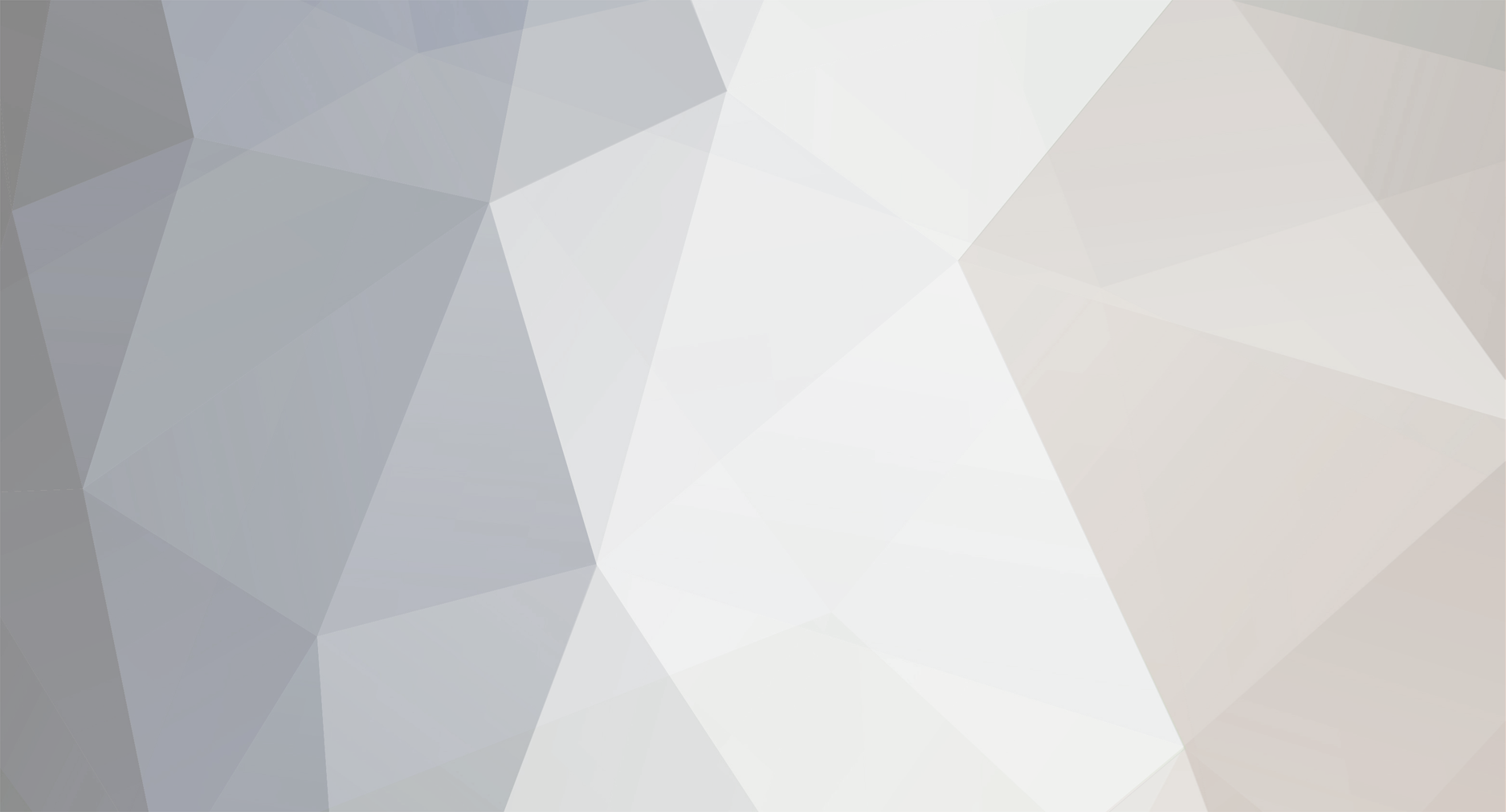
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
register_globals would be the default problem if you haven't changed anything It causes exactly the problem you describe. When the script starts, it sets your standard variables from the values in $_SESSION, $_GET, $_POST and $_COOKIE according to a fixed set of rules (the rules can be changed also, if you're that keen). Some people like it for the convenience, but it's safer to switch it off if you are able to. And indeed they are the same variables. If you modify $username, then you will be modifying $_SESSION['username'] (providing it existed in $_SESSION when you called session_start()). A variable which hasn't been placed in $_SESSION will not magically appear in $_SESSION however, which makes sense.
-
PLEASE HELP! mutliple field search in PHP/mySQL
btherl replied to monkeywright's topic in PHP Coding Help
Yes, part of the problem is the name/id of each field. You'll need to make seperate fields for each possible input, and name them appropriately. Eg <td width="72">Company</td> <td colspan="2"><input type="text" name="title"></td> That's the most straightforward anyway. Then you can see which of your inputs are set, and construct the SQL. It'll need to be constructed dynamically, such as: $sql = "Select * from LISTINGS WHERE "; $sep = ''; if (!empty($title)) { $sql .= $sep . "title like '%$title%' "; $sep = ' AND '; } if (!empty($website)) { ... With one "if" for each possible input. Well, there's other ways, but this way will work. And don't worry if it's tough, it's not that simple -
var_dump($var) will tell you the type of a variables. Very handy for those type-related debugging sessions.
-
You can store the generated values in a session, like this: session_start(); # At the very top of the script $_SESSION['keyone'] = $keyone; $_SESSION['keytwo'] = $keytwo; $_SESSION['funcid'] = $funcid; Then there's no need to convert back. You need to clear this data once the test has been completed, to make sure the same data can't be re-used by a bot. It also somewhat defeats the purpose to let the bot know the names of the images you are using I guess you will fix that later.
-
Try this: $add_app = " INSERT INTO `apps` ( `$newapp` ) VALUES (NULL , '$title');"; mysql_query($add_spp) or die("Error in $add_app\n" . mysql_error());
-
Try this: if (preg_match('|/([0-9]+)\.php$|', $file, &$matches) === 1) { print "Matched {$matches[1]}\n"; } Then you can use $matches[1] to construct your new path (if all you want to do is replace mydir with NEWmydir)
-
Best is to combine both approaches. Post hides the data from the URL when logging in, and sessions keep the "logged in status" stored on php's side, rather than alterable by the user. For high security applications, SSL (in addition to the other two techniques) will prevent the post data from being intercepted while travelling over the network.
-
Halp with a piece of code (cookies, mysql, php)
btherl replied to Antonella's topic in PHP Coding Help
You have a lot of repeated code there.. for example, you call setcookie() 3 times for the same cookie. And the entire script is repeated twice. As for debugging it, I suggest printing out variables throughout the script, such as the values of $newskin, $_COOKIE and $_REQUEST -
First mail() statement works; second one intermittent?
btherl replied to bgoulette612's topic in PHP Coding Help
Were they failed mail delivery notifications or were they the actual emails you sent? If they failed it should give a reason. Either way, it looks like your php script is fine It's what happens to the email after php sends it that's the problem. Normally mail logs will be in /var/log .. where exactly depends on which package you are using. /var/log/mail.log and /var/log/postfix often have something useful in them. -
Use this: curl_setopt($ua, CURLOPT_USERAGENT, 'Mozilla 0.0.1 Apple ][e 8-bit'); If you wanted to spoof an Apple II browser, for example.
-
Do you want to keep only those column names in the array which aren't empty? Everything except the blank one?
-
No, as far as I know. Try doing a tutorial on mysql in php. Once you've got the basics, you can easily grab an SQL query from phpmyadmin and integrate it into your php script.
-
First mail() statement works; second one intermittent?
btherl replied to bgoulette612's topic in PHP Coding Help
I've tried the "staring at the code" approach, but it all looks fine to me. Hmm.. do you have access to the mail logs? If mail() returns true, that means php is telling you that the mail system accepted the message for delivery. If it's not getting through, then the mail system must have done something with it.. maybe it tagged it as spam and ate it or something similar. -
I believe the problem may be that you add the item to $_SESSION['items_in_sale'] before you check for duplicates. Also, $k should start at 0 (as in the first code you posted), not 1. You can check this by using var_dump($_SESSION['items_in_sale']). That will show you that the first array index is 0.
-
First mail() statement works; second one intermittent?
btherl replied to bgoulette612's topic in PHP Coding Help
Checking the return value of mail() would be a good start. My next step would be to print out all the values you pass to mail() and do a manual inspection. -
This may be the problem http://sg.php.net/manual/en/ref.pdo-sqlite.php My understanding of the docs is that sqlite version 2 uses the old interface, but sqlite version 3 must use the PDO interface, not the direct sqlite interface (and the sqlite_* functions)
-
Try printing out the values you are comparing inside the for loop. You may find something you don't expect, which will lead you in the right direction to fixing it. You might also want to print_r($_SESSION) or var_dump($_SESSION) Based on only the code you gave, I can't give any specific advice, because there is not enough information.
-
Need help in working with Class/Object using PHP/HTML
btherl replied to navjak's topic in PHP Coding Help
Try adding more echo statements until you find the exact location that execution stops. That may give you enough clues to fix it. -
exec only returning first line of output when run through apache
btherl replied to BrianK's topic in PHP Coding Help
Ok, now that i've read the question properly That is odd. What if you try another function like shell_exec()? When you've got behaviour that doesn't make sense, sometimes a solution that doesn't make sense will work -
newbie: sql injection question - is this script secure?
btherl replied to chelnov63's topic in PHP Coding Help
The script is secure from sql injection, since you use mysql_real_escape_string() on your inputs immediately before using them, and you place single quotes around them. BUT, you might want to check that unusual passwords, particular ones including html special characters like less than, greater than and so on, are handled correctly. You may need to urldecode() your inputs before escaping them for mysql. -
If you post the data and use SSL, then you have a high level of security. The data will be encrypted all the way through the network.
-
[SOLVED] Using && and || Operator in same line??
btherl replied to GregL83's topic in PHP Coding Help
You can use brackets to enforce the order of evaluation. Another method is to create temporary variables to hold intermediate results, and then use those temporary variables in the "if". Temporary variables often results in MUCH more readable code. Example: $submitted = isset($_POST['submit']; $item_valid = ($item == "width" or $item == "height"); if ($submitted && $item_valid) { } Also note that you must use == or === when testing values, NEVER =. "=" will set the variable to a new value. -
It's very difficult to detect from the server side, particularly if the user resumes the download. The thing is, even if you send out the file, you don't know for 100% if the user got it or not. If you have all your downloads go through a script then you can at least track the number of download attempts. If it's critical that the user receives the complete file, there are two main options i can think of 1. Have the user tell you that they received the file. 2. Allow re-downloading of the file in case the first attempt fails, giving the user a chance to complete the download. Then assume that if they don't try again within some specified time period, they probably got the file.
-
Try with this little alteration: <?php if (!isset($_GET) || !isset($_POST)) { $arraySer = urlencode(serialize($array)); print '<input type="hidden" name="productids" value="' . $arraySer . '" />'; }else { $arraySer = (isset($_POST['productids']))?$_POST['productids']:$_GET['productids']; $arraySerDec = urldecode($arraySer); $array = unserialize($arraySerDec); print_r($array, true); } ?> If it doesn't work, try inspecting the HTML source. Also try printout out the variables to see if they look sane. Actually, urlencode() is not necessary .. there's a more appropriate function but it slips my mind right now.. maybe htmlentities()
-
What do you mean by "When I enter my variable $Address in all I get back is null." ? What do you get if you print out $mapaddress and $url?