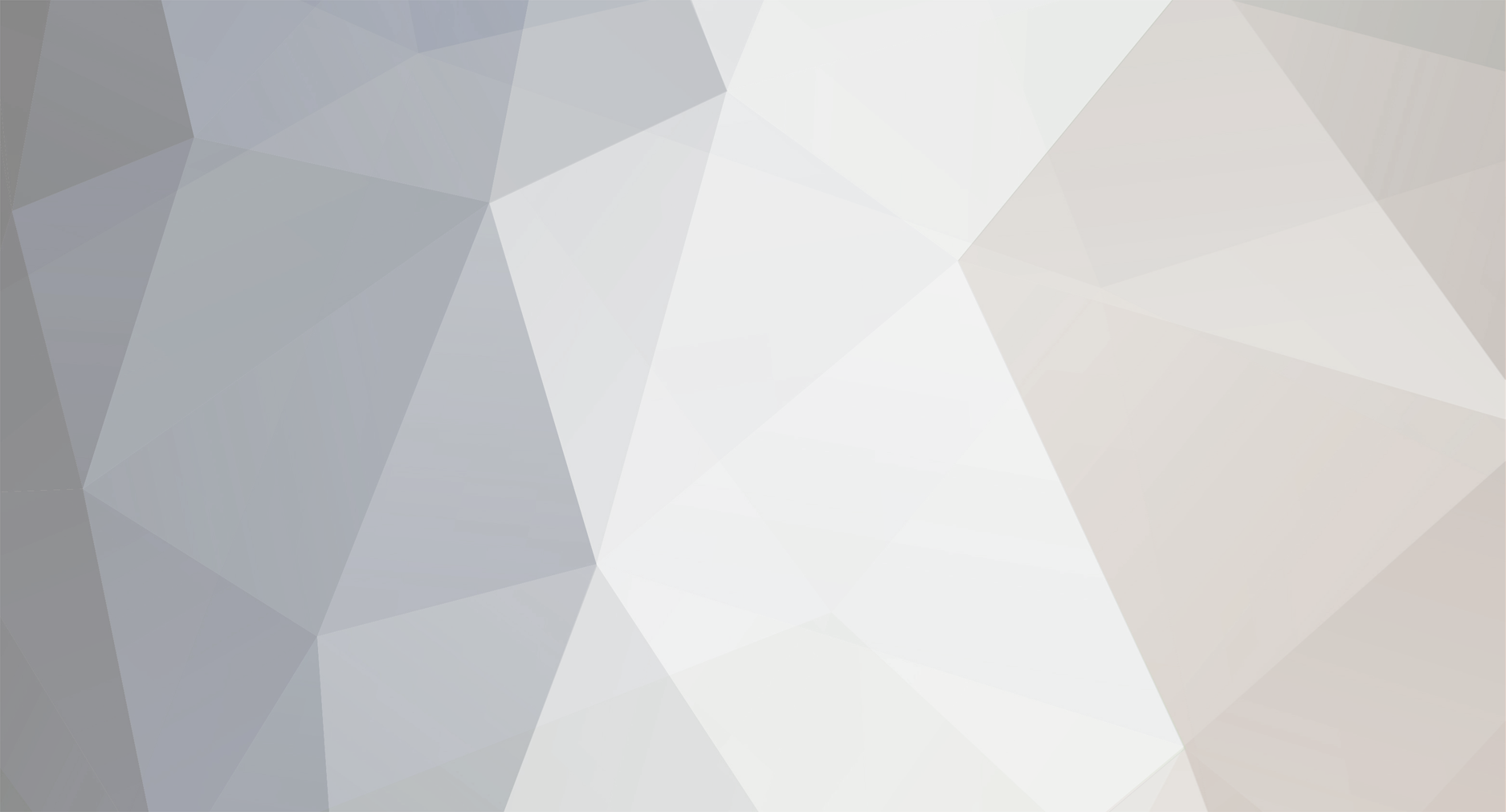
Corona4456
Members-
Posts
244 -
Joined
-
Last visited
Never
Everything posted by Corona4456
-
<?php class Connection { var $result; function Connection($localhost, $username, $password, $database){ $con = mysql_connect($localhost,$username,$password); mysql_select_db($database, $con); if($con) { $this->result = 'successful connection'; } else { $this->result = 'failed connection'; } } } // Add curly bracket here $test = &new Connection('localhost','***username(removed)***','***password(removed)***','cpt'); //line 18 echo $test->result; ?> You never close your brackets for your Connection class (see code for added curly bracket)
-
[SOLVED] getElementsByTagName('a')[0]; not working? please help.
Corona4456 replied to sayedsohail's topic in Javascript Help
No problem -
[SOLVED] count all records in a database
Corona4456 replied to british_government's topic in MySQL Help
no problem -
[SOLVED] using javascript & php togeather
Corona4456 replied to owen.aaron's topic in PHP Coding Help
It's an escape character. http://www.phpnoise.com/tutorials/25/3 -
[SOLVED] need assistance with displaying row of MySQL data with JS
Corona4456 replied to webguync's topic in PHP Coding Help
Ah I see the problem... I apologize ... since I don't have a way to test it, it's hard for me to see the error. <?php $connection = mysql_connect("localhost","username","password"); mysql_select_db ('dbname'); $sql = "SELECT f2,f3,phone,f8,f9,f10,f11,f12,f13,f15 FROM station ORDER BY f11 ASC" ; $sql_resulta = mysql_query($sql,$connection) or die('Query failed: ' . mysql_error()); //result set $rs = mysql_query($sql); $img= "<img src='icons/apo.gif' width='15' height='17' alt='airportindicator'>"; //creating the table w/ headers echo "<table id='display' cellpadding='0' cellspacing='0' align='center'><tr><td class='header'>Airport Indicator</td><td class='header'>State</td><td class='header'>Airport Code</td><td class='header'>Airport Name</td><td class='header' colspan='2'> </td></tr>"; //row for each record while ($row = mysql_fetch_array($rs)) { echo"<tr><td>$img</td><td> $row[6] </td><td> $row[0]</td><td> $row[1] </td><td id='header'><a href='#' onClick=\"FuncShowHide('$row[0]')\"> More Info.</a></td><td id='header'><a class='WHATSTHIS' onclick='selectLoc([0])' href='javascript:;'>Select this Location</a></td></tr>\n"; echo"<tr id='$row[0]' style='display:none'><td><strong>Address:</strong> $row[3] </td><td><strong>Address:</strong>$row[4] </td><td><strong>City:</strong> $row[5] </td><td> $row[6]</td><td>$row[7] </td><td><strong>Phone:</strong> $row[2] </td></tr>\n"; } echo "</table>"; //close the db mysql_close(); echo "</body>"; echo "</html>"; ?> -
[SOLVED] count all records in a database
Corona4456 replied to british_government's topic in MySQL Help
The only way I can think of doing it is this way: $sql = "SHOW TABLES" ; $result = mysql_query($sql,$connection) or die('Query failed: ' . mysql_error()); $total = 0; while($table = mysql_fetch_row($result)){ $sql = "SELECT COUNT(*) FROM `" . $table[0] . "`"; $result2 = mysql_query($sql, $connection); $row = mysql_fetch_row($result2); $total += $row[0]; } echo $total; -
[SOLVED] using javascript & php togeather
Corona4456 replied to owen.aaron's topic in PHP Coding Help
onmouseover="showInfo('Austins Acres','austinsacres')" onmouseout="return nd();" Should be: onmouseover=\"showInfo('Austins Acres','austinsacres')\" onmouseout=\"return nd();\" -
[SOLVED] need assistance with displaying row of MySQL data with JS
Corona4456 replied to webguync's topic in PHP Coding Help
Well I took a closer look at your code and it's pretty buggy so here take this code: Javascript: <script language="JavaScript" type="text/javascript"> function FuncShowHide(rowid){ row = document.getElementById(rowid); if(row.style.display == "none"){ row.style.display = "table-row"; } else { row.style.display = "none"; } } </script> <?php $connection = mysql_connect("localhost","username","password"); mysql_select_db ('dbname'); $sql = "SELECT f2,f3,phone,f8,f9,f10,f11,f12,f13,f15 FROM station ORDER BY f11 ASC" ; $sql_resulta = mysql_query($sql,$connection) or die('Query failed: ' . mysql_error()); //result set $rs = mysql_query($sql); $img= "<img src='icons/apo.gif' width='15' height='17' alt='airportindicator'>"; //creating the table w/ headers echo "<table id='display' cellpadding='0' cellspacing='0' align='center'><tr><td class='header'>Airport Indicator</td><td class='header'>State</td><td class='header'>Airport Code</td><td class='header'>Airport Name</td><td class='header' colspan='2'> </td></tr>"; //row for each record while ($row = mysql_fetch_array($rs)) { echo"<tr><td>$img</td><td> $row[6] </td><td> $row[0]</td><td> $row[1] </td><td id='header'><a href='#' onClick='FuncShowHide($row[0])'> More Info.</a></td><td id='header'><a class='WHATSTHIS' onclick='selectLoc([0])' href='javascript:;'>Select this Location</a></td></tr>\n"; echo"<tr id='$row[0]' style='display:none'><td><strong>Address:</strong> $row[3] </td><td><strong>Address:</strong>$row[4] </td><td><strong>City:</strong> $row[5] </td><td> $row[6]</td><td>$row[7] </td><td><strong>Phone:</strong> $row[2] </td></tr>\n"; } echo "</table>"; //close the db mysql_close(); echo "</body>"; echo "</html>"; ?> Also, I'd suggest limiting the amount of results and add pagination... the page will load much faster. so your $sql will be: <?php $sql = "SELECT f2,f3,phone,f8,f9,f10,f11,f12,f13,f15 FROM station ORDER BY f11 ASC LIMIT 0, 20" ; ?> This is for testing purposes only though... later you can add code for pagination. -
[SOLVED] need assistance with displaying row of MySQL data with JS
Corona4456 replied to webguync's topic in PHP Coding Help
<script language="javascript" type="text/javascript"> function FuncShowHide(rowid){ if (document.getElementById('hideShow').style.display == document.getElementById(rowid).style.display){ document.getElementById('hideShow').style.display = "none"; } else { document.getElementById('hideShow').style.display = document.getElementById(rowid).style.display } } </script> This is the code you need to have... copy and paste it exactly as written above -
[SOLVED] need assistance with displaying row of MySQL data with JS
Corona4456 replied to webguync's topic in PHP Coding Help
Err... no, the function definition is function FuncShowHide(rowid) - where rowid is the parameter you pass in. -
[SOLVED] need assistance with displaying row of MySQL data with JS
Corona4456 replied to webguync's topic in PHP Coding Help
oh I see what you are confused about ... here... let's change it up: <script language="javascript" type="text/javascript"> function FuncShowHide(rowid){ if (document.getElementById('hideShow').style.display == document.getElementById(rowid).style.display){ document.getElementById('hideShow').style.display = "none"; } else { document.getElementById('hideShow').style.display = document.getElementById(rowid).style.display } } </script> The PHP code for passing in the parameter should remain the same: <?php echo ... onClick='FuncShowHide(" . $row[0] . ")'> ... ?> -
[SOLVED] using javascript & php togeather
Corona4456 replied to owen.aaron's topic in PHP Coding Help
Well... whether you do it with "" or '' you'll have to make sure and escape the proper character. So if you are using "" then you'll have to escape every " in the string and if you are using '' you'll have to escape every ' in the string. -
[SOLVED] getElementsByTagName('a')[0]; not working? please help.
Corona4456 replied to sayedsohail's topic in Javascript Help
To define the class name for a tag just do the following: var pcss=document.getElementById('tabnavigation').getElementsByTagName('a')[0]; pcss.className = "whateverTheClassNameIs"; -
[SOLVED] getElementsByTagName('a')[0]; not working? please help.
Corona4456 replied to sayedsohail's topic in Javascript Help
I'm assuming you want to get the text within the 'a' tag: alert(pcss.text); -
[SOLVED] need assistance with displaying row of MySQL data with JS
Corona4456 replied to webguync's topic in PHP Coding Help
Yeah that's a typo it should be $row[0] -
[SOLVED] need assistance with displaying row of MySQL data with JS
Corona4456 replied to webguync's topic in PHP Coding Help
You never passed in the row[0] into your function... look over the code again and look at the change made to the function call FuncShowHide(). -
[SOLVED] Convincing Firefox that php output is xhtml
Corona4456 replied to Mike Daly's topic in PHP Coding Help
Also: -
[SOLVED] need assistance with displaying row of MySQL data with JS
Corona4456 replied to webguync's topic in PHP Coding Help
Change the following line: <?php echo"<tr id='trX'><td> $img </td><td> $row[6] </td><td> $row[0]</td><td> $row[1] </td><td id='header'><a href='#' onClick='FuncShowHide()'> More Info.</a></td><td id='header'><a class='WHATSTHIS' onclick='selectLoc([0])' href='javascript:;'>Select this Location</a></td></tr>"; echo"<tr id='hideShow' style='display:none'><td><strong>Address:</strong> $row[3] </td><td><strong>Address:</strong>$row[4] </td><td><strong>City:</strong> $row[5] </td><td> $row[6]</td><td>$row[7] </td><td><strong>Phone:</strong> $row[2] </td></tr>"; } ?> Just an FYI I didn't test this code so there may be some syntax errors or something like that. to <?php echo"<tr id='". $row[0] . "'><td> $img </td><td> $row[6] </td><td> $row[0]</td><td> $row[1] </td><td id='header'><a href='#' onClick='FuncShowHide(" . $row0 . ")'> More Info.</a></td><td id='header'><a class='WHATSTHIS' onclick='selectLoc([0])' href='javascript:;'>Select this Location</a></td></tr>"; echo"<tr id='hideShow' style='display:none'><td><strong>Address:</strong> $row[3] </td><td><strong>Address:</strong>$row[4] </td><td><strong>City:</strong> $row[5] </td><td> $row[6]</td><td>$row[7] </td><td><strong>Phone:</strong> $row[2] </td></tr>"; } ?> Then change your javascript to: <script language="javascript" type="text/javascript"> function FuncShowHide(row){ if (document.getElementById('hideShow').style.display == document.getElementById(row).style.display){ document.getElementById('hideShow').style.display = "none"; } else { document.getElementById('hideShow').style.display = document.getElementById(row).style.display } } </script> -
[SOLVED] Convincing Firefox that php output is xhtml
Corona4456 replied to Mike Daly's topic in PHP Coding Help
the php code worked on my end. -
[SOLVED] need assistance with displaying row of MySQL data with JS
Corona4456 replied to webguync's topic in PHP Coding Help
Ah I see, document.getElementById returns the first element with the id 'trX'. You'll need to figure out a different method of uniquely identifying each row and passing in the unique id into your FuncShowHide() function. My suggestion would be to use the row id from the MySQL table to identify each table row. -
[SOLVED] Convincing Firefox that php output is xhtml
Corona4456 replied to Mike Daly's topic in PHP Coding Help
<?php header("Content-type: application/xhtml+xml"); ?> -
[SOLVED] need assistance with displaying row of MySQL data with JS
Corona4456 replied to webguync's topic in PHP Coding Help
Could you show the javascript? Looks like you are using Ajax for this. Thanks. -
If you are using amaroK you can just use their web interface app: http://www.kde-apps.org/content/show.php?content=31871 Or if using XMMS: http://www.joethielen.com/xmms-control/ Whatever player you are using, odds are there is a web interface implementation somewhere out there .
-
D'oh chigley beat me to it!
-
so are you sure that whatever is stored in $username is in the 'account' table? use 'mysql_error()' to see if you get an error for your mysql_query().