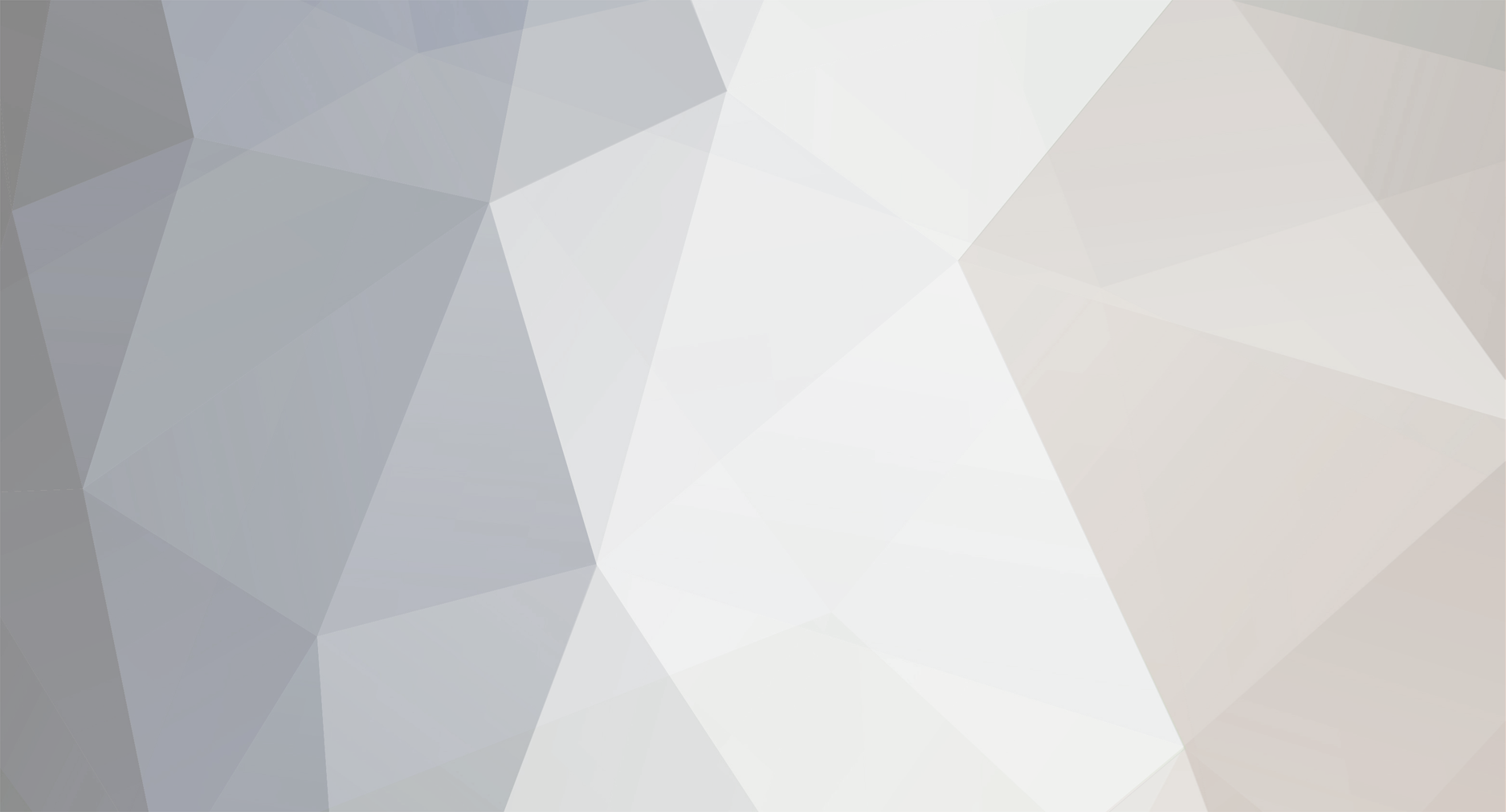
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
Did you try googling "send mail with smtp with username and password in php" ? In case you get different results to me, here's my first result: http://email.about.com/od/emailprogrammingtips/qt/et073006.htm
-
For duplicates SELECT email FROM table1 JOIN table2 USING (email) For emails in table1 but not in table2 SELECT email FROM table1 LEFT JOIN table2 USING (email) WHERE table2.id IS NULL You can replace "id" with any column from table2. You can also use this syntax which is more flexible: SELECT email FROM table1 LEFT JOIN table2 ON (table1.email = table2.email) WHERE table2.email IS NULL The reason it works is that a left join will place nulls in the columns of the right table where no matching row is found, so you just check for these nulls. If table2 really CAN have nulls in that column, then of course this will not work. Choose a column that can't be null.
-
Even if you change it, how can you force every user to logout? A shopping cart is one of the places users are least likely to logout when their session is finished. They will just browse somewhere else. What do you mean by "There is no cookie"? I didn't mention cookies.
-
You can add an additional table, indexed by user id. Eg. CREATE TABLE logged_in ( user_id integer PRIMARY KEY, last_logged_in DATETIME, ); Then, you can check the time they were last logged in from there, and make a choice about whether or not to allow a new login from a new location. The hardest part to deal with is that people usually don't logout when moving from location to location, so you need to be able to time out sessions after a while. That's why you need to store the time of the last login, and not just whether or not the user is logged in. Or you can store the data in a text file, if you prefer that. As long as the data is stored on the server, it really doesn't matter where
-
Only displaying one row from Table... should be more
btherl replied to techiefreak05's topic in PHP Coding Help
Can you add this line? print "There were " . mysql_num_rows($query1) . " offers made<br>"; immediately after the first mysql_query() ? -
That information is best stored in mysql. You could add it to the user table, for example. You will need a method to detect situations such as user1 being logged in from home and forgetting to logout, then logging in from work (if such a situation will happen for your system).
-
Only displaying one row from Table... should be more
btherl replied to techiefreak05's topic in PHP Coding Help
Try this (I moved the "}" down to the bottom, no other changes): <?php $query1 = mysql_query("SELECT * FROM trade_offers WHERE trade_id = $_GET[tradeid]") or die(mysql_error()); while ($array1 = mysql_fetch_array($query1)){ $u__id = $array1[user_id]; $query3 = mysql_query("SELECT * FROM members2 WHERE id = $u__id") or die(mysql_error()); while ($array3 = mysql_fetch_array($query3)){ $offerer_Name = $array3[username]; } $query2 = mysql_query("SELECT * FROM trade_offers WHERE trade_id = $_GET[tradeid] AND user_id = $u__id") or die(mysql_error()); while ($array2 = mysql_fetch_array($query2)){ $item__id = $array2[item_id]; } ?> <h4>Offer made By: <?php echo $offerer_Name; ?></h4> <img src=<?php echo $base_url; ?>/images/user_images/opg_1/items/item_<?php echo $item__id;?>.gif> <?php } ?> -
[SOLVED] email only half works now (i didn't change anything..really)
btherl replied to matthewst's topic in PHP Coding Help
Try tracking the value back through your code by printing it out. First, print it out immediately before the spot where it has the wrong value. If it's still wrong there, print it out a bit further back. Eventually you will find the exact location at which the value changes from being correct to incorrect (it may even have never been correct from the start). That's where the bug is. -
Try putting this code at the very top, and see what it shows: print "<pre>"; print "_REQUEST contains:\n"; var_dump($_REQUEST); print "_POST contains:\n"; var_dump($_REQUEST); print "_GET contains:\n"; var_dump($_GET); exit(0);
-
Script causing another script not to display info
btherl replied to thefreebielife's topic in PHP Coding Help
If you want to go back over a set of rows from mysql, you must use this function: http://sg2.php.net/manual/en/function.mysql-data-seek.php eg msql_data_seek($result, 0); -
That tutorial uses the php4 interface: http://sg2.php.net/manual/en/ref.domxml.php Since you're using php5, you've got the new interface: http://sg2.php.net/manual/en/ref.dom.php
-
Which version of php are you using? What does "echo phpversion();" show?
-
[SOLVED] not getting the right info, stuck on one row
btherl replied to HAVOCWIZARD's topic in PHP Coding Help
The simplest way is like this: while ($row = mysql_fetch_array($data)) { print "<pre>"; var_dump($row); print "</pre>"; } Try placing that before your "$result = mysql_fetch_Array($data)" line. The key is that you must "fetch_array" for EACH row of data. A single call will not fetch all your results. Once you understand how that works, you can replace the inside of that loop with your html display code. The values you will be referencing will look like: echo $row['register_companyName']; -
Please post your error message
-
The easiest way (that I can think of) to achieve this is to have an extra column in your table that records whether or not the row has been added to the file. Then you can just do a query with "WHERE added = false ORDER BY id". I'm not sure how much detail you want, so ask again if you need more.
-
Aha, then fsockopen on its own will not be helpful. Opening a udp connection does absolutely nothing. The only way to ping a udp port is to send something and check the response you get back. So you need to capture a packet from the game protocol and send that over the udp port, and see if you get a response. Or, you can check port 80 like smc suggested, and just assume that if that responds, then the game server will be running That's less reliable but considerably easier. Another option is to use true "ping", which uses ICMP rather than TCP or UDP. That has the same drawbacks as checking port 80, which is that you don't really know if the game server is running or not. It probably has a higher risk of being firewalled than port 80.
-
Is the service you are pinging running on tcp or udp? Or both?
-
You can store the data in an array in php and sort it before displaying. As for doing it in mysql, you can do this (untested, also I had to guess your column name for G.group_id): SELECT name, id, count(*) AS member_count FROM groups G JOIN members M ON (G.group_id = M.group_id) GROUP BY name, id ORDER BY count(*) DESC
-
Hmm.. a proxy is possible. You need one that can be connected to on port 80, but can send data out to any other port number. An SSL proxy might be able to do that (using the CONNECT method). I'm not sure about HTTP proxies, because I assume port 9339 is not a webserver. You may be able to distinguish connection and non-connection from how the proxy acts though.. I wouldn't go for packet based methods because 1. They are complex. 2. They require superuser access, which you may not have. 3. The probably won't work, unless the firewall is badly configured.
-
You can test it by running the code you posted.. if it doesn't work, you're firewalled If you are running the script from a web hosting service, then I would guess that's your problem. If it works for port 80 but not for any non-standard port, then I would be 99% sure that's your problem. If you're running the script from home, then it would depend on your ISP .. usually ISPs don't block such traffic, otherwise they would interfere with online gaming. But webhosts are different.
-
I tested your code here and it works fine on non-standard ports (22 and 5433 I tested). Are you running it from a web host which may firewall outgoing traffic to non-standard ports?
-
[SOLVED] mysql query error not making sense
btherl replied to cooldude832's topic in PHP Coding Help
Try printing out your query and looking. You have your values inside both single AND double quotes there, which certainly won't help. But the error reported appears to be earlier, near "Show". Try putting "Show" inside backquotes like this: `Show` The other thing I would change is this: $values = "'$querynumber', ... "; Then instead of calling the query directly, put it in a variable first and print it out so you can inspect it. -
Can you be more specific about which ports, and which services you are testing for?
-
Do you want to fetch xxxxx items for each category returned from the gettype query?
-
I don't see a call to mysql_connect(). Is that your entire script?