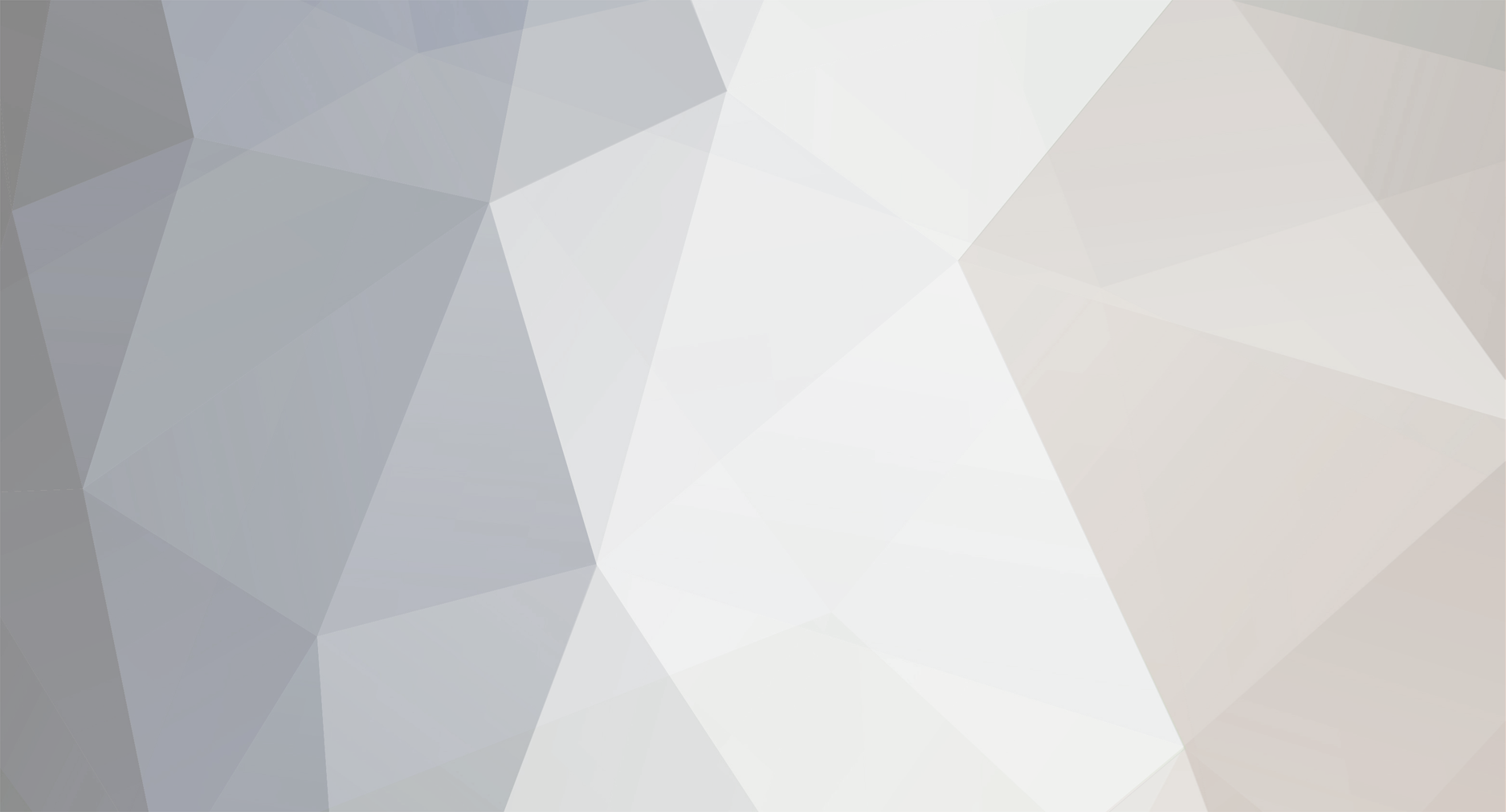
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
Do you have indexes for your common queries?
-
I would guess it's because your original query produces multiple columns with the same column name but different table name. When you "AS" your subquery, you are creating a conflict, as Mysql doesn't know which one to use. In postgres it looks like this: live:parking=> \d import_metadata; Table "public.import_metadata" Column | Type | Modifiers --------+-------------------+----------- name | character varying | not null value | character varying | Indexes: "import_metadata_pkey" primary key, btree (name) live:parking=> select foo.value from (select * from import_metadata as im1 join import_metadata as im2 using (name)) as foo; ERROR: column reference "value" is ambiguous In your case, it's thinking "There's more than one article_id in the subquery results, which one is articles.article_id?" Was it intended that you list na.article_id twice in the select list?
-
You can't just drop them.. the grouping and having in each clause is how Toxi implements "AND". If you dropped the inner group bys, you would lose the meaning of "life & run & jump" for example. That requires the group by and "having count(qt.id) = 3". What fenway is suggesting is just to do the query as it is, accept that it's going to produce duplicates, but then remove the duplicates by wrapping EVERYTHING inside another query that only does duplicate removal. GROUP BY and DISTINCT are equivalent for straight duplicate removal. And mysql lets you get away with only specifiying qt.id. Something like: SELECT * FROM ( ... your entire current query in here ... ) GROUP BY qt.id So we will still have no idea why the union distinct doesn't work, but at least you'll be getting the results you want
-
Change display_errors to on.
-
You should use readfile() instead, as suggested in the manual: http://sg.php.net/manual/en/function.include.php But I don't expect that will fix the error. If you're familiar with curl, try connecting to the URL using that and see what happens. "Connection refused" indicates that php can't connect to the webserver.
-
A possible replacement for that array would be $mon = range(0, 20); $positions = implode(',', $mon); $sql .= "WHERE team='$team_ident' AND position IN ($positions)"; If you wanted to use that array in a foreach loop, you would do foreach ($mon as $m) { print "Array element is $m\n"; }
-
A timestamp is just a time. Usually it'll be the number of seconds since midnight 1 Jan 1970. Probably they expect you to use it to see when the other users were last active. A user not active for more than 10 minutes or so should probably be considered "logged out". Regarding those cookies, users can forge their user id and logged in status in just by altering their cookies. Sessions are better.
-
That sounds more suited to javascript (php would still be involved on submission of the data).
-
"Connection refused in /usr/www/www.example.com/board/deletepost.php on line 46" This is talking about an error in deletepost.php, not in your scheduler. Also, it's very strange to use include() to call a script using a URL. include() is usually for local files. CURL or file_get_contents() would make more sense.
-
Can you show us your form as well? Or an extract of it at least (2 questions minimum, and the opening form tag).
-
Try this out. You can do slightly better using "exists", but I don't think it's worth the effort. $query="SELECT * FROM UserName WHERE UserName = '$UserName'"; $result=mysql_query($query) or die("Query failed: $query\nError: " . mysql_error()); $num_rows = mysql_num_rows($result); if ($num_rows == 1) { # dup } else { # not dup }
-
If the query is fine apart from not returning cars which are not in the reservations table, you can use a left join SELECT * FROM vehicle LEFT JOIN registration ON vehicle.vehicleID=registration.vehicleID WHERE makemodelID=3 AND branchID=1 AND ( NOT (collect_date <= 20070409 AND return_date > 20070409) OR (reserID IS NULL) ); reserID will be null for those rows where there is no data from the reservations (registration?) table.
-
Try if ($_POST['submit'] === 'submit') { And ensure your form has <input type="submit" name="submit" value="submit"> Name: Name of the submit button in php ($_POST['submit']) Value: What to display on the submit button. Also the *value* of $_POST['submit'] Type: This says it's a submit button, and not something else Note that if you use javascript's submit(), you may have trouble with naming your submit button "submit" as well.
-
cron does not accept cookies and sessions. It just runs programs at a specific time, that's it. It's very simple and dumb. The simple solution is to have cron call a wrapper php script, and have that php script call the real script (the one on your url) with whatever arguments you want to pass along to it. Then you have the full flexibility of php available to you.
-
No, there's no need to make anything a child class of anything. Static method calls can be made from anywhere to any object, and will work fine as long as the method doesn't reference $this. That's demonstrated by your example code. The code you just posted doesn't seem to match your example though. In the code you posted $Users::updateUser($attackerData['userID'], "updatedata=here"); $Users is an instance of the Users object. But :: is for making direct calls to a class, without a particular instance. Using code like this gives me an error here with php 5.1.4 Class2::class2function(); This says to call class2function() from Class2, with no instance. This is fine as long as class2function() does not mention $this $c = new Class2(); $c->class2function(); This says to call class2function() from the $c instance of Class2. Since there is an object instance given, it's fine for class2function() to reference $this (and you would expect it to in this case).
-
The structure you're proposing is the right one (or at least the right starting point). Definitely ditch the columns, that will drive you crazy later Can characters really hold 1000 unique items each? At one time? That's a lot of pockets You might notice that most games impose a limit on the number of items that can be held, either weight or size based, or a fixed number. Part of the reason for that is efficiency. Access can still be quite fast. How often do you need to list EVERY item a character has? If you need to test for a given item, then an index on the character id and item column will give you instant access to that knowledge. The same index can be used partially (on the character id column only) to fetch all items belonging to a character. If you're really worried, setup the table and setup 10k dummy characters, each who hold 1 of each of 1000 items. Then do a few queries and see what happens. I imagine you'll have no trouble unless your players are compulsively checking their inventory every few seconds, AND they all happen to have their pockets stuffed full with 1000 unique items.
-
http://dev.mysql.com/doc/refman/5.0/en/blob.html
-
With that array structure, you should only use one level to access it: print "Element 0 is " . $guildRanks[0] . "\n"; If you use two levels, then you will not get what you want print "This is nonsense: " . $guildRanks[0][0] . "\n"; Probably you just need to replace $guildRanks[$char['Rank']]['Title'] with $guildRanks[$char['Rank']]
-
Did you forget to include your form tags and your submit button when you posted, or are they missing altogether? The other thing you should do is check mysql_query() for errors. $results = mysql_query($sqlquery) or die("Query failed: $sqlquery\n" . mysql_error());
-
Please post your code.
-
Yes, the code posted runs perfectly. We need the actual code if we are going to debug it.
-
[SOLVED] Website worked with php4, now we use php5.
btherl replied to matthewst's topic in MySQL Help
It looks like your scripts expect register_globals to be set to "on" (in your php.ini). You can fix it by either changing all the input variables (those that come from a form) to like this: if($_REQUEST['submit'] || $_REQUEST['FrontPage']) { Or you can just switch register_globals on. If you're not too familiar with PHP, switching on register_globals is the easiest solution. -
A lot of it is down to experience. You'll learn over time where objects are appropriate and where they aren't. Too much abstraction and you'll have code that spends more time dealing with the OOP framework than actually doing the task it's supposed to. Too little and your code will be difficult to debug, maintain and re-use. A situation where OOP works well is Smarty templates. Another is the SimpleXML object in php 5.
-
Oh.. I see. It's not the php tags that are your problem. The heredoc syntax is not escaping php variables, that is what is causing your problem. Try this: <?php $htmlforfile = <<<HEREDOC <?php session_start(); if (\$_SESSION['translation'] != "jamba-translation") { die("not allowed"); } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"><html xmlns="http://www.w3.org/1999/xhtml"><head><meta http-equiv="Content-Type" content="text/html; charset=utf-8" /><title>Untitled Document</title></head><body>\$text</body></html>' HEREDOC; print $htmlforfile; ?> The difference is that I have escaped the "$" signs.
-
Using the % will kill efficiency though, if your table is large. Particularly if you have it at the start. If you exploded with \n, it's possible you have a dos format file, and so you have a hidden \r in every string in your database. You can try using some of the string length and substring functions in mysql to investigate further.