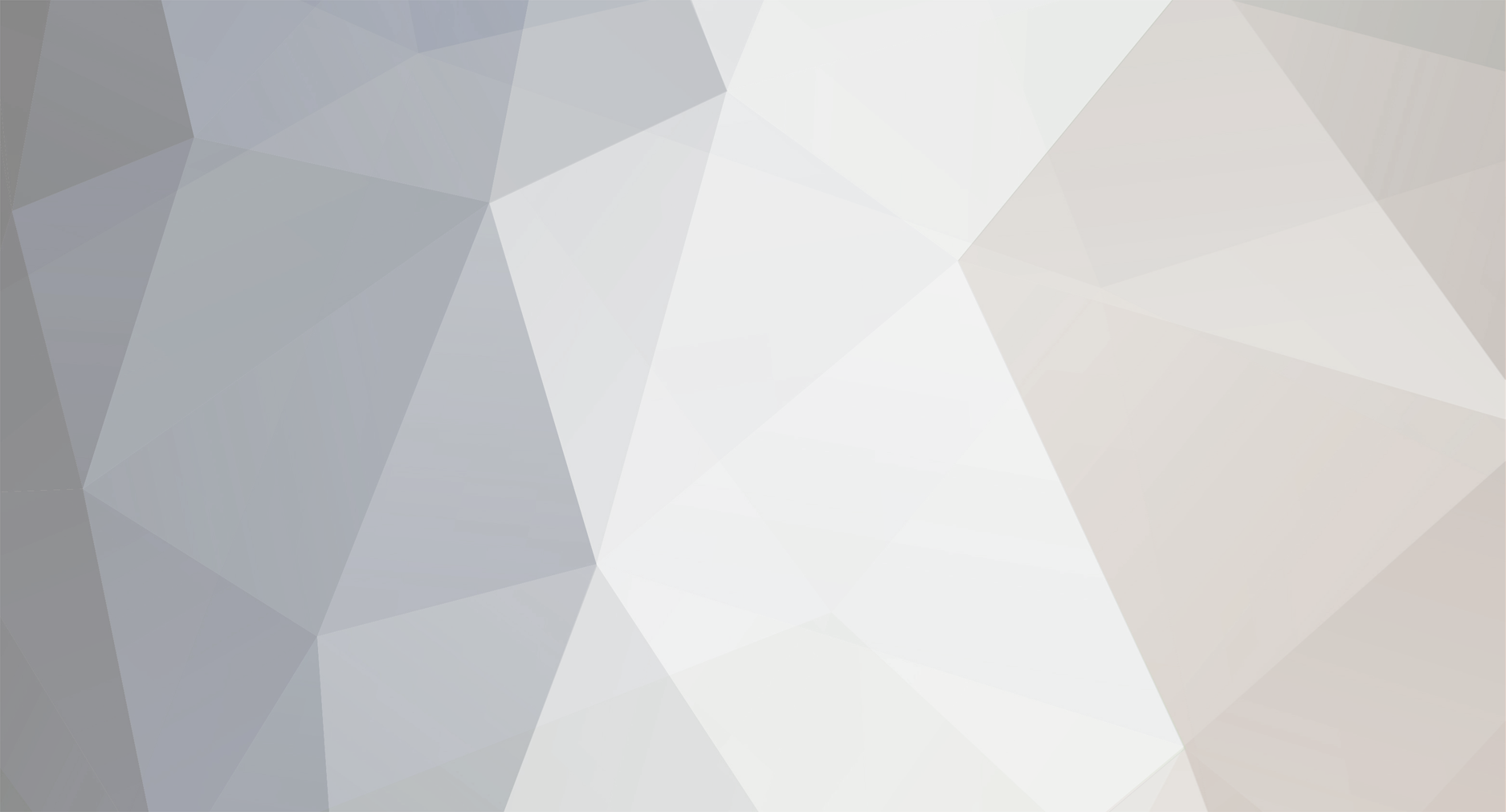
HGeneAnthony
-
Posts
95 -
Joined
-
Last visited
Posts posted by HGeneAnthony
-
-
Most frameworks make it very easy to program according to the Model, View, Controller design pattern. This alone makes them worth a lot. They also offer a lot of helpers for common tasks that are undefined in PHP like creating pagination. They also offer more advanced features for things like sessions, post variables, etc. They will cut down development time and more importantly make it easier to maintain your program. Most frameworks are designed to give PHP users some of the best features of Ruby on Rails, which is supposedly insanely powerful.
-
I meant arguments. I don't want to have to pass the __LINE__, __FILE__, __CLASS__, etc. each time I call a log or error. I would just like to use a log function and have it grab the data of the function that called it.
-
I'm creating a logging class and I'd like to record the function name where an error was logged, as well as line number, file name, etc. I know I can pass the details __LINE__, __FILE__, __FUNCTION__, __CLASS__, as arguments to a function but this is a pain in the ass to do this for every error and it makes the code look sloppy. I would like to just put these details in the error function so I can keep it clean, however this will put in the current function details not the calling function so I was wondering is there a way to do this?
-
Thank you I wish I knew about these before they're perfect for the job. Appreciate it!
-
I know how to check to see if a variable is an instanceof a class that I define, however I don't know how to check if it's a string, int, double, etc. Let's say this was my code how could I modify it to check if the data is a string:
$name = "gene";
if ($name instanceof string):
echo "This is a string";
else:
echo "This isn't a string";
endif;
-
Yeah I agree a little harse. Frameworks serve different purposes but the main purpose of any framework is to make you more productive. If you were looking for a quality framework I'm partial to Kohana. It requires a bit of a learning curve but once you do it makes you far more productive, and makes you design good code from the start. It also provides a ton of helper classes that can abstract you from more complicated things like database access as well as allow you to use PHP to write things that would normally need to be done in HTML, plus it can give you some powerful tools to cut down on development time. Go to KohanaPHP.com and read up on it it has very nice documentation. Sorry I'm in a rush or I'd tell you more.
-
Another trick I forgot to mention is creating your own Error class that can manage all errors and take different actions based on type. You create variables for type (where the error is user login for example), level (whether it's a user error like unsuccessful login or system error like database not available), message text, where the error occurred (line, file, etc.), etc. Then you can manage message errors by automatically creating log files for system errors or having it email you if you wish. You can use a database to log messages instead if you prefer. A class gives you a lot of flexibility for the future as well.
-
Been using Aptana for my PHP. I've been very happy with it. I used to use XDebug I'll have to download that again. Error Reporting is pretty standard though I always leave that on.
I tend to be big on doing it right the first time. Experience has taught me that if you put more effort in at the beginning you have less issues down the road and it ends up saving far more time later on.
-
I also forgot to mention one technique I heard of was done by ruby on rails where there's 3 projects going on simultaneously a development, test, and distribution along with 3 equally named databases. Does anyone use this method here. Also I use a SVN repository which I use to sync my updates with.
-
I've been working on developing better coding techniques for awhile now but I don't have all the answers so I was wondering other things you think I should do that I'm currently not doing.
1. I use psuedocode to define the logic of my functions before I write them. Later they work for comments.
2. I stress object oriented techniques and I try to keep my functions small with one central purpose, well labeled, and loose coupled.
3. My classes have strong cohesion
4. I make use of asserts for assumptions I assume to always be correct
5. I use constants for any numbers defined. I keep my code clean of numbers whenever possible.
6. I use an MVC design pattern for my programs
7. I try to make use of Unit testing and Mock objects
8. I'm trying to make more use of debugging but PHP doesn't seem very good for that
A couple of things I'd like to learn is how to people profile an application? Are there any good tools for debugging PHP? Any of tips you can think of?
-
I'm interested in implementing a fulltext search for my site and I'm trying to decide between 2 different ones. First is MySQL's native fulltext search. The perk is it's native and offers limits to results returned. It's negatives is that it can't use innodb tables, doesn't offer half of the available functions of Lucene's search, and it doesn't tokenize a field so to get an accurate result if you have 30 keywords you need 30 fields. I also hear it's slow for large results. Then we have Lucene. Lucene is part of the Zend framework and offers a standalone index. The perks are it's fast and feature rich. It also tokenizes fields. It's negatives are that it (as far as I've seen) only returns full results not limits and that I need to maintain something else for my site when MySQL offers a native solution. I'm wondering what opinions others have about this topic.
-
I was recently reading up on ArrayObject and ArrayIterator and I had a few questions. I was hoping someone hear can answer them.
1) I read that an ArrayIterator doesn't store the contents of the array in memory just the current item. So where does it store the items?
2) Is there a way to store a multi dimensional array in an ArrayObject?
-
I'm looking for a tool to give xpath like functionality to json. I tried out jsonpath but I'm getting a
"Cannot use object of type stdClass as array" error running their code. Any one know of a better class?
-
Wasn't sure how this was done. Thank you for your help.
-
I was using Kohana (which is based on CodeIgniter but PHP5 oriented) and one thing I find interesting is how it can use functions that are generated at runtime. For example, for it's ORM database frontend it wraps sql statements and creates functions using field names like find_by_name() where name is a field name. It also gives you ways to access the field names like $user->name. I can think of a few uses for this and I was wondering how they do this? Is there a way to catch invalid function names with PHP and pass them to another function? IE I call find_by_name('gene') and the class sees the request and then passes the function name and argument to another function which then handles the event? Any ideas?
-
What I'd like to do is be able to edit my settings for my mysql database in a directory structure like a registry. You know I could double click on a setting and change the value. Settings are grouped together in a folder to make it easy to edit in a group, etc. and I was thinking about writing something but I have to figure someone else already created something like this so I'm wondering if anyone knows of a program. I figured as long as you kept a similar structure it should work. Any help?
-
I'm not trying to say anything bad about the others. Zend is just the first one I discovered and I tended to like some of their tools. It seems by reviews though a lot of people are fans of Code Igniter. By the way I heard these frameworks were meant to be PHP versions of Ruby on Rails (which I've been hearing a lot about). Do you know how similar they are?
-
I would like to perform fast filtering searches so I was wondering if MySQL lets you perform a query based on your last query retrieved? Also what would be the ideal uses for a memory table in MySQL? I know it should be lightening fast but I was wondering where I should implement one.
-
I do like some of the features Zend offers like it's port of Lucene search. I don't know if Code Igniter offers a full text search but this is a port of the Java version and offers some great search criteria options beyond what you could get with Google and such. I do like though that Zend seems to be an extension of what you are already doing, hence you can import it and get its functions even in projects you started years ago. Code Igniter and Kohana seem to require you to build your app around their system from the start. I have heard though Code Igniter is definitely the fastest framework.
-
I've been looking into frameworks lately and I stumbled upon CodeIgniter and the subsequently Kohana. There are some things I like about the concepts but I was wondering how good they are? They have some nice features but I'm not huge on how arguments are passed, etc. Has anyone used them here and what opinions do you have on them if you have? Also FYI I would recommend checking out the Zend Framework. It has some very nice features and I think it's a tremendous framework.
-
I'm working on a project where I need to have 1 frame displaying a web site and the other side (javascript) parsing data from it. I was wondering how can I grab the source string from the other frame. I don't want to use a function that goes out and grabs the page from the website itself. The web sites have anti scrapping techniques in place which prevents them.
-
I'm working on a project in which I'd like to filter data out of several pages and combine them into an XML document. PHP has a command to grab the source of a page but some websites are worried about people scrapping their sites so I was thinking about displaying the content in another frame and using PHP to grab the source from the frame. Is this possible?
-
Thank you for the suggestion. I never used paypal before I wasn't aware of this. I'll have to look into them.
-
I need to set up a shopping cart for my business and I'm currently looking into open source shopping carts primarily CRE Loaded or Zen. Although these systems can handle the interface nicely I don't know how I could process credit card orders. Is there a company I should go through that handles transactions for a fee, or is it easy to integrate this with my existing credit card machine. I'm on a shoestring budget so I'm trying to keep cost low. Any help?
How to make 404 page for Kohana Framework
in Frameworks
Posted
I'd switch off Kohana if I was you. I used to use it for years, however their documentation tends to be incomplete, they break old cold with new updates, and they don't offer downloadable documentation. It's also hard to find help with it because it's really not that popular. I switched to codeigniter and I've been happier with it.