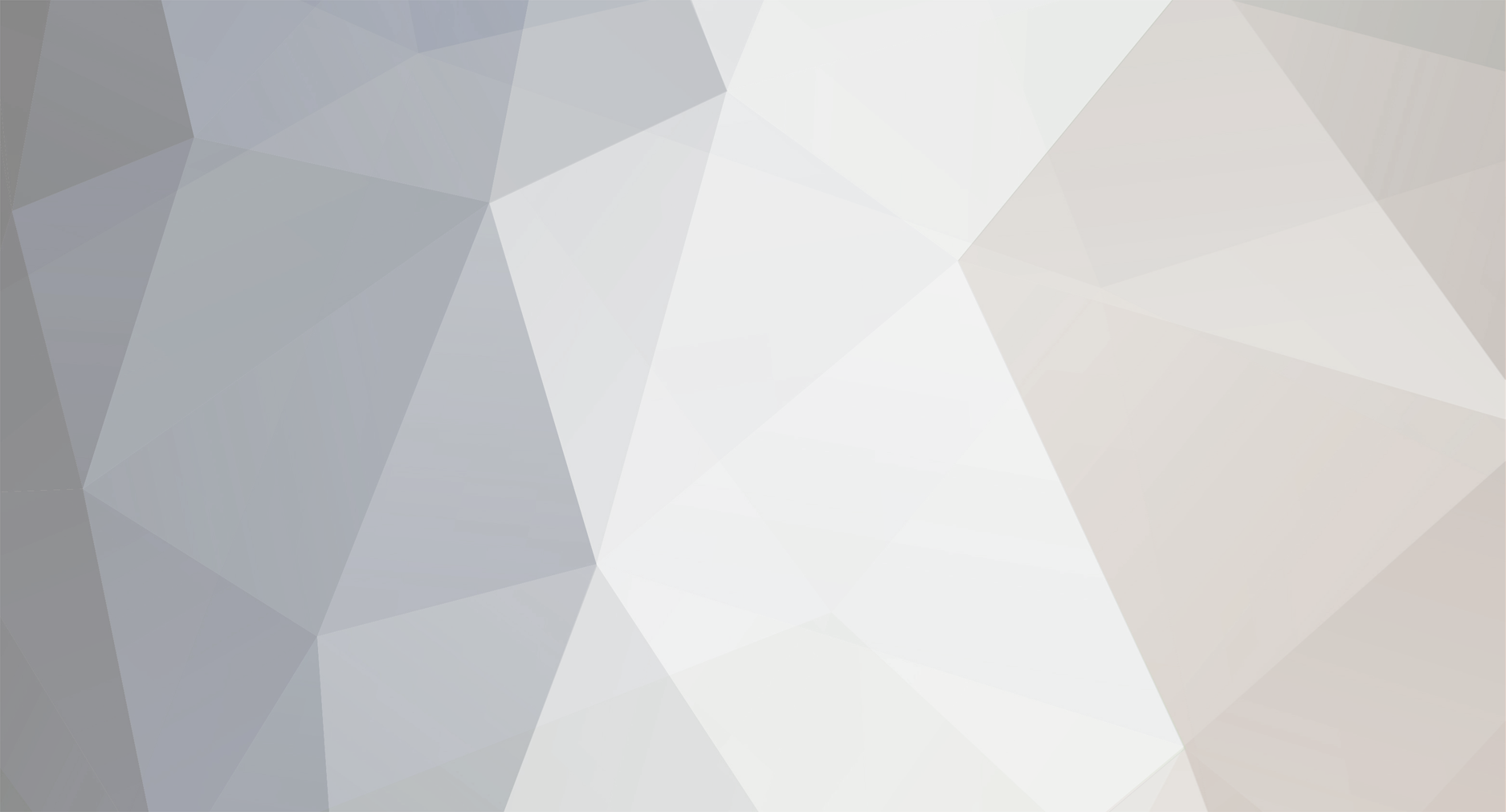
simcoweb
-
Posts
1,104 -
Joined
-
Last visited
Posts posted by simcoweb
-
-
I knew it would be something simple. Thanks for the help! :)
-
Here's the code designed to create a CSV file from the MySQL results:
[code]$data = "";
$result = mysql_query("SHOW COLUMNS FROM `iplog`") or die(mysql_error());
while($row = mysql_fetch_array($result)) {
$data .= $row[0].",";
}
$data = substr($data,0,-1)."\r\n";
$result = mysql_query("SELECT * FROM `iplog`") or die(mysql_error());
while($row = mysql_fetch_assoc($result)) {
foreach($row as $r) {
$data .= "$r,";
}
$data = substr($data,0,-1)."\r\n";
}
$handle = fopen("data.csv","wb");
fwrite($handle,$data);
fclose($handle);[/code]
The way this works right now is it writes the 'data.csv' file to the same folder the script(s) are in which means, on my servers, I have to set permissions to 777 for that to work. What I want to do is have it write the 'data.csv' file to a sub-folder so I can set the permissions on that to 777 and not have to worry about it.
Right now it writes like this:
/iplog/data.csv
I need:
/iplog/csv/data.csv
Thanks! -
This worked like a charm. Thanks for taking the time!
Quick question, you inserted an HTML tag with 3 <<<'s Just curious what the purpose is for the 3 and if it's required that I have the HTML label in there.
-
** bump ** :)
-
This is a simple script that logs and then displays a log of IP addresses that has accessed certain areas of a website. I'd like to require that the site owner use a password in order to access it. But, I don't want to use standard .htaccess stuff cuz that lame grey box is butt ugly. So, when they want to access this 'view' script I want them to enter a password into a form field and on submit it would check it against a password variable set in say a config.php file then, if true, display the data as normal. If the password entered is false then it should display the 'failed' message with a chance to re-enter.
The question is, can I do this within one script or do I need a separate login.php script to perform that action then it takes them to the view-ips.php script?
I found a login snippet here in the code library and was trying to adapt that. Here it is:
[code]session_start();
include("config.php");
$password = strip_tags(chop($_POST[password]));
if($_GET[logout] == "true") {
session_start();
unset($_SESSION['logged_in']);
session_destroy();
}
if($password == $admin_password) {
session_register("logged_in");
$_SESSION[logged_in] = "true";
}
if($_SESSION[logged_in] == "true") {
echo "Logged in!<bR>
<a href=$_SERVER[PHP_SELF]?logout=true>logout</a>";
} else {
echo "<form method=post action=$_SERVER[PHP_SELF]>
<input type=text name=password></form>";
}[/code]
Here's the script part of my view-ips.php file. If this can be adapted to work within then great. If externally, then that's great also but need some help with how to do that.
[code]<?php
//IP logger file viewer - set variables
include 'config.php';
include 'header.php';
$title = "<center><h4>IP Log File - Unauthorized Access Results</h4></center><p>";
// Here's The Snippet Inserted
// authenticate the user as the administrator
session_start();
include("config.php");
$password = strip_tags(chop($_POST[password]));
if($_GET[logout] == "true") {
session_start();
unset($_SESSION['logged_in']);
session_destroy();
}
if($password == $admin_password) {
session_register("logged_in");
$_SESSION[logged_in] = "true";
}
if($_SESSION[logged_in] == "true") {
echo "Logged in!<bR>
<a href=$_SERVER[PHP_SELF]?logout=true>logout</a>";
} else {
echo "<form method=post action=$_SERVER[PHP_SELF]>
<input type=text name=password></form>";
}
[b]END OF THE SNIPPET[/b]
// if successful it will connect to the database and display the info
mysql_connect('$dbhost', '$dbuser', '$dbpass') or die(mysql_error());
mysql_select_db($dbname) or die(mysql_error());
echo "$title<p>";
print "<table align='center' width='600' border='0'><tr><td>";
// loop through array and print each line
$query = "SELECT * FROM iplog";
$result = mysql_query($query) or die(mysql_error());
while($row = mysql_fetch_array($result)){
echo $row['ip']. " - ". $row['date'];
echo "<br />";
}
echo "</tr></td><tr><td><p><font face='Verdana' size='2'><strong>When you are through viewing these results click below to download an Excel CSV file or to erase the entries from the database.</strong></font><p></td></tr></table>";
?>
<table width="400" align="center">
<tr><td width="200" align="center">
<form action="closefile.php" method="POST">
<input type="submit" name="submit" value="Close File">
</form>
</td><td>
<form action="download.php" method="POST">
<input type="submit" name"download" value="Download Excel File">
</form>
</td></tr></table>[/code]
I marked in there where the snippet starts and ends. It's between the 'Bold' tags that look out of place.
Keeping in mind i'm a total newber and i'm using this little script as my launching pad to PHP greatness, any explanations of the code you change/add/suggest and what it does is GREATLY appreciated!
Thanks! This forum rocks! -
Dayum! You guys are fast! :)
Ok, the single quotes got rid of the immediate error. I did, however, adapt your code suggestion as well, kenrbnsn. Thanks for that.
I'll repost if there's any additional issues. Thanks again! -
This is a simple script designed to log an IP address of the visitor as well as the date of the visit. Basically it's a default page that, if landed on' will log the IP address and date then redirect the visitor to an authorized page. Here's the code:
[code]<?php //this is your default page for your unprotected directories
$ip = $_SERVER["REMOTE_ADDR"];
include 'config.php'; //edit the location of your logging file or MySQL database in the config.php
//set the date
$v_date = date("l d F H:i:s");
print "<table width='500' align='center' border='1'><tr><td>";
print "<table width='100%'><tr><td bgcolor='#C8D4DF'>";
echo "<center><font face='Verdana'><h3>Unauthorized Access Warning Message</h3></font>";
print "</td></tr>";
print "<tr><td bgcolor='#FFF0C0'>";
print "<center><h3>You should not be here</h4><p>";
print "</td></tr>";
print "<tr><td bgcolor='#FFF0C0'>";
echo "<center><font size='2' face='Verdana'>Your IP address is ". $ip . " and has been logged</font><p>";
echo "$v_date<p>";
echo "<center><font size='1' face='Verdana'><strong>You will be redirected to an authorized page.</strong></font>";
print "</td></tr></table></td></tr></table>";
//open the MySQL database and write to the table
mysql_connect('localhost', 'username', 'password') or die('Database will not open');
mysql_select_db('test');
// Insert a row of information into the table "example"
mysql_query("INSERT INTO iplog
(ip, date) VALUES($ip, $v_date) ")
or die(mysql_error());
echo "Data logged successfully!<br />";
?>[/code]
When I try to execute this I get this error:
[quote]You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near ' Sunday 16 July 14:39:48)' at line 2[/quote]
However, line 2 has nothing to do with the date. I'm using MySQL 5.0.21 with XAMP. Any ideas why this is happening? Or, perhaps what the proper syntax would be to insert the date? Thanks in advance for your help! -
I figured out the way to delete the file contents using the 'ftruncate' method.
-
Even though i'm a total noob, this one has been a great tool and has all types of built in libraries of common PHP/HTML/XML/JAVASCRIPT functions and code. Highly rated, reivewed by the big software shareware freebie sites, etc. etc.
PHP Designer 2006 = [url=http://www.mpsoftware.dk/]http://www.mpsoftware.dk/[/url] -
Here's what I wound up with:
[code]<?php //IP logger file viewer - set variables
$filename = "../pictures/ips.txt"; //edit location here
$path = "/home2/wwwxxx/public_html/pictures/";
$title = "<center><h4>IP Log File - Unauthorized Access Results</h4></center><p>";
$data = file($filename) or die('Cannot open $filename');
echo $title;
print "<table align='center' width='600' border='0'><tr><td>";
// loop through array and print each line
echo '<pre>';
foreach ($data as $line)
echo htmlentities($line);
echo '</pre><br/>';
echo "</tr></td><tr><td><p><font face='Verdana' size='2'><strong>When you are through viewing these results click below to close this file.</strong></font><p></td></tr></table>";
?>[/code]
This produced the required results. The first attempt included the brackets around your echo($data as $line) etc etc section like this:
[code]<?php //IP logger file viewer - set variables
$filename = "../pictures/ips.txt"; //edit location here
$path = "/home2/wwwxxx/public_html/pictures/";
$title = "<center><h4>IP Log File - Unauthorized Access Results</h4></center><p>";
$data = file($filename) or die('Cannot open $filename');
echo $title;
print "<table align='center' width='600' border='0'><tr><td>";
// loop through array and print each line
echo '<pre>';
foreach ($data as $line) {
echo htmlentities($line);
echo '</pre><br/>';
}
echo "</tr></td><tr><td><p><font face='Verdana' size='2'><strong>When you are through viewing these results click below to close this file.</strong></font><p></td></tr></table>";
?>[/code]
Which produced the first line being formatted properly and the ones following were still in a lump.
Another question, say I want to give the 'admin' viewing these stats the ability to delete these entries in one swipe. How would I code that? I'm assuming there'd be a form button with an action pointing back to SELF that would run the file contents/delete code. Once again, i'm complete noob so this may seem basic but it's like building the pyramids for me :)
Thanks again for your help!
-
Hold the presses! Ok, Kenrbnsn... in your code I noticed you left out the { }'s in the foreach statement. I didn't know if that was intentional or not so I originally left them in which produced the first line being formatted as shown above. After my post I removed them, reupoaded and then ran the script and the formatting came out fine on each one. Like this (IP's removed on purpose):
IP: - DATE: Sunday 09 July 17:18:10
IP: - DATE: Sunday 09 July 17:19:01
IP: - DATE: Sunday 09 July 17:19:58
IP: - DATE: Sunday 09 July 17:20:36
IP: - DATE: Sunday 09 July 17:20:38
IP: - DATE: Sunday 09 July 17:20:39
IP: - DATE: Sunday 09 July 17:20:40
So, this works! But, I don't know WHY it works. Could I trouble you for a quick explanation of what that line of code does/means so I can put it in my memory banks? Thanks! -
Shogun, your guess on the file contents was right on. It actually looks like this:
IP: 71.231.197.177 - DATE: Sunday 09 July 12:24:31
I used your code and this is the display that resulted, however:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
Access from IP: I at: P
Access from IP: at:
So, something's amiss there. It's splitting the first two letters and displaying those. It would be great if it laid out the way your code logic is outlined whereas it would be 'Someone at IP 444.44.44.444 hit this page on Monday July 10 2006 at 9:45am' showing on each line.
Kenrbnsn, your code worked ok on the first line. Then the rest of them were that jumbled mess. Here's a snapshot:
[quote]IP Log File - Unauthorized Access Results
IP: 71.231.197.177 - DATE: Sunday 09 July 11:50:03
IP: 71.231.197.177 - DATE: Sunday 09 July 12:01:25 IP: 71.231.197.177 - DATE: Sunday 09 July 12:02:06 IP: 71.231.197.177 - DATE: Sunday 09 July 12:23:45 IP: 71.231.197.177 - DATE: Sunday 09 July 12:23:47 IP: 69.93.229.186 - DATE: Sunday 09 July 12:28:35 IP: 69.93.229.186 - DATE: Sunday 09 July 12:39:24 IP: 69.93.229.186 - DATE: Sunday 09 July 12:45:51 IP: 69.93.229.186 - DATE: Sunday 09 July 12:45:54 IP: 71.231.197.177 - DATE: Sunday 09 July 13:40:43 IP: 81.157.199.76 - DATE: Sunday 09 July 13:44:23 IP: - DATE: Sunday 09 July 17:18:10 IP: - DATE: Sunday 09 July 17:19:01 IP: - DATE: Sunday 09 July 17:19:58 IP: - DATE: Sunday 09 July 17:20:36 IP: - DATE: Sunday 09 July 17:20:38 IP: - DATE: Sunday 09 July 17:20:39 IP: - DATE: Sunday 09 July 17:20:40 IP: 71.231.197.177 - DATE: Sunday 09 July 17:22:12 IP: 71.231.197.177 - DATE: Sunday 09 July 17:23:43 IP: 71.231.197.177 - DATE: Sunday 09 July 17:24:05 IP: 71.231.197.177 - DATE: Sunday 09 July 17:24:33 IP: 71.231.197.177 - DATE: Monday 10 July 11:45:21 IP: 71.231.197.177 - DATE: Monday 10 July 11:47:47 IP: 71.231.197.177 - DATE: Monday 10 July 11:52:54 IP: 71.231.197.177 - DATE: Monday 10 July 11:52:56 IP: 71.231.197.177 - DATE: Monday 10 July 15:04:03[/quote]
We're really close! Any ideas? :) -
heh heh... well, i'm using those cuz i'm a total noob. Just learning this stuff and fighting my way from tutorial to tutorial and forum to forum making these little cheesbrain scripts. Sort of the crawl before I run kinda thing.
I'm using fopen since the description of that function is what I needed it to do. Same with the file_get_contents. That did the trick. But, I'm sure there's much better ways to do this but I just don't have the knowledge... yet ;)
An example of the proper code would set me on the right track! -
Hello:
Here's a summary. I have a small script writing the IP address and access date to a simple text file. Next I created another little script to display that text file. However, it just displays all the content of the text file in a lump. How would I have it display the content in a nicely formatted one-listing-per-line format?
Here's the code of the display:
<?php //IP logger file viewer - set variables
$filename = "../pictures/ips.txt"; //edit location here
$path = "/home2/xxxxxxt/public_html/pictures/";
$title = "<center><h4>IP Log File - Unauthorized Access Results</h4></center><p>";
$data = file_get_contents($filename) or die('Cannot open $filename');
echo $title;
print "<table align='center' width='600' border='0'><tr><td>";
fopen($filename, 'r') or die("cannot open $filename");
echo $data;
echo "</tr></td><tr><td><p><font face='Verdana' size='2'><strong>When you are through viewing these results click below to close this file.</strong></font><p></td></tr></table>";
?>
<center><form action="closefile.php" method="POST">
<input type="submit" name="submit" value="Close File">
</form></center>
This works pretty much the way I wanted it to but the 'echo $data' just puts the glob in the middle of the page.
I'd search the forums for this answer but I honestly don't have a clue what search term would pull the results. Thanks in advance for the help! -
First, thanks for your efforts and ideas. I got it to work by using a combination of the ideas you suggested. Here's the final code:
<?php
$ip = $_SERVER["REMOTE_ADDR"];
$ips = "../pictures/ips.txt";
//set the date
$v_date = date("l d F H:i:s");
echo "Unauthorized Access Warning Message";
print "<h3>You should not be here</h4><p>";
echo "Your IP address is ". $ip . " and has been logged<p>";
echo "$v_date<p>";
echo "You will be redirected to an authorized page";
//open the file and write the IP + date
$fp = fopen("$ips", "a") or die("cannot open $ips\n");
fputs($fp, "IP: $ip - DATE: $v_date\n\n");
//close the file
fclose($fp);
?>
Note I took out the variable from the fopen function as you suggested. My 'logical' mind thought I needed a path indicator there in order to find the right file.
The 'getenv' didn't seem to work so I kept the original 'REMOTE_ADDR' call there and it works fine.
The key to all this is the ips.txt file resides in a specific folder that are on the same level as the others instead of being a sub-directory. Therefore I had to direct the script to write to that common file.
I'm a complete noob with PHP but am studying tutorials like a mad man to learn it as quick as possible. These forums are great. Keep up the good work :) -
Hello:
I'm setting up a simple PHP page to display as a default directory home page to protect against intruders searching for directories on our sites that don't contain an index.xxx file. This way they hit my 'warning' page, their IP and the date are logged to a file and they are redirected back to the site's home page. Here's the code:
<?php
$ip = $_SERVER['REMOTE_ADDR'];
$filepath = "http://www.nameofsite.com/pictures/";
$ips = 'ips.txt';
//set the date
$v_date = date("l d F H:i:s");
echo "Unauthorized Access Warning Message";
print "<h3>You should not be here</h4><p>";
echo "Your IP address is ". $ip . " and has been logged<p>";
echo "$v_date<p>";
echo "You will be redirected to an authorized page";
//open the file and write the IP + date
$fp = fopen("$filepath", "$ips", "a") or die("cannot open $ips\n");
fputs($fp, "IP: $ip - DATE: $v_date\n\n");
//close the file
fclose($fp);
?>
<script language=javascript> <!--
// Redirect code
window.setTimeout("location='http://www.nameofsite.com/index.php'",6000);
//-->
</script>
I'm inserting this page into multiple directories but want it to write to one common file. The way I have it set up now with these variables:
$ip = $_SERVER['REMOTE_ADDR'];
$filepath = "http://www.nameofsite.com/pictures/";
$ips = 'ips.txt';
it writes to the file fine but instead of writing the person's IP address it writes the IP address of the server. However, on that page it displays the visitor's IP address. I can't figure out why it's not writing the visitor's IP address and, instead, is writing the server's IP to the file.
Here's the page in question: http://www.guitar4sale.com/pictures/
Notice it displays your IP address. It's not writing that IP. I think it's something to do with the $filepath variable and the location I have placed there for the common file.
Help anyone? ;D
-
[!--quoteo(post=383111:date=Jun 13 2006, 12:57 AM:name=Barand)--][div class=\'quotetop\']QUOTE(Barand @ Jun 13 2006, 12:57 AM) [snapback]383111[/snapback][/div][div class=\'quotemain\'][!--quotec--]
Uou are referring to $_POST variables but the default method for forms is GET.
Specify form method as "POST"
[/quote]
DOHHHH! Wow, can't believe I missed that. Ok, i'll post back with the results. Thanks! -
Hey..thanks for the quick reply.
Ok, tried that. I see you added some '!'characters in there. I also notice that their position in the statement is a little different but I don't think that makes a difference... does it?
Here's what happened when I made the changes. The 'true' statements printed but when entering the 'false' data (anything but what the variables hold true at which is 'halo' and 'rockmetal') then it still displays the 'true' statements. I type in anything in the two form fields and it displays the 'true' text. It's not parsing the 'false' statements.
So, adding those '!' now makes the true happen no matter what.
Just make sure that when your at the proctologists that while feeling the probe you don't also notice both his hands on your shoulders ;) -
Ok, I've already announced that i'm a newb. JUST learning PHP and going through literally dozens of tutorials and moving along. However, like I say to my proctologist... be gentle! :)
Here's the scoop. I've created a simple form in order to practice the if/else routines and experiment with the output. I already created a simulated order form and got that working without a hitch while swapping displayed messages depending upon how many of each item they ordered and also what their total number of items ordered. Worked great.
But! Now what I tried was to compare text input and 'if' it meets a certain name (in this case a game) then it would display one message and if they entered something else it would display a different message.
here's the code for the form:
<html>
<head>
</head>
<body>
<form name="shanetest" action="shanetest.php">
<font face="Verdana" size="2">Enter Your Favorite Game</font><br>
<input type="text" name="gamename" size="20"><p>
<font face="Verdana" size="2"><b>Your Gamer Tag</b></font><br>
<input type="text" name="gamertag" size="20"><p>
<input type="submit" name="submitinfo" value="Submit yo stuff" style="cursor:pointer">
</form>
</body>
</html>
And my code for the 'shanetest.php' file:
<?php
// First we create some variables
$gamertag = $_POST['gamertag'];
$gamename = $_POST['gamename'];
// Now we process the information
if ( $gamename == "halo" ) {
echo "You are the bomb, baby!<p>";
} else {
echo "you are a LOSER!<p>";
}
if ( $gamertag == "rockmetal" ) {
echo "That rocks, dude!<p>";
} else {
echo "Why would you play that lame game?";
}
?>
Now, all I get is the 'false' statements displayed. Even if you type in the proper entries of 'halo' and 'rockmetal' it won't display the 'if' true statements.
Once again, logically it should work. Code wise I must be missing something or trying to produce something that PHP needs additional info or a different operator.
I see lots of examples of this but none that showed the variables being populated by a form input and then being compared in an 'if' statement.
Can someone point me in the right direction? Thanks! -
We ran this query and it removed everything from that particular field:
UPDATE table_name SET product_shipemail=\'\'
(replace \'table_name\' with the actual name)
We wanted to remove the data in the \'product_shipemail\' and leave it blank. If we placed text in between the two \' \' marks then it would\'ve replaced what was there with the new stuff.
-
Thanks! But we did successfully get this taken care of.
-
Thanks for the post!
What you\'ve described is for deleting an entry. If you can visualize this, you\'ve mentioned deleting a \'row\' (or editing). I want to delete the content in a \'column\'.
To further clarify, there are approximate 1860 products (rows) and 27 fields (columns). I want to delete the information in field #27 for all 1860 products.
-
Hi all. This is a simple question. But, as we all know, if you don\'t have the answers then it\'s not so simple
I\'m new to MySQL databases and i\'m using PHPMyadmin to administrate a shopping cart database. I\'m not that familiar with the interface and there\'s absolutely no tutorial type instruction with the program.
Here\'s the scoop:
I need to remove the data from one field of one of the tables. There\'s 1850 products in the database. When I first log in i\'m presented with the various tables on the left. I select \'Products\'. Then that displays all the fields in that particular table. One of them is:
product_shipemail
I need to remove the entries from that field. NOT the field itself.
I\'m presented with the following choices and looking at this list of fields with a checkbox next to it:
Browse
Select
Insert
Empty
Drop
The reason i\'m paranoid is simple. I haven\'t a clue if any of these commands (not Browse or Select) will just wipe out the entire database, or, just the field I selected, or, the entire table i\'m viewing, or, etc. etc. etc. and there\'s not indication before I click it if it\'s a one-click command, warns me 12 times, says a prayer, or what.
So before my shaking hand accidentally deletes literally days worth of work, I wanted to see if I could get a little guidance on this.
Thanks!
How can I write this file & turn it into a downloadable zip file at same time?
in PHP Coding Help
Posted
[code]$data = "";
$result = mysql_query("SHOW COLUMNS FROM `iplog`") or die(mysql_error());
while($row = mysql_fetch_array($result)) {
$data .= $row[0].",";
}
$data = substr($data,0,-1)."\r\n";
$result = mysql_query("SELECT * FROM `iplog`") or die(mysql_error());
while($row = mysql_fetch_assoc($result)) {
foreach($row as $r) {
$data .= "$r,";
}
$data = substr($data,0,-1)."\r\n";
}
$handle = fopen("data.csv","wb");
fwrite($handle,$data);
fclose($handle);[/code]
Which works great in creating the CSV file. However, we'd like to make this newly generated file downloadable. As it stands right now all i've been able to do is have it display the CSV file and the person would need to copy and paste it. Sorta lame i'd say. So, what I would like it to do is either:
1) write/create the file AND turn it into a downloadable zip file, or
2) write/create the file AND have another 'option' to download it which would then turn it into a zip file (so instead of write/zip at once it's two different functions).
With scenario #2 the person could then decide if they want to copy and paste or zip and download.
Ideas?