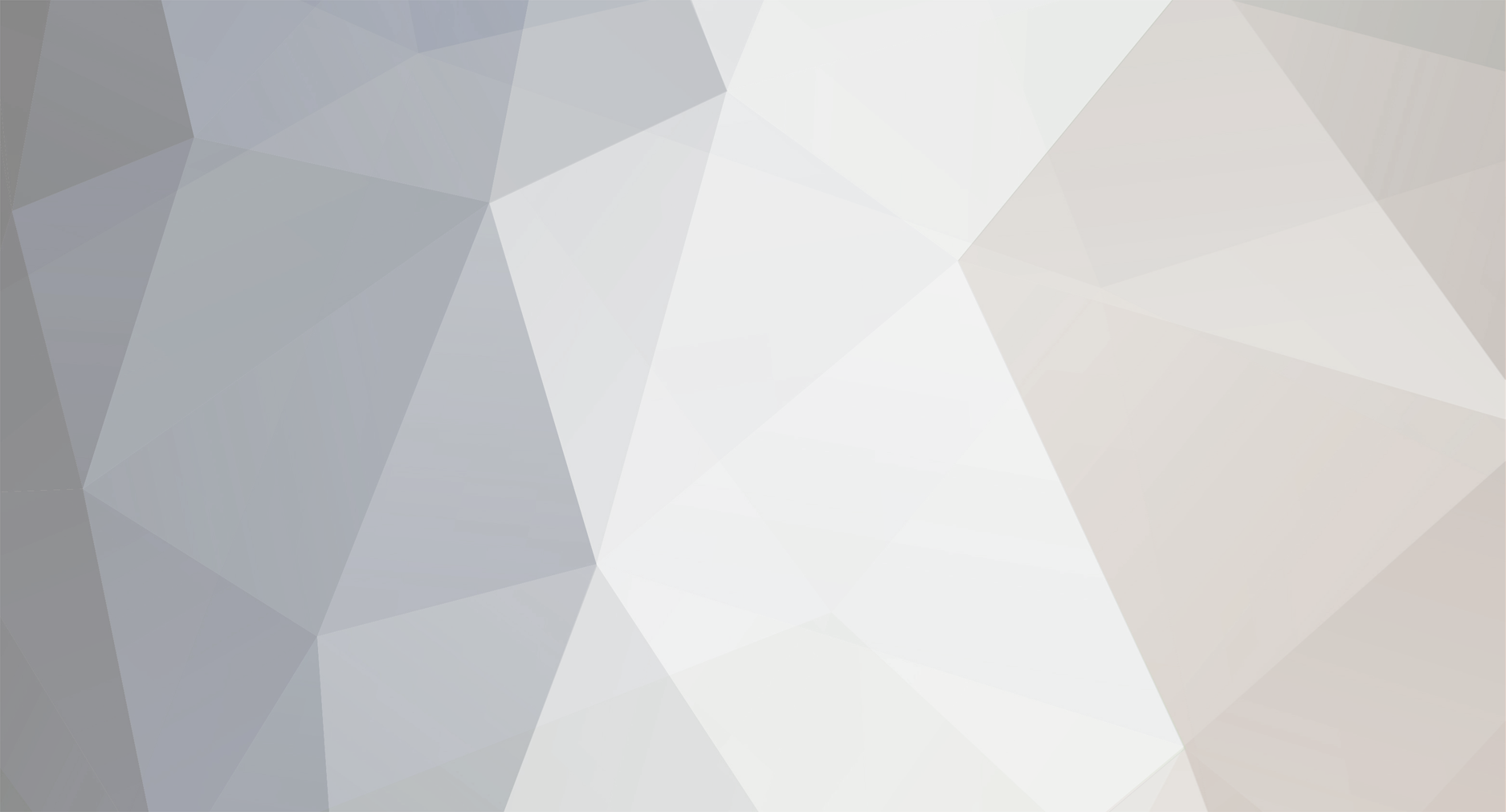
nogray
Members-
Posts
930 -
Joined
-
Last visited
Everything posted by nogray
-
Different timers need to pauze the global page on specific triggers
nogray replied to Darghon's topic in Javascript Help
You can simply declare a global variable called pause and set it to false. When you want to pause everything, set it to true and when the move is finished reset it to false. Varify the pause variable on all your other functions, e.g. var pause = false; my_func(){ if (pause) return; // do other stuff } move_func(){ pause = true; // move code pause = false; } -
If you use setAttribute, you would need to use getAttribute to see the value. e.g. var player = document.createElement( 'audio' ); player.setAttribute('volume', 0.; alert(player.getAttribute('volume')); // alerts 0.8 alert(player.volume); // alerts 1 // setting the volume directly player.volume = 0.8; alert(player.volume); // alerts 0.8
-
OnSubmit versus OnClick... but not the normal question
nogray replied to LLLLLLL's topic in Javascript Help
Newer browsers seem to trigger a click even when the user hit enter key on a form. However, older browsers dont (that's include IE8 and lower). So you should stick on the onsubmit event for now. -
You are using getElementById but your select elements don't have an id attribute. Second, you can't add the time as the format you use in the select values. You'll get three strings put togather instead of the total time. You might want to change the value into the minutes (as numbers) so you can add them togather.
-
The only way to read a file local is via ActiveX (IE only) and the user will get a scary warning every time they load your page.
-
try to change onkeydown to onkeyup or onkeypress
-
Javascript - How can I replace a textarea with its contents?
nogray replied to DeX's topic in Javascript Help
Your code should work fine, but you might have a structure problem. If all the textareas are under the same parent, the parent innerHTML will be the first textarea (after that you'll get an error). So, you HTML should be something like this <div id="purchase-order-area"> <div> <textarea id = "notes5" rows = "3" cols = "105" value = ""></textarea> </div> <div> <textarea id = "notes6" rows = "3" cols = "105" value = ""></textarea> </div> </div> -
Javascript - How can I replace a textarea with its contents?
nogray replied to DeX's topic in Javascript Help
what is "textareaBoxes", are you using getElementsByTagName('textarea')? -
Issue with Visibility and InnerHTML not working IE9
nogray replied to BuildMyWeb's topic in Javascript Help
If you want to use innerHTML for a select menu in IE, you would need to write the whole select tag and populate the div innerHTML e.g. var html = '<select><option>Hello in IE</option></select>'; document.getElementById('my_div').innerHTML = html; Otherwise, you would need to create the options as elements (e.g. using document.createElement) and append them to the select menu. -
First, you would need to use a radio button since the user can only select one option and pass the object to the function because I assume you have more than on table row <input type="radio" value="#FF0000" onclick="changeColor(this);" name="Table_Row_1" /> <input type="radio" value="#FF0000" onclick="changeColor(this);" name="Table_Row_1" /> If your function, you can get the table row by looking up the parents node of the element function changeColor(obj){ var td = obj.parentNode; // table cell var tr = td.parentNode; // table row tr.style.backgroundColor = obj.value; // change the tr background color } NOT TESTED
-
First you are using getElementById('head1') and your input doesn't have an id property. If you have a div with the id "head1", it might cause a conflict because IE uses the names for input fields as variables. You might want to rename it to "head1_div" or something like that. You are trying to show, hide the input checkbox (head1) instead of the form and since it's undefined, you'll get an error.
-
Cant see the difference between these two functions.
nogray replied to Entiranz's topic in Javascript Help
Javascript is case sensative, you declared formInputs and use forminputs -
Regardless of what code you use, the final HTML (produced by JavaScript) is a simple iframe and you can't change it.
-
try $('.'+tag)[effect](0,function(){
-
Countdown timer not working in IE do you know why?
nogray replied to Presto-X's topic in Javascript Help
test with something like this to find the error crossbrowsertesting.com -
Yes, your onclick will work. If you want to make a function, you can do the following <input type="radio" onclick="my_name_change_func('english');" .... ... later in your script... function my_name_change_func(nm){ document.getElementById('pfc_words').name = nm; }
-
You can just use the following document.getElementById('pfc_words').name = 'MY_NEW_NAME'; you don't need jquery or anything else
-
The best way to learn ajax is to play with it. You can use the examples on jquery website or make your own try to create a PHP file as the following <?php echo "Hello Ajax"; ?> and create another HTML file to request the content, create a script to as the following $.get('URL_TO_PHP_PAGE', function(txt){ alert(txt); });
-
getElementsByClassName is not supported in IE before version 9, use getElementsByTagName, e.g. // "first" is the id for the main div that is holding all the input fields var eles = document.getElementById('first').getElementsByTagName('input');
-
You can use innerHTML instead of creating a txt node, e.g. obj.innerHTML = quote[qno] + obj.innerHTML; // append html to the top obj.innerHTML += quote[qno]; // append html to the bottom
-
how to get the current UTC / GMT date? javascript
nogray replied to tastro's topic in Javascript Help
Date.UTC requires the year, month and date to work. e.g. var d = Date.UTC(2012, 2, 26); You can also search for getUTCDate, getUTCMonth, getUTCFullYear, etc...