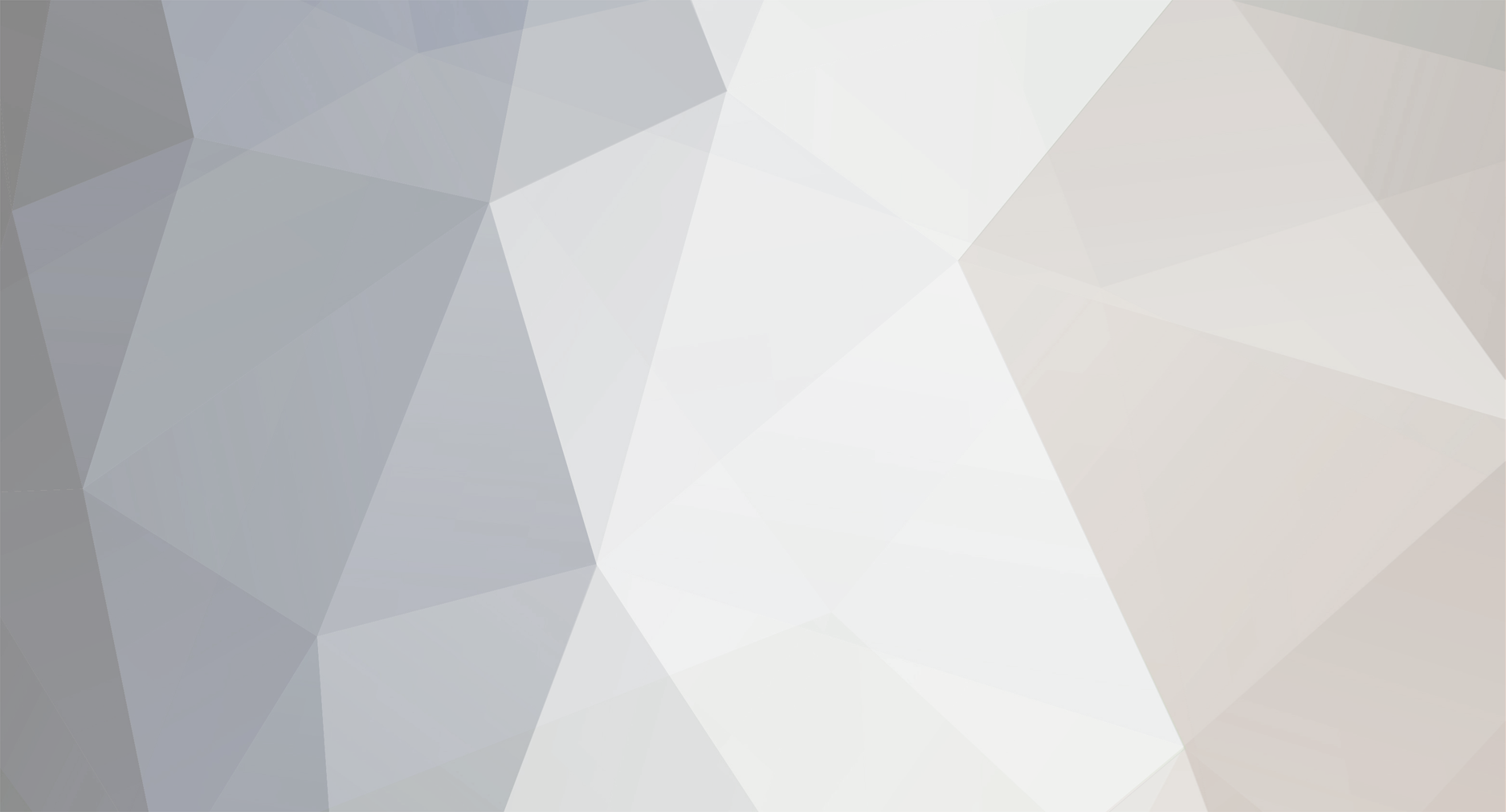
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
Objects are a convenient way of grouping data in one spot, along with the functions related to that data. They also have features for declaring what data and functions are public and private, also for convenience. That's all. End of story Here's an example: $cat = array( 'name' => 'Kitty', 'food' => 'Whiskas', 'sound' => 'Mrrow', ); function cat_sound($cat) { print "{$cat['name']} goes {$cat['sound']}\n"; } or.. class Cat { var $name; var $food; var $sound; function sound() { print "{$this->name} goes {$this->sound}\n"; } } $kitty = new Cat(); $kitty->name = 'Kitty'; $kitty->food = 'Whiskas'; $kitty->sound = 'Mrrow'; $kitty->sound(); Yep, it's not all that different. Some people like it, some people don't. I still write traditional style code, even though I understand OO and know that it is available. Serious OO programmers would provide methods for getting and setting name, food and sound, which also allows the class to decide how to process data as it goes into and out of the class.
-
"When used in conjunction with the optional extension attribute ZEROFILL, the default padding of spaces is replaced with zeros. For example, for a column declared as INT(5) ZEROFILL, a value of 4 is retrieved as 00004. Note that if you store larger values than the display width in an integer column, you may experience problems when MySQL generates temporary tables for some complicated joins, because in these cases MySQL assumes that the data fits into the original column width." Taken from http://dev.mysql.com/doc/refman/5.1/en/numeric-types.html Note that zerofill affects display only, not storage.
-
Need a better way to write a select query that repeats subqueries...?
btherl replied to Balagi's topic in MySQL Help
That's a tough one. Yes, adding a rank field will drive you bonkers trying to keep it up to date. You might want to do it in two stages. First fetch it in rank order, then have php add in the ranks, then sort it in php in your display order. You could do something similar in mysql using a temporary table I suppose.. but I'm not sure how you would do that, being a postgres user. -
[SOLVED] Is using JOIN to access multiple tables a Hard Must?
btherl replied to chadrt's topic in MySQL Help
Hmm.. do you have indexes on both callsign and unique_id on all table? Not having those indexes would cause a VERY slow query, if those tables are large. Try these: CREATE INDEX EN_unique_id ON EN (unique_id); CREATE INDEX EN_callsign ON EN (callsign); Both of those for each table. If it doesn't help, then run your query with "EXPLAIN" at the start, like "EXPLAIN SELECT ...", and post the output here. -
[SOLVED] Is using JOIN to access multiple tables a Hard Must?
btherl replied to chadrt's topic in MySQL Help
If the unique ids match between tables, then you can try something like this: SELECT * FROM HD JOIN EN USING (unique_id) JOIN AM USING (unique_id) WHERE HD.callsign = 'blah' That will look up callsigns using the HD table, and then use the unique ids to find matching rows from the other two tables. Is that what you're looking for? You might also want to try this condition: WHERE HD.callsign = 'blah' OR AM.callsign = 'blah' OR EN.callsign = 'blah' -
I was intending to show the difference between using a variable directly, and using a string I think that using "string $var" or "." style in the arguments to sprintf is fine, just not in the format string. Using it in the format string is missing the point of sprintf altogether.
-
This information is normally available in the webserver logs. Can you access those? I have php scripts which analyze the logs and generate counts of actual hits.
-
The others have given you 90% of the story, so here's the last 10% .. all rules can be broken. But you can only break them when you understand them fully. There ARE situtations in which using @ is the right thing to do. I use it when dealing with functions that produce warnings for failure conditions that I am actually expecting to happen. But those situations are very rare.
-
Here's an example $i = 0; while ($i < 10) { $str = sprintf("[%s] %s\n", $i, "This is row $i"); print $str; $i++; } The % placeholders match the order of the arguments. So the first one is replaced by $i, and the second replaced by "This is row $i". It's usually a bad idea to mix "." style and sprintf() style. They are two different ways to accomplish the same thing.
-
So the message you see is "File number 1 cannot be moved"?
-
Please post your full code (or enough to generate the error), and fix your variable names, particularly here: function add_class2_object_to_array ($xclass2_object) { array_push($this->arreglomochilas, $xobjetomochila); }
-
Hmm.. is the query fast if you don't join to those two other tables? Just the match?
-
Can you show output for "explain select ..." for your query?
-
$ip1 = getenv('REMOTE_ADDR'); $q="select * from kawalan_punch where id_kursus ='$id_kursus'"; $are = mysql_query($q) or die("Error in $q\n" . mysql_error()); $row=mysql_fetch_array($are); $ip2=$row["ip_client"]; I added a missing line. Where does $id_kursus come from? What do you want the code to do?
-
What happens when you run the query manually, such as in phpmyadmin? Also are you sure the hang happens during query execution? Try adding "exit(0);" immediately before the query and run the script, then move it immediately after the query and run the script again. Is your database identical (including indexes) on your dev box and on the live server?
-
That's an issue with your server configuration. You should talk to your hosting provider about it. Btw, it's not related to curl. You'll have the same problem with any php script.
-
It looks pretty good.. though it validates ",123". I can't think of any nice way to fix that. You could say (1-3 digits OR (1-3 digits followed by 0-n sets of , and 3 digits)) followed by optional "." and so on That way you ensure that commas always follow a digit group, while still making them optional.
-
Warning: Unknown: failed to open stream error
btherl replied to onewaylife's topic in PHP Coding Help
What did you change just before the error started? -
If you are constructing the URL in php, do it like this: $url = 'my.php?myvar=' . urlencode($myvar); When browsers submit form data, they do this process automatically, so it's only necessary for when you construct your own urls. When you receive the data in $_REQUEST['myvar'], you must use urldecode() to convert it back.
-
I tested your claim, akitchin.. $a = "$arr[id]"; is much the same speed as $a = "{$arr['id']"; But, $a = $arr[id]; is three times slower than $a = $arr['id']; Very interesting results there.. it seems that the presence of double quotes changes things greatly. Results for 5 million runs of each statements were: $a = $arr[id]; # 15 seconds $a = $arr['id']; # 5 seconds $a = "$arr[id]"; # 9.5 seconds $a = "{$arr['id']}"; # 9.5 seconds
-
Hmm.. Based on the example you posted, you will need to restrict your data type in the table definition as well as in the index to the same value. Only then will mysql know that the index contains all needed data. Text actually has a much greater range than 255 characters.. it's only a text index that's limited to 255 characters. So you must limit the table data to be no larger than the index length if you want the index to contain all the data. I wouldn't recommend doing that though. "ALL" is not so bad, when you're only reading 3 integers and a text column in each row. Putting the entire ext column into an index won't help all that much, since the index will be nearly as big as the table itself.
-
You might be interested in the comments here: http://sg.php.net/manual/en/function.is-numeric.php#74700 As for that regexp, a little commenting would help It looks like perl code to me (sorry perl programmers)
-
Need a better way to write a select query that repeats subqueries...?
btherl replied to Balagi's topic in MySQL Help
Queries like "most recent" or "biggest" are usually messy in SQL, when combined with straightforward queries. If I have to do such queries very often, AND it is causing performance trouble, I usually cache the values I'm interested in. For your case, you could add a "most recent project for each student" table, which stores the most recent project. That can be joined back with the projects table to fetch the date. That table will need to be updated each time a project is created, deleted, or has its date altered. -
While I can't answer your question, I can suggest using CASE to choose between tables. Or partitioning may suit your needs, if you are splitting the tables for efficiency rather than for design reasons. Looks like partitioning in MySQL was only introduced in 5.1. Partitioning is very powerful when used appropriately.
-
In my first post or last post? It looks somewhat relevant to both My first post wasn't quite right because I didn't realize that mysql could fetch all the column data from an index, if a suitable index was found. So yes, it must fetch all rows, but it can choose either full table scan or "full index scan", if a suitable index can be found. Restricted indexes like sales_pitch(10) are no good because the index only contains partial data. Regarding what the book said about ALL usually being bad.. it did say usually. In most queries, it's bad. But for your particular query, ALL is what you would expect, unless there happens to be a matching index for the columns you are fetching.