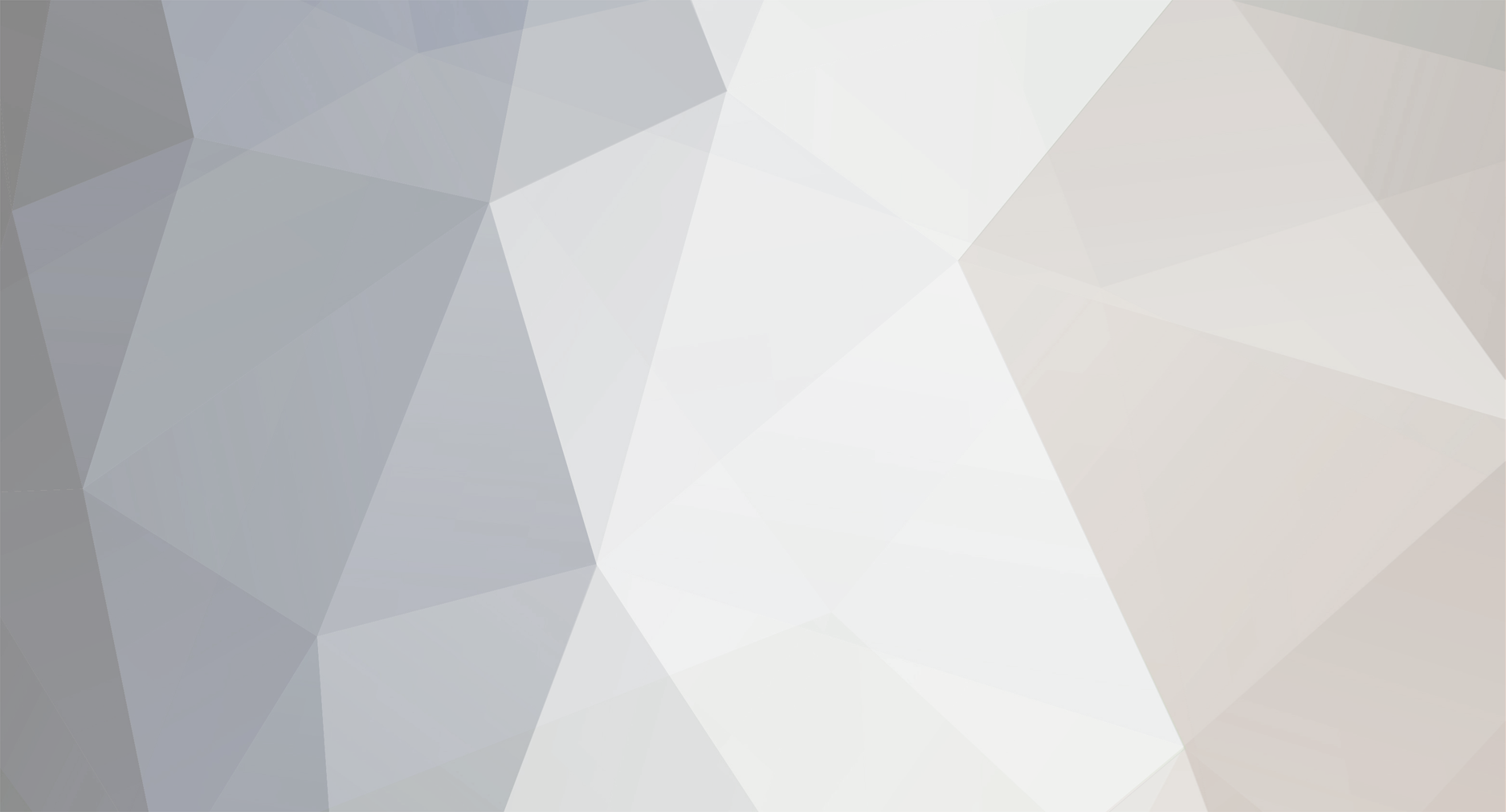
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
Heredoc syntax will escape everything.. like so <?php $var = <<<HEREDOC <?php blah ?> HEREDOC; print $var; ?>
-
Please post your actual code, not just an "example". Your example works fine.
-
It's not really something you would want to do normally. If you want to combine related data together then you can use joins. And if you want to combine similar result sets into one result set you would use union. But combining several unrelated (in the sense of how they are calculated) data items into one result set is just not "logical" in SQL. You an look at an SQL query this way. It starts with an entire table or tables: ------- ------- ------- Then it uses the "where" condition to pick certain elements from that - - - - Then it either returns those items as they are (as many rows), or aggregates them, such as taking a sum or count. When you have different "where" conditions in one query, there's no natural way for SQL to deal with that. Usually it chooses items based on the "where", and then operates on them. But if you have different conditions for each of your different outputs, then which conditions does it use? Or does it use each condition in turn, starting from the full set of data each time (which is basically the same as doing individual queries) ? So you might as well use several queries, each with its own "where" condition.
-
It depends what you mean by "less desirable" A single query is probably more efficient. Multiple queries are more simple. When given a choice between efficiency and simplicity, I almost always go for simplicity. Simple code means fewer bugs, and if you're dealing with peoples' investments, less bugs is VERY important. Much more important than having your code run a little bit faster. The way the code is written in your latest post is how I would do it. Every query is accompanied by a description of what it does, and every query is simple to understand. That to me is very good code. There is one situation that I would change it to a single query, and that is if the system runs too slowly AND it is not feasible to purchase better hardware. It also depends on the cost of your time vs the cost of better hardware. If your time is cheap, then by all means spend more time optimizing the code and more time maintaining the optimized query. But if your time is valuable, you may find it cheaper to buy a faster machine and leave the code as it is.
-
You need to include() or require() your class definitions inside your main file. After that, the code works perfectly. For example: require_once('class1.php'); require_once('class2.php');
-
Can I ask the obvious question.. why are you merging your queries instead of using seperate queries? The difficulty in merging the queries you've given are that each one has different "where" conditions. And they have outputs of a different nature as well. Given those two facts, I don't see why you would want to merge them. The other argument against merging the queries is that you would replace several simple queries with one complex one. A complex query is much more difficult to debug.
-
No, you don't have to provide source code for your application if you use an LGPL library. You might have to provide source for the library though (or at least make the source available for download). I wouldn't try reading the license itself unless you're really keen. They tried to make it comprehensible, but it's still a legal document.
-
Since both queries are on the same table, you should be able to use this: SELECT SUM(deposits) AS total_deposits, SUM(withdrawals) AS total_withdrawals FROM table1 There's no need for unions or subqueries. Union is for a different situation - it's for when you have some rows from table 1 and some more rows from table2 which look the same, and you want to merge them into one huge blob of rows.
-
I wouldn't recommend that.. instead use: $user_result = @mysql_query("SELECT username FROM members WHERE uid=$uid") or die(mysql_error()); $user = mysql_result($user_result, 0); The reason is that Wildbug's code will not fail on errors. Instead it will give you an incorrect $user as the result. As for the join, it'll look like this: $sql = "SELECT * FROM movies JOIN members ON (movies.uid = members.uid) WHERE mid=$mvid"; $randmovie=@mysql_query($sql) or die(mysql_error()); then username will be available without any additional queries.
-
Actually it should be mysql_affected_rows(), not mysql_num_rows() See the description here: http://sg2.php.net/manual/en/function.mysql-num-rows.php
-
I would convert it all to strings, like "ff", "ff/New Folder". Then you can convert back when you receive that data back from the form. Is this what you are trying to do?
-
how to transfer my mysql database from one computer to another
btherl replied to ani_anirban's topic in MySQL Help
The safest way is to dump the database and then re-import it on the new machine. I can't help you with the details unfortunately. The only time you could get away with copying over the data files is if you have the exact same configuration on both machines (for mysql), and when you don't mind overwriting anything already in mysql on the target machine. -
Does your query return any rows?
-
Instead of $update4 = mysql_query("UPDATE users SET nickname = '$_POST[nickname]' WHERE username = '$_COOKIE[username]'"); use $sql = "UPDATE users SET nickname = '$_POST[nickname]' WHERE username = '$_COOKIE[username]'"; $update4 = mysql_query($sql) or die("Mysql error in $sql\nError: " . mysql_error()); Make that change to each mysql_query() call. Otherwise you will not be notified of errors.
-
I have had success with this one (from the html_entity_decode() manual page comments) for decoding greek characters: <? function utf8_replaceEntity($result){ $value = (int)$result[1]; $string = ''; $len = round(pow($value,1/8)); for($i=$len;$i>0;$i--){ $part = ($value & (255>>2)) | pow(2,7); if ( $i == 1 ) $part |= 255<<(8-$len); $string = chr($part) . $string; $value >>= 6; } return $string; } function utf8_html_entity_decode($string){ return preg_replace_callback( '/&#([0-9]+);/u', 'utf8_replaceEntity', $string ); } $string = '’‘ – “ ”' .' ć ń ř' ; $string = utf8_html_entity_decode($string,null,'UTF-8'); header('Content-Type: text/html; charset=UTF-8'); echo '<li>'.$string; ?>
-
Here's a few ideas to try: 1. Temporarily change the name of the function. Do you get any errors coming up where it was being called from before? 2. Try changing the output of the function. Does the output of your script change? If no, then your function isn't being called.. it's another function which looks the same.
-
Is $redirect set correctly, and correctly encoded? Try printing out its value, and using "view source" to see what it really looks like. You may need to use urldecode() on it.
-
It's quite difficult to know when to use an index for a "greater than" condition. If a lot of values match the condition, then using an index will actually make it slower. If you know there will only be a small number of matching rows, you might want to try FORCE INDEX. On the other hand, if there actually are a small number of rows in that table at all times, then using an index may not help. Have you profiled your program to see which queries are taking up the time? It's good to be sure that that query is one of the problem queries before trying to optimize it. A good approach is to use a wrapper for your database queries which times every single one, and logs the query along with the time taken to a log file.
-
I hope timed_query() does error checking. If you're finding that query is slow, you probably need an index on info.
-
Table names shouldn't be inside ' , instead they should be inside ` , or inside no quotes at all.
-
Try printing out the values of the variables you are using, to verify that they are what you expect them to be.
-
From the page you referenced in your original post: "When asked to store a value in a numeric column that is outside the data type's allowable range, MySQL's behavior depends on the SQL mode in effect at the time. For example, if no restrictive modes are enabled, MySQL clips the value to the appropriate endpoint of the range and stores the resulting value instead. However, if the mode is set to TRADITIONAL, MySQL rejects a value that is out of range with an error, and the insert fails, in accordance with the SQL standard."
-
Seems no-one has answered the OP's question.. The answer is "no". You should not get an integer, even if the column is an integer type. You should get a string, or the null type if the value in the column is null. End of story http://sg.php.net/manual/en/function.mysqli-fetch-array.php "Returns an array of strings that corresponds to the fetched row or NULL if there are no more rows in resultset."
-
$new_arr = array(); foreach ($orig as $o) { $new_arr[$o['filedID']] = $o['description']; } That ought to work..
-
Both the sql AND the php please That sort of strange behaviour makes sense when dealing with cowboy code. Now we just need to turn it into professional programmer code