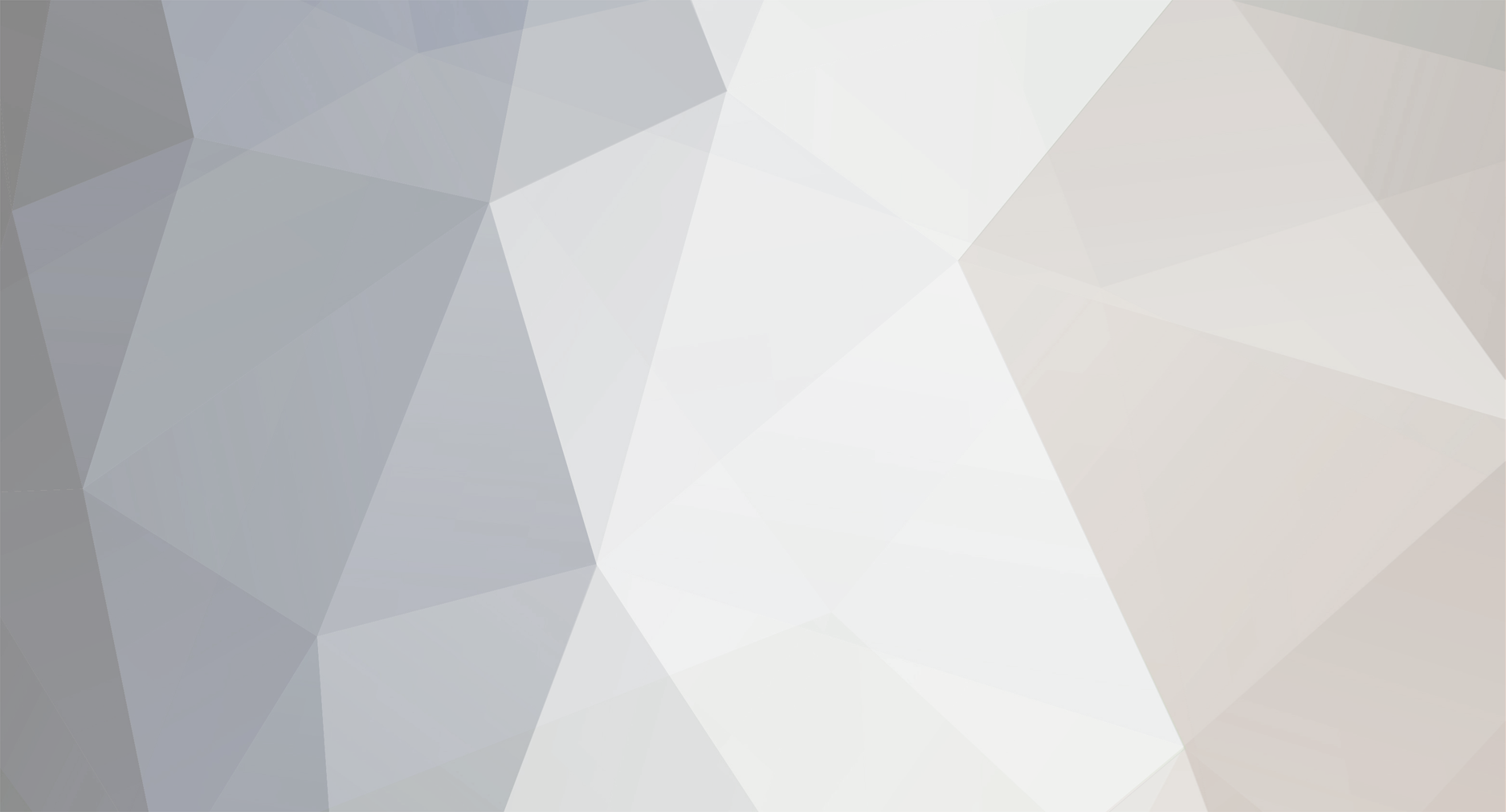
simcoweb
Members-
Posts
1,104 -
Joined
-
Last visited
Everything posted by simcoweb
-
So I understand...you're echo'ing the text INTO a text area? So it's populating the text area form field as if someone was going to edit it? Like in a profile, for example?
-
What mode is your field in the mysql database? Is the textarea field set as 'text'?
-
Cool! :) Now that you have the 'basic' functionality in there and it works, you should seriously consider some code to protect against malicious files being uploaded.
-
There's a couple of things to do in order to get the actual name into the database. This should work: add this line into your code: [code]$imagename = basename($_FILES['Picture']['name']);[/code] Then in your INSERT query change the reference for the picture to simply: '$imagename' Basically what we've done is set a variable populated with the image's actual name. Then, in your query, it should use that for populating the database field. In your pages where you want the image to show you'd use a <img src tag like usual but like this: <img src='http://www.yoursitename.com/filedir/$imagename'>
-
Yeppers. That can be done in the form or/also in the PHP with 'if' validation.
-
You're missing a whole bunch of code relative to the uploading and moving of the image. [code]<?php $target_path = "../Database/pics"; $target_path = $target_path . basename( $_FILES['Picture']['name']); if(move_uploaded_file($_FILES['Picture']['tmp_name'], $target_path)) { echo "The file ". basename( $_FILES['Picture']['name']). " has been uploaded"; } else{ echo "There was an error uploading the file, please try again!"; } ?>[/code] This moves the temporary image location (which is what you're getting) into a permanent location into your /pics folder. This does not provide any validation of the image or its extension. You should include that as well or someone could upload something ugly to your site.
-
Could you use the printf function for this as well?
-
How would I create random display of multiple fields
simcoweb replied to simcoweb's topic in PHP Coding Help
Ok, worked like a charm. Thanks! -
How would I create random display of multiple fields
simcoweb replied to simcoweb's topic in PHP Coding Help
Ok, but do I need to do anything with the $row_array[] variable as I pointed out? -
How would I create random display of multiple fields
simcoweb replied to simcoweb's topic in PHP Coding Help
My bad, that query isn't from my database. That's from the tutorial. My query would be more like: "SELECT * FROM testimonials" What I want to do is display one random testimonial at a time on various pages. -
I'm doing a sort of 'random quote' but it's going to display 5 items instead of just 1 and will be pulling from a MySQL database instead of a text file. With that said, I visited the tutorials here and found one that shows how to pull the one field ['messages'] but I need to pull these: name headline message photo date The snippet of code from the tutorial is: [code]<? $cnx = mysql_connect("localhost", "root", ""); mysql_select_db("irclog", $cnx); $sql = mysql_query("SELECT message FROM irclog") or die (mysql_error()); while($row = mysql_fetch_array($sql)){ $row_array[] = $row['message']; } mysql_close($cnx); $random_row = $row_array[rand(0, count($row_array) - 1)]; echo $random_row; ?> [/code] I have the mysql stuff, no problem. The fetching of the array pulls just the $row['message'] field. I'm sure that's where I need to expand and i'm assuming I would do it sort of like this: $row_array[] = $row['name'], $row['headline'], $row['photo'], $row['message'], $row['date']; Then the remaining code would stay the same. Would this be the proper way?
-
Voila! That was the missing snippet. I opted for the comma separated list. Thanks for that. Another related question. Once the form parses it sends the contents to the site owner in mail() function. I would like to adapt that same principle to the output of the mail. Here's what I have now: [code]@mail($to, "Appointment request for " . $_POST['subject'], "Drew,\nOn " . date("r") . ", " . $_POST['name'] . " " . $_POST['email'] . " " . $_POST['phone'] ." sent the following message.\nNeed an appointment for " . $_POST['appt_for'] . " The best days for them are " .$_POST['best_days']. ". The best times are " .$_POST['best_time']. " Here are further instructions \n\n" . stripslashes($_POST['message']), "From: " . $_POST['email']);[/code] Which basically isn't parsing that array for 'best_days'. Would I adapt the same snippet to this in order to have it display the days? Right now it just comes up with the word 'Array'.
-
I've got the best time and appointment for fields working fine and inserting proper selections in the database. The one that is still funky is the 'best_days' field which is the checkboxes. All it does is insert the word 'Array' into the database instead of the actual selections. My query looks like this: mysql_connect($dbhost, $dbuser, $dbpass) or die(mysql_error()); mysql_select_db($dbname) or die(mysql_error()); $sql = "INSERT INTO schedule VALUES ('', '$name', '$email', '$phone', '$appt_for', '$best_days', '$best_time', '$message', '$date')"; $results = mysql_query($sql) or die("Error occurred: ".mysql_error());
-
I'm assuming that the page before the landing page with the link to the report.php IS the login page? And...that the username field is actually named 'username'?
-
Ok, let's do this. We need to make sure the $username variable is actually being passed. On the reports.php page add this below your $username = $_GET['username']; line. [code] if (isset($username)) { echo "This is the dang username: $username"; } else { echo "There is nothing in this variable"; } [/code] If there's nothing there then the username isn't being passed which would explain why there's no results.
-
Ok, I noticed an error in your query. You have this: [code]("SELECT * FROM report WHERE username='$username';")[/code] The ; needs to be after the double quotes " like this: [code]"SELECT * FROM report WHERE username='$username' ";[/code] You don't really need the braces either. Try that.
-
Did you place the $username = $_GET['username']; on your Reports page?
-
Bummer. Those 3 fields are just NOT populating the variables. I also have the results being inserted into the MySQL database. For those three fields I get: 1. best_days (instead of the choice(s) ) 2. Array (just the word Array) 3. blank ( as in nothing ) All the other variables are fine. Name, email, phone, message. But, those three multiple selections just ain't doin it. Ideas?
-
First, thanks for the posts. I'm a bit fuzzy still. I hear (read) what you're saying but I don't comprehend totally since there's 2 different field types. Let's break it down for me if you would please. On the checkboxes, that is a multiple choice. So, first... setting up the form properly so that the selections are all passed to the results. I have one $best_days = $_POST['best_days']; but there could be multiple selections for that field. Will the variable hold all the selections? And, you mentioned changing the names to match the values plus eliminating the []'s. Here's a snippet of my code to use as an example. Can you show me exactly how you'd change it please? [code]<tr> <td class="body" align="left">Best Days For You (select all that apply):</td> <td class="body" align="center"><input type="checkbox" name="best_days[]" value="best_days">Monday <input type="checkbox" name="best_days[]" value="best_days">Tuesday <br> <input type="checkbox" name="best_days[]" value="best_days">Wednesday <input type="checkbox" name="best_days[]" value="best_days">Thursday <br> <input type="checkbox" name="best_days[]" value="best_days">Friday <input type="checkbox" name="best_days[]" value="best_days">Saturday </td> </tr>[/code] Then the drop down selectors, here's the snippet for that: [code]<tr> <td class="body" align="left">Appointment is for:</td> <td class="body" align="center"><select name="appt_for[]" value="appt_for"> <option value="appt_for">Myself</option> <option value="appt_for">My Son</option> <option value="appt_for">My Daughter</option> <option value="Other">Other</option> </select></td> </tr>[/code] Thanks in advance!
-
Sure. Let's go through the logical steps. 1. person logs in using username/password combo 2. if successful, they get to the 'landing' page which contains this link to their report 3. if they click on the link you want it to summon the data for that username If these 3 steps are correct then on the 'landing' page you need to set the $username variable so it can be used again in our link to the report page. So, on the landing page have the variable $username = $_POST['username']; (if that's the login form field name. If not, then change to what your form field name is). Now, you have the $username variable populated when they log in. So, change your link to the report page by just adding the ?username=$username The ? is a operand that PHP recognizes to snag info from the url being passed using the $_GET statement. So, say i've logged in as bozotheclown. When I click on my link to the reports page it will pass my username onto the next page (the reports page and subsequent query display) where you would have the query set up to work off the person's username. Like this: View Your Report! ( report.php?username=bozotheclown would be the actual hyperlink). On the report page place the $username = $_GET['username']; variable at the top of the page and that will retrieve the username that is passed in the url. Then it will use that username in your query: WHERE username='$username' "; Then it will display the report for only that particular username. Make sense?
-
Ok, tested the contents of those 3 variables and they are not populating. Blank-o. Zip. Nada. So, basically I should start with the form. It's not clear to me how to set those 3 drop downs up in order to pass the data using the [] array signifier. Two of them are single choice while the checkboxes are multiple.
-
It's an auto-incremented id field. If I don't place the blank ' ' there I get a column count error.
-
Ok, so it's AFTER they log in they have a link they can click on. The simplest way is to do this: [list] [*]Change the $username = $_POST['username']; to $username = $_GET['username]; [*]In the link to the report, append it with ?username=$username [*]Set a variable on the 'landing' page with the link to the report $username = $_POST['username]; so it populates the variable at the end of the url string [/list] By doing this you're passing the person's username to the query properly and it will locate that user and display their data.
-
I have a form for contacting the site owner for an appointment. There are 3 drop down menu selectors in the form where the code is like so: [code] <?php <tr> <td class="body" align="left">Appointment is for:</td> <td class="body" align="center"><select value="appt_for[]"> <option value="Myself">Myself</option> <option value="My Son">My Son</option> <option value="My Daughter">My Daughter</option> <option value="Other">Other</option> </select></td> </tr> <tr> <td class="body" align="left">Best Days For You (select all that apply):</td> <td class="body" align="center"><input type="checkbox" name="best_days[]" value="Monday">Monday <input type="checkbox" name="best_days[]" value="Tuesday">Tuesday <br> <input type="checkbox" name="best_days[]" value="Wednesday">Wednesday <input type="checkbox" name="best_days[]" value="Thursday">Thursday <br> <input type="checkbox" name="best_days[]" value="Friday">Friday <input type="checkbox" name="best_days[]" value="Saturday">Saturday </td> </tr> <tr> <td class="body" align="left">Best Time For You:</td> <td class="body" align="center"><select value="best_time"> <option value="Before Noon" selected name="best_time[]">Before Noon</option> <option value="Afternoon before 5" name="best_time[]">Afternoon before 5</option> <option value="Evenings after 5" name="best_time[]">Evenings after 5</option> </select> </td> </tr> ?>[/code] When I try to insert the results of these 3 fields into my database they are blank. Here's a sample of the query: [code]<?php { $name = $_POST['name']; $email = $_POST['email']; $phone = $_POST['phone']; $appt_for = $_POST['appt_for']; $best_days = $_POST['best_days']; $best_time = $_POST['best_time']; $message = $_POST['message']; mysql_connect($dbhost, $dbuser, $dbpass) or die(mysql_error()); mysql_select_db($dbname) or die(mysql_error()); $sql = "INSERT INTO schedule VALUES ('', '$name', '$email', '$phone', '$appt_for', '$best_days', '$best_time', '$message', '$date')"; $results = mysql_query($sql) or die("Error occurred: ".mysql_error()); if (!results) { echo "An error occured while attempting to send your email request. Please try again."; } } ?>[/code] I need some guidance on 1) if the form is laid out properly for the select fields regarding the array format, 2) how the selection would be inserted into the database. Thanks!