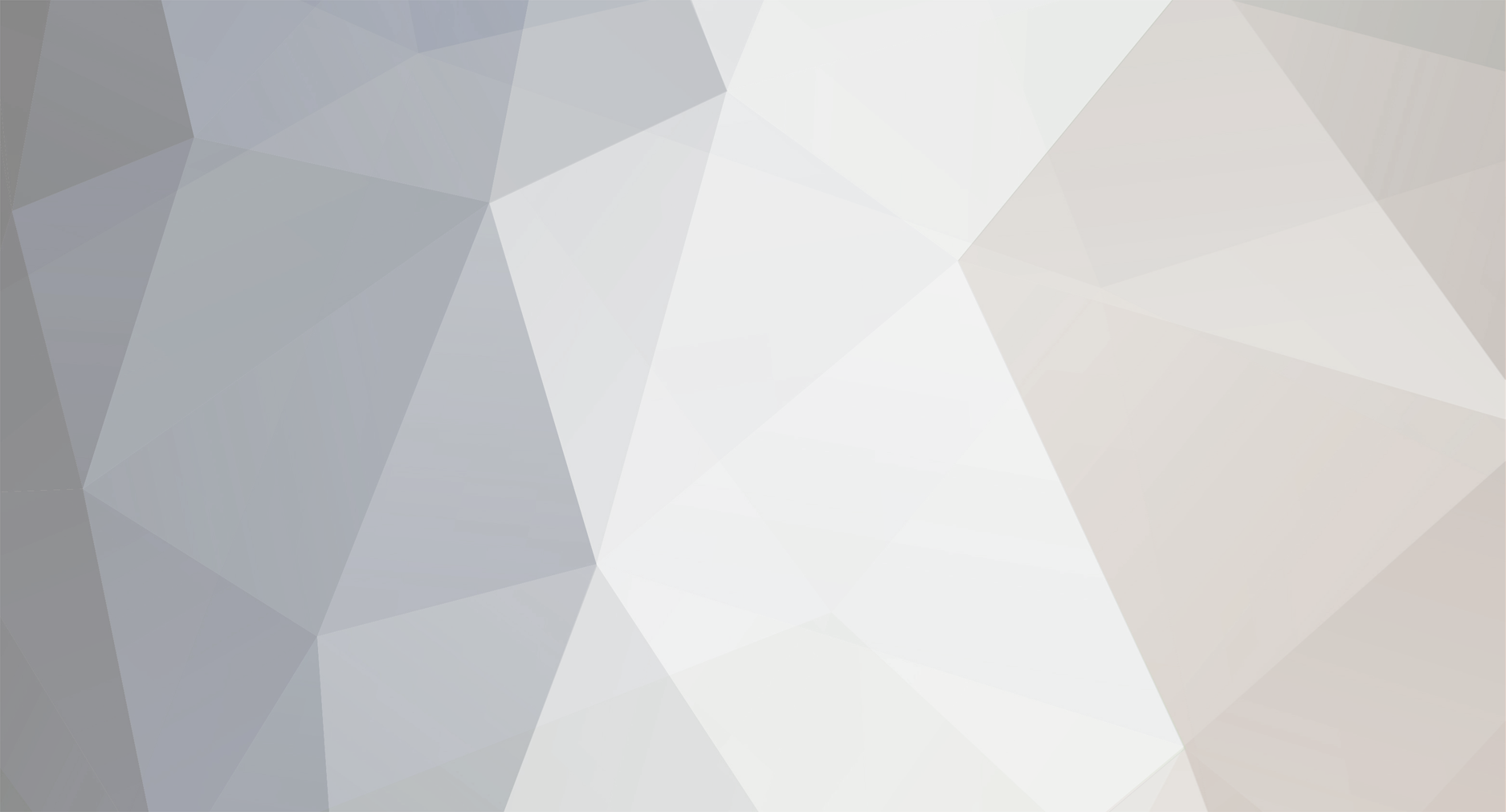
dcro2
Members-
Posts
489 -
Joined
-
Last visited
Never
Everything posted by dcro2
-
Are you sure the directory where web documents go is correct? And where you're uploading is correct? Because the error says /temperature_logger.php. That would mean it's in the disk's root (/) folder. Typically these files are under some form directory like /var/www/ or /home/username/etc/. Unless you just stripped off the path. Or you have some really weird host.
-
Multiple Checkboxes HTML w/PHP: Error with Foreach
dcro2 replied to Doc20's topic in PHP Coding Help
I think they meant implode... $project_lists_string = implode(',', $project_lists); // "chat,forums,tech" -
Right, that warning just means that $_POST['userid'] isn't set. This variable is only set when a form is submitted to this page with the POST method. When phpDesigner checks it, nothing's been submitted, right? So nothing in $_POST will be set. You should be using isset like you are doing inside the foreach loop.
-
Even if you only update the stock of products using this page (and not if someone's payment went through) you should be using IPN or PDT with verification from PayPal:
-
<?php foreach($feed->get_items('0, 4') as $item): ?> Notice the colon. That's how alternative syntax works.
-
Notice the name of your file input: <input type="file" name="photo"> Its name is photo, but you're using $_FILES['image']. They have to match. $image=$_FILES['photo']['name']; Also, I don't see the logic in this: mysql_query("INSERT INTO users (prof_pic) VALUES ('$image_name')"); This will insert a completely NEW row into the users table with JUST the prof_pic. You should be using UPDATE instead: UPDATE users SET prof_pic = '$image_name' WHERE username = '$username' Or something like that.
-
You'll have to select the `image` column from the database as well: $query = "SELECT id, image, deceased_name, deceased_date, DATE_FORMAT(deceased_date, '%M %D, %Y') as datetext"; Then echo it with the other things: $image = $listing["image"]; echo("<tr><td width=\"100%\"><a href=\"obitdetail.php?id=".$id."\"><img src=\"".$image."\" alt=\"".$deceased_name."\"><br><strong>".$deceased_name."</strong></a></td><td> </td>");
-
This might be what you're looking for: http://forums.mysql.com/read.php?20,128132,128134#msg-128134 Basically, use HAVING COUNT(column) <= 4 on your JOINs.
-
To use date_default_timezone_set with your form you'd have to change the values to "+1", "+2", etc. and then prefix it with "Etc/GMT ". date_default_timezone_set("Etc/GMT $timezone"); If you want Daylight Savings to work though, you'll have to use timezones like US/Eastern/Central/Pacific etc. See here for a list of supported timezones: http://www.php.net/manual/en/timezones.php
-
header("Content-Type: text/plain; charset=UTF-8"); Change text/plain to text/html if you're going to be using it inside html code.
-
The third parameter for mktime is seconds, not timezone. To set the timezone, you have to use date_default_timezone_set. Can we see the code where you actually set up those variables and the form? That's probably where the problem is.
-
That's right, because that search turns up nothing to match: window.google.ac.h(["angelina jolie movie 2019",[],{}]) So, just declare $kw at the top as $kw = array();
-
How does it appear in the html source (in your browser)?
-
set_time_limit(0); //no time limit Unless you're in safe mode...
-
Found this, which might help: http://www.php.net/manual/en/language.variables.variable.php#87564 You can use a foreach to build the index part ([1][4][2][0]) foreach($return as $key) $index .= "[$key]"; Maybe someone else will know an easier way...
-
$tid = $_GET["tid"]; header('Location: &xat=WU-'.$tid); It's called string concatenation <<clicky.
-
Bad boy. If you're going to use nested loops, don't use the same variable names. The value that you set for $num_results in the second loop affects the number of iterations of the outer loop (which would be better off as a while loop, actually). Also, you assign the mysql result of the inner loop to the same variable as the outer loop. Same thing with $row, but that doesn't affect anything since you don't use it after starting another loop. Use different variables for each inner loop.
-
Give new value to variable from MYSQL UPDATE statement
dcro2 replied to nightkarnation's topic in PHP Coding Help
Simply put, it's not gonna happen. mysql_query won't return a result unless it's a SELECT query (or SHOW, DESCRIBE, EXPLAIN). I don't understand why you can't just SELECT the row to get the new price. -
If you want PHP to evaluate your strings (like turn $i into its value), you have to enclose them in double quotes. But in this case, you don't even need to use quotes. All the single/double quotes do is tell PHP that you're writing a string. for ($i = 1; $i < 29; $i++) { if ($_POST[$letters[$i]]){ echo "You have selected the following products:" . "<br>" . $_POST[$letters[$i]]; } } PS: I'm not so good at explaining these kinds of things, so if you don't understand maybe someone else could explain better.
-
mysql_fetch_array returns just what it says on the box, an array. Also, it makes more sense to check for differences AFTER you've gotten all the filenames from the database. Try this: while ($fetch = mysql_fetch_array($results)) { $clean_fetch[] = str_replace("productImages/", "", $fetch[0]); } $diff = array_diff($images, $clean_fetch); foreach (array_unique($diff) as $key => $value) { $output .= $value . "<br />"; } Answer to your last question: $clean_fetch wasn't being setup as an array, so it only had one member ($fetch) every time you looked for differences.
-
That sounds pretty easy... just loop through the keywords again if ($_SERVER['QUERY_STRING']!='') { gsscrape($_SERVER['QUERY_STRING']); foreach ($kw as $keyword => $pages) { gsscrape($keyword); } $appends = range('a', 'z'); foreach($kw as $keyword => $pages) { foreach($appends as $append) { gsscrape($keyword." ".$append); } } } You might want to think about using curl instead of file_get_contents. If you're going to be doing a lot of requests, you'll want something more optimized for http requests so it takes less time.
-
Try setting this error_reporting(E_ALL); ini_set('display_errors', 1); on top to see if you get any missed errors. Also, do an echo $i[0] just to make sure you're getting what you expect.
-
Instead of trying to fix that broken text_between function, I think you'll be better off with a nice, stable, regular expression. <?php function gsscrape($keyword) { $keyword=str_replace(" ","+",$keyword); $keyword=str_replace("%20","+",$keyword); global $kw; $data=file_get_contents('http://clients1.google.com/complete/search?hl=en&gl=us&q='.$keyword); if(preg_match_all('/\["([a-z0-9 "]+)",".*","(\d+)"\]/iU', $data, $matches)) { for($i=0; $i<count($matches[1]); $i++) { $kw[$matches[1][$i]] = $matches[2][$i]; } } } #simple to use, just use yourscriptname.php?keywords //echo $_SERVER['QUERY_STRING']; if ($_SERVER['QUERY_STRING']!='') { gsscrape($_SERVER['QUERY_STRING']); foreach ($kw as $keyword => $pages) { gsscrape($keyword); } } #all results are in array $kw... echo "<pre>"; print_r($kw); echo "</pre>"; ?> This is the array I get with the keyword 'keyword': Array ( [keyword tool] => 0 [keyword bidding] => 0 [keyword spy] => 0 [keyword anchor text links] => 0 [keyword generator] => 0 [keyword density] => 0 [keyword discovery] => 0 [keyword elite] => 0 [keyword stuffing] => 0 [keyword density tool] => 0 [keyword tool dominator] => 1 [keyword toolbox] => 2 [keyword tool search volume] => 3 [keyword tool api] => 4 [keyword tool ipad] => 5 [keyword tool by city] => 6 [keyword tool yahoo] => 7 [keyword tool seo] => 8 [keyword toolkit] => 9 [keyword bidding tool] => 1 [keyword bidding google adwords] => 2 [keyword bidding strategy] => 3 [keywordspy promo code] => 2 [keyword spy vs] => 3 [keyword spy review] => 4 [keyword spy download] => 5 [keyword spy vs spyfu] => 6 [keyword spy alternative] => 7 [keyword spy coupon] => 8 [keyword spy free trial] => 9 [keyword generator google] => 1 [keyword generator tool] => 2 [keyword generator free] => 3 [keyword generator online] => 4 [keyword generator free online] => 5 [keyword generator from text] => 6 [keyword generator excel] => 7 [keyword generator seo] => 8 [keyword generator for seo] => 9 [keyword density checker] => 2 [keyword density analyzer] => 3 [keyword density seo] => 4 [keyword density analysis] => 5 [keyword density google] => 6 [keyword density calculator] => 7 [keyword density count] => 9 [keyword discovery tool] => 1 [keyword discovery review] => 2 [keyword discovery api] => 3 [keyword discovery vs wordtracker] => 4 [keyword discovery trellian] => 5 [keyword discovery from trellian] => 6 [keyword elite review] => 1 [keyword elite vs market samurai] => 2 [keyword elite training] => 4 [keyword elite university] => 5 [keyword elite trial] => 6 [keyword elite blackhat] => 7 [keyword elite login] => 8 [keyword stuffing seo] => 1 [keyword stuffing google] => 2 [keyword stuffing tool] => 3 [keyword stuffing in url] => 4 [keyword stuffing penalty] => 5 [keyword stuffing not allowed] => 6 [keyword stuffing examples] => 7 [keyword density tool free] => 1 [keyword density tool paste] => 2 [keyword density tool google] => 3 [keyword density tool text] => 4 [keyword density tool for documents] => 5 [keyword density tool for word] => 6 [keyword density tool download] => 7 [keyword density tool firefox] => 8 ) Not sure if you want it some other way, it was kind of hard to decipher what you were trying to do.
-
Actually, it'll parse almost any form of date/time.. from the man page: Which variable actually contains the date? Is it $month_end_date[$surgeryinvoicescount], or is it $i[0]? Because for $month_end_date to be the string containing the date would make no sense, since it's an array, or you treat it like one at least.
-
UPDATE array...what's wrong with this short code?
dcro2 replied to TCombs's topic in PHP Coding Help
Haha, hi. I think this is what you want instead then: for($i=0; $i<count($arrid); $i++) { $sql = "UPDATE colors SET color='".$arrcolor[$i]."' WHERE id = '".$arrid[$i]."'"; mysql_query($sql) or die("Error: ".mysql_error()); echo $sql; }