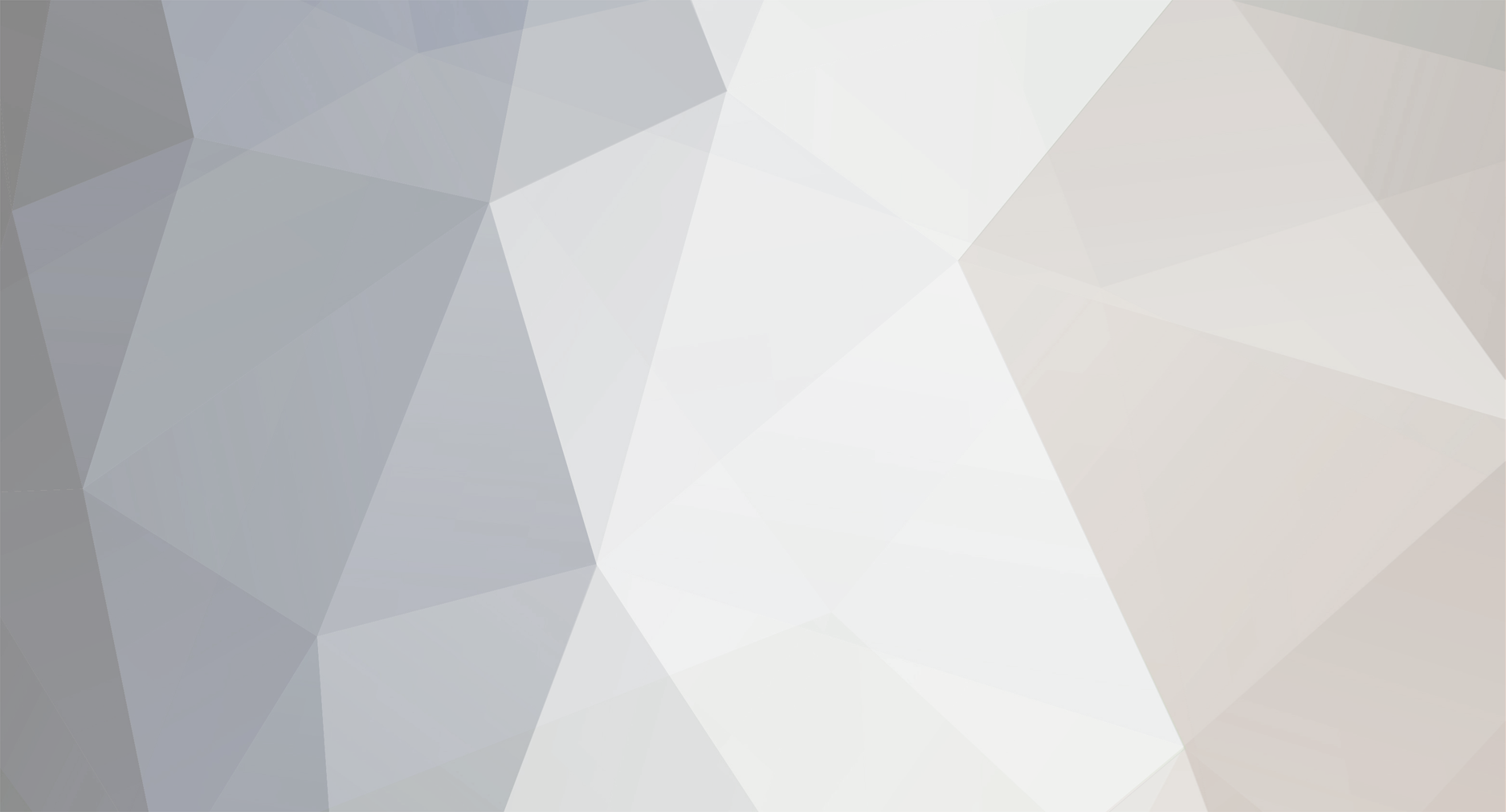
simcoweb
Members-
Posts
1,104 -
Joined
-
Last visited
Everything posted by simcoweb
-
How is that $username variable getting populated? Is there a form they complete that points to this script that asks for it? Or do they click on a link of some sort after they log in something like ' Click here to view your info' or similar?
-
I believe that should be written like this: $id = $_GET['id]; $first = "SELECT * FROM users WHERE id='$id'"; The $_GET snags the id from the url assuming you're passing the id in the url like this: http://www.yoursitename.com/somefile.php?id=XXXXX
-
I mean a query to the database. You have to summon the data first then echo the data in the page. Post your MySQL query code so we can take a look at it.
-
For it to 'echo' you need to run a query. Do you have a query code established?
-
I've been cruising right along in creating two contact forms and scripts that parse the forms, send emails to the respective destinations AND insert the data into a MySQL database. The 'contact' form works fine. The 'schedule an appointment' form works but does not insert the selected items into the database. And, i'm not sure how to include the results of those fields into the email message. Here's the form code: [code]<?php echo <<<EOD <form method="POST"> <table class="dl" cellpadding="5" cellspacing="1" width="95%" align="center"> <tr> <td class="head" align="center" colspan="2">Schedule An Appointment</td> </tr> <tr> <td class="body" align="left">Name</td> <td class="body" align="center"><input type="text" name="name" value="{$_POST['name']}" /></td> </tr> <tr> <td class="body" align="left">Email Address</td> <td class="body" align="center"><input type="text" name="email" value="{$_POST['email']}" /></td> </tr> <tr> <td class="body" align="left">Phone</td> <td class="body" align="center"><input type="text" name="phone" value="{$_POST['phone']}" /></td> </tr> <tr> <td class="body" align="left">Appointment is for:</td> <td class="body" align="center"><select value="appt_for[]"> <option value="Myself">Myself</option> <option value="My Son">My Son</option> <option value="My Daughter">My Daughter</option> <option value="Other">Other</option> </select></td> </tr> <tr> <td class="body" align="left">Best Days For You (select all that apply):</td> <td class="body" align="center"><input type="checkbox" name="best_days[]" value="Monday">Monday <input type="checkbox" name="best_days[]" value="Tuesday">Tuesday <br> <input type="checkbox" name="best_days[]" value="Wednesday">Wednesday <input type="checkbox" name="best_days[]" value="Thursday">Thursday <br> <input type="checkbox" name="best_days[]" value="Friday">Friday <input type="checkbox" name="best_days[]" value="Saturday">Saturday </td> </tr> <tr> <td class="body" align="left">Best Time For You:</td> <td class="body" align="center"><select value="best_time"> <option value="Before Noon" selected name="best_time[]">Before Noon</option> <option value="Afternoon before 5" name="best_time[]">Afternoon before 5</option> <option value="Evenings after 5" name="best_time[]">Evenings after 5</option> </select> </td> </tr> <tr> <td class="body" align="center" colspan="2">Additional Details:<br><textarea name="message" rows="8" cols="50">{$_POST['message']}</textarea></td> </tr> <tr> <td class="body" align="center" colspan="2"><img src="securimage_show.php"></td> </tr> <tr> <td class="body" align="left">Enter the security text shown above</td> <td class="body" align="center"><input type="text" name="imagetext" /></td> </tr> <tr> <td class="body" align="center" colspan="2"><input type="submit" name="submit" value="Send Form" /></td> </tr> </table> </form> EOD; ?>[/code] I have two 'SELECT' items and one 'MULTIPLE' item in checkboxes. I know how to write the code if I wish to print those to the browser (with a foreach statement) but not sure how to adapt these choices into insertion for the database. Right now, upon submit, those three fields produce this: appt_for: blank best_days: array (the word array) best_time: blank And, here's the code for the email message: [code]<?php @mail($to, "Appointment request for " . $_POST['subject'], "Drew,\nOn " . date("r") . ", " . $_POST['name'] . " " . $_POST['email'] . " " . $_POST['phone'] ." sent the following message.\nWe need an appointment for " . $_POST['appt_for'] . " The best days for them are " .$_POST['best_days']. ". The best times are " .$_POST['best_time']. " Here are further instructions \n\n" . stripslashes($_POST['message']), "From: " . $_POST['email']); ?>[/code] So, how to get those form elements to insert into the database and how to get the results of those form elements into the message. Thanks in advance for you help!
-
Here's an example of form field validation from a form I use: [code]<?php // validate input and if form is already submitted once if (!isset($_POST['submit'])) { showForm(); } else { //form submitted $error = 0; if(empty($_POST['name'])) { $error = 1; $errstr[] = "Please enter a name"; } if(!preg_match("/^(?:[\w\d]+\.?)+@(?:(?:[\w\d]\-?)+\.)+\w{2,4}$/", $_POST['email'])) { $error = 1; $errstr[] = "Please enter a valid email address"; } if (empty($_POST['subject'])) { $error = 1; $errstr[] = "Please enter a subject"; } if(empty($_POST['message']) || preg_match("/^enter your message here$/i", $_POST['message'])) { $error = 1; $errstr[] = "Please enter a message or question so we can assist you."; } if(empty($_POST['imagetext'])) { $error = 1; $errstr[] = "Please validate the image code"; } else { include "securimage.php"; $img = new securimage(); $valid = $img->check($_POST['imagetext']); if(!$valid) { $error = 1; $errstr[] = "The code you entered was incorrect"; } } if ($error == 1) { echo "<center>\n<font style=\"color: #FF0000\">\n"; foreach($errstr as $err) { echo "<li> " . $err . "</li>\n"; } echo "</font>\n</center>\n<br />\n\n"; showForm(); } ?>[/code]
-
It helps if you use the [code] [/code] tags in posting your code. That way we can read it. Once we get that out of the way we can assist with the Captcha
-
CSS isn't utilized by PHP. You can, though, use CSS in the HTML that your PHP script outputs. For example, say you create a 'header' file for the top of your page that contains HTML and the standard HTML tags like <head> and so on. You'd place your CSS link tag in the <head> section like you would normally. Then call the header into your scripts like: include 'header.php'; Then, say you want to have some HTML mixed into your script: echo "<table><tr><td class='[b]yourCSSclasshere[/b]'"; echo "<font class='[b]yourCSSclasshere[/b]'>blah blah blah</font>"; echo "</td></tr></table>\n"; Like that.
-
newbie on php, needs help with the "get"- function
simcoweb replied to xprez's topic in PHP Coding Help
yeah, i'm not sure what that is. But, if you want to get a count then you'd have to do a query of some sort that tells you how many results there are. Like this: [code]<?php $sql = "SELECT * FROM categories"; $results = mysql_query($sql); $num_rows = mysql_num_rows($results); echo "There are $num_rows ads in this category."; ?>[/code] That will return the number of results. -
This is the easiest pagination code i've ever worked with. First an example of how it's laid out in a script: [code]<?php // product display page session_start(); include 'db-config.inc.php'; include 'header.inc.php'; include 'db_cart_functions.php'; db_connect(); // If current page number, use it // if not, set one! if(!isset($_GET['page'])){ $page = 1; } else { $page = $_GET['page']; } // Define the number of results per page $max_results = 20; // Figure out the limit for the query based on the current page number. $from = (($page * $max_results) - $max_results); $sql = "SELECT * FROM products ORDER BY id LIMIT $from, $max_results"; $results = mysql_query($sql) or die(mysql_error()); $num_rows = mysql_num_rows($results); // output our display of the products echo "<table width='600' border='0' align='center'>"; echo "<tr><td align='center'>There are $num_rows product available.\n</td></tr>"; echo "<tr><td>"; // Build Page Number Hyperlinks echo "<center>Select a Page<br /><br />"; for($i = 1; $i <= $total_pages; $i++){ echo " | "; if(($page) == $i){ echo "Page $i "; } else { echo "<a class=\"body\" href=\"".$_SERVER['PHP_SELF']."?page=$i\">Page $i</a> "; } } echo "</center><p>\n"; echo "</td></tr>"; // loop through our results while ($row = mysql_fetch_array($results)) { echo "<tr> <td>".$row['image']."</td><td>".$row['product_name']."<br>".$row['product_desc']."</td> <td><form action='addtocart.php?product_id=$product_id' method='POST'><input type='text' name='quantity' size='4'> <input type='submit' name='addtocart' value='Add To Cart'></form></td> </tr>"; } echo "</table>\n"; include 'footer.php'; ?>[/code] The way it's set up is to display at the top of the results, centered. And now just the pagination code so you can have it separate: [code]// If current page number, use it // if not, set one! if(!isset($_GET['page'])){ $page = 1; } else { $page = $_GET['page']; } // Define the number of results per page $max_results = 75; // Figure out the limit for the query based on the current page number. $from = (($page * $max_results) - $max_results); //SQL clause ORDER BY id LIMIT $from, $max_results // Build Page Number Hyperlinks echo "<center>Select a Page<br /><br />"; for($i = 1; $i <= $total_pages; $i++){ echo " | "; if(($page) == $i){ echo "Page $i "; } else { echo "<a class=\"body\" href=\"".$_SERVER['PHP_SELF']."?page=$i\">Page $i</a> "; } } echo "</center><p>\n";[/code]
-
Need input on shopping cart using points for currency
simcoweb replied to simcoweb's topic in PHP Coding Help
Thanks for the advice, HotDawg. I do prefer to code the majority of it myself. However, I am under some time constraints and having perhaps a well written class file handling the basic cart functions would be a big boost. As i've been told in these forums many time, 'don't try to reinvent the wheel'. :) Thanks to these forums and lots of great responses to my other posts i've been able to go from total zilch to about a 5.5 within a short period of time. With help/guidance/suggestions from many of these great people i've successfully written two standalone projects plus a complete website in PHP that included registration, logins, referral tracking, file uploading, profile updating and much more. I read the forums daily to see if I can answer the questions that others post. If I can then I know that i'm learning! :) It's always good to get some ideas on the fastest and most efficient way to code something. I guess that's what i'm looking for here. That way, like in your case, I don't spend a lot of time spinning my wheels. With the last project I posted here I was able to get solid advice on the right way to set up the database (using multiple tables and 'join' queries instead of one table, for example). Anyway, thanks again! -
Starting a new project whereas the products will be purchased utilizing 'loyalty points' for purchasing instead of money. Points totals are generated by another site and software. All I need to do is tap into their points totals and create a cart that subtracts what they spend on merchandise and updates the total to reflect that. The cart will be member's only (it's a gaming site) and the products are more like 'gifts' since there's no currency exchanged. And, most likely, no shipping charged. They will need to log in using user/pass established at their gaming site. So, questions are..should I try to take an existing cart and redo the payment/checkout system to reflect points as currency? Or, create something from scratch since it's going to be a basic 'catalog' style with minimal full-featured cart modules in it? Does anyone know of a set of classes that could provide the cart functionality so I can just add in the payment/checkout method? Any ideas/pointers/etc. would be greatly appreciated! :)
-
Can you post ALL the code so we can also see your query?
-
Umm..that's not all the code. Only 47 lines there. :)
-
Help with a user database looking for some good tutorials
simcoweb replied to wtfsmd's topic in PHP Coding Help
Have you looked at http://www.phpfreaks.com/tutorials/ ? -
Yep, you can use the include function. :)
-
There's a Photoshop plugin you can download for the .ico format. I don't have the url but you can Google it.
-
That's a 'favicon'. http://favicon.com/
-
Ok, I think I get it now. Not sure why you want to save it to a text file but hey... :) The text file can store anything. It's just text. So, you'd have to write your code in a way that the variable name is part of the variable value you're writing to the file. Sorta like this (not sure if this is syntax perfect but let's pretend it's logical) [code]<?php $myFile = "testFile.txt"; $fh = fopen($myFile, 'w') or die("can't open file"); $yourVariable = "\$yourVariable = Bobby Bopper\n"; fwrite($fh, $yourVariable); $yourVariable2 = "\$yourVariable2 = Tracy Tanner\n"; fwrite($fh, $yourVariable2); fclose($fh); ?>[/code] You have to escape the dollar sign so it doesn't act like a variable signifier.
-
Sorry, still a bit fuzzy on this. Ok, so you're running a MySQL query in order to populate the variables...correct? And what you want to do is write the actual variable name and value to a text file? Like: $thisismyvariable = "value"; So you want that to be written to the text file, correct?
-
How are you gathering the values for your variables? Are they coming from a form?
-
It should work if you change this line as follows: echo "The Id is $id and the text is $testfield";
-
I'm not sure what you're trying to accomplish but the simplest way might be to use CSS then just place the class name into your output in the script.
-
Can you post the code, pleasae.
-
[SOLVED] New User PHP script ( thanks for all your help )
simcoweb replied to PC Nerd's topic in PHP Coding Help
Not sure what kind of server you're on but if it's Unix it won't allow this blank space in the path to your include file include("inc files\Database_link.inc"); Check that to make sure it doesn't contain an underscore between the inc and files.