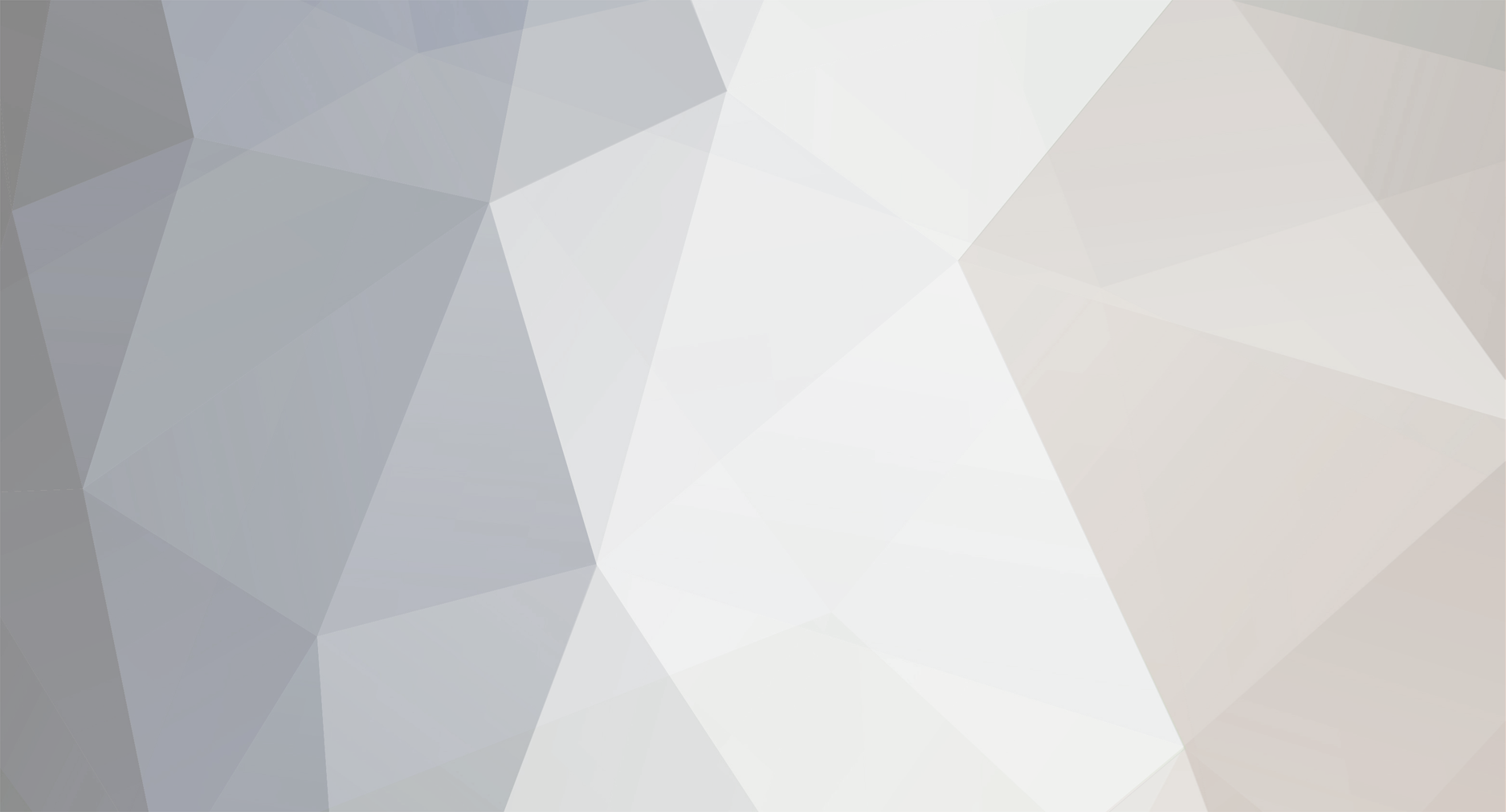
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
How do I turn a variable into an array?
btherl replied to horseatingweeds's topic in PHP Coding Help
If you want state code => name, try this: $state_arr = explode("|\n", $state); # if "|\n" is the delimineter $lookup_tab = array(); foreach ($state_arr as $s) { list($country, $code, $name) = explode(':', $s); $lookup_tab[$code] = $name; } print_r($lookup_tab); -
The cost of pulling an entire row compared to pulling one column from the database is not much different. Not unless there is a LOT of data in that row. You may save some time in parsing the data though. I would expect you will see little difference though. But I could be wrong It's always difficult to predict performance. Another option is to store the data in a session, and let the script itself refresh the data only if the script itself makes a change to it. That means that if some other script modifies the user's data, the change won't show up. But you do save the trouble of checking to see if it's updated. Whether or not that works depends on your situation.
-
The default session handler locks the session file, so only one script can use that session at one time. You can solve this by writing your own session handler (there is a simple example in php's online documentation: http://sg2.php.net/manual/en/function.session-set-save-handler.php )
-
Newbe with simple questions long time programmer not on php though
btherl replied to zapdbf's topic in PHP Coding Help
Hi, Yes it is. The best online reference manual for php is here: http://www.php.net/manual/en/ -
Your form is using get, so you should check for $_GET['submit']
-
Short answer is no, it's not easy Here is my code: <?php function get_page_numbers($query_count, $rows_per_page, &$page_num, &$num_pages, &$first_link, &$prev_link, &$next_link, &$last_link, &$prevnext) { # Page number related calculations and query addition # $query_count is the number of items to be paged through $num_pages = (($query_count - ($query_count % $rows_per_page)) / $rows_per_page); if ($query_count % $rows_per_page != 0) $num_pages++; if (!is_numeric($page_num)) $page_num = 0; if ($page_num > $num_pages - 1) { $page_num = 0; } # Information for displaying page 1 2 3 4 ... links if ($num_pages > 10) { if ($page_num >= 5) { $prev_link = $page_num - 5; $first_link = 0; for ($i = 0; $i < 9 && $page_num + $i - 4 < $num_pages; $i++) { $prevnext[$i] = $page_num + $i - 4; } } else { $prev_link = -1; $first_link = -1; } if (($num_pages - $page_num) >= 5) { $next_link = $page_num + 5; $last_link = $num_pages - 1; if ($page_num < 5) { for ($i = 0; $i < 9; $i++) { $prevnext[$i] = $i; } } # If $page_num >= 5, then list was populated earlier } else { $next_link = -1; $last_link = -1; } } if ($num_pages <= 10) { $prev_link = $next_link = -1; $first_link = $last_link = -1; for ($i = 0; $i < $num_pages; $i++) { $prevnext[$i] = $i; } } } ?>
-
Your logic is fine. And in my mind, it translates directly into code. So I am not sure what it is that you need. How about going with a top down approach? You can start with high level functions like this: landing_request($airport, $plane, $time) { Check if $airport is free for landing at $time If free, add $plane to timetable and return "granted" Otherwise, return "not granted" } Then you can start thinking about the implementation of the timetable, the airport, the plane. Knowing how you will use a structure is very helpful when you start designing that structure. For example, the timetable will probably be searched frequently by time, so it should be a structure that can be efficiently searched by time.
-
Help! someone. Big struggle with multi dimentional arrays
btherl replied to gabrielkolbe's topic in PHP Coding Help
Nakor's code looks like it'll work. If you want to add the access keys into the array itself, you can do: foreach ($arr as $key => $value) { $arr[$key]['accesskey'] = $arr[$key]['title']{0}; } The {0} takes the first character of a string. -
That kind of behaviour indicates to me that it's a problem with your web host. Have you tried asking them about it? You can write a message that says "I am having this trouble, do you know what could be causing it?". That way they won't feel like you are blaming them for the problem, and they will feel more like helping you. Make sure you include instructions for replicating the problem.
-
If it's a shared hosting account and you do not have root access, then you cannot chown or lchown. It doesn't matter where the file is or who it belongs to. It just can't be done. If you explain why you need to chown, then perhaps we can suggest an alternative. Probably you need chmod instead. chmod can be used to give other users permission to use files that belong to you.
-
Where is your site hosted?
-
It's fine, except that you should be using $key instead of $title in your first echo.
-
Problem with cookies. Need to encompass all domains
btherl replied to morphboy23's topic in PHP Coding Help
It can also be done in apache using rewrite rules.. but I'm not clear on the details of doing it that way. -
Problem with cookies. Need to encompass all domains
btherl replied to morphboy23's topic in PHP Coding Help
You can do this at the top of every script (this can be included in a global configuration file) if ($_SERVER['HTTP_HOST'] === 'bloowoo.com') { $new_url = str_replace('bloowoo.com', 'www.bloowoo.com', $_SERVER['SCRIPT_URI']); header('Location: ' . $new_url); exit(0); } Hopefully that'll work. Different servers sometimes set different variables in $_SERVER, so you might need to adjust it. -
Yes they'll queue up. That may cause big problems if you have too many people trying to write to the file! So you should keep an eye on it at peak times to make sure the queue of waiting scripts is emptying fast enough.
-
Yes, the problem is well known. There are a few solutions. 1. http://sg.php.net/manual/en/function.flock.php 2. Notice this in the notes for fwrite(): If you're writing to an nfs mounted filesystem though, you may have trouble. To answer your second question, no, any number of scripts can open the same file unless you lock it.
-
Problem with cookies. Need to encompass all domains
btherl replied to morphboy23's topic in PHP Coding Help
This doesn't answer your question, but it may solve your problem. One option is to redirect all requests that hit bloowoo.com to hit www.bloowoo.com instead. Then you don't need to worry about supporting two possible hostnames anywhere in your code. -
Ok, that's better. First, are you really trying to lchown a symbolic link? There's usually very little reason to do that. Normally you would use chown() on a standard file. Secondly, do you have permission to chown files? Usually that will require root (superuser) access. This also comes back to the question of why you are using lchown(), because it is a very unusual function to use. If you explain what you are trying to do, then perhaps we can suggest an alternative.
-
Why do you want to lchown a link that is outside your website's directory? Your website is in /home/ppl, but you are trying to modify files in /var/www
-
Is there a function to search a string within another string ?
btherl replied to jd2007's topic in PHP Coding Help
There are many functions strstr() strpos() stripos() (php5) stristr() Edit: And you can look them up in the online documentation at http://www.php.net -
The simple solution is to switch off open_basedir. But it depends on your situation, which you haven't described to us.
-
[SOLVED] session_start() rejects my first login attempt?
btherl replied to sayedsohail's topic in PHP Coding Help
Sayed, I tested your script here and it works on the first try. The only oddity is I have to click "unreg" twice to unregister the session. This is because the username and password are unregistered AFTER being tested. Just to confirm, do you remove the "m=1" before testing the script each time. -
Can you post your code?
-
If you're talking about the single query, you can do this: 1. Order the query by dl_id 2. In the loop that fetches the data, remember the last dl_id and check if the new one is equal. If it's equal, then this is another image from the same dl_id. If it's not equal, then it's a new dl_id. Then take appropriate action.
-
Please post your complete code.