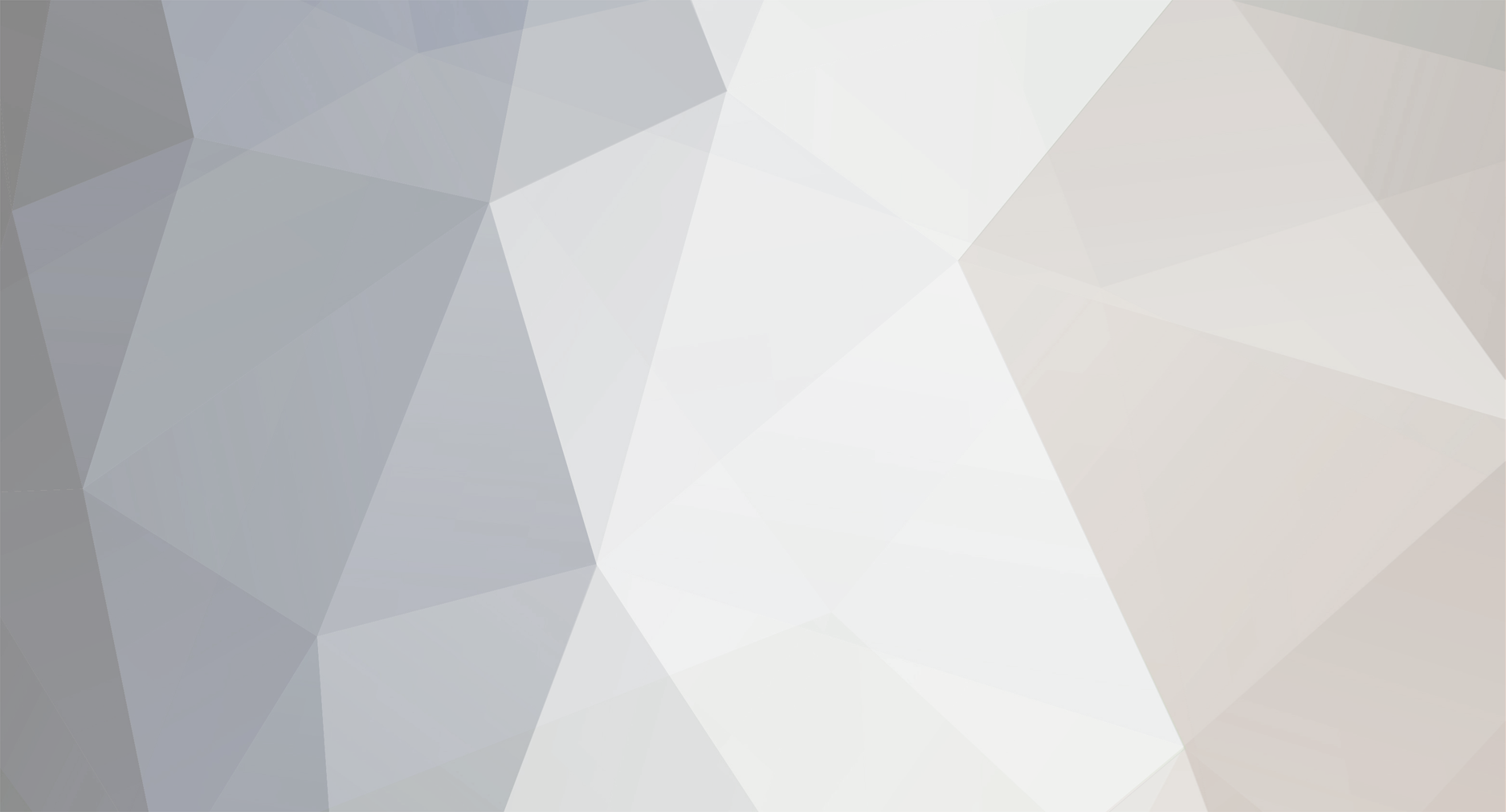
simcoweb
Members-
Posts
1,104 -
Joined
-
Last visited
Everything posted by simcoweb
-
how to only allow upload of ms word documents?
simcoweb replied to chrisuk's topic in PHP Coding Help
Normally you'd just validate against the extension since Word documents have the .doc extension. For example, here's how I validate an image extension on a file upload: <?php $allowedUpload = preg_match('/\\.(gif|jpg|jpeg|png)$/i', $_FILES['photo']['name']) ? true : false; ?> -
[SOLVED] Get next and previous records of the table
simcoweb replied to Soleil's topic in PHP Coding Help
I don't have the specific syntax to provide but you should look into the next() and previous() functions of PHP that moves to the next item in the array regardless of the id number. http://us2.php.net/manual/en/function.prev.php http://us2.php.net/manual/en/function.next.php -
Looking for some help on creating a schedule script
simcoweb replied to peterbarone's topic in PHP Coding Help
Honestly? Not so good. Two variables don't make a script. I'm still confused as to what you are looking to do. Aside from the fact you want a scheduling system you don't have any idea of how it would work. I outlined that in my first post. Getting info into the database requires some insertion technique...typically a form with the questions/info you need. Then you need a way to extract it. Typically with a MySQL query. Then a way to display it. Typically a web page. I'm not sure what else I can tell you. -
Looking for some help on creating a schedule script
simcoweb replied to peterbarone's topic in PHP Coding Help
Umm, I don't see how you can automate it without someone doing some input. What did you have in mind? -
Looking for some help on creating a schedule script
simcoweb replied to peterbarone's topic in PHP Coding Help
First, figure out what fields/info you have to have on each team. Then, create the input form for it. Next, write the script that inserts it into the database. Last, write the script that retrieves and displays it. -
[SOLVED] Grrrr... login page not redirecting after login
simcoweb replied to simcoweb's topic in PHP Coding Help
Ta Daaa!! Ok, that was the missing link. I had md5'd the password but wasn't referring to it in the login script. I just deleted and reinserted the admin profile using the md5 hash for the password, modified that line you pointed out in the login script, and was able to log in successfully without incident. Thanks to everyone! -
Not sure why you'd want to call a field that's blank ???
-
[SOLVED] Grrrr... login page not redirecting after login
simcoweb replied to simcoweb's topic in PHP Coding Help
Can you elaborate on that, puhleeeez? -
[SOLVED] Grrrr... login page not redirecting after login
simcoweb replied to simcoweb's topic in PHP Coding Help
I believe i've found the problem. I went into the database and removed the md5 encrypted password and replaced it with the unencrypted password and when logging in I received a syntax error for a function I needed to edit. This is a milestone in itself since all I was getting before was a blank page! So, I fixed the function and tried again. Voila! logged right in with no problems and the page displayed perfectly. So, the problem is with how i've created the username/password and inserted it into the mysql database. Basically I wrote a quickie little script to add the administrator's username/password. Here it is: <?php // create admin identity include 'adminheader.php'; // this is a one time effort $username = "bozotheclown"; $password = "bozosbigtop"; $enc_password = md5($password); // run query to insert include 'dbconfig.php'; // Connect to database $con = mysql_connect($dbhost, $dbuser, $dbpass) or die(mysql_error()); mysql_select_db($dbname, $con) or die(mysql_error()); $sql = "INSERT INTO eastside_admin (username, password) VALUES ('$username', '$enc_password')"; $results = mysql_query($sql) or die(mysql_error()); $num_rows = mysql_affected_rows($con); if ($num_rows == 1) { echo "Administrator successfully added"; } else { echo "Could not insert administrator for some reason."; } include 'adminfooter.php'; ?> I'm not sure at all if i've handled the encryption part properly ( I had another thread on that and this is what we settled on ) or if I have the field attributes set properly. VARCHAR and size 32 -
[SOLVED] Grrrr... login page not redirecting after login
simcoweb replied to simcoweb's topic in PHP Coding Help
Ok, i've made both of those last changes. STILL it just goes to the index.php and is totally blank. There has to be something in the code in the login page perhaps that is causing it to loop? During the log in process I don't see any incidence of where it goes to the eastside_admin.php page then returns to the index.php page. It just 'stays' on the index.php page (login page) and goes blank. -
[SOLVED] Grrrr... login page not redirecting after login
simcoweb replied to simcoweb's topic in PHP Coding Help
Arggh! Still doing the same thing! -
[SOLVED] Grrrr... login page not redirecting after login
simcoweb replied to simcoweb's topic in PHP Coding Help
Adika, I changed that line of code but, alas, it still does the same thing When I enter username/password into the index.php (login) page it remains on that page and is blank. -
Check this out: http://us3.php.net/unset
-
[SOLVED] Grrrr... login page not redirecting after login
simcoweb replied to simcoweb's topic in PHP Coding Help
You mean the login page? Here's the full code: <?php session_start(); $loginError = ""; // declare this so it is always available // Turn on magic quotes to prevent SQL injection attacks if(!get_magic_quotes_gpc()) set_magic_quotes_runtime(1); // Validate users input if(!empty($_POST)) { // Check username has a value if(empty($_POST['username'])) $loginError['username'] = "Please enter a user name!"; // Check password has a value if(empty($_POST['password'])) $loginError['password'] = "Please enter a password!"; // Check if any errors were returned and run relevant code if(empty($loginError)) { $username = $_POST['username']; $password = $_POST['password']; $_SESSION['loggedin'] = $_POST['loggedin']; include 'dbconfig.php'; // Connect to database $con = mysql_connect($dbhost, $dbuser, $dbpass) or die(mysql_error()); mysql_select_db($dbname, $con) or die(mysql_error()); // Get Record Set $sql = ("SELECT * FROM eastside_admin WHERE username = '$username' AND password = '$password'"); // mysql_query($sql) or die(mysql_error()); $results = mysql_query($sql, $con) or die(mysql_error()); $num_rows = mysql_num_rows($results) or die(mysql_error()); if (mysql_num_rows($results) == "1") { $_SESSION['loggedin'] = $_POST['loggedin']; header("Location: eastside_admin.php"); exit; } else { echo "Your user name and password do not match any in our database! Please try again. <a class='body' href='login.php'>Return to login page.<br>\n"; } } } include 'adminheader.php'; ?> <div align="center"> <table style="BORDER-COLLAPSE: collapse" bordercolor="#666666" cellpadding="4" width="530" border="0"> <tbody> <tr> <td> <h2 align="center">Login page</h2> <font color="red"><div align="center"> <? // Loop through all errors if(!empty($loginError)) { ?> <ul> <? foreach($loginError as $eg_message) { ?> <li id="validationError"><?= @$eg_message ?></li> <? } ?> </ul> <? } ?> </font></div> <form action="<? $_SERVER['PHP_SELF'] ?>" name="login" method="post" > <input type="hidden" value="loggedin" name="loggedin"> <table cellspacing="0" cols="2" cellpadding="0" align="center" border="0"> <tbody> <tr><td>User name: </td><td><input type='text' size='21' name="username" ></td> </tr> <tr> <td>Password:</td><td><input type="password" size="21" name="password"></td> </tr> <tr> <td></td> <td align="right"><br><input type="submit" value="Login"></td> </tr> </tbody> </table> </form> </td></tr> </tbody> </table> </div> <p> <? include 'adminfooter.php'; ?> -
[SOLVED] Grrrr... login page not redirecting after login
simcoweb replied to simcoweb's topic in PHP Coding Help
Adika, can you provide me some samples of what you mean, please? -
Getting mysql error when trying to insert md5 password
simcoweb replied to simcoweb's topic in PHP Coding Help
Ok, didn't know that. Thanks! No more errors -
I've used this same snippet of code in other login forms without a problem (with some slight modifications from script to script) but this one seems to baffle me. Basically it takes the login and validates the entries (validation works, error messages display if left blank), then checks the database for a match. If there's a match then it is supposed to forward the person onto the proper page using the 'header' function. Problem is it's not. Basically it's reverting back to the 'index.php' page (login page) and the display is completely blank. Here's the code for index.php: <?php $con = mysql_connect($dbhost, $dbuser, $dbpass) or die(mysql_error()); mysql_select_db($dbname, $con) or die(mysql_error()); // Get Record Set $sql = ("SELECT * FROM eastside_admin WHERE username = '$username' AND password = '$password'"); // mysql_query($sql) or die(mysql_error()); $results = mysql_query($sql, $con) or die(mysql_error()); $num_rows = mysql_num_rows($results) or die(mysql_error()); if (mysql_num_rows($results) == "1") { $_SESSION['loggedin'] = $_POST['loggedin']; header("Location: eastside_admin.php"); exit; } else { echo "Your user name and password do not match any in our database! Please try again. <a class='body' href='login.php'>Return to login page.<br>\n"; } ?> The code in the 'eastside_admin.php': <?php ob_start(); session_start(); if isset($_SESSION['loggedin']) { include 'adminheader.php'; include 'admin_menu.php'; include 'adminfooter.php'; } else { echo "This is a restricted area requiring login. Please return to the login page"; header("Location: index.php"); exit; } ?>
-
Getting mysql error when trying to insert md5 password
simcoweb replied to simcoweb's topic in PHP Coding Help
I got it to insert and to encrypt it by changing to this: <?php $username = "bozotheclown"; $password = "bozosbigtop"; $enc_password = md5($password); // run query to insert include 'dbconfig.php'; // Connect to database $con = mysql_connect($dbhost, $dbuser, $dbpass) or die(mysql_error()); mysql_select_db($dbname, $con) or die(mysql_error()); $sql = "INSERT INTO eastside_admin (username, password) VALUES ('$username', '$enc_password')"; $results = mysql_query($sql) or die(mysql_error()); ?> But, when I try to validate that it worked I get an error using this code: <?php $num_rows = mysql_affected_rows($results); if ($num_rows == 1) { echo "Administrator successfully added"; } else { echo "Could not insert administrator for some reason."; } ?> What's strange is that it IS inserting the user info but then fails on the validation and shows the "Could not insert..." message. When I view the database I see the entry, however. -
Ok, simple stuff. I'm writing a quickie script to insert a user (username & encrypted password) into a mysql table for the purpose of adding an administrator/user/login. I have this: $username = "bozotheclown"; $password = md5("bozosbigtop"); with query written in standard stuff: $sql = "INSERT INTO admin_table (username, password) VALUES '$username', '$password'"; But I get a mysql error: I've not used the md5 for anything yet so not familiar with how i'd set this up.
-
Aren't you using a 'username' and 'password' for the registration?
-
[SOLVED] Quick little simple thing ((UNSOLVED))
simcoweb replied to djfox's topic in PHP Coding Help
HTML is HTML whether it's inside or outside. The only difference is the escaping of the quotes. Even though you can get away with leaving out the # sign in your hex color code it's best to remain with strict HTML coding policies and include it. The browser wars are still raging. Personally I like using double quotes (" " ) for surrounding the code and singles quotes within. That way I don't have to escape them: $res = "<tr><td valign=top width=100% bgcolor='#312D37' colspan=3><b>Basic Information</b></td></tr>"; -
So it's converting the quotes to their equivalent HTML code characters when you write the file? Is that what i'm understanding?
-
Just keep in mind that the mysql query gets all the info from the database as per instructions then the mysql_fetch_array splits each field into a separate item based on the field name which you can then echo or manipulate individually like I outlined there. So, each item in the array can have its own HTML parameters surrounding it. For example: <b>" . $row['song'] . "</b> would bold face the results for that field.
-
On a second look, my guess is probably wrong since further down it's referring to >= which is greater than or equal to 0. But, the error comes when it's trying to set the value of the variable with the mysql_num_rows function. So, the fact that the table is empty shouldn't produce the error. It's something in the line that's setting the variable.
-
Like this: <?php $sql= "SELECT * FROM Downloads"; $results = mysql_query($sql) or die(mysql_error()); echo "<table border='0' width='500'> <tr><th>| Song |</th><th>Size |</th><th>Download |</th><th>Mirror |</th></tr>"; while ($row = mysql_fetch_array($results) { echo "<tr><td>" . $row['song'] . "</td><td>" . $row['size'] . "</td><td><a href='download.php?song=" . $row['download'] . "'>Download</a></td><td>" . $row['mirror'] . "</td></tr>"; } echo "</table>\n"; ?> This won't be 100% pretty but you can take it from there to clean up the HTML to make it prettier with some style tags.