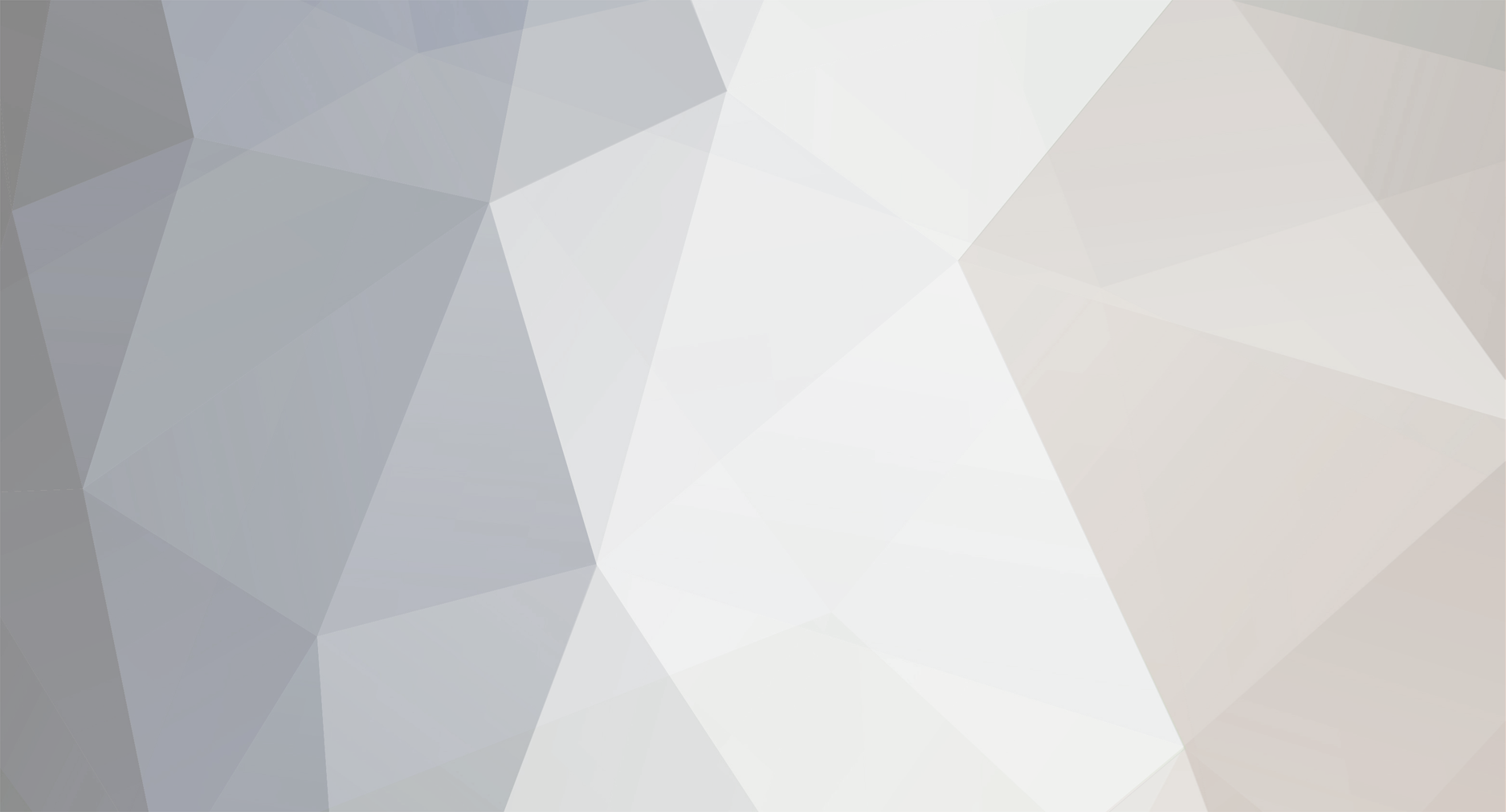
simcoweb
-
Posts
1,104 -
Joined
-
Last visited
Posts posted by simcoweb
-
-
Here's the whole page:
[code]<?php
// test to display addbiz results
include 'config.php';
include 'header.php';
mysql_connect($dbhost, $dbuser, $dbpass) or die('No database here, boss');
mysql_select_db($dbname) or die('That database can not be found, boss.');
$sql=("SELECT * FROM members");
$result = mysql_query($sql) or die('Can not find that database today');
// insert extract function
extract(mysql_fetch_array($result,EXTR_SKIP));
while ($row = mysql_fetch_assoc($result)) {
echo <<<HTML
<div align='center'>
<table border='0' cellpadding='0' style='border-collapse: collapse' width='530'>
<tr><td colspan='2' background='http://www.plateauprofessionals.com/images/top2.gif' width='530' height='35' style='padding: 10px'><h2>$name</h2>
</td>
</tr><tr>
<td height='15' colspan='2'>
</td>
</tr>
<tr>
<td style='padding-left: 10px; padding-right: 10px; padding-top:5px; padding-bottom:5px' width='229' valign='top'>$image</td>
<td style='padding-left: 10px; padding-right: 10px' width='261'>
<div align='center'>
<table border='0' cellpadding='0' style='border-collapse: collapse' width='100%'>
<tr>
<td style='border-left-width: 1px; border-right-style: solid; border-right-width: 1px; border-top: 1px dotted #666633; border-bottom-width: 1px; padding: 4px' width='46%' bgcolor='#E3E1E1'><font class='bodytext'>
<i>• $title</i></td>
</tr><tr>
<td style='border-left-width: 1px; border-right-style: solid; border-right-width: 1px; border-top-width: 1px; border-bottom: 1px dotted #666633; padding: 4px' width='46%' bgcolor='#EFEDED'>
<font class='bodytext'>• $phone</td></tr>
<tr>
<td style='border-left-width: 1px; border-right-style: solid; border-right-width: 1px; border-top-width: 1px; border-bottom: 1px dotted #666633; padding: 4px' width='46%' bgcolor='#E3E1E1'>
<font class='bodytext'>• $email</td>
</tr><tr>
<td style='border-left-width: 1px; border-right-style: solid; border-right-width: 1px; border-top-width: 1px; border-bottom: 1px dotted #666633; padding: 4px' width='46%' bgcolor='#EFEDED'>
• <a class="body" href="$url">Click Here To Visit My Site</a></td>
</tr>
<tr>
<td style='border-left-width: 1px; border-right-style: solid; border-right-width: 1px; border-top-width: 1px; border-bottom: 1px dotted #666633; padding: 4px' width='46%' bgcolor='#E3E1E1'>
• <a class="body" href='contact.php'>Click here to contact $name</a>
</td>
</tr>
</table>
</div>
</td>
</tr>
<tr>
<td style='padding: 10px' colspan='2'>
<font class='bodytext'><b>About My Services</b></td>
</tr>
<tr>
<td style='padding: 10px' colspan='2' class='bodytext' bgcolor='#e9efef' width='500'>$details</td></tr>
<tr><td style='padding: 10px' colspan='2'>
<font class='bodytext'><b>My Specialties</b></td>
</tr>
<tr><td style='padding: 10px' colspan='2' class='bodytext' bgcolor='#edecde' width='500%'>$specialties</td>
<tr>
<td style='padding-left: 0px; padding-right: 0px; padding-top: 4px; padding-bottom: 4px' background='images/bottom.gif' height='37' colspan='2'>
<p align='center' class='bodytext'>You can use our <a class="body" href='search.php'>key word search</a> option also.</td>
</tr>
</table>
</div>
HTML;
}
include 'footer.php';
?> [/code] -
I have about 20 entries in the database. I'm trying to extract them and display one at a time in a display. Here's the code:
[code]mysql_connect($dbhost, $dbuser, $dbpass) or die('No database here, boss');
mysql_select_db($dbname) or die('That database can not be found, boss.');
$sql=("SELECT * FROM members");
$result = mysql_query($sql) or die('Can not find that database today');
// insert extract function
extract(mysql_fetch_array($result,EXTR_SKIP));
while ($row = mysql_fetch_assoc($result)) {
echo <<<HTML[/code]
But here's the display showing just the first entry over and over again: http://www.plateauprofessionals.com/display.php
-
Thanks Ray. Actually this script was a test to see if there was a problem with uploading images to my server since i've been working on another script that for some reason just won't upload the image and create the thumbnail.
See this thread: http://www.phpfreaks.com/forums/index.php/topic,104388.45.html
I'm creating a member profile page and a member's list/summary page. The profile will contain the full image and the summary page will have a thumbnail. I'm looking for code to parse the data for the profile entry form which also has a file upload field. The goal is to upload the original image and also create a thumbnail at the same time. The reference for each is entered into the mysql database so I can then summon them up in the profile display pages.
Any ideas? -
It appears as though that code wasn't working and you were seeking help with it. Does it work?
What i'm actually trying to do is create a upload a pic, create a thumbnail that uploads at the same time, and import data into the mysql database pertaining to all the form fields and the pic references. -
Thanks for the post and the code suggestion. Unfortunately it just displays the submit button. No form field shows.
-
This code should upload a picture file to the designated folder. But.. it's not. NO pic is getting uploaded even though it provides a confirmation and no errors.
[code]<html>
<head>
<title>Test Upload Form</title>
</head>
<body>
<?php
//image upload test
$file_dir = "/home2/wwwplat/public_html/images/";
$file_url = "http://www.plateauprofessionals.com/images";
if (isset($image))
{
print "<center>Image path: $image<br>\n";
print "<center>Image name: $image_name<br>\n";
print "<center>Image size: $image_size bytes<br>\n";
print "<center>Image type: $image_type<p>\n\n";
if ($image_type == "image/gif" )
{
copy ($image, "$image_dir/$image_name") or die("Could not copy");
print "<img src=\"$file_url/$image_name\"><p>\n\n";
}
}
?>
<form action="<?print $PHP_SELF?>" method="POST" enctype="multipart/form-data">
<input type="hidden" name="MAX_FILE_SIZE" value="100000">
<input type="file" accept=".jpg" size="20" name="image" title="Image Upload" /><br>
<input type="submit" value="Submit">
</form>[/code]
I've validated this code by comparing it to some code in a Learn PHP book. Anyone see why it doesn't work? -
Ok, once again i'm lost. So then what do the 'resize' codes refer to?
-
Grrrr... ok, I should've known that and it didn't dawn on me until you mentioned it. I think i'm getting brain dead.
Made that change and (of course) another error appears.
This line:
[code]resize($_FILES['image']['tmp_name'], $fuller, 250);[/code]
Gets this error:
[quote]Fatal error: Call to undefined function: resize() in /home2/wwwxxxx/public_html/addbiz.php on line 38[/quote]
There's two 'resize' functions. I'm a betting man so I'm pretty certain that either/and/or of these would produce the error.
-
Ok, I agree with what you're describing.
I've inserted this code just behind the data query that inserts all the form contents so the function runs right after the data is processed. This line seems to be an issue as it produces an error no matter where I put it:
[code]UPDATE members SET image = '$fuller', thumb_image = '$thumb' WHERE memberid = $newid;[/code]
Error:
[code]Parse error: parse error, unexpected T_STRING in /home2/wwwxxxx/public_html/addbiz.php on line 40[/code] -
Barand, as always you're a great help. Thank you.
Quick question on that. Is there a specific spot in the script where this code should be inserted/implemented? I'm assuming it would be ahead of the mysql_query since the details of the image(s) needs to be created in order to be inserted.
My form already has an 'image' and 'file' input field. I just haven't done anything in the code to process it. I tried another script I found but once again it's for what would be considered a 'stand alone' file upload script and not code that would be for the use in this profile creation setup i'm working on.
I have two folders set up for the images:
/images/
/images/thumbs/
set both permissions to 777 so no problems there.
This is just a guess, but i'm supposed to ignore the first line of your pseudocode i'm assuming?
An edited version would be like this:
[code]get $newid = mysql_insert_id()
$fuller = "images/image_$newid.jpg";
$thumb = "images/thumbs/thumb_$newid.jpg";
resize($_FILES['image']['tmp_name'], $fuller, 250);
resize($_FILES['image']['tmp_name'], $thumb, 80);
UPDATE members SET image = '$fuller', thumb_image = '$thumb' WHERE memberid = $newid[/code] -
Did that and it did produce an error. It was my turn for a typo. I had 'member_cat' as the table name while the query was looking for 'member[color=red]s[/color]_cat'. I made the switch and the data inserted fine.
The next step is figuring how to set up the image and the thumbnail functions. The 'add' form for the member's includes an image upload. I need to have that image available at a specific size for the 'summary' page and then for a fuller size for the 'profile' page.
I've looked around the forums and see lots of issues about thumbs or images but nothing specific to how to operate this during the initial insertion of the data into the mysql database. The other topics seem to address it in more of a 'File Uploader' kind of thing and this is a profile generator whereas the image is uploaded at the same time as their personal and business data.
Ideas? -
Heh, thought I caught all the typos when I copied the code but didn't know that 'unsert' was supposed to be 'insert' :)
Ok, i'm running this query and nothing is getting inserted into the database(s) at this time.
[code]$sql = "INSERT INTO members (business, name, title, phone, email, url, details, specialties, image, thumb_image) VALUES ('$business', '$name', '$title', '$phone', '$email', '$url', '$details', '$specialties', '$image', '$thumb_image')";
mysql_query($sql);
$newid = mysql_insert_id();
$sql = "INSERT INTO members_cat (memberid, catid) VALUES ('$newid', '$catid')";
mysql_query($sql);[/code]
Oddly enough i'm not getting any error messages either. I'll try again with an 'or die' in there and see if something pops up. But why it's not inserting is another question. -
Ok, that's all figured out. But this error is happening now:
[quote]Fatal error: Call to undefined function: mysql_unsert_id() in /home2/wwwxxxx/public_html/addbiz.php on line 161[/quote]
Here's my code:
[code]$sql = "INSERT INTO member (business, name, title, phone, email, url, details, specialties, image, thumb_image) VALUES ('$business', '$name', '$title', '$phone', '$email', '$url', '$details', '$specialties', '$image', '$thumb_image')";
mysql_query($sql);
$newid = mysql_unsert_id();
$sql = "INSERT INTO members_cat (memberid, catid) VALUES ('$newid', '$catid')";
mysql_query($sql);[/code] -
Ok, that took care of that one. Next in line after fixing that is:
[quote]Unknown column 'mc.memberid' in 'on clause'[/quote]
Now, i'm not familiar with this type of inquiry where you are joining the 'm' and the 'mc' to the query parameters, but there IS a column named 'memberid' in the 'members' database. -
I'm getting this error message on this bit of code that's based on the reply #12:
[quote]You have an error in your SQL syntax. Check the manual that corresponds to your MySQL server version for the right syntax to use near 'catid = '1'' at line 4[/quote]
[code]<?php
include 'config.php';
$cat = '1';
$img_src = 'images/thumbs/';
// Make connect to DB first
mysql_connect($dbhost, $dbuser, $dbpass) or die(mysql_error());
mysql_select_db($dbname) or die("Where is the dang database");
$sql = "SELECT m.name, m.memberid, m.title, m.details, m.thumb_image
FROM member m INNER JOIN member_cat mc
ON m.memberid = mc.memberid
WHERE mc,catid = '$cat'";
$res = mysql_query($sql) or die(mysql_error());[/code] -
Hi Barand. In which post and where? I've stared at that until I passed out. I can't see where it's missing the ')' anywhere.
-
Hey, thanks for your posts. I referred to another file in my project and used this line:
[code]$sql=("SELECT * FROM members");[/code]
and it worked fine. No more error messages. Just a couple of other bugs to work out. Thanks for the help! -
Hi: I tried that along with a few other combinations. I just took your code and pasted it over what I had. It displays the 'if' echo statement about the profile but also still displays these errors:
[quote]Warning: mysql_fetch_array(): supplied argument is not a valid MySQL result resource in /home2/wwwxxxx/public_html/profile.php on line 10
Warning: mysql_num_rows(): supplied argument is not a valid MySQL result resource in /home2/wwwxxxx/public_html/profile.php on line 12
'We are sorry. That profile is unavailable at this time. Please select another.[/quote]
View it at: [url=http://www.plateauprofessionals.com/profile.php]http://www.plateauprofessionals.com/profile.php[/url] -
Ok, this should be simple. I've seen it a dozen times but can't find the right reference to it. I want to display a message if no results show from a database query. So.. 'IF' no results then 'display this message' ... 'ELSE' display this HTML for the results.
Here's my code snippet for this part of the script:
[code]mysql_connect($dbhost, $dbuser, $dbpass) or die('Database has gone bye bye');
mysql_select_db($dbname) or die('Where oh where is that database');
$sql="SELECT * FROM 'members' WHERE 'name' = '$name'";
$results = mysql_query($sql);
$row = mysql_fetch_array($results);
//$num = mysql_num_rows($results);
$num_rows = mysql_result($results, 0, 0);
//start HTML code for display of profile
if ($num_rows == '0') {
echo "<font class='bodytext'>'We are sorry. That profile is unavailable at this time. Please select another.<br />";
} else {
echo <<<HTML[/code]
Getting this error:
[quote]Warning: mysql_fetch_array(): supplied argument is not a valid MySQL result resource in /home2/wwwxxxx/public_html/profile.php on line 10
Warning: mysql_result(): supplied argument is not a valid MySQL result resource in /home2/wwwxxxx/public_html/profile.php on line 12[/quote] -
For some odd reason it would only work if I ran these queries. Note that they are different but within the same file. I don't know what the syntax error was referring to but this worked:
[code]<?php
include 'config.php';
// Make connect to DB first
mysql_connect($dbhost, $dbuser, $dbpass) or die(mysql_error());
mysql_select_db($dbname) or die("Where is the dang database");
// Now we create the tables and rows
mysql_query("CREATE TABLE members(
memberid INT(30) NOT NULL AUTO_INCREMENT,
PRIMARY KEY(memberid),
name VARCHAR(50) NOT NULL,
business VARCHAR(255) NOT NULL,
title VARCHAR(50) NOT NULL,
phone VARCHAR(30) NOT NULL,
email VARCHAR(50) NOT NULL,
url VARCHAR(50) NOT NULL,
details VARCHAR(255) NOT NULL,
specialties VARCHAR(255) NOT NULL,
image VARCHAR(255) NOT NULL,
thumb_image VARCHAR(255) NOT NULL,
category VARCHAR(30) NOT NULL)") or die(mysql_error());
// need to create the category table
mysql_query ('CREATE TABLE `category` ('
. ' `catid` INT(30) NOT NULL AUTO_INCREMENT PRIMARY KEY, '
. ' `catname` VARCHAR(50) NOT NULL'
. ' )')
. ' TYPE = myisam;';
if (mysql_error()) {
echo "Problem with creating database. Try again.";
} else {
echo "Category and Member Tables Were Created Successfully!";
}
?>[/code] -
Thanks for the quick response. Actually I already tried that and got the same error. I think what I posted was the 'unfixed' version of the code. Here's the corrected version and it's still producing the same error.
[code]<?php
include 'config.php';
// Make connect to DB first
mysql_connect($dbhost, $dbuser, $dbpass) or die(mysql_error());
mysql_select_db($dbname) or die(mysql_error());
// need to create the category table
mysql_query("CREATE TABLE category(
catid INT NOT NULL AUTO_INCREMENT,
PRIMARY KEY(catid),
catname VARCHAR(100)") or die(mysql_error());
if (mysql_error()) {
echo "Problem with creating database. Try again.";
} else {
echo "Category and Member Tables Were Created Successfully!";
}
?>[/code]
I don't see anything that could cause the problem. I tried also without the 'if' statement. Same error.
I ran this query on the same database without a hitch. It's basically identical to the one causing the problem up to the point of the table name and fields.
[code]<?php
include 'config.php';
// Make connect to DB first
mysql_connect($dbhost, $dbuser, $dbpass) or die(mysql_error());
mysql_select_db($dbname) or die("Where is the dang database");
// Now we create the tables and rows
mysql_query("CREATE TABLE members(
memberid INT NOT NULL AUTO_INCREMENT,
PRIMARY KEY(memberid),
name VARCHAR(50) NOT NULL,
business VARCHAR(255) NOT NULL,
title VARCHAR(50) NOT NULL,
phone VARCHAR(30) NOT NULL,
email VARCHAR(50) NOT NULL,
url VARCHAR(50) NOT NULL,
details VARCHAR(255) NOT NULL,
specialties VARCHAR(255) NOT NULL,
image VARCHAR(255) NOT NULL,
thumb_image VARCHAR(255) NOT NULL,
category VARCHAR(30) NOT NULL)") or die(mysql_error());[/code] -
WHY is this mysql code giving me this error message? ???
[code]<?php
include 'config.php';
// Make connect to DB first
mysql_connect($dbhost, $dbuser, $dbpass) or die(mysql_error());
mysql_select_db($dbname) or die(mysql_error());
// need to create the category table
mysql_query("CREATE TABLE category(
catid INT NOT NULL AUTO_INCREMENT,
PRIMARY KEY(cat_id),
catname VARCHAR(100)") or die(mysql_error());
if (mysql_error()) {
echo "Problem with creating database. Try again.";
} else {
echo "Category and Member Tables Were Created Successfully!";
}
?> [/code]
[quote]You have an error in your SQL syntax. Check the manual that corresponds to your MySQL server version for the right syntax to use near '' at line 4[/quote]
I ran an identical query without a hitch to create another table. The error just refers to line 4 but i've even gone so far as to rewrite line 4 and 5 to see if there were any hidden spaces or characters. Still get the error. -
Ok, thanks for the clarification. I'll work with that until I get stuck. Shouldn't be more than...oh... 10 minutes ;)
-
Barand, I thought that code was for displaying the 'summary' page from a particular category. Maybe I misunderstood that. I've snagged that bit of code to work as a model for the summary pages.
What i'm trying to figure out right now is how to get all entries of the database to display in the HTML format i've layed out in the url I provided (display.php). Or is this the same method you implied in reply #12?
[b]redarrow[/b], I made that change but the display still shows the same entry twice instead of the two separate entries.
Why is this returning the same row over and over?
in PHP Coding Help
Posted
That display is back up. I just hadn't uploaded the 'fixed' version when you tried.
http://www.plateauprofessionals.com/display.php