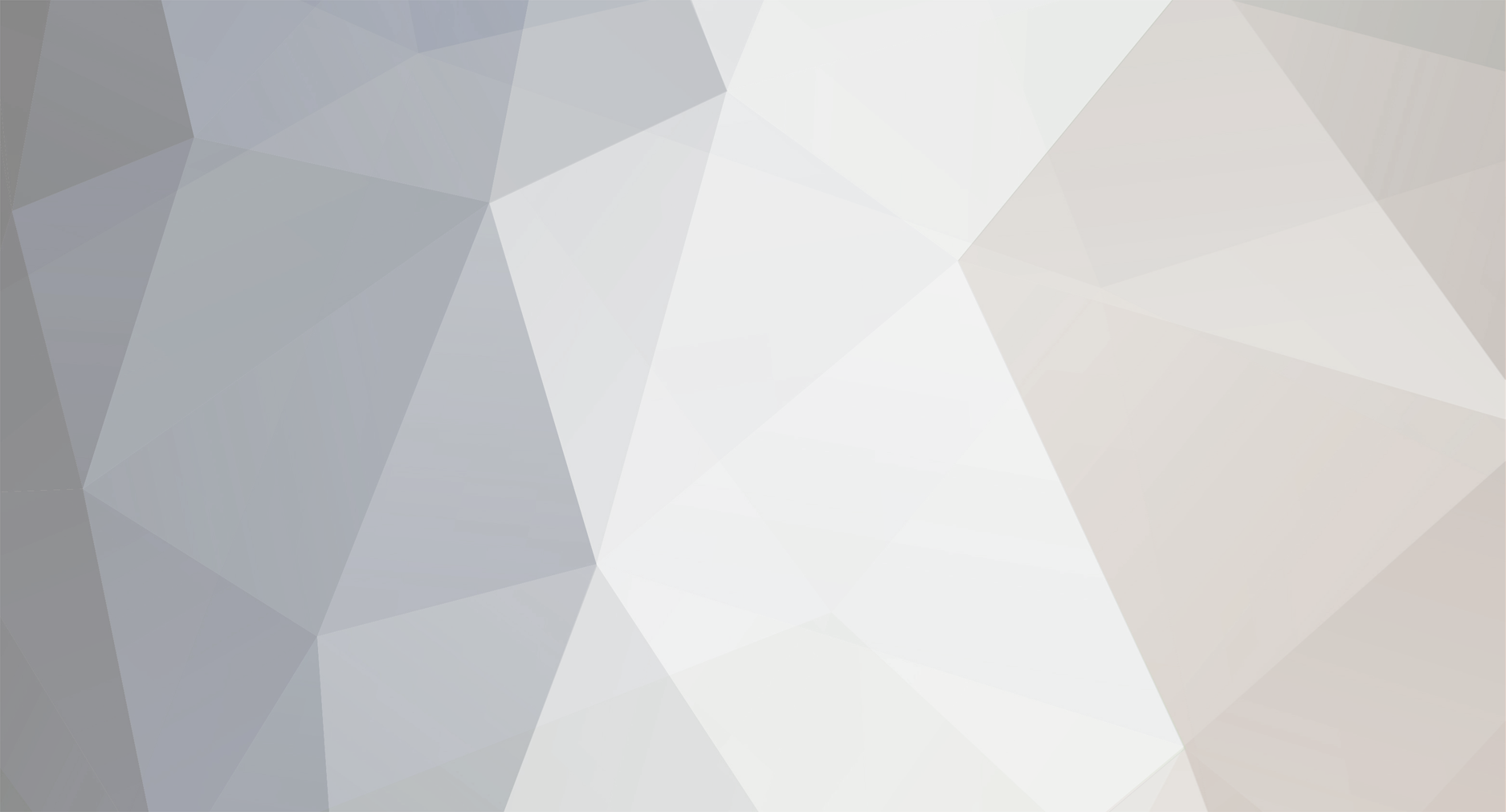
rab
Members-
Posts
155 -
Joined
-
Last visited
Never
Everything posted by rab
-
<?php include("dbc.php"); $file="data.txt"; $data=file_get_contents($file); $data=explode("\n", $data); foreach($data as $qstring) { $qstring = explode("|", $qstring); foreach($qstring as $k => $v) $qstring[$k] = "'$v'"; $qstring = implode(",", $qstring); $query="INSERT INTO `stauctioneer_users` (`biddernum`, `lname`, `fname`, `address`, `city`, `state`, `zip`) VALUES ($qstring)"; $do=mysql_query($query); if( !$do ) mysql_error(); echo $query; echo "<br>"; } ?> Try it out.
-
Try $_SESSION instead of $_session.
-
Try using <?php instead of <?. Some hosts have short_tag off. Also the mysql functions should be all lowercase.
-
...Why don't you try it... and fyi, foreach loops arrays.
-
<?php foreach($xml['FSHOST']['0']['FLIGHTPLANS']['0']['FLIGHTPLAN'] as $user) { print $user['PLAYERNAME'][0]['VALUE']."\n"; } ?> Try that.
-
Well, your accessing the PHP script as a file on the system. Try http://localhost/flowertree/index.php
-
<?php $file = file_get_contents("maillist"); $emails = explode("\n",$file); print_r($emails); ?>
-
I think it depends on the programmers style of coding.
-
You need to connect to the mysql host and select a database. <?php $conn = @mysql_connect("localhost", "root", "neveruseasroot"); if( !$conn ) die("Error connecting to MySQL host"); $db = @mysql_select_db("database",$conn); if( !$db ) die("Error selecting a database"); //... continue with your code ?>
-
<?php session_start(); $loggedIn = False; // Either not logged in or session expired if( empty($_SESSION['user']) ) { if( isset($_COOKIE['user']) ) { $data = unserialize($_COOKIE['user']); // Assumes loginUser escapes variables... if( loginUser($data['user'],$data['pass']) ) { $_SESSION['user'] = $data; // Now logged in $loggedIn = True; } } } else $loggedIn = True; ?> When they actually do login, assign $_COOKIE['user'] serialized data of the $_SESSOION['user']. Users can modify cookies, but with the login check you can see if the cookie is valid or not.
-
<?php if( !isset($_GET['page']) ) $_GET['page'] = 'home'; switch($_GET['page']) { case 'home': include "index.php"; break; case 'gallery': include ("gallery.php"); break; case 'animation': include ("animation.php"); break; case 'contact': include ("contact.php"); break; default: include "content.php"; break; } ?> Your syntax was incorrect. if(!isset($_GET['page'])) else $page='home'; PHP was expecting a code between the if/else statements
-
SELECT * FROM playlist WHERE column=1
-
Check your php.ini settings to see if it allows url_fopen. If not, enable it.
-
[SOLVED] Controlling amount of results outputted by an array
rab replied to Michan's topic in PHP Coding Help
<?php $offset = (int)$_GET['page']; $size = 10; $topics = array_slice($topiclist, $offset, $size); foreach($topics as $key => $val) echo "$val<hr>"; ?> Try something like that. -
Its a multi-dimensional array, so $earned[1] is just an array. $earned[1][0] should have your achievements. Please read the docs at php.net
-
If it works, then sure. It might not be the best way, but the way you altered the function isn't the best way either. You should modify the function to return what you want then go on with the script there. <?php $stats = fetchHaloStats($_GET['gamertag']); if( $stats === False ) { if( empty($_GET['gamertag']) ) { // need a tag } else { // Invalid tag } } // print out the stats now. ?> In my opinion, it's not good to set a function to do error handling, depends on the error handling, and exiting the program. That should all be done outside the funciton.
-
I'm matching 2 things, (ServiceTag|Skill|Rank|TotalRP) and (.*?), which fills $match. The first match isn't needed, so i _should_ make it (?:...) so it doesn't back reference that text. <?php function fetchHaloStats($gamertag, $specific='') { if(empty($gamertag)) return False; $stats = file_get_contents("http://www.bungie.net/Stats/Halo3/Default.aspx?player=".urlencode($gamertag)); if(preg_match('/Halo 3 Service Record Not Found/i', $stats)) return False; preg_match_all('/<span id="ctl00_mainContent_identityStrip_lbl(?:ServiceTag|Skill|Rank|TotalRP)">(.*?)<\/span>/', $stats, $match); $stats = array('Tag' => $match[1][0], 'Rank' => $match[1][1], 'Skill'=> $match[1][2], 'EXP' => $match[1][3]); return (!empty($specific) && array_key_exists($specific,$stats) ? $stats[$specific] : $stats); } var_dump(fetchHaloStats("xrab","Tag")); ?>
-
<?php function fetchHaloStats($gamertag, $specific='') { if(empty($gamertag)) return False; $stats = file_get_contents("http://www.bungie.net/Stats/Halo3/Default.aspx?player=".urlencode($gamertag)); if(preg_match('/Halo 3 Service Record Not Found/i', $stats)) return False; preg_match_all('/<span id="ctl00_mainContent_identityStrip_lbl(ServiceTag|Skill|Rank|TotalRP)">(.*?)<\/span>/', $stats, $match); $stats = array('Tag' => $match[2][0], 'Rank' => $match[2][1], 'Skill'=> $match[2][2], 'EXP' => $match[2][3]); return (!empty($specific) && array_key_exists($specific,$stats) ? $stats[$specific] : $stats); } var_dump(fetchHaloStats("xrab","Tag")); ?> Slightly modified. The reason why your getting "Array" back is because preg_match fills the third argument, $match, with the matched text. If matches is provided, then it is filled with the results of search. $matches[0] will contain the text that matched the full pattern, $matches[1] will have the text that matched the first captured parenthesized subpattern, and so on. So $matches[1][0] should have the service tag.
-
<?php function fetchHaloStats($gamertag) { if(empty($gamertag)) return False; $stats = file_get_contents("http://www.bungie.net/Stats/Halo3/Default.aspx?player=".urlencode($gamertag)); if(preg_match('/Halo 3 Service Record Not Found/i', $stats)) return False; preg_match_all('/<span id="ctl00_mainContent_identityStrip_lbl(ServiceTag|Skill|Rank|TotalRP)">(.*?)<\/span>/', $stats, $match); return array('Tag' => $match[2][0], 'Rank' => $match[2][1], 'Skill'=> $match[2][2], 'EXP' => $match[2][3]); } var_dump(fetchHaloStats("xrab")); ?> In action... rab@death:~$ php halo3.php array(4) { ["Tag"]=> string(3) "R23" ["Rank"]=> string(16) "Captain, Grade 1" ["Skill"]=> string(2) "23" ["EXP"]=> string(3) "129" } There ya go, there is always room for expansion with this...
-
<?php $_REQUEST['contentID'] = (int)$_REQUEST['contentID'] < 0 ? 0 : (int)$_REQUEST['contentID']; if( $_REQUEST['contentID'] > 0 ) { $result = mysql_query("SELECT * FROM content WHERE contentID = '{$_REQUEST['contentID']}'"); if(!$result) { // ERROR } } else { // Need an ID } ?>
-
Filter that variable as an integer and quote it in the SQL command.
-
It appears as if Wes's account was hacked after he posted his hash. Anyways, MD5 is "uncrackable" but there have been advancements in generating the same MD5 sum with a different file. I don't think this applies to raw MD5 on strings though, correct me if I'm wrong.
-
You can use javascript to do this if you just want to display it.
-
Post the array before asort, then after to debug it. print_r($dates)."\n"; asort($dates); print_r($dates)."\n";
-
<?php session_start(); //... rest of your code