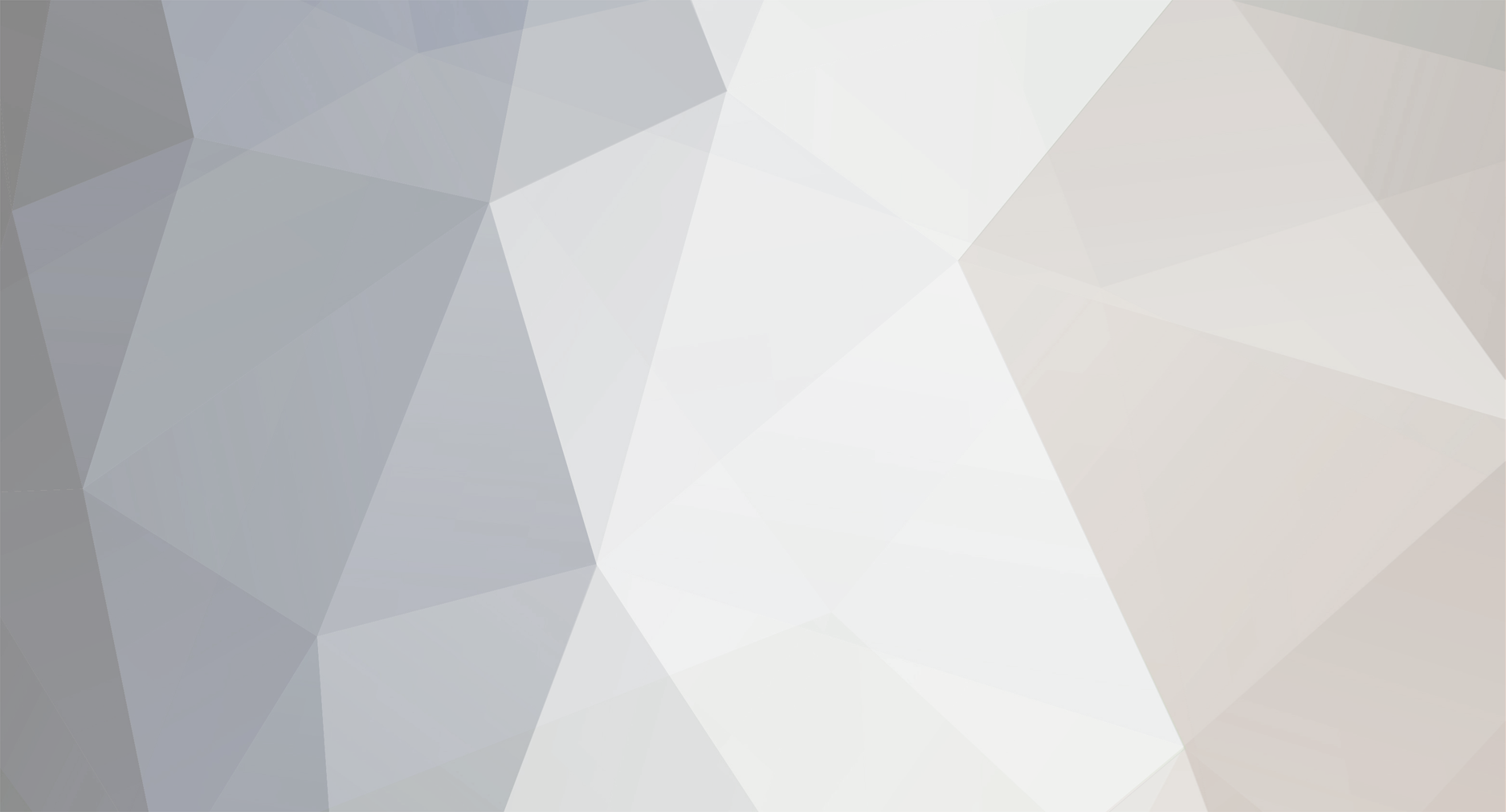
Darklink
Members-
Posts
126 -
Joined
-
Last visited
Never
Everything posted by Darklink
-
<?php require_once('../recaptcha/recaptchalib.php'); // Get a key from http://recaptcha.net/api/getkey $publickey = "6LdhQAIAAAAAADD0DAmQYiLV-blwLkxhykzay2us "; $privatekey = "6LdhQAIAAAAAAEELsaFefmf4y-C0KbyzU3Qj37Xw "; # the response from reCAPTCHA $resp = null; # the error code from reCAPTCHA, if any $error = null; # was there a reCAPTCHA response? if ($_POST["recaptcha_response_field"]) { $resp = recaptcha_check_answer ($privatekey, $_SERVER["REMOTE_ADDR"], $_POST["recaptcha_challenge_field"], $_POST["recaptcha_response_field"]); if ($resp->is_valid) { $redirect = true; header('Location: http://www.google.com'); } else { # set the error code so that we can display it $error = $resp->error; } } if ( !$redirect ) { echo recaptcha_get_html($publickey, $error); } ?> Could be something with reCaptcha though. What was your error exactly.
-
Oh and on your SQL query you have a comma just before the WHERE function. You should take that away. You only use that before each SET key. UPDATE Admin_Page_Style SET Style_Left = '$left', Style_Top = '$top', Style_Height = '$height', Style_Width = '$width', Style_Position = '$Position', Style_bgcolor = '$Background', Style_Cusor = '$Cursor', Border = '$Border', div_name = '$div_name' WHERE div_name = 'adam'
-
Did you alert the values before you sent them or did you alert them when they came back? Alert the responseText value when it comes back.
-
It's not the proper way to solve all your header problems, but put ob_start(); at the top of the page.
-
Try debugging. When the script terminates, in Javascript alert the results and see if there is an error. Or echo each individual value.
-
It shouldn't do. Anyway, you can check the users browser by doing some expression checking on the users agent. You can then determine what to do from there on in.
-
If you send emails, make sure they aren't from a noreply address. Some inbox's don't accept them and chuck them straight in the spam.
-
If you echo using single apostraphes you can't quickly pass variables nor can you make \r\n linebreaks. Anyway, I use the first one in my code.
-
Have you actually tried using nl2br. It should work. It turns invisible linebreaks into HTML.
-
You can't pull a script from an external site easily and without permission from the owner. You might want to use some form of output reading of an external page and use preg_match. In which case you will want to learn about fsocket and regular expressions.
-
Well thats nice for you. But remember, not everyone is as clever or have the patience to actually read the code and find out what it does themselves. If you see any decent script out there, it will be covered with comments like those. Scripts without, I find are very messy and have a very unfortunate programmer on it's head.
-
I code very quickly with very efficient code. I use my own framework. For a portfolio you will want to use other frameworks and there are plenty out there. If you ever get the chance, try and build your own framework. You understand it better and if you ever need to make any changes you know where to look. Everything is so much quicker!
-
Well I don't really document any HTML (a little for CSS but not much) unless I'm debugging templates, in which case comments are generated by the template engine. The things I will comment on is a PHP file itself (header comments including description, version, copyrights, etc). I will comment on functions, classes, object vars, and some details here and there about what is going on and why I'm doing this. If I've added something in for a new version, I may also say so! Here's a small example of how I document. Notice that's clear and it brings out the important parts. Images and links are not really important to link unless a link isn't so obvious. But in that case, one would just go to the link and find out where it goes or what it does. <?php /* ---------------------------------------------- */ // Function description // // @param string example string // @param boolean show string // @return string string to return /* ---------------------------------------------- */ function example($string='', $show=false) { // ------------------------------------- // If show is enabled, echo string // ------------------------------------- if ( $show ) { echo $string; } // ------------------------------------- // Return string to outside world // ------------------------------------- return $string; } ?>
-
have php receive an email and insert it into a database?
Darklink replied to android6011's topic in PHP Coding Help
You will want to check out the IMAP functions that php provides you (in an extension). I've done it before. -
The only way to do this is by using JavaScript or another web application language like Java or flash.
-
Maybe documenting the link and images as owners of them. But documenting everything is practically impossible at times. You should go through a document it as it comes up. Like you would with a function. You would show the name, description and it's paramaters/return values. What is there to document about links and images anyway? "This one goes here..." and "this is a picture of..." would just be silly.
-
If your reply-to address has noreply in it, hotmail may filter it out as spam. I've heard it does that before.
-
After the filename like: http://www.example.com/index.php?act=test&do=something The ? indicates the start of the query and the & is the seperator between each paramater. So the URL above would return the following $_GET array: [act] => test [do] => something The use of ; in a URL is not really used as far as I am now unless you are parsing that as one of the parameter values. A common mistake is the use of & in a URL when redirecting via JavaScript. This would accidently represent the URL above like so: http://www.example.com/index.php?act=test&do=something However if you do use & to seperate URL's in HTML (which you should) it would take you to: http://www.example.com/index.php?act=test&do=something
-
Reverse the array first? Check out the function array_reverse
-
I agree. They are all pretty awful. But if my life depended on it, I would choose Lutaro.
-
Hmm... it seems your objective here is very strange. You are trying to instanitiate the object with an initiation function that messes with the variables, yet you want to echo a value from an array of which you have changed in the past. Your best idea would be to do the following: <?php class info { var $name = array ("Jeff", "Ducky", "Zach"); var $age = array ("18", "19", "20"); var $group = array ("1", "2", "3", "4", "5"); function info($num) { $this->name = "Hello, my name is ". $this->name[$num] .", "; $this->age = "and I am ". $this->age[$num] ." year's old"; $this->group = $this->group[$num]; } } $info = array(); $info[0] = new info(0); // Object 1 $info[1] = new info(1); // Object 2 echo <<<EOT {$info[0]->name}{$info[0]->age}, and am in group {$info[0]->group}! EOT; ?>
-
Theres different ways of doing this. It's not as easy as it may look. You may want to check out some XML tutorials online or use an XML Parser. I believe there are a few.
-
I'm not sure if UPDATE produces an error. If there are no rows to edit, it should just not update any rows. Check that the user cookie is actually set and see what value it is.
-
PHP: Impossible to instal on vista?
Darklink replied to s_ff_da_b_ff's topic in PHP Installation and Configuration
Come to think of it, I've never heard of ANYONE installing php on a vista operating system. I would be interested if knowing if anyone has had any successful installations on the system. Although I am absolutely sure it's possible to install it on Vista. I wander if a preset LAMP installation will work such as EasyPHP. Although those are purely for development purposes only. You wouldn't expect to use vista for a server!