING it would be a boolean...a 1 or a 0 for TRUE / FALSE...) change the datatype to TINYINT and get rid of the quotes around valid so that it's ...valid=1"; Now onto the problem... //Original block of code if ($num >0) { $username = mysql_fetch_array($results); //fetches result row $_SESSION['username'] = $username['username']; $end_date = mysql_fetch_array($results); //fetches 'next' row...non existent $_SESSION['end_date'] = $end_date['end_date']; (header("location: header.php")); } //New block if($num > 0) { $result = mysql_fetch_array($results); //you should only pull the result once, otherwise it'll attempt to fetch the next row $_SESSION['username'] = $username; //Remember we're not fetching the username result anymore $_SESSION['end_date'] = $result['end_date']; (header("location: header.php")); }
-
If it's a single field of numbers separated by spaces...run something like... $array = explode(" ", $field); $count = count($array);
-
Can anyone show me how to make a internet store?
mem0ri replied to realestninja's topic in PHP Coding Help
You're grabbing onto a rather large project there for a pure beginner. E-Commerce solutions are generally very robust and complex applications, especially when you get to the level of Best Buy, Circuit City, or Amazon.com. You might want to take a look at the open-source E-Commerce programs available (I don't like them, but they are open source and free--Zen Cart is the most widely known one). You may also want to check out other open-source PHP E-Commerce solutions that you would have to shell out a few $$ for (like X-Cart...uses Smarty templating for easy UI manipulation)... ...if you're set on making your own or having someone to teach you to make your own...I'm sure someone will do it for you...but it won't be cheap. -
Not sure if you completely made sense...but if you're just trying to filter out already approved texts vs. new texts coming in...and compare the new ones against the approved ones to make sure you never get duplicates... ...why don't you just add a boolean value column to your table stating "approved" or "new" (1 or 0)...and check all 0's against 1's....
-
I failed to mention...and couldn't edit: You will NOT be able to use the functions "as is"...they are tied with the data of the entire MySQL Class...and, as I mentioned before, it's a pretty dirty setup...but it'll give you an idea.
-
What you're going to want to do is: 1--Grab all categories that don't have a parent (parent_id = 0 or whatnot...your 1 and 2--Grab the children of the first root category...and for each child, check if they have children...and for each child...check if they have children...etc. You can do so with 2 functions...with the second calling itself repeatedly. I've got a rather dirty version in a MySQL class I've written for PHP 5 that I'll post below to help you out: // categorize(id, parent_id, category_name, prefix_to_add_each_next_level) public function categorize($parent, $child, $name= '', $pre=' ') { $cols = $this->getnumcolumns(); while($row = $this->getrow()) { $rawkeys = array_keys($row); $y = count($rawkeys); for($x = 1; $x < $y; $x+=2) $keys[] = $rawkeys[$x]; for($x = 0; $x < $cols; $x++) $data[$keys[$x]][] = $row[$x]; } if($name != '' && !array_key_exists($name, $data)) $name = ''; $root = array_keys($data[$child], 0); $y = count($root); for($x = 0; $x < $y; $x++) { $prefix = ''; for($z = 0; $z < $cols; $z++) $temp[$keys[$z]] = $data[$keys[$z]][$root[$x]]; $this->categorized[] = $temp; $this->getchild($cols, $parent, $child, $keys, $data, $data[$parent][$root[$x]], $name, $prefix, $pre); } return $this->categorized; } private function getchild($cols, $parent, $child, $keys, $data, $value, $name, $prefix, $pre) { $prefix .= $pre; $children = array_keys($data[$child], $value); $x = count($children); if($x >= 1) { for($y = 0; $y < $x; $y++) { if($name != '') $data[$name][$children[$y]] = $prefix.$data[$name][$children[$y]]; for($z = 0; $z < $cols; $z++) $temp[$keys[$z]] = $data[$keys[$z]][$children[$y]]; $this->categorized[] = $temp; $this->getchild($cols, $child, $parent, $keys, $data, $temp[$parent], $name, $prefix, $pre); } } }
-
make it where users can't put < or leave form blank?
mem0ri replied to A2xA's topic in PHP Coding Help
If you really want to make your visitors happy, it's a good idea to throw a little DHTML / JavaScript validation into the form.