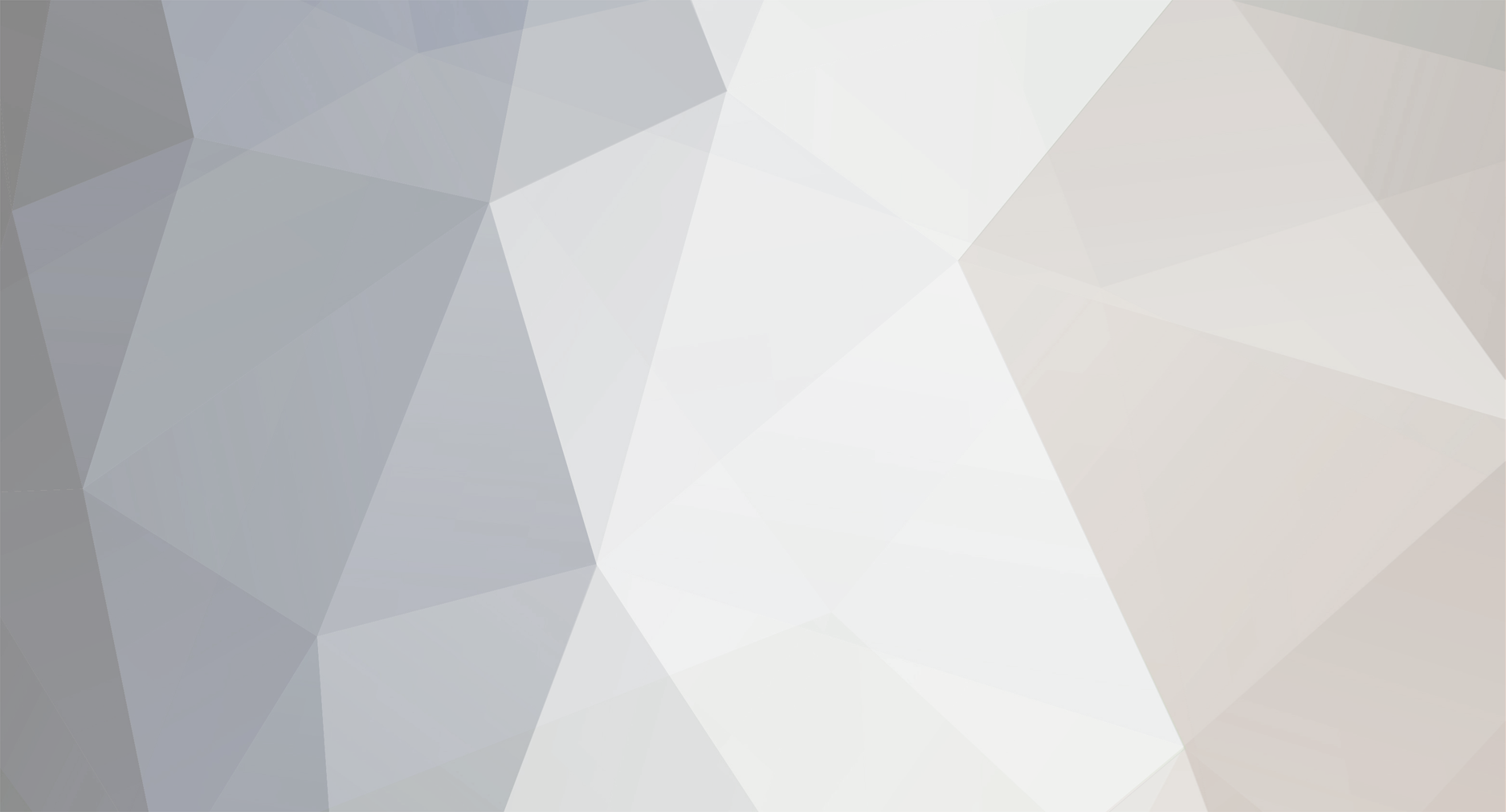
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
Try the check I posted.. it'll tell you not just that the query failed, but also the reason why it failed. Plus it will show you the query, which will show you if one of the input variables was not what you expected it to be. It can save a lot of debugging time
-
Just printing out the data should be fine, using print or echo. Your client program should parse the HTTP headers as text, then treat the document itself as binary data. Something like: <?php if ($_GET['file'] == 1) { readfile("/path/file1"); } elseif ($_GET['file'] == 2) { readfile("/path/file2"); } ought to work (This is a simple example with no error checking). If you were dealing with a browser, you would have to set appropriate content-type and content-disposition headers, but since it's your own program there's no problem.
-
From my understanding of your situation, it's not possible unless the third party allows you to give them a template to use. Actually, it is possible to call the form using php or javascript, providing you parse the html yourself and add in your nav bar before displaying the results. That approach is dangerous though, as you could easily break the third party site's operation. Frames are much safer due to the clear seperation between the sites.
-
IF statement - not running correctly, prob something simple
btherl replied to Canman2005's topic in PHP Coding Help
Try echo "GET_folder = " . $_GET['folder'] . "<br>"; either just before the if, or inside the if. -
Looks ok, except now() and null should not be quoted. Try "echo $q" also so you can see the final query. And it's good to have a check like this: $addinfo = mysql_query($q) or die("Error in $q: " . mysql_error());
-
Name retrieved from mysql_query won't show up in echo
btherl replied to Lt. Geyser's topic in PHP Coding Help
It should be this: $player_name_result = mysql_query("SELECT lName FROM Player WHERE playerID = $player") or die(mysql_error()); if (mysql_num_rows($player_name_result) == 1) { $player_name = mysql_result($player_name_result, 0, 0); } There are many other ways to get the data out of a mysql result. -
There's also the saying "If it ain't broke, don't fix it" Ideally you need a way to measure the performance. That way you know if there really is a problem. Measuring and logging query times is a good way to do this. 1 million rows is probably fine as long as you are using an index. If there is a problem, then I think the biggest wins you will get are by archiving off old rows (this is what friendster does for example, it only tells you about recent views) or by partitioning your table by username (for example, all users starting with 'a' in one table, all starting with 'b' in another table, etc etc) You can do broad partitioning, like A-K, L-M, N-Z or very fine partitioning, such as every starting letter. This basically gives you lots of small tables instead of one big table. But once again, don't fix it unless it's actually broken If you commonly do time range queries, like 'all users in the last month', then partitioning by date may be appropriate.
-
Is there any easier way to count rows in multiple columns
btherl replied to rcorlew's topic in MySQL Help
Those are three tables, not three columns Better is to do it like this: $result = mysql_query("SELECT count(*) FROM users", $con) or die(mysql_error()); $usercount = mysql_result($result, 0, 0); When you give mysql a count(*) query instead of just select *, then it is able to do significant optimizations, because it knows you only want the count and not the actual results themselves. -
http://sg2.php.net/manual/en/reference.pcre.pattern.modifiers.php
-
[SOLVED] Logging out after a certain time.
btherl replied to HappyPandaFace's topic in PHP Coding Help
To add to Glyde's good suggestion, if you have a high volume of users you may want to make it so that the check is only done some of the time, not all of the time. For example, if you have 10 hits per second, you might set it so the check is done every 100 hits (every 10 seconds). A simple way to do this is if (rand(1,100) == 1) { # do the check } -
[SOLVED] returning mulitple variables from a function
btherl replied to s0c0's topic in PHP Coding Help
Try doing just $a = $DragonAct->__construct($action); and then var_dump($a);. That lets you inspect the return value before trying to assign it with list(). Your code looks ok when action is sword, but not ok when action is arrow. As a side note, it seems odd to me to have code like that in a constructor, if the object represents a Dragon. -
It's an odd request. I would start be selecting all rows where that column is empty, in order of increasing submitid, and take the first row returned. Then put the data into that row. I would do that for each column. Then if you have data left over, create a new row and put it in there.
-
Do you want "John Brown" to show up when you press B? If so, that'll require modification of the javascript rather than your php. That's because it's the javascript that decides which strings to display when you press a letter.
-
To apply a condition to an aggregated result (such as sum(), count(), max(), min()), you need to use HAVING instead of WHERE. The reason is that WHERE is applied before the sum is calculated, and HAVING is applied afterwards. SELECT d.dnumber, SUM( p.points ) AS points FROM dogs d JOIN points p ON d.dnumber = p.dog GROUP BY p.dog HAVING SUM(p.points) >= 20000
-
If you're using unix, you may want to call the external gzip command exec("gzip $file"); If that's available, it's by far the easiest way. The compressed file will be named "$file.gz". Make sure that you validate $file if it comes from data sent by the user, otherwise they can easily hack your site.
-
Is there a way to protect the a PHP site from being copied?
btherl replied to gondalmk's topic in PHP Coding Help
You get what you pay for. There's plenty of other, cheaper obfuscators on listed on that site. If the administrator of the site is not an experienced programmer, then simple obfuscation will be enough to stop him using your code. It takes quite some skill to convert obfuscated code back into something useable. -
Yes, you're getting it Those instances are what you need to store and restore. There's a little catch here with sessions and objects.. When you call session_start(), the class definition must be available. PHP can't restore an instance of class Dragon unless you've included the definition of Dragon already. So make sure you include the Dragon class on every page that will use it, before calling session_start(). So require_once('actions.php'); # Contains class definitions session_start(); # Restore stored classes, now that class definitions are available $dragon = $_SESSION['dragon']; # Get the dragon out from storage $dragon->dance(); # Make the dragon dance!
-
Sorry, I didn't read your post properly. It sounds like permissions then.. I'm sure sudo can do it, but I've never configured it before. Are you using a packaged hosting service?
-
Update MySQL database record one bye one, but ................
btherl replied to Kiongkavi's topic in PHP Coding Help
Hmm.. is the problem that you read the data from the database before doing the update? -
Regarding your mysql .. are you 100% sure that you are having "Yes" and "No" converted to bools? Did you check them before you put them into the database, while they are in the database and also when you took them out? The reason I am suspicious is that SQL interfaces, including php's mysql interface, always return strings. You can see this in the return type on this page: http://sg.php.net/manual/en/function.mysql-result.php The function cannot return a boolean. Additionally, Mysql does not even have a boolean data type. It uses integers. Can you try var_dump($field) immediately after the code you posted?
-
iptables is probably not in your path. Try /sbin/iptables or /usr/sbin/iptables. It's a good habit to use exact paths too, for security. I would expect it to require root access.. you wouldn't want normal users examining your firewall rules. I can't advise you on how to set that up though.
-
Update MySQL database record one bye one, but ................
btherl replied to Kiongkavi's topic in PHP Coding Help
Try printing out your queries. I notice that you print out the values for your queries, but it's much MUCH better to print the queries themselves. I am particularly suspicious of this code: $query_B2_2="update putonServer SET id2=1 where id='$next_id'"; echo $this_id.$newline; The query uses $next_id, but you echo out $this_id (for debugging?) -
How are you accessing the data (both the ini files and the database)? With which functions?
-
while loop in a foreach loop.... semi-results!
btherl replied to suttercain's topic in PHP Coding Help
Try this $val_escaped = mysql_real_escape_string($val); $rez = mysql_query ("SELECT name, character_id FROM characters WHERE name = '$val_escaped'") or die(mysql_error()); The die() is just to make sure there's no errors in your query. You could do it with a single query as well, which will be more complex but also more efficient. -
Upgrading to the latest versions of your software may help. Reinstalling them would also be a good idea. In the meantime, you may want to add password authentication to your entire site while you mop up. As for the hack modifications being instantly restored, you might try the simple approach of renaming your index page until you can find out what's going on.