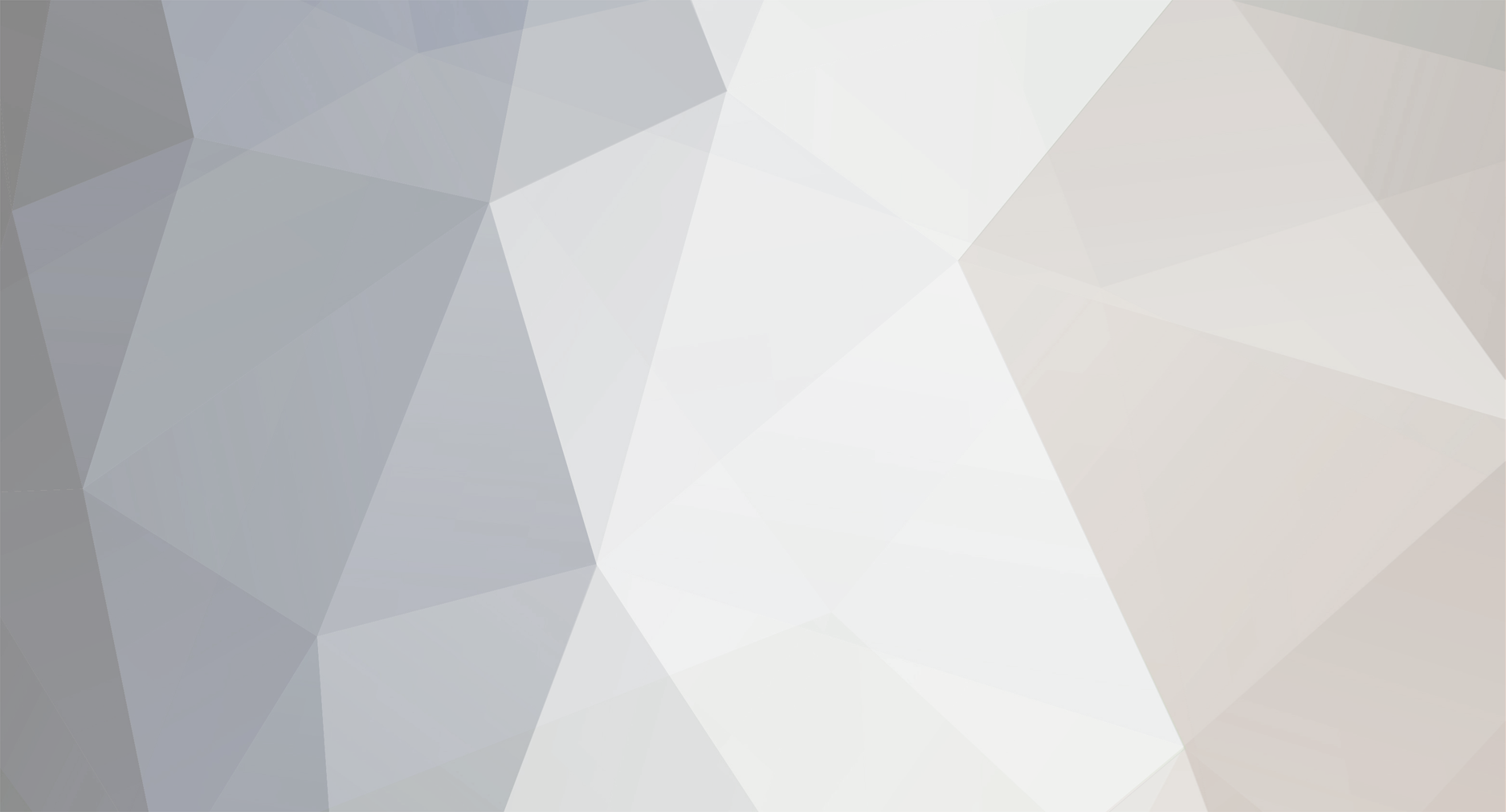
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
foreach with an array with multiple/two indexes?
Psycho replied to galvin's topic in PHP Coding Help
personally I would only iterate over the parent array and use the inner array key values. it makes it more apparent what is being output when reading the code, IMO. foreach($myarray as $ID => $data) { echo "Player {$ID} -- color: {$data['color']}, animal: {$data['animal']}, state: {$data['state']}, food: {$data['food']}"; } Of course you could use the indexes of the child array for the labels but it makes it impossible to "see" what field is what in that case. -
I think you are going to have a tough time of this since you are getting data from so many sources and you can't control heir format. But, it may work out for you. I do have some suggestions though: Since the columns in the input data will not be consistent, you could rely upon the first row headers (if there are any) as another poster suggested. But, if there are no headers or there is no consistent format to them, you can submit the data and specify the columns after you have preprocessed the data. Basically, after they submit the form do your processing and present the data back to the user (yourself) and then select for each column which field in the database it will belong to. So, it is basically a three step process. Step 1 is submitting the data via a form. Step two is processing the data into columns for verification and column selection, and step three is submitting that additional data for input into the database. You could also combine functionality of reading the column headers to preselect the fields in step 2. But, because the data will likely be very "dirty" you definitely want a step to validate the data before actually saving it. Otherwise cleanup will be an arduous task. And, if the data is processed correctly you can easily build in a process to allow you to manually fix it first. EDIT: Doesn't the decimal in your example above represent a 0? If you removed those you would have the wrong number of columns in the data. And there is no need to explode the data before modifying the characters. Do that first - it is more efficient.
-
As ChristianF was saying that foreach loop is not doing what you think it is. It is only creating/resetting a variable called $elem on each ioteration which has nothing to do with the $1d_arr array. But, you are making it more difficult than it needs to be. Can the query result have values that are not in the list 'a', 'b', 'c' AND must you have a result in $1d_arr for each of those? If not, just create the output array dynamically when processing the results. Second, since you are only adding one value at a time, using $array[] = $value is more efficient: $sql = 'SELECT letter, num FROM table'; $result = $db->query($sql); $1d_arr = array(); while($row=mysql_fetch_array($result)) { $1d_arr[$row['letter']][] = $row['num']; } However, if you must have a dimension in the array for all those primary keys and the result from the query may not have values for each one, then you could define each element as an array to begin with: $1d_arr = array('a'=>array(), 'b'=>array(), 'c'=>array()); Or if you just want to define the keys as values in an array and then create the two-dimensional array programmatically you can do this $keys = array('a', 'b', 'c'); $1d_arr = array_fill_keys ($keys, array());
-
I think you meant true. Also, note that all of those "Unset" conditions will give a Notice: Undefined variable error. I know that that isn't the point, but it is good to know anyway. On point #1, you are 100% correct. That is what I meant. Thanks for the catch! On point #2, you are about 80% correct. the empty() function will work, without errors/notifications, on an unset variable the same as the isset() function would. I always try to write code with full error reporting on and ensure no notifications of that type would occur. But, in reality, a production environment likely have a lower threshold for error reporting and would not display those. It would be funny to see what would occur on most sites with full error reporting on.
-
This conversation is riddled with misinformation. The fact that one comparison is faster than another or suggesting to use a function is not appropriate unless and until you know EXACTLY the purpose of the condition to be checked: As stated before == null and === null are very similar, but not exactly the same. For example, if the value to be checked can be an empty string then the first comparison would return true and the latter false. The only way the latter comparison can be false, to my knowledge, is if the variable isn't set or it is explicitly set to null. All of these different comparisons will produce different results for some values. It is important to understand the purpose. For example, if the purpose is to check for a required field in POST data, empty() would be a poor choice since just a single space would cause the validation to pass. But, it could possibly be used in conjunction with trim()ing the value first. So, the discussion needs to first be what is the appropriate validation. Then if there are multiple, correct solutions then the discussion can be what is the most efficient. For illustrative purposes, here is a table showing the results using different comparisons with some possible values:
-
variables from array dissappear in while looped query
Psycho replied to turpentyne's topic in PHP Coding Help
Not only that, but concatenating a bunch of WHERE clauses for conditions against the same field is unnecessary. Just use the "IN" operator. -
That won't work. He needs the three lowest differentials from the last 20 rounds. Using the above approach you might end up with the following results If the user only had 10 rounds with the above differential scores the lowest scores would not be at the top of the result set. I actually tried doing a query on the lowest differentials using a sub-query of the last 20 rounds. But, I couldn't get it to work. The above approach works. It may not be the most efficient, but it would work. I don't think it would add an significant performance issues - if any at all. But, I would like to see a query only solution if someone has one.
-
Yep, I changed some variable names halfway through when I realized what they really contained. Missed updating that one. Thx.
-
I had initially stated the same thing in my reply/code, but then changed it. In the output to the user he wants to display the total rounds back to the user. But, for the purposes of the calculations only the last 20 are needed.
-
Although I believe I understand what you are trying to achieve, I'm not really understanding what your exact question is. But, based upon what I understand, this is what I would do. //Get the users total rounds $query = "SELECT COUNT(scoreID) FROM scores WHERE userID='$user_id'"; $result = mysql_query($query); $total_rounds = mysql_result($result, 0); if($total_rounds<5) { $scoreMsg = "You have {$total_rounds} of 5 rounds entered to complete your handicap index."; } else { //Determine how many scores will be used if($total_rounds<7) { $score_count = 1; } elseif($total_rounds<9) { $score_count = 2; } elseif($total_rounds<11) { $score_count = 3; } elseif($total_rounds<13) { $score_count = 4; } elseif($total_rounds<15) { $score_count = 5; } elseif($total_rounds<17) { $score_count = 6; } elseif($total_rounds<18) { $score_count = 7; } elseif($total_rounds<19) { $score_count = 8; } elseif($total_rounds<20) { $score_count = 9; } else { $total_rounds = 10; } //Get the differenential from (up to) the last 20 rounds $query = "SELECT differential FROM scores WHERE userID='$user_id' ORDER BY date DESC LIMIT 20"; $result = mysql_query($query); //Put differential scores into array $score_results = array(); while($row = mysql_fetch_assoc($result)) { $score_results[] = $row['differential']; } //Sort the scores, lowest to highest sort($score_results); //Get the slice of the array with only the scores we want $score_results = array_slice($score_results, 0, $score_count); //Calculate the sum of the scored $handicap = array_sum($score_results) / $score_count; $scoreMsg = "You have {$total_rounds} rounds entered<br/><br/>\n"; $scoreMsg .= "Your HANDICAP INDEX is now: {$handicap}"; }
-
Then you should be using the User ID in the posts table to associate the post back to the user - not the username. As for your question, I mean "forum_replies". Your names are really obtuse in my opinion. Well, what you are trying to accomplish is hard because you are not doing it correctly. Your queries and structure are very disorganized. I see a lot of problems. I was going to try and give you some tips, but to be honest I would just rewrite that from scratch.
-
Does the members table have an id field? If so, you should be using that instead of the username. And what is "forum_replies" - is that the number of "forum_answer"'s associated with the forum post? And, I'm not understandin this Are you trying to update a value in the user's table when they make a post? If so, you are doing this completely wrong. You should really go back and read some tutorials on databases. You can very easily get a users post count by JOINing the posts table on the user table and having the database do the calculation for you. Here is an example of a query to return each user along with their associated post count from a properly structured DB. The table/field names are not specific to your structure. SELECT users.name, COUNT(posts.user_id) AS post_count FROM users LEFT JOIN posts ON posts.user_id = users.id GROUP BY users.id
-
Well, what you have is a complete mess. I tried making sense of it and just gave up. Why don't you give some details about your table structures and what you are trying to achieve.
-
Perhaps one has something to do with the other. When you write sloppy code you will get sloppy results. You have three nested while loops that are running queries. For one, you should not have queries nested in loops - learn how to do JOINs in your queries. But the way you have them structure looks completely off.
-
You can't use "NOW()" as the default, but you can use "CURRENT_TIMESTAMP" CREATE TABLE news (time timestamp NOT NULL default CURRENT_TIMESTAMP, news varchar(10000)); But, if you are going to have a field with a default, what's the point of having to include an empty value when creating the record? Just don't include the field in the insert. Include the field list that you are inserting for and the associated values. INSERT INTO news (news) VALUES ('$newnews'); And, for what it's worth, I would suggest not having a field name and a table name with the same value. Only creates confusion in my opinion.
-
Um, the mouse-wheel works just fine for me as an intuitive, simple solution when needing to scroll up and down. As for side-to-side, the vast majority of sites build their pages in such a manner that the content will expand/collapse to fit the available width. Ones that don't are not very good at what they do. But, if you really want to do that go right ahead. I have no idea where Google employs such a feature. But, if they do, perhaps you should spend more time using the primary feature of Google (searching) instead of looking for flashy features that provide no real value. because, I find it impossible to believe that you even did a cursory search for this feature on Google. My first search brought up tons of examples, code examples, etc., etc.
-
While I whole heartily agree that you should stick to standard form elements, the above is not entirely true. It is perfectly valid to use an image as a submit button with standard HTML <input type="image" src="images/submit.gif" height="30" width="173" border="0" alt="Submit Form">
-
Yes, try another approach. Disabling the right-click within a web page is something that was routinely used in the 90's to try and "secure" the page from those wanting to see the code, copy images, etc. It is easily circumvented and only frustrated users that used the context menu (e.g. for printing, saving a bookmark, etc.). The context menu is a built in feature of the Operating System/Application. By disabling a feature that people expect, and possibly rely on, for the ability to scroll in a DIV will not be seen as an enhancement.
-
Absolutely not! There are multiple threads on this same subject and the details are very involved so I'm not going to open that can of worms here. But, here's some of the problems I see: 1. Your end results contains fixed values ($hash, 'L08Jh', and '3Kjn', '$""', and sha1($hash)) that have nothing to do with increasing the security of the resulting value 2. This is the most egregious, you actually put un-hashed content of the password into the output ($randomText1 and $randomText2). If someone identifies those as coming from the password it would be very easily to identify a lot of users' passwords from your result. Using a dictionary check. 3. The only real hash of the password is md5($string). There are far too many rainbow tables available for MD5() and it is too easy for someone to try and brute force. You should be using a hash such as MD5 or SHA1 along with an appropriate custom salt for each value. That way an attacker could still try and brute force, but they would have to brute force each value independently.
-
Dropdown select submit and placing variables also syntax help
Psycho replied to Bill Withers's topic in PHP Coding Help
The approach you have implemented requires many more queries and is not good practice in my opinion. I gave you what I believe is a more efficient method. I'm not going to spend my time to review code which I believe is flawed to begin with. But, this is your site, so go with it if it suits you. -
Dropdown select submit and placing variables also syntax help
Psycho replied to Bill Withers's topic in PHP Coding Help
I guess your original question was not very clear. On rereading your first post I think maybe you are wanting the number of select fields and the processing to be dynamic based upon the number of tables you want utilized. All you need to do is create one block of code to create ONE select field and create one block of code to process that data. Then just create an array of the tables and put your blocks within a foreach loop. Have have written and tested the following code against two sample tables. I think this is what you are trying to achieve. If not, please be more specific. To test this you should only need to modify the array at the very top to ensure you have a valid table name as the key of each item and an associated label. Each table listed must have fields labeled 'id' and 'name'. <?php //Create array of table names as keys and labels as values $tables = array( 'Places' => 'Place', 'color' => 'Color'); //Function to create the options list. //The $dataResult is expected to be a DB //Result set with fields named 'id' and 'name' function createOptions($dataResult) { $optionsIDs = array(); $optionsHTML = ''; //Process result set into options //echo mysql_num_rows($dataResult); while($row = mysql_fetch_assoc($dataResult)) { $optionsIDs[] = $row['id']; $optionsHTML .= "<option value='{$row['id']}'>{$row['name']}</option>\n"; } //Get a random ID from the records $randomID = $optionsIDs[array_rand($optionsIDs)]; $optionsHTML = "<option value='{$randomID}'>Random</option>\n" . $optionsHTML; return $optionsHTML; } //Connect to database mysql_connect('localhost', 'root', ''); mysql_select_db('test'); $output = ''; if(isset($_POST['selections'])) { //User submitted for, display selected vaues $output .= "<b>Your selections:</b><br>\n"; foreach($_POST['selections'] as $table => $idValue) { //Query the value based upon selected ID $query = "SELECT name FROM {$table} WHERE id = '{$idValue}' LIMIT 1"; $result = mysql_query($query); $value = mysql_result($result , 0); $output .= "{$tables[$table]}: $value<br>\n"; } $output .= "<br><br><a href=''>Change Selections</a>\n"; } else { //Display the form $output .= "<form action='' method='post'>\n"; foreach($tables as $table => $label) { $query = "SELECT id, name FROM {$table}"; $result = mysql_query($query) or die(mysql_error()); $placesOptions = createOptions($result); $output .= "{$label} \n"; $output .= "<select name=\"selections[{$table}]\">"; $output .= $placesOptions; $output .= "</select><br><br>\n"; } $output .= "<button type='submit'>Submit</button>\n\n"; $output .= "</form>\n"; } ?> <html> <head></head> <body> <?php echo $output; ?> </body> </html> -
Change this }elseif($name == 'Bind') $return = self::$Stmt->bind_param(implode(', ', $arg)); To this }elseif($name == 'Bind') echo implode(', ', $arg); //$return = self::$Stmt->bind_param(implode(', ', $arg)); And you should see the problem. When you implode the values you are providing a SINGLE string to the function and none of the values are even encapsulated in quotes! So it would be something like this $return = self::$Stmt->bind_param(format, value1, value2, value3); instead of $return = self::$Stmt->bind_param('format', 'value1', 'value2', 'value3'); But, I don't think just adding the quotes will work, but I think there's an easier way. Just remove the formats parameter from the array for the first argument and pass the remaining for the values. Thy this: $return = self::$Stmt->bind_param(array_shift($arg), $arg));
-
Is the currency symbol the only thing that would be different? For example, could the value be a decimal such as $44.32? What about grouping, e.g. 1,234.56? Will the value always have a space before and after it? Can a space be between the currency symbol and the first digit, e.g. $ 12? All of these are very important considerations when developing the right solution. As for the currency symbol you would have to define a list of the currency symbols that would be used. But, I'm not going to take time to write anything without answers to the above.
-
You are also going to have a problem in the update functionality once you fix that because you would have no way to know which entry the user submitted for update. If you want the user to be able to update all the records at once you want ONE form encompassing all the input fields for all records. In that case I would use input fields named as array with the unique id of the record as the index. If you want the user to only update one record at a time then you can create individual forms for each and just use a hidden field for the record id. Right now your update query is using what appears to be the ID of the user - which would update all the records of the user based upon the content of one set of input fields.
-
That is not a Get method, that is the superglobal $_GET array. Two completely different things. The reason you are probably not getting the results you expect is due to the semicolon at the end of the condition. That terminates that line. So, the next line with the echo will always execute. You should have used if ($_GET['insect_slug'] === ($insect['insect_slug'])) echo $insect['insect_mdes']; Personally, I hate that method of if/else statements I always wrap the code associated with my conditions within curly braces for readability if ($_GET['insect_slug'] === ($insect['insect_slug'])) { echo $insect['insect_mdes']; } But, there is nothing inherently wrong with your usage there. But, depending on how those values are determined and what they actually contain I can't say whether they would work for your needs. If you have a situation where values do or do not compare as you think they should then you need to verify the contents of those values.