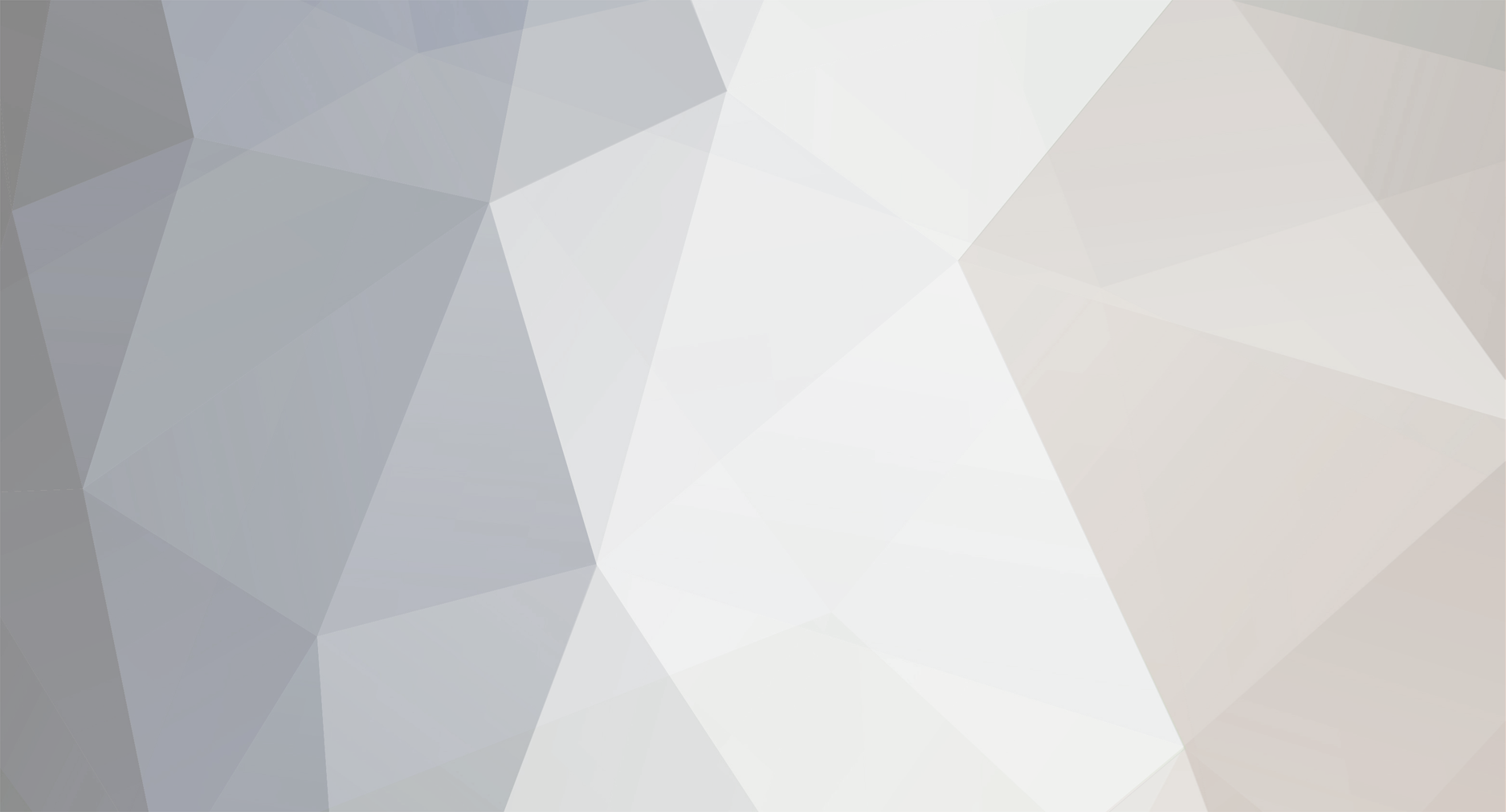
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
$dirPath = './live'; function mtime_sort($a, $b) { if (filemtime($a) == filemtime($b)) { return 0; } return (filemtime($a) > filemtime($b)) ? -1 : 1; } //Get PDF files from directory and sort $dirFiles = glob($dirPath.'/*.pdf'); usort($dirFiles, 'mtime_sort'); foreach($dirFiles as $file) { echo "<li><a href='".$file."'>".substr($file, 0, -4)."</a></li>\n"; }
-
I did a quick Google search for "filemtime stat failed". The first result was to a post on this forum for the same issue and the second result was to the PHP manual for that function which also has a reference to the error. Both results would give you the reason for the error. Per the manual for filemtime() Note the text I colored in red. Your function to get the file list readdir() returns the following: You need the complete path and file name for filemtime(). I woud suggest using glob() to get the files because 1) It returns the complete path AND 2) you can set it to only get the .pdf files by default and not need to check the extensions AND 3) It makes the code much more compact.
-
No, not simpler. YOU need to decide what makes sense for the problem at hand. If you want to put all the files in one directory it definitely makes the code simpler. But, if you don't that's fine too. You would just need to modify the code accordingly. A recursive function would make sense if all the files are in directories under a parent directory. But, if there are many other directories under that same parent that are not for music files that probably won't make sense. In that case, I'd define the "music" directories within an array and then process each of those in the code. But, on further consideration, I would ask how often you expect the files to change? It seems a waste to regenerate the javascript data for hundreds of files every time you load the web page. It might make sense to instead create a script to process the files and write a file with the data needed by the JavaScript. Then, include that code into the HTML page when you load it. If your music files change then rerun the processing script.
-
Do you have an mp3 AND an ogg version of EVERY file? if so, then just do a search for mp3 (or ogg) files and build the output from that. If not, then you will need to process the jpb and ogg files into an array or some structure to verify those that are paired up and those that aren't. Then either create output only for those that are paired or allow them to be in the output as an unmatched pair. A couple other things. your logic int he while loop is overly complicated. Just check for the file types you are interested in instead of trying to program around all the other types. Also, the sort() function only returns true/false. It will sort the original array. So, the following lines that try to foreach() over the result will fail (unless I am reading something wrong) This might get you closer //Define directory to scan $directory='./music/'; //Define acceptable file types $extensions = array('jpg', 'ogg'); $filesArray = array(); $dir_handle = @opendir($directory) or die("There is an error with your file directory!"); while ($file = readdir($dir_handle)) { $filePath = $directory.$file; $info = pathinfo($filePath); if(in_array(strtolower($info['extension'])), $extensions) { $filesArray[$info['filename']][$info['extension']] = $filePath; } } sort($files_array, SORT_STRING); $path_parts = pathinfo('musicogg'); foreach ($filesArray as $fileName => $files) { if(count($files)==2) { echo "{mp3:\"{$files['mp3']}\",ogg:\"{$files['ogg']}\"}\n"; } }
-
You're right. Myself and all the professionals I work with don't have a clue.
-
You are totally off base here and completely naive. I work for a multinational company that serves markets worldwide. We offer software and other technology solutions in multiple market segments. In addition to Desktop and browser-based solutions (ironically none of which are PHP) we also have mobile apps. But, you know what, our web sites and browser apps are not "device aware" to support mobile devices. Why? Because it doesn't make good business sense to do so. The types of browser-applications we host and current usage by our customers shows that mobile devices make up a miniscule percentage of our traffic. To support ALL browser and ALL devices is no trivial task. Just for our mobile apps we have to support a minimum of four different style sheets: android phone, android tablet, iPhone and iPad (and soon we'll be adding support for Win Phone and Tablets). But, even those don't work great for the Galaxy Note (a 7" tablet). The phone style sheet is not big enough and the Tablet style sheet is too big. Add to that the many different idiosyncrasies with mobile operating systems, browsers (especially JS support), connection speeds, number of cores, etc. etc. In fact, I had a meeting last week with a vendor regarding testing of our mobile apps. They had labs with over 1,400 devices! I never stated that one should not support mobile devices, but the extent to which you will support them is wholly dependent upon your user base and how they will use the application. The extent that you will support them is a business decision that needs to be made in order to know how much resources to invest in those solution. To simply state that you will support all of them is foolish.
-
I'm not understanding everything you said. Especially about It looks like the fields would always be in one directory. Anyway, I expect your problem is that the curly braces are in the wrong positions. Also, why hardcode the directory in that line when you made the directory a variable above? echo "mp3: \"{$directory}{$file_name}\"<br>\n";
-
How so? You can just as easily put images in directories that are not accessible via the internet. Since you would have to look up the image record in the DB to get the path/name you can just as easily control access as you could if the images were in the database. I'm not trying to argue, just trying to understand the logic behind such a blanket statement.
-
Hmm . . . could you provide some reasoning for that statement? I'm not following that.
-
There is no one right answer to this - it all depends on context. In a business environment, you would want to start with the actual functionality of the site. When a developer/team needs to show value to the organization for what they are doing demo'ing a login would not go too far. Heck, even if it is a personal project you may want to see if what you are trying to accomplish is even worthwhile before spending time developing login/registration functionality. Many times I'll have a config script that is included with every page load and put something like $userID = 1; and use that in my script whenever needing to reference the user id. I can easily go back and implement a login process that checks for valid login and sets that variable accordingly.
-
How to sort data without javascript while Php scraping
Psycho replied to Nuv's topic in PHP Coding Help
Actually, Salathe found the trick to get all the results on one page - which is definitely the best route.. But, what I provided earlier was still valid and would be necessary if the site didn't have the option to get all the results in one page. So, just to elaborate on what I was suggesting: You replied: When I clicked a link to go to another page the URL would change as follows: http://www.google.de/products/catalog?hl=de&q=4242002690209&cid=2594634728159287170 http://www.google.de/products/catalog?hl=de&q=4242002690209&cid=2594634728159287170#start=10 http://www.google.de/products/catalog?hl=de&q=4242002690209&cid=2594634728159287170#start=20 So, if you had to, you could increase the #start=nn and iteratively grab one page at a time using file_get_contents() until no new records were begin generated. until you had all the records. But, thankfully, you don't need to do that. -
How to sort data without javascript while Php scraping
Psycho replied to Nuv's topic in PHP Coding Help
Click the Page Next button and look at how the URL changes. You will need to scrape multiple pages to get what you need. -
OK, if you are talking about supporting mobile devices then that aspect alone should be your criteria, IMHO. A mobile device has limited screen real-estate and you should limit the number of photos on that rather than their connection speed. In addition to limiting the number of records per page you can also implement different style sheets for mobile vs. desktop.
-
I don't think there is any accurate way to accomplish this. But, changing the number of records per page will have little performance benefit unless each record has a lot of images displayed with it. One image "normal" sized image will typically be larger than all of the HTML code.
-
How to sort data without javascript while Php scraping
Psycho replied to Nuv's topic in PHP Coding Help
Grab the contents of the page, put the relevant data into a multi-dimensional array, sort the array as you please. -
That is an ASSIGNMENT operator not a comparison. So, if the PHP parser is able to assign 'iu' to the variable $user_reason then the condition passes. In other words, it would always be true. You should be using $user_reason == "iu" Note the double equal sign.
-
@gerkintrigg, In case you didn't catch the meaning from the last few posts, the `book_id`=`book_id` should come out of the query since it is providing no value. I also prefer to use explicit JOINs as it makes reading the query and debugging easier. In the original query I'm pretty sure that it would exclude books that have had no sales. If you want to include those, then you should use a LEFT JOIN. But, if you do want to exclude books with no sales, then use a normal JOIN. Lastly, you prefaced the table name before each field name - except the "currency" field. I'm assuming it is from the "book_purchases". Again, for consistency and readability I would incude the table name there as well. I'd suggest the following: SELECT `books`.`title`, `books`.`id`, SUM(`book_purchases`.`price`) as `book_sales_total` FROM `books` LEFT JOIN `book_purchases` ON `books`.`id` = `book_purchases`.`book_id` WHERE `book_purchases`.`currency`= 'p' GROUP BY `book_purchases`.`book_id` ORDER BY `book_sales_total` DESC
-
Well, the description for this forum is But, you haven't written any code and are asking about the "fastest" way to do something. So, are you expecting us to try a bunch of different methods and benchmark them for your benefit? Did you even do a simple Google search for what you want to do? I have to assume not since the very first search I did, I found a page which provided code for doing what you want. Is it fast? I don't know - go do your own work.
-
As my signature states, I do not always test the code I provide. It would take too much time to try and set up the appropriate sample data, databases, etc. to do so for every post. The code I provide is typically meant to be used as guidance on developing a full-fledged solution. I expect that the person receiving my code will perform any basic debugging to fix any minor typos. And, that was the case here: $questionNo++ $length = ($row["answer_length"]!=0) ? $row["answer_length"] : $answerSize; Line 24 needs a semi-colon at the end. Also, If you have an error, don't post that you have an error and leave it at that. Provide the actual error message.
-
You may use PHP to connect to and execute queries. But one does not necessitate the other. You can use other databases with PHP and you can use different web based scripting languages with MySQL. They are completely different technologies. If you used that logic then you might as well post questions about setting up an Apache server in here since you can run PHP on it. Or even post HTML, CSS, and JavaScript questions here since you can create the output for those using PHP. We have dedicated forums for specific technologies to help the posters and the helpers. There are multiple dedicated forums here specifically for databases. PHP is a scripting language. It only provides the "hooks" in order to interact with a database. There is nothing in the PHP manual about how to use databases. Forum DO #5
-
Here are some things off the top of my head: 1. Never trust ANY input from the user: POST, GET, COOKIE, files, etc. Just because you have a select list doesn't mean the user will always be submitting a value from that select list. So, always analyze what should be a valid input and escape/validate accordingly. "How" you do that will be dependent upon how the data will be used and the type of data. See #2 2. Always escape/sanitize the data based upon context. You need to escape data differently based upon how it is used. It would be different for a DB query vs. displaying in the web page vs in an XML file. Some things to prevent: SQL Injection and XSS scripting. 3. Secure your files. You will likely have many files that are not meant for direct access (i.e. they are used as includes). You can put those in a directory that is not in the web root, use HTTACCESS files or put a check at the top of the file to prevent direct access. 4. Always verify access. If you have content that should only be shown to certain users you would want to check that security to determine if the links should be shown AND you should do the same before displaying the content. Never rely upon COOKIE data for storing credentials.
-
Um, what? Is this a PHP question? If you can't take the time to write a clear, concise question I see no reason for anyone to provide a response.
-
Right. So when you create a post record you would ONLY store the user_id in the forum table (called a foreign key reference). Then when you want to display the post along with the user info you select the record(s) from the forum table and JOIN the user's name and avatar (etc.) from the users table. It might look something like this: SELECT forum.title, forum.text, forum.post_date, users.user_id, users.username, users.avatar FROM forums JOIN users ON forums.user_id = users.user_id WHERE forum.forum_id = 12 -- Or whatever id of the post you want to get As stated above, this is basic database functionality. This is NOT the place to be given an education on this. I provided a link above that would get you started. There are tons of other resources available. Go start learning. Then, if you have a specific problem or question go ahead and post in the appropriate forum here (this is the PHP forum).
-
There is no way for us to answer your question from what you have provided. We need to know the "context" of what you are trying to achieve. JOIN would probably be the solution for most situations. But, in others a UNION might be correct. But, JOINs have many, many ways to be used. So, just saying "JOIN" is the answer is understating how it would be used. Not only are there many types of JOINs but how you would define the JOIN is significant. For example, this SELECT * FROM users LEFT JOIN forum would likely NEVER be used. Why? Because it JOINs every forum record on every user record. That wouldn't make any sense. It all depends on what you are trying to achieve. If you wanted to get information about users from the users table and you wanted a list of the posts each user has made you might have a query such as this: SELECT * FROM users LEFT JOIN forum ON users.id = forum.user_id Trying to explain JOINs in a forum is not feasible. There is many resources available to understand how to effectively set up and utilize a database. Tizag has some good tutorials. They are not very in-depth, but they will give you the very basics from which to start learning: http://www.tizag.com/mysqlTutorial/ NOTE: Although I used '*' in my example (for brevity), that is a poor practice in my opinion, and should almost never be used. Instead you should explicitly list out the fields you want. Explaining why would take more time than I am willing to take here.