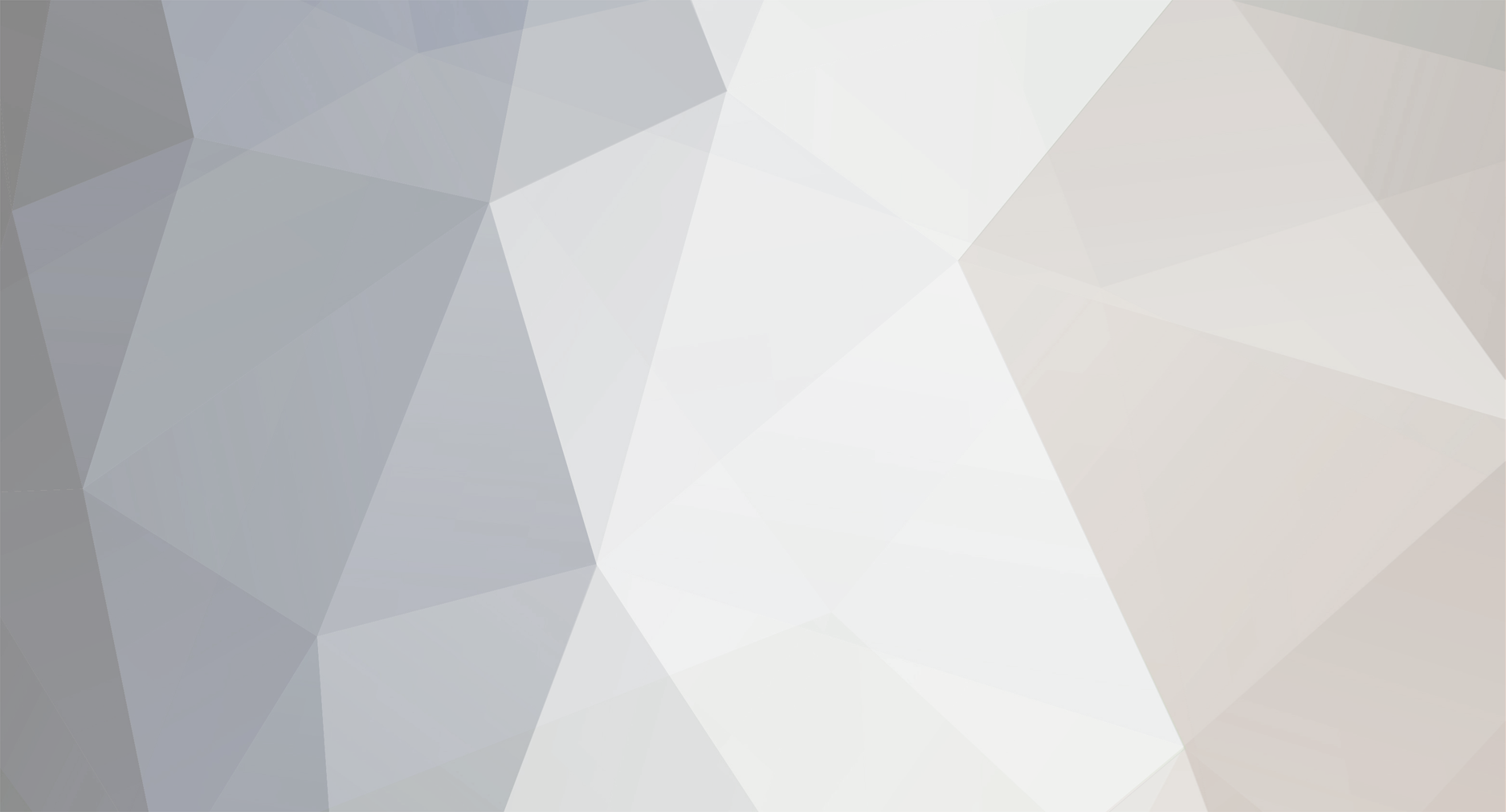
simcoweb
Members-
Posts
1,104 -
Joined
-
Last visited
Everything posted by simcoweb
-
Ok, found the problem! I was repeating the show_results(); function in the header.inc file! That issue is resolved!
-
I was doing an 'if' and 'else' statement so if the 'if' returned false then the 'else' would prevail. I've since changed it to two separate 'ifs' while experimenting. Here's the entire block of code with the query, the 'if' statements dependent upon that query. <?php $link = mysql_connect($dbhost, $dbuser, $dbpass) or die(mysql_error()); mysql_select_db($dbname, $link) or die(mysql_error()); // run our query to insert the record $sql = "INSERT INTO leads (first_name, last_name, email, day_phone, alt_phone, best_time, address, city, state, zip, type_mortgage, first_company, months_behind, back_payments, notice_of_foreclosure, auction_date, date_of_auction, second_company, combined_balances, combined_payments, in_bankruptcy, situation, keep_property, referred_by ) VALUES ('$fname', '$lname', '$email', '$dayphone', '$altphone', '$besttime', '$address', '$city', '$state', '$zip', '$mortgage', '$first_mtg', '$months_behind', '$back_payments', '$notice', '$auction', '$auction_date', '$second_mortgage', '$balances', '$payments', '$bankruptcy', '$situation', '$keep_sell', '$referred')"; $results = mysql_query($sql, $link) or die(mysql_error()); $aff_rows = mysql_affected_rows($link); $new_id = mysql_insert_id(); if ($aff_rows == 1){ include 'header.inc'; show_results(); include 'footer.inc'; } if ($aff_rows <> 1) { include 'header.inc'; echo "<h3>Error</h3><br />There appears to be a problem submitting your data. Please review and correct it to try again.<br />\n"; show_form_params(); include 'footer.inc'; } ?> A quick note, the second function in the second 'if' statement displays completely different info. The show_results(); function shows the data that was inserted in the INSERT query. The show_form_params(); shows the form with the input fields populated with their input. The dual display is of the show_results(); function.
-
No problemo! <?php if ($aff_rows == 1){ include 'header.inc'; show_results(); include 'footer.inc'; } ?> Basically the premise is IF the INSERT query was successful then show the results of the function. If not, then there's another function that displays.
-
Hey, thanks for your post! We were on the same brain wave with that solution as it dawned on me that the 'while' loop needed to extract data, not insert. So, in a step ahead of your post, I modified the code to this: <?php // function for showing form submission results function show_results() { global $new_id; echo $new_id ."is your new ID"; echo "<h3>Request Sent</h3><br />\n"; echo "<font face='Verdana' size='2'>Your information has been sent. A representative will contact you shortly to discuss your current situation and your options based upon the information provided.<br />\n"; echo "Thank you for submitting your contact request!<br />\n"; echo "Cordially yours,<br />\n"; echo "David Corbaley</font><p>"; // run a query for this insert $query = mysql_query("SELECT * FROM leads WHERE id='$new_id'"); while ($row = mysql_fetch_array($query)){ echo "<table width='90%' border='0' cellpadding='2' align='center'> <tr><td colspan='2'><font face='Verdana' size='2'>The information you submitted:</td></tr> <tr><td>First name:</td><td>" . $row['first_name'] . "</td></tr> <tr><td>Last name:</td><td>" . stripslashes($row['last_name']) . "</td></tr> <tr><td>Email:</td><td>" . stripslashes($row['email']) . "</td></tr> <tr><td>Daytime phone:</td><td>" . stripslashes($row['day_phone']) . "</td></tr> <tr><td>Alternate phone:</td><td>" . stripslashes($row['alt_phone']) . "</td></tr> <tr><td>Best time to call:</td><td>" . stripslashes($row['best_time']) . "</td></tr> <tr><td>Address:</td><td>" . stripslashes($row['address']) . "</td></tr> <tr><td>City:</td><td>" . stripslashes($row['city']) . "</td></tr> <tr><td>State:</td><td>" . stripslashes($row['state']) . "</td></tr> <tr><td>Zip:</td><td>" . stripslashes($row['zip']) . "</td></tr> <tr><td>Type of mortgage:</td><td>" . stripslashes($row['type_mortgage']) . "</td></tr> <tr><td>1st Mortgage holder:</td><td>" . stripslashes($row['first_company']) . "</td></tr> <tr><td>Months behind:</td><td>" . stripslashes($row['months_behind']) . "</td></tr> <tr><td>Back payments:</td><td>" . stripslashes($row['back_payments']) . "</td></tr> <tr><td>NOD filed:</td><td>" . stripslashes($row['notice_of_foreclosure']) . "</td></tr> <tr><td>Auction date set:</td><td>" . stripslashes($row['auction_date']) . "</td></tr> <tr><td>Date of auction:</td><td>" . stripslashes($row['date_of_auction']) . "</td></tr> <tr><td>2nd Mortgage holder:</td><td>" . stripslashes($row['second_company']) . "</td></tr> <tr><td>Combined balances:</td><td>" . stripslashes($row['combined_balances']) . "</td></tr> <tr><td>Combined payments:</td><td>" . stripslashes($row['combined_payments']) . "</td></tr> <tr><td>Currently in bankruptcy:</td><td>" . $row['in_bankruptcy'] . "</td></tr> <tr><td>Situation details:</td><td>" . stripslashes($row['situation']) . "</td></tr> <tr><td>Keep or sell property:</td><td>" . $row['keep_property'] . "</td></tr> <tr><td>Referred by:</td><td>" . $row['referred_by'] . "</td></tr> </table>\n"; } } ?> Whereas I added the global statement for the $new_id variable that was set during the INSERT query, created a SELECT query pulling the data from the $new_id reference (WHERE id='$new_id') and now the invalid query statement is gone. BUT, I still get a complete repeat of the data being displayed. Check it out:
-
Here's the query code: <?php $link = mysql_connect($dbhost, $dbuser, $dbpass) or die(mysql_error()); mysql_select_db($dbname, $link) or die(mysql_error()); // run our query to insert the record $sql = "INSERT INTO leads (first_name, last_name, email, day_phone, alt_phone, best_time, address, city, state, zip, type_mortgage, first_company, months_behind, back_payments, notice_of_foreclosure, auction_date, date_of_auction, second_company, combined_balances, combined_payments, in_bankruptcy, situation, keep_property, referred_by ) VALUES ('$fname', '$lname', '$email', '$dayphone', '$altphone', '$besttime', '$address', '$city', '$state', '$zip', '$mortgage', '$first_mtg', '$months_behind', '$back_payments', '$notice', '$auction', '$auction_date', '$second_mortgage', '$balances', '$payments', '$bankruptcy', '$situation', '$keep_sell', '$referred')"; $results = mysql_query($sql, $link) or die(mysql_error()); $aff_rows = mysql_affected_rows($link); ?>
-
I have this function set to call when a form is successfully submitted. Basically it's supposed to display a confirmation/thanks message and the results of their form input. The problem is: 1. it's repeating itself in the display (See below for example) 2. I'm getting this 'invalid query' message for the 'while' function Here's the function code: <?php // function for showing form submission results function show_results() { echo "<h3>Request Sent</h3><br />\n"; echo "<font face='Verdana' size='2'>Your information has been sent. A representative will contact you shortly to discuss your current situation and your options based upon the information provided.<br />\n"; echo "Thank you for submitting your contact request!<br />\n"; echo "Cordially yours,<br />\n"; echo "David Corbaley</font><p>"; while ($row = mysql_fetch_array($results)){ echo "<table width='90%' border='0' cellpadding='2' align='center'> <tr><td colspan='2'><font face='Verdana' size='2'>The information you submitted:</td></tr> <tr><td>First name:</td><td>" . $row['first_name'] . "</td></tr> <tr><td>Last name:</td><td>" . stripslashes($row['last_name']) . "</td></tr> <tr><td>Email:</td><td>" . stripslashes($row['email']) . "</td></tr> <tr><td>Daytime phone:</td><td>" . stripslashes($row['day_phone']) . "</td></tr> <tr><td>Alternate phone:</td><td>" . stripslashes($row['alt_phone']) . "</td></tr> <tr><td>Best time to call:</td><td>" . stripslashes($row['best_time']) . "</td></tr> <tr><td>Address:</td><td>" . stripslashes($row['address']) . "</td></tr> <tr><td>City:</td><td>" . stripslashes($row['city']) . "</td></tr> <tr><td>State:</td><td>" . stripslashes($row['state']) . "</td></tr> <tr><td>Zip:</td><td>" . stripslashes($row['zip']) . "</td></tr> <tr><td>Type of mortgage:</td><td>" . stripslashes($row['type_mortgage']) . "</td></tr> <tr><td>1st Mortgage holder:</td><td>" . stripslashes($row['first_company']) . "</td></tr> <tr><td>Months behind:</td><td>" . stripslashes($row['months_behind']) . "</td></tr> <tr><td>Back payments:</td><td>" . stripslashes($row['back_payments']) . "</td></tr> <tr><td>NOD filed:</td><td>" . stripslashes($row['notice_of_foreclosure']) . "</td></tr> <tr><td>Auction date set:</td><td>" . stripslashes($row['auction_date']) . "</td></tr> <tr><td>Date of auction:</td><td>" . stripslashes($row['date_of_auction']) . "</td></tr> <tr><td>2nd Mortgage holder:</td><td>" . stripslashes($row['second_company']) . "</td></tr> <tr><td>Combined balances:</td><td>" . stripslashes($row['combined_balances']) . "</td></tr> <tr><td>Combined payments:</td><td>" . stripslashes($row['combined_payments']) . "</td></tr> <tr><td>Currently in bankruptcy:</td><td>" . $row['in_bankruptcy'] . "</td></tr> <tr><td>Situation details:</td><td>" . stripslashes($row['situation']) . "</td></tr> <tr><td>Keep or sell property:</td><td>" . $row['keep_property'] . "</td></tr> <tr><td>Referred by:</td><td>" . $row['referred_by'] . "</td></tr> </table>\n"; } } ?> Here's what it displays after submitting the form: Line 143 is the 'while' statement. Notice how it repeats the output? That's a mystery. Plus, i'm fuzzy on the query issue. This function is contained in an 'include' file which the main script contains ( include 'db_config.inc'). Which, if i'm correct, would allow the function's 'while' loop to work off the query that's in the main script which posts the input into variables and inserts them into the database. That may be the problem as it may not allow me to handle the while loop in that fashion?
-
getting error message with mysql_affected_rows..not sure why
simcoweb replied to simcoweb's topic in PHP Coding Help
Thanks for the post! That took care of that part. But, as with most coding efforts, now a new issue has arisen from its ashes! Basically, using the same code i've posted already regarding the INSERT of the data, i'm attempting to then display the results, if successful, on the next page. What i'm getting, though, is a duplicate display of the code. In other words, it's displaying the results twice vertically. Here's the code snippets in question. First, in the form processing script i'm using an if/else to summon a function that displays the form results in a table. Like so: <?php $results = mysql_query($sql, $link) or die(mysql_error()); $aff_rows = mysql_affected_rows($link); if ($aff_rows == 1){ include 'header.inc'; show_results(); include 'footer.inc'; } else { include 'header.inc'; echo "<h3>Error</h3><br />There appears to be a problem submitting your data. Please review and correct it to try again.<br />\n"; show_form_params(); include 'footer.inc'; } ?> The basics here is that IF the query was successful and IF at least one affected row proves that then run the code and display the contents of the show_results() function which is this code: <?php // function for showing form submission results function show_results() { echo "<h3>Request Sent</h3><br />\n"; echo "<font face='Verdana' size='2'>Your information has been sent. A representative will contact you shortly to discuss your current situation and your options based upon the information provided.<br />\n"; echo "Thank you for submitting your contact request!<br />\n"; echo "Cordially yours,<br />\n"; echo "David Corbaley</font><p>"; while ($row = mysql_fetch_array($results)){ echo "<table width='90%' border='0' cellpadding='2' align='center'> <tr><td colspan='2'><font face='Verdana' size='2'>The information you submitted:</td></tr> <tr><td>First name:</td><td>$fname</td></tr> <tr><td>Last name:</td><td>" . stripslashes($row['last_name']) . "</td></tr> <tr><td>Email:</td><td>" . stripslashes($row['email']) . "</td></tr> <tr><td>Daytime phone:</td><td>" . stripslashes($row['day_phone']) . "</td></tr> <tr><td>Alternate phone:</td><td>" . stripslashes($row['alt_phone']) . "</td></tr> <tr><td>Best time to call:</td><td>" . stripslashes($row['best_time']) . "</td></tr> <tr><td>Address:</td><td>" . stripslashes($row['address']) . "</td></tr> <tr><td>City:</td><td>" . stripslashes($row['city']) . "</td></tr> <tr><td>State:</td><td>" . stripslashes($row['state']) . "</td></tr> <tr><td>Zip:</td><td>" . stripslashes($row['zip']) . "</td></tr> <tr><td>Type of mortgage:</td><td>" . stripslashes($row['type_mortgage']) . "</td></tr> <tr><td>1st Mortgage holder:</td><td>" . stripslashes($row['first_company']) . "</td></tr> <tr><td>Months behind:</td><td>" . stripslashes($row['months_behind']) . "</td></tr> <tr><td>Back payments:</td><td>" . stripslashes($row['back_payments']) . "</td></tr> <tr><td>NOD filed:</td><td>" . stripslashes($row['notice_of_foreclosure']) . "</td></tr> <tr><td>Auction date set:</td><td>" . stripslashes($row['auction_date']) . "</td></tr> <tr><td>Date of auction:</td><td>" . stripslashes($row['date_of_auction']) . "</td></tr> <tr><td>2nd Mortgage holder:</td><td>" . stripslashes($row['second_company']) . "</td></tr> <tr><td>Combined balances:</td><td>" . stripslashes($row['combined_balances']) . "</td></tr> <tr><td>Combined payments:</td><td>" . stripslashes($row['combined_payments']) . "</td></tr> <tr><td>Currently in bankruptcy:</td><td>" . $row['in_bankruptcy'] . "</td></tr> <tr><td>Situation details:</td><td>" . stripslashes($row['situation']) . "</td></tr> <tr><td>Keep or sell property:</td><td>" . $row['keep_property'] . "</td></tr> <tr><td>Referred by:</td><td>" . $row['referred_by'] . "</td></tr> </table>\n"; } } ?> Now, 1st, the function code produces an error that the while loop is an invalid mysql query. 2nd, it still displays the HTML without the results but, as I mentioned, it repeats itself. It displays the text message, the table with the names of the fields, no results of those fields though....then displays it all again just below that. Ideas anyone? -
Here's my code: <?php // run our query to insert the record $sql = "INSERT INTO leads (first_name, last_name, email, day_phone, alt_phone, best_time, address, city, state, zip, type_mortgage, first_company, months_behind, back_payments, notice_of_foreclosure, auction_date, date_of_auction, second_company, combined_balances, combined_payments, in_bankruptcy, situation, keep_property, referred_by ) VALUES ('$fname', '$lname', '$email', '$dayphone', '$altphone', '$besttime', '$address', '$city', '$state', '$zip', '$mortgage', '$first_mtg', '$months_behind', '$back_payments', '$notice', '$auction', '$auction_date', '$second_mortgage', '$balances', '$payments', '$bankruptcy', '$situation', '$keep_sell', '$referred')"; $results = mysql_query($sql) or die(mysql_error()); $aff_rows = mysql_affected_rows($results); if ($aff_rows == 1){ include 'header.inc'; show_results(); include 'footer.inc'; } ?> I'm getting this error message when running this: with line 65 being the $aff_rows = mysql_affected_rows($results) It's gotta be something obvious but I need a new set of eyes to find it
-
Try r+ Read/Write. Starts at the beginning of the file.
-
In that case, having a PHP login script is pointless. In other words, it doesn't accomplish anything because all it would do is pull up the same ugly gray box. If that's the method they use then not much you can do with it.
-
You're better off using a database method of login instead of the .htaccess Apache method. That way you use a nice looking login form instead of that ugly gray box.
-
I'd take this part out of the 'while' loop: <?php echo "<table width='100%' border='0' bgcolor=#CCCCCC cellpadding='2' cellspacing='1'> <tr> <td width='135'><font size=1.5><b>$makes[$current]</b></font></td> <td width='55'><font size=1.5><b>DISP</b></font></td> <td width='60'><font size=1.5><b>FUEL</b></font></td> <td width='55'><font size=1.5><b>CLASS</b></font></td> <td width='110'><font size=1.5><b>EMISSION STD</b></font></td> <td width='90'><font size=1.5><b>TEST GROUP</b></font></td> <td width='90'><font size=1.5><b>EO NUMBER</b></font></td> </tr> ?> Otherwise it will repeat in the while loop. I'm assuming you just want those to be the titles at the top of the fields/columns. Then this part: <? //Populate the Tables from the Database echo "<tr class=\"$class\">\n"; echo strtoupper("<td width='135'><font size=1.5>$row['sub_model']</font></td>\n"); echo "<td width='55'><font size=1.5>".sprintf( '%01.1f',$row['disp'])." L</font></td>\n"; echo "<td width='60'><font size=1.5>".$fuel_types[$row['fuel']]."</font></td>\n"; echo "<td width='55'><font size=1.5>$row['veh_class']</font></td>\n"; echo "<td width='110'><font size=1.5>$row['arb_std']</font></td>\n"; echo "<td width='90'><font size=1.5>$row['test_group']</font></td>\n"; echo "<td width='90'><font size=1.5>$row['eo']</font></td>\n"; echo "</tr>\n"; ?> would be in the while loop so it displays as many rows as needed for the results in your query. Also, I didn't notice the closing </table> tag. You need to echo that after your closing } of the while loop. echo "</table><br />\n";
-
This is bugging the hell out of me. This page contains a form that is supposed to insert input based to the MySQL database after it's inserted and the submit button is pressed. Instead it's submitting blank data upon page load. I can't see where it's doing this. I need some new sets of eyes to look this over. Here's the PHP code which validates if there's a valid record for that date. If not, then it allows the user to post the data. What I get instead is all fields blank except the id which is auto-incremented and the date which is set in the variables and posted automatically. I need to stop this form from submitting upon page load. <? $v_sbm=$_REQUEST['submit']; $connection = mysql_connect($dbhost, $dbusername, $dbpass); $SelectedDB = mysql_select_db($dbname); // set our POST variables $step1 = mysql_real_escape_string($_POST['step1']); $step2 = mysql_real_escape_string($_POST['step2']); $step3 = mysql_real_escape_string($_POST['step3']); $step4 = mysql_real_escape_string($_POST['step4']); $details = mysql_real_escape_string($_POST['details']); $opentrades = mysql_real_escape_string($_POST['opentrades']); $date = date("m/d/Y"); $err = ""; // validate data /* if(trim($step1)== "") { $err .="Step One cannot be blank.<br>"; } if(trim($step2)== "") { $err .="Step Two cannot be blank.<br>"; } if(trim($step3) == "") { $err .="Step Three cannot be blank.<br>"; } if(trim($step4) == "") { $err .="Step Four cannot be blank.<br>"; } if (!$err) { */ // check to see if today's date already exists in picks' $check = "SELECT * FROM picks WHERE date='$date'"; $results = mysql_query($check) or die(mysql_error()); $num_rows = (mysql_num_rows($results)); if ($num_rows >= 1) { echo "There is already a pick for this date. You can not add another but you can edit the pick by choosing Edit Today's Picks in the menu on the left.<br/>\n"; exit(); } else { $sql = "INSERT INTO picks (step1, step2, step3, step4, details, opentrades, date) VALUES ('$step1', '$step2', '$step3', '$step4', '$details', '$opentrades', '$date')"; $results = mysql_query($sql) or die(mysql_error()); if(!results ) { echo "Could not enter new picks into database. Please re-enter.<br>\n"; } else { if (isset($_POST['submit'])) { echo "New picks successfully entered for $date.<br>\n"; echo "<ul><li>Step 1: $step1</li>\n <li>Step 2: $step2</li>\n <li>Step 3: $step3</li>\n <li>Step 4: $step4</li>\n <li>Details: $details</li>\n <li>Open Trades: $opentrades</li>\n </ul><br>\n"; } } } //} ?> Just a note, the form field validation was commented out on purpose. The client didn't want the fields required.
-
That's what is causing that orphaned } then. Nice spot
-
This bottom } is 'orphaned' meaning it either doesn't belong and it's one too many or you had it there to close an if statement that isn't set up right. if ($rsUpdate) { header("Location: welcome.php"); // redirects members to a welcome member page } }
-
Also, a word to the wise, don't post your database access parameters for the world to see
-
mysql_fetch_array(): not a valid mysql result resource
simcoweb replied to scottreid1974's topic in PHP Coding Help
btherl is onto something here. First 'no database selected' is not a standard mysql_error() message. Therefore that message has to be coming from some echo statement in your script you're using in your login system which is basically stating you don't have your database parameters inputted correctly where they are supposed to be for the script to read them when trying to connect. Copy and paste this into a text file, call it db_test.php, upload it to your public_html folder and then summon it in your browser. Let us know what it says. <?php $host = "localhost"; $user = "gvs_abc"; $pass = "password"; // make sure this is correct! $db = "gvs_abc"; $dbh=mysql_connect ($host, $user, $pass) or die ('I cannot connect to the database because: ' . mysql_error()); $dbase = mysql_select_db ($db); $sql = "SHOW TABLES"; $results = mysql_query($sql) or die(mysql_error()); echo $results; ?> If your database is truly there and truly has tables in it then this will display that and since it's not reliant upon any other scripts then it should either display a mysql_error OR the results. BE SURE that you edit the $pass variable! Wasn't sure of your password -
You'll need to ask specific questions and post what code you have that may be producing errors or not producing what you need. Then we're glad to help.
-
You mean the difference between an 'absolute' and a 'relative' url/source? Absolute: http://www.yoursite.com/imagefolder/nameofimage.jpg Relative: nameofimage.jpg or ./imagefolder/nameofimage.jpg etc.
-
Ok, cool. Rock on and thanks!
-
Thanks for the post. Ok, so if i'm understanding correctly, i'd have to do it this way? <?php echo "<ul><li>Step 1: stripslashes($step1)</li> <li>Step 2: " . stripslashes($step2) . "</li> <li>Step 3: " . stripslashes($step3) . "</li> <li>Step 4: " . stripslashes($step4) . "</li> <li>Details: " . stripslashes($details) . "</li> <li>Extra Pick #1: " . stripslashes($extra_pick1) . "</li>\n <li>Extra Pick #2: " . stripslashes($extra_pick2) . "</li>\n <li>Extra Pick #3: " . stripslashes($extra_pick3) . "</li>\n <li>Extra Pick #4: " . stripslashes($extra_pick4) . "</li>\n </ul><br>\n"; ?>
-
Should be simple question but just want to make sure since i've only used stripslashes in a different manner of output. Here's what I have in mind. This list will be echoed to the browser showing updated information. I'm using mysql_real_escape_string for the insertion of the data and want to strip the slashes for this output: <?php echo "<ul><li>Step 1: stripslashes($step1)</li> <li>Step 2: stripslashes($step2)</li> <li>Step 3: stripslashes($step3)</li> <li>Step 4: stripslashes($step4)</li> <li>Details: stripslashes($details)</li> <li>Extra Pick #1: stripslashes($extra_pick1)</li>\n <li>Extra Pick #2: stripslashes($extra_pick2)</li>\n <li>Extra Pick #3: stripslashes($extra_pick3)</li>\n <li>Extra Pick #4: stripslashes($extra_pick4)</li>\n </ul><br>\n"; ?> It looks funky in my PHP editor in regards to the color coding.
-
[SOLVED] Getting Data From Database And Turning Into Variables?
simcoweb replied to Muncey's topic in PHP Coding Help
The display of the guestbook entries is done using a MySQL query then displaying the data by parsing HTML in the output. I would assume this is done using a 'while' loop so it displays all the entries of your guestbook (or so many per page). So, in that query it's probably asking for all the info in the guestbook entries like this: "SELECT * FROM guestbooks"; Then display it with a while look and in the HTML output you'd specify where their ID would be located in your HTML $results = mysql_query($sql); while ($row = mysql_fetch_array($results) { echo "<table><tr> <td>" . $row['member_id'] . "</td><td>" . $row['member_name'] . "</td> </td> </table><br/>\n"; } -
You can use a SESSION variable or pass it in the url. Then just echo it: > if passed in the URL $username = $_GET['username']; echo "Welcome back" .$username ; It's not real secure to do it that way, though, since the username is now part of the display of the url in the address bar. I'm sure someone has a better more secure way.